Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / XamlRtfConverter.cs / 1 / XamlRtfConverter.cs
//---------------------------------------------------------------------------- // // File: XamlRtfConverter.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Xaml-Rtf Converter. // //--------------------------------------------------------------------------- using System.IO; using System.Text; namespace System.Windows.Documents { ////// XamlRtfConverter is a static class that convert from/to rtf content to/from xaml content. /// internal class XamlRtfConverter { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// create new instance of XamlRtfConverter that convert the content between xaml and rtf. /// internal XamlRtfConverter() { } #endregion Constructors // ---------------------------------------------------------------------- // // Internal Methods // // --------------------------------------------------------------------- #region Internal Methods ////// Converts an xaml content to rtf content. /// /// /// The source xaml text content to be converted into Rtf content. /// ////// Well-formed representing rtf equivalent string for the source xaml content. /// internal string ConvertXamlToRtf(string xamlContent) { // Check the parameter validation if (xamlContent == null) { throw new ArgumentNullException("xamlContent"); } string rtfContent = string.Empty; if (xamlContent != string.Empty) { // Creating the converter that process the content data from Xaml to Rtf XamlToRtfWriter xamlToRtfWriter = new XamlToRtfWriter(xamlContent); // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { xamlToRtfWriter.WpfPayload = WpfPayload; } // Process the converting from xaml to rtf xamlToRtfWriter.Process(); // Set rtf content that representing resulting from Xaml to Rtf converting. rtfContent = xamlToRtfWriter.Output; } return rtfContent; } ////// Converts an rtf content to xaml content. /// /// /// The source rtf content that to be converted into xaml content. /// ////// Well-formed xml representing XAML equivalent content for the input rtf content string. /// internal string ConvertRtfToXaml(string rtfContent) { // Check the parameter validation if (rtfContent == null) { throw new ArgumentNullException("rtfContent"); } // xaml content to be converted from rtf string xamlContent = string.Empty; if (rtfContent != string.Empty) { // Create RtfToXamlReader instance for converting the content // from rtf to xaml and set ForceParagraph RtfToXamlReader rtfToXamlReader = new RtfToXamlReader(rtfContent); rtfToXamlReader.ForceParagraph = ForceParagraph; // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { rtfToXamlReader.WpfPayload = WpfPayload; } //Process the converting from rtf to xaml rtfToXamlReader.Process(); // Set Xaml content string that representing resulting Rtf-Xaml converting xamlContent = rtfToXamlReader.Output; } return xamlContent; } #endregion Internal Methods // ---------------------------------------------------------------------- // // Internal Properties // // ---------------------------------------------------------------------- #region Internal Properties // ForceParagraph property indicates whether ForcePagraph for RtfToXamlReader. internal bool ForceParagraph { get { return _forceParagraph; } set { _forceParagraph = value; } } // WpfPayload package property for getting or placing image data for Xaml content internal WpfPayload WpfPayload { get { return _wpfPayload; } set { _wpfPayload = value; } } #endregion Internal Properties // --------------------------------------------------------------------- // // Internal Fields // // ---------------------------------------------------------------------- #region Internal Fields // Rtf encoding codepage that is 1252 ANSI internal const int RtfCodePage = 1252; #endregion Internal Fields // --------------------------------------------------------------------- // // Private Fields // // --------------------------------------------------------------------- #region Private Fields // Flag that indicate the forcing paragragh for RtfToXamlReader private bool _forceParagraph; // The output WpfPayload package for placing image data into it private WpfPayload _wpfPayload; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlRtfConverter.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Xaml-Rtf Converter. // //--------------------------------------------------------------------------- using System.IO; using System.Text; namespace System.Windows.Documents { ////// XamlRtfConverter is a static class that convert from/to rtf content to/from xaml content. /// internal class XamlRtfConverter { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// create new instance of XamlRtfConverter that convert the content between xaml and rtf. /// internal XamlRtfConverter() { } #endregion Constructors // ---------------------------------------------------------------------- // // Internal Methods // // --------------------------------------------------------------------- #region Internal Methods ////// Converts an xaml content to rtf content. /// /// /// The source xaml text content to be converted into Rtf content. /// ////// Well-formed representing rtf equivalent string for the source xaml content. /// internal string ConvertXamlToRtf(string xamlContent) { // Check the parameter validation if (xamlContent == null) { throw new ArgumentNullException("xamlContent"); } string rtfContent = string.Empty; if (xamlContent != string.Empty) { // Creating the converter that process the content data from Xaml to Rtf XamlToRtfWriter xamlToRtfWriter = new XamlToRtfWriter(xamlContent); // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { xamlToRtfWriter.WpfPayload = WpfPayload; } // Process the converting from xaml to rtf xamlToRtfWriter.Process(); // Set rtf content that representing resulting from Xaml to Rtf converting. rtfContent = xamlToRtfWriter.Output; } return rtfContent; } ////// Converts an rtf content to xaml content. /// /// /// The source rtf content that to be converted into xaml content. /// ////// Well-formed xml representing XAML equivalent content for the input rtf content string. /// internal string ConvertRtfToXaml(string rtfContent) { // Check the parameter validation if (rtfContent == null) { throw new ArgumentNullException("rtfContent"); } // xaml content to be converted from rtf string xamlContent = string.Empty; if (rtfContent != string.Empty) { // Create RtfToXamlReader instance for converting the content // from rtf to xaml and set ForceParagraph RtfToXamlReader rtfToXamlReader = new RtfToXamlReader(rtfContent); rtfToXamlReader.ForceParagraph = ForceParagraph; // Set WpfPayload package that contained the image for the specified Xaml if (WpfPayload != null) { rtfToXamlReader.WpfPayload = WpfPayload; } //Process the converting from rtf to xaml rtfToXamlReader.Process(); // Set Xaml content string that representing resulting Rtf-Xaml converting xamlContent = rtfToXamlReader.Output; } return xamlContent; } #endregion Internal Methods // ---------------------------------------------------------------------- // // Internal Properties // // ---------------------------------------------------------------------- #region Internal Properties // ForceParagraph property indicates whether ForcePagraph for RtfToXamlReader. internal bool ForceParagraph { get { return _forceParagraph; } set { _forceParagraph = value; } } // WpfPayload package property for getting or placing image data for Xaml content internal WpfPayload WpfPayload { get { return _wpfPayload; } set { _wpfPayload = value; } } #endregion Internal Properties // --------------------------------------------------------------------- // // Internal Fields // // ---------------------------------------------------------------------- #region Internal Fields // Rtf encoding codepage that is 1252 ANSI internal const int RtfCodePage = 1252; #endregion Internal Fields // --------------------------------------------------------------------- // // Private Fields // // --------------------------------------------------------------------- #region Private Fields // Flag that indicate the forcing paragragh for RtfToXamlReader private bool _forceParagraph; // The output WpfPayload package for placing image data into it private WpfPayload _wpfPayload; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
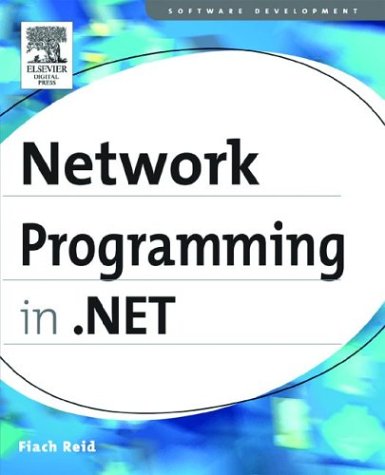
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmissiveMaterial.cs
- AnnotationAuthorChangedEventArgs.cs
- NetworkInterface.cs
- KeyedCollection.cs
- Identity.cs
- AssertSection.cs
- PackUriHelper.cs
- ListViewTableCell.cs
- IssuedTokenParametersElement.cs
- RowParagraph.cs
- XmlSchemaChoice.cs
- IPHostEntry.cs
- MouseDevice.cs
- CellTreeNode.cs
- ManagementException.cs
- EventLogEntryCollection.cs
- PrintDialogDesigner.cs
- TextEditorParagraphs.cs
- DynamicEndpointElement.cs
- HwndSource.cs
- WpfXamlLoader.cs
- GridViewHeaderRowPresenter.cs
- HttpSessionStateBase.cs
- XPathItem.cs
- SiteMapSection.cs
- StandardMenuStripVerb.cs
- NonClientArea.cs
- TextBox.cs
- ResourcePermissionBaseEntry.cs
- Switch.cs
- HeaderedItemsControl.cs
- ObjectView.cs
- HatchBrush.cs
- ForeignConstraint.cs
- columnmapfactory.cs
- TextTreeTextElementNode.cs
- TokenizerHelper.cs
- AsyncPostBackErrorEventArgs.cs
- CapacityStreamGeometryContext.cs
- RuntimeWrappedException.cs
- SR.cs
- LinqDataSourceValidationException.cs
- FormsAuthenticationCredentials.cs
- ParenExpr.cs
- InstanceLockException.cs
- ActivityScheduledQuery.cs
- DataKey.cs
- TreeNodeSelectionProcessor.cs
- CellRelation.cs
- SendingRequestEventArgs.cs
- AttributeUsageAttribute.cs
- TimelineCollection.cs
- ProxyElement.cs
- RoutedEventValueSerializer.cs
- Timer.cs
- LambdaCompiler.ControlFlow.cs
- ValueUtilsSmi.cs
- SchemaImporterExtensionsSection.cs
- UserPreferenceChangingEventArgs.cs
- AnimationClock.cs
- EncoderParameters.cs
- ClientScriptItemCollection.cs
- CommandEventArgs.cs
- Attributes.cs
- CheckBoxFlatAdapter.cs
- DispatcherExceptionEventArgs.cs
- KeyConstraint.cs
- DefinitionUpdate.cs
- DataSourceXmlTextReader.cs
- FormViewRow.cs
- ByteStreamMessageEncoderFactory.cs
- ReferenceConverter.cs
- AlphaSortedEnumConverter.cs
- MenuItem.cs
- OleDbCommandBuilder.cs
- TextSelectionHighlightLayer.cs
- Byte.cs
- EmptyReadOnlyDictionaryInternal.cs
- StylusDevice.cs
- TdsParserSafeHandles.cs
- InputEventArgs.cs
- BidOverLoads.cs
- HwndMouseInputProvider.cs
- RequestCachePolicyConverter.cs
- EmulateRecognizeCompletedEventArgs.cs
- IIS7UserPrincipal.cs
- DataChangedEventManager.cs
- AssociationProvider.cs
- ImmutablePropertyDescriptorGridEntry.cs
- Menu.cs
- Rotation3DAnimation.cs
- EventMap.cs
- CompilationLock.cs
- UInt16.cs
- CorePropertiesFilter.cs
- DeviceContexts.cs
- LexicalChunk.cs
- ExtensionDataObject.cs
- GlyphRun.cs
- XsltException.cs