Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / AtomEntry.cs / 1407647 / AtomEntry.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to represent an ATOM entry as parsed and as it // goes through the materialization pipeline. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Reflection; using System.Xml; using System.Xml.Linq; using System.Text; #endregion Namespaces. ////// Use this class to represent an entry in an ATOM payload as it /// goes through the WCF Data Services materialization pipeline. /// ////// Different properties are set on instances of this type at different /// points during its lifetime. /// [DebuggerDisplay("AtomEntry {ResolvedObject} @ {Identity}")] internal class AtomEntry { #region Private fields. ///Entry flags. private EntryFlags flags; ////// Masks used get/set the status of the entry /// [Flags] private enum EntryFlags { ///Bitmask for ShouldUpdateFromPayload flag. ShouldUpdateFromPayload = 0x01, ///Bitmask for CreatedByMaterializer flag. CreatedByMaterializer = 0x02, ///Bitmask for EntityHasBeenResolved flag. EntityHasBeenResolved = 0x04, ///Bitmask for MediaLinkEntry flag (value). MediaLinkEntryValue = 0x08, ///Bitmask for MediaLinkEntry flag (assigned/non-null state). MediaLinkEntryAssigned = 0x10, ///Bitmask for EntityPropertyMappingsApplied flag. EntityPropertyMappingsApplied = 0x20, ///Bitmask for IsNull flag. IsNull = 0x40 } #endregion Private fields. #region Public properties. ///MediaLinkEntry. Valid after data values applies. public bool? MediaLinkEntry { get { return this.GetFlagValue(EntryFlags.MediaLinkEntryAssigned) ? (bool?)this.GetFlagValue(EntryFlags.MediaLinkEntryValue) : null; } set { Debug.Assert(value.HasValue, "value.HasValue -- callers shouldn't set the value to unknown"); this.SetFlagValue(EntryFlags.MediaLinkEntryAssigned, true); this.SetFlagValue(EntryFlags.MediaLinkEntryValue, value.Value); } } ///URI for media content. null if MediaLinkEntry is false. public Uri MediaContentUri { get; set; } ///URI for editing media. null if MediaLinkEntry is false. public Uri MediaEditUri { get; set; } ///Type name, as present in server payload. public string TypeName { get; set; } ///Actual type of the ResolvedObject. public ClientType ActualType { get; set; } ///Edit link for the ATOM entry - basically link used to update the entity. public Uri EditLink { get; set; } ///Self link for the ATOM entry - basically link used to query the entity. public Uri QueryLink { get; set; } ////// Identity link for the ATOM entry. /// This is set by the parser (guaranteed, fails to parser if not available on entry). /// public string Identity { get; set; } ////// Whether the entry is a null. /// This is set by the parser only on inlined entries, as it's invalid ATOM in /// top-level cases. /// public bool IsNull { get { return this.GetFlagValue(EntryFlags.IsNull); } set { this.SetFlagValue(EntryFlags.IsNull, value); } } ///Data names and values. public ListDataValues { get; set; } /// Resolved object. public object ResolvedObject { get; set; } ///Tag for the entry, set by the parser when an entry callback is invoked. public object Tag { get; set; } ///Text for Etag, possibly null. public string ETagText { get; set; } ///Text for ETag corresponding to media resource for this entry. public string StreamETagText { get; set; } ///Whether values should be updated from payload. public bool ShouldUpdateFromPayload { get { return this.GetFlagValue(EntryFlags.ShouldUpdateFromPayload); } set { this.SetFlagValue(EntryFlags.ShouldUpdateFromPayload, value); } } ///Whether the materializer has created the ResolvedObject instance. public bool CreatedByMaterializer { get { return this.GetFlagValue(EntryFlags.CreatedByMaterializer); } set { this.SetFlagValue(EntryFlags.CreatedByMaterializer, value); } } ///Whether the entity has been resolved / created. public bool EntityHasBeenResolved { get { return this.GetFlagValue(EntryFlags.EntityHasBeenResolved); } set { this.SetFlagValue(EntryFlags.EntityHasBeenResolved, value); } } ///Whether entity Property Mappings (a.k.a. friendly feeds) have been applied to this entry if applicable. public bool EntityPropertyMappingsApplied { get { return this.GetFlagValue(EntryFlags.EntityPropertyMappingsApplied); } set { this.SetFlagValue(EntryFlags.EntityPropertyMappingsApplied, value); } } #endregion Public properties. #region Private methods. ///Gets the value for a masked item. /// Mask value. ///true if the flag is set; false otherwise. private bool GetFlagValue(EntryFlags mask) { return (this.flags & mask) != 0; } ///Sets the value for a masked item. /// Mask value. /// Value to set private void SetFlagValue(EntryFlags mask, bool value) { if (value) { this.flags |= mask; } else { this.flags &= (~mask); } } #endregion Private methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
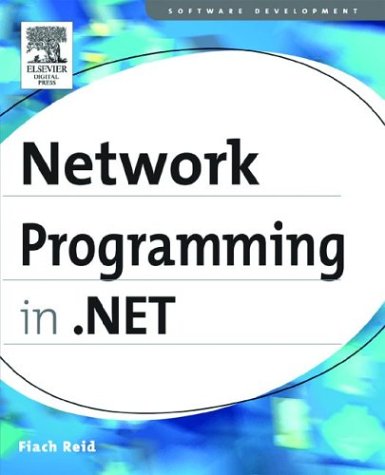
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ISAPIApplicationHost.cs
- While.cs
- IntegerFacetDescriptionElement.cs
- IInstanceContextProvider.cs
- HostProtectionPermission.cs
- TypeLoadException.cs
- GetBrowserTokenRequest.cs
- ResXResourceSet.cs
- TextParagraph.cs
- ReadOnlyAttribute.cs
- BroadcastEventHelper.cs
- ObjectStateEntry.cs
- Point3D.cs
- DataSourceSelectArguments.cs
- CurrencyManager.cs
- ContainerParaClient.cs
- UshortList2.cs
- BinaryFormatter.cs
- UserCancellationException.cs
- COM2TypeInfoProcessor.cs
- DataGrid.cs
- RadioButtonList.cs
- RectangleGeometry.cs
- SingleObjectCollection.cs
- TypedElement.cs
- GrammarBuilder.cs
- ConfigurationSection.cs
- WmlSelectionListAdapter.cs
- EncodingTable.cs
- LinqDataSourceDisposeEventArgs.cs
- Connection.cs
- OleDbCommandBuilder.cs
- FlowLayoutPanelDesigner.cs
- NameNode.cs
- VersionConverter.cs
- DesignerForm.cs
- Sentence.cs
- MonthCalendar.cs
- Image.cs
- DeviceOverridableAttribute.cs
- SHA256Cng.cs
- SingleConverter.cs
- AspCompat.cs
- RuleSettings.cs
- PermissionSetTriple.cs
- DataGridViewCellPaintingEventArgs.cs
- SessionEndingEventArgs.cs
- DebugView.cs
- HttpFileCollectionBase.cs
- SelectionEditor.cs
- DataGridDetailsPresenterAutomationPeer.cs
- ContextMarshalException.cs
- WebPartConnectionsCancelEventArgs.cs
- NativeBuffer.cs
- DataViewManagerListItemTypeDescriptor.cs
- TreeNodeConverter.cs
- DebuggerAttributes.cs
- BufferedGraphics.cs
- MetafileHeaderWmf.cs
- StaticDataManager.cs
- _KerberosClient.cs
- _TLSstream.cs
- DbTransaction.cs
- LineSegment.cs
- ActivationServices.cs
- storepermissionattribute.cs
- DataGridViewAutoSizeModeEventArgs.cs
- SQLConvert.cs
- RowParagraph.cs
- SelectingProviderEventArgs.cs
- PropertyStore.cs
- SamlAttributeStatement.cs
- ErrorWrapper.cs
- DnsPermission.cs
- ResourcePool.cs
- SchemaCollectionCompiler.cs
- FindCriteriaElement.cs
- FlowchartDesigner.Helpers.cs
- NetDispatcherFaultException.cs
- Wildcard.cs
- ConstrainedGroup.cs
- RuntimeWrappedException.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- XamlWriter.cs
- MarkupCompiler.cs
- WebPartDisplayModeEventArgs.cs
- NetNamedPipeSecurityMode.cs
- Misc.cs
- MaskedTextBox.cs
- Literal.cs
- MessageSecurityException.cs
- PathStreamGeometryContext.cs
- TextRunCache.cs
- UnmanagedMemoryStream.cs
- HorizontalAlignConverter.cs
- DeferredTextReference.cs
- Process.cs
- BackStopAuthenticationModule.cs
- RecognizerStateChangedEventArgs.cs
- SimpleBitVector32.cs