Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / XamlWriter.cs / 1305600 / XamlWriter.cs
//---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { ////// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, TypeConverterHelper.InvariantEnglishUS); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { /// /// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, TypeConverterHelper.InvariantEnglishUS); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
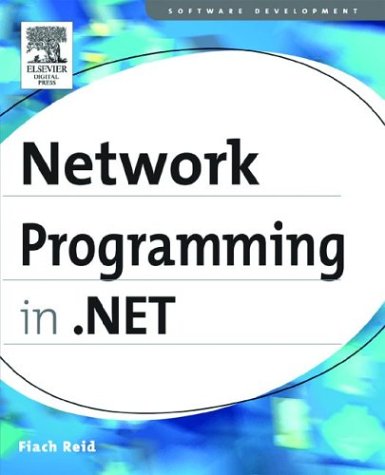
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GroupBox.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- X509Logo.cs
- ConfigXmlSignificantWhitespace.cs
- CaretElement.cs
- mongolianshape.cs
- AlignmentYValidation.cs
- LinkConverter.cs
- XmlTypeMapping.cs
- SqlDataSourceFilteringEventArgs.cs
- HttpHandlersSection.cs
- ProcessThread.cs
- Table.cs
- SecurityContext.cs
- ToolStripRendererSwitcher.cs
- SQLSingle.cs
- XmlLanguage.cs
- DbConnectionStringCommon.cs
- PropertySourceInfo.cs
- StorageComplexTypeMapping.cs
- RegexMatch.cs
- KeyMatchBuilder.cs
- SqlClientWrapperSmiStream.cs
- ThreadNeutralSemaphore.cs
- EnumValidator.cs
- SqlBulkCopyColumnMapping.cs
- WindowsRichEdit.cs
- filewebresponse.cs
- DisplayNameAttribute.cs
- IPPacketInformation.cs
- PathSegmentCollection.cs
- SiteMapNode.cs
- BufferedGraphics.cs
- DirtyTextRange.cs
- Process.cs
- DataGridViewHeaderCell.cs
- ISAPIWorkerRequest.cs
- SessionStateSection.cs
- TypeGeneratedEventArgs.cs
- WebChannelFactory.cs
- WinEventWrap.cs
- SqlDependencyUtils.cs
- WindowsListView.cs
- SecureConversationSecurityTokenParameters.cs
- EntitySetBase.cs
- SvcMapFile.cs
- Expander.cs
- SafeEventLogReadHandle.cs
- Asn1IntegerConverter.cs
- WebPartTransformerCollection.cs
- CompositeFontParser.cs
- SystemFonts.cs
- ClientRuntimeConfig.cs
- LogManagementAsyncResult.cs
- XmlUTF8TextReader.cs
- CaseInsensitiveHashCodeProvider.cs
- UIElementPropertyUndoUnit.cs
- DataGridTableCollection.cs
- ConstraintEnumerator.cs
- TCPClient.cs
- ImageBrush.cs
- HttpHandlerActionCollection.cs
- WebPartsPersonalizationAuthorization.cs
- OdbcConnectionStringbuilder.cs
- PackageFilter.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- ProfileGroupSettings.cs
- DocumentXPathNavigator.cs
- InfoCardTrace.cs
- CommunicationException.cs
- ControlValuePropertyAttribute.cs
- SplineKeyFrames.cs
- XmlTypeAttribute.cs
- Trace.cs
- CommonProperties.cs
- DbConnectionPoolGroup.cs
- MarkupObject.cs
- ReferenceAssemblyAttribute.cs
- WebPartDisplayModeCollection.cs
- OracleBoolean.cs
- CodeGeneratorOptions.cs
- SerializableTypeCodeDomSerializer.cs
- SortedSet.cs
- UInt32Converter.cs
- WebRequest.cs
- AccessKeyManager.cs
- ExternalCalls.cs
- Animatable.cs
- SchemaObjectWriter.cs
- StateWorkerRequest.cs
- SchemaImporterExtension.cs
- MILUtilities.cs
- XmlWriter.cs
- FixedSOMLineCollection.cs
- GeneralTransform2DTo3DTo2D.cs
- RuntimeResourceSet.cs
- DocumentXPathNavigator.cs
- RubberbandSelector.cs
- Socket.cs
- SqlDataSourceConnectionPanel.cs