Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartDisplayModeCollection.cs / 1305376 / WebPartDisplayModeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; public sealed class WebPartDisplayModeCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; // Prevent instantiation outside of our assembly. We want third-part code to use the collection // returned by the base method, not create a new collection. internal WebPartDisplayModeCollection() { } public bool IsReadOnly { get { return _readOnly; } } public WebPartDisplayMode this[int index] { get { return (WebPartDisplayMode)List[index]; } } public WebPartDisplayMode this[string modeName] { get { foreach (WebPartDisplayMode displayMode in List) { if (String.Equals(displayMode.Name, modeName, StringComparison.OrdinalIgnoreCase)) { return displayMode; } } return null; } } public int Add(WebPartDisplayMode value) { return List.Add(value); } internal int AddInternal(WebPartDisplayMode value) { bool isReadOnly = _readOnly; _readOnly = false; // Extra try-catch block to prevent elevation of privilege attack via exception filter try { try { return List.Add(value); } finally { _readOnly = isReadOnly; } } catch { throw; } } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } public bool Contains(WebPartDisplayMode value) { return List.Contains(value); } public void CopyTo(WebPartDisplayMode[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartDisplayMode value) { return List.IndexOf(value); } public void Insert(int index, WebPartDisplayMode value) { List.Insert(index, value); } protected override void OnClear() { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnInsert(int index, object value) { CheckReadOnly(); WebPartDisplayMode displayMode = (WebPartDisplayMode)value; foreach (WebPartDisplayMode existingDisplayMode in List) { if (displayMode.Name == existingDisplayMode.Name) { throw new ArgumentException(SR.GetString( SR.WebPartDisplayModeCollection_DuplicateName, displayMode.Name)); } } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnSet(int index, object oldValue, object newValue) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantSet)); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartDisplayMode)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartDisplayMode"), "value"); } } internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; public sealed class WebPartDisplayModeCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; // Prevent instantiation outside of our assembly. We want third-part code to use the collection // returned by the base method, not create a new collection. internal WebPartDisplayModeCollection() { } public bool IsReadOnly { get { return _readOnly; } } public WebPartDisplayMode this[int index] { get { return (WebPartDisplayMode)List[index]; } } public WebPartDisplayMode this[string modeName] { get { foreach (WebPartDisplayMode displayMode in List) { if (String.Equals(displayMode.Name, modeName, StringComparison.OrdinalIgnoreCase)) { return displayMode; } } return null; } } public int Add(WebPartDisplayMode value) { return List.Add(value); } internal int AddInternal(WebPartDisplayMode value) { bool isReadOnly = _readOnly; _readOnly = false; // Extra try-catch block to prevent elevation of privilege attack via exception filter try { try { return List.Add(value); } finally { _readOnly = isReadOnly; } } catch { throw; } } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } public bool Contains(WebPartDisplayMode value) { return List.Contains(value); } public void CopyTo(WebPartDisplayMode[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartDisplayMode value) { return List.IndexOf(value); } public void Insert(int index, WebPartDisplayMode value) { List.Insert(index, value); } protected override void OnClear() { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnInsert(int index, object value) { CheckReadOnly(); WebPartDisplayMode displayMode = (WebPartDisplayMode)value; foreach (WebPartDisplayMode existingDisplayMode in List) { if (displayMode.Name == existingDisplayMode.Name) { throw new ArgumentException(SR.GetString( SR.WebPartDisplayModeCollection_DuplicateName, displayMode.Name)); } } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnSet(int index, object oldValue, object newValue) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantSet)); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartDisplayMode)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartDisplayMode"), "value"); } } internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
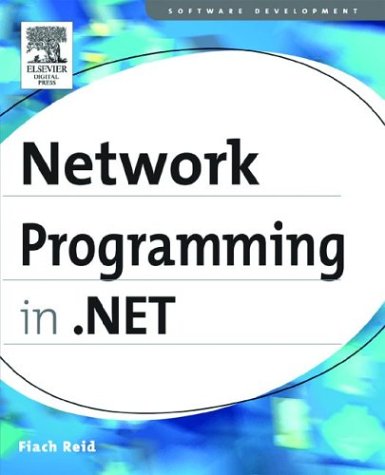
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsStatic.cs
- Token.cs
- LocalizableResourceBuilder.cs
- SpecialFolderEnumConverter.cs
- MetaModel.cs
- WebPartHeaderCloseVerb.cs
- PropertyDescriptorComparer.cs
- BufferedReadStream.cs
- ConcatQueryOperator.cs
- PropertyRef.cs
- ContractMapping.cs
- SecurityKeyUsage.cs
- SQLSingle.cs
- StackBuilderSink.cs
- ConfigurationSection.cs
- ExtentKey.cs
- XmlStringTable.cs
- OleDbParameter.cs
- PixelFormats.cs
- PageRanges.cs
- SqlCommandBuilder.cs
- ChannelManager.cs
- FlowDocumentReaderAutomationPeer.cs
- DescendantBaseQuery.cs
- PolyQuadraticBezierSegment.cs
- CacheHelper.cs
- WebEventCodes.cs
- Scheduler.cs
- SQLBytesStorage.cs
- DNS.cs
- OwnerDrawPropertyBag.cs
- SingleAnimation.cs
- PresentationTraceSources.cs
- ConfigurationSectionCollection.cs
- ScalarOps.cs
- GAC.cs
- FocusChangedEventArgs.cs
- FileNotFoundException.cs
- DataListCommandEventArgs.cs
- BamlVersionHeader.cs
- DataStreams.cs
- SID.cs
- Metafile.cs
- EventItfInfo.cs
- BindingContext.cs
- TypeLibConverter.cs
- Matrix3D.cs
- EntityException.cs
- QueryReaderSettings.cs
- Profiler.cs
- EncodingStreamWrapper.cs
- MouseDevice.cs
- AutomationEvent.cs
- QilExpression.cs
- InvokeBinder.cs
- UniqueIdentifierService.cs
- DbgCompiler.cs
- Function.cs
- FileChangesMonitor.cs
- UnaryNode.cs
- PenThreadPool.cs
- ExtenderProviderService.cs
- MetadataProperty.cs
- WinFormsComponentEditor.cs
- TreeNodeStyleCollectionEditor.cs
- WsatTransactionInfo.cs
- GuidelineSet.cs
- ProfileInfo.cs
- XMLSyntaxException.cs
- SqlTypeSystemProvider.cs
- EmptyTextWriter.cs
- OutputCacheModule.cs
- ping.cs
- Events.cs
- TextUtf8RawTextWriter.cs
- CapabilitiesUse.cs
- WorkflowMessageEventArgs.cs
- PropertyManager.cs
- PixelShader.cs
- EntityReference.cs
- BitmapDownload.cs
- LoadedOrUnloadedOperation.cs
- ObjectQuery.cs
- WebFaultException.cs
- XmlAtomErrorReader.cs
- RunInstallerAttribute.cs
- DependencyPropertyChangedEventArgs.cs
- OutputScope.cs
- ProxyWebPartManager.cs
- PrintingPermission.cs
- HtmlEmptyTagControlBuilder.cs
- ToolStripStatusLabel.cs
- PerformanceCounterLib.cs
- MatrixStack.cs
- ProxySimple.cs
- CodeDefaultValueExpression.cs
- PowerStatus.cs
- ThreadExceptionDialog.cs
- CodeDomSerializationProvider.cs
- ConfigurationStrings.cs