Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / Mail / BufferedReadStream.cs / 1 / BufferedReadStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.IO; internal class BufferedReadStream : DelegatedStream { byte[] storedBuffer; int storedLength; int storedOffset; bool readMore; internal BufferedReadStream(Stream stream) : this(stream, false) { } internal BufferedReadStream(Stream stream, bool readMore) : base(stream) { this.readMore = readMore; } public override bool CanWrite { get { return false; } } public override bool CanSeek { get { return false; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { ReadAsyncResult result = new ReadAsyncResult(this, callback, state); result.Read(buffer, offset, count); return result; } public override int EndRead(IAsyncResult asyncResult) { int read = ReadAsyncResult.End(asyncResult); return read; } public override int Read(byte[] buffer, int offset, int count) { int read = 0; if (this.storedOffset < this.storedLength) { read = Math.Min(count, this.storedLength - this.storedOffset); Buffer.BlockCopy(this.storedBuffer, this.storedOffset, buffer, offset, read); this.storedOffset += read; if (read == count || !this.readMore) { return read; } offset += read; count -= read; } return read + base.Read(buffer, offset, count); } public override int ReadByte() { if (this.storedOffset < this.storedLength) { return (int)this.storedBuffer[this.storedOffset++]; } else { return base.ReadByte(); } } internal void Push(byte[] buffer, int offset, int count) { if (count == 0) return; if (this.storedOffset == this.storedLength) { if (this.storedBuffer == null || this.storedBuffer.Length < count) { this.storedBuffer = new byte[count]; } this.storedOffset = 0; this.storedLength = count; } else { // if there's room to just insert before existing data if (count <= this.storedOffset) { this.storedOffset -= count; } // if there's room in the buffer but need to shift things over else if (count <= this.storedBuffer.Length - this.storedLength + this.storedOffset) { Buffer.BlockCopy(this.storedBuffer, this.storedOffset, this.storedBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; } else { byte[] newBuffer = new byte[count + this.storedLength - this.storedOffset]; Buffer.BlockCopy(this.storedBuffer, this.storedOffset, newBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; this.storedBuffer = newBuffer; } } Buffer.BlockCopy(buffer, offset, this.storedBuffer, this.storedOffset, count); } class ReadAsyncResult : LazyAsyncResult { BufferedReadStream parent; int read; static AsyncCallback onRead = new AsyncCallback(OnRead); internal ReadAsyncResult(BufferedReadStream parent, AsyncCallback callback, object state) : base(null,state,callback) { this.parent = parent; } internal void Read(byte[] buffer, int offset, int count){ if (parent.storedOffset < parent.storedLength) { this.read = Math.Min(count, parent.storedLength - parent.storedOffset); Buffer.BlockCopy(parent.storedBuffer, parent.storedOffset, buffer, offset, this.read); parent.storedOffset += this.read; if (this.read == count || !this.parent.readMore) { this.InvokeCallback(); return; } count -= this.read; offset += this.read; } IAsyncResult result = parent.BaseStream.BeginRead(buffer, offset, count, onRead, this); if (result.CompletedSynchronously) { // this.read += parent.BaseStream.EndRead(result); InvokeCallback(); } } internal static int End(IAsyncResult result) { ReadAsyncResult thisPtr = (ReadAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.read; } static void OnRead(IAsyncResult result) { if (!result.CompletedSynchronously) { ReadAsyncResult thisPtr = (ReadAsyncResult)result.AsyncState; try { thisPtr.read += thisPtr.parent.BaseStream.EndRead(result); thisPtr.InvokeCallback(); } catch (Exception e) { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(e); } catch { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.IO; internal class BufferedReadStream : DelegatedStream { byte[] storedBuffer; int storedLength; int storedOffset; bool readMore; internal BufferedReadStream(Stream stream) : this(stream, false) { } internal BufferedReadStream(Stream stream, bool readMore) : base(stream) { this.readMore = readMore; } public override bool CanWrite { get { return false; } } public override bool CanSeek { get { return false; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { ReadAsyncResult result = new ReadAsyncResult(this, callback, state); result.Read(buffer, offset, count); return result; } public override int EndRead(IAsyncResult asyncResult) { int read = ReadAsyncResult.End(asyncResult); return read; } public override int Read(byte[] buffer, int offset, int count) { int read = 0; if (this.storedOffset < this.storedLength) { read = Math.Min(count, this.storedLength - this.storedOffset); Buffer.BlockCopy(this.storedBuffer, this.storedOffset, buffer, offset, read); this.storedOffset += read; if (read == count || !this.readMore) { return read; } offset += read; count -= read; } return read + base.Read(buffer, offset, count); } public override int ReadByte() { if (this.storedOffset < this.storedLength) { return (int)this.storedBuffer[this.storedOffset++]; } else { return base.ReadByte(); } } internal void Push(byte[] buffer, int offset, int count) { if (count == 0) return; if (this.storedOffset == this.storedLength) { if (this.storedBuffer == null || this.storedBuffer.Length < count) { this.storedBuffer = new byte[count]; } this.storedOffset = 0; this.storedLength = count; } else { // if there's room to just insert before existing data if (count <= this.storedOffset) { this.storedOffset -= count; } // if there's room in the buffer but need to shift things over else if (count <= this.storedBuffer.Length - this.storedLength + this.storedOffset) { Buffer.BlockCopy(this.storedBuffer, this.storedOffset, this.storedBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; } else { byte[] newBuffer = new byte[count + this.storedLength - this.storedOffset]; Buffer.BlockCopy(this.storedBuffer, this.storedOffset, newBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; this.storedBuffer = newBuffer; } } Buffer.BlockCopy(buffer, offset, this.storedBuffer, this.storedOffset, count); } class ReadAsyncResult : LazyAsyncResult { BufferedReadStream parent; int read; static AsyncCallback onRead = new AsyncCallback(OnRead); internal ReadAsyncResult(BufferedReadStream parent, AsyncCallback callback, object state) : base(null,state,callback) { this.parent = parent; } internal void Read(byte[] buffer, int offset, int count){ if (parent.storedOffset < parent.storedLength) { this.read = Math.Min(count, parent.storedLength - parent.storedOffset); Buffer.BlockCopy(parent.storedBuffer, parent.storedOffset, buffer, offset, this.read); parent.storedOffset += this.read; if (this.read == count || !this.parent.readMore) { this.InvokeCallback(); return; } count -= this.read; offset += this.read; } IAsyncResult result = parent.BaseStream.BeginRead(buffer, offset, count, onRead, this); if (result.CompletedSynchronously) { // this.read += parent.BaseStream.EndRead(result); InvokeCallback(); } } internal static int End(IAsyncResult result) { ReadAsyncResult thisPtr = (ReadAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.read; } static void OnRead(IAsyncResult result) { if (!result.CompletedSynchronously) { ReadAsyncResult thisPtr = (ReadAsyncResult)result.AsyncState; try { thisPtr.read += thisPtr.parent.BaseStream.EndRead(result); thisPtr.InvokeCallback(); } catch (Exception e) { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(e); } catch { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
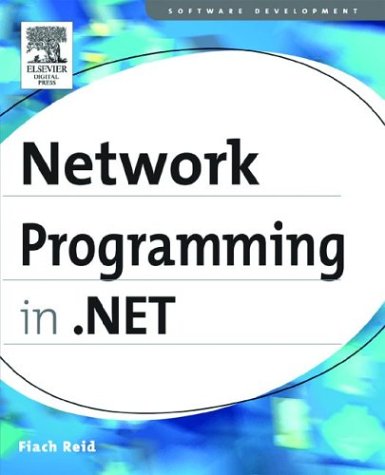
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinarySerializer.cs
- Version.cs
- autovalidator.cs
- SerializationSectionGroup.cs
- WindowsEditBoxRange.cs
- PaperSize.cs
- _DynamicWinsockMethods.cs
- CompressionTransform.cs
- BatchServiceHost.cs
- SqlBooleanizer.cs
- Model3D.cs
- Directory.cs
- CodePageEncoding.cs
- BooleanAnimationBase.cs
- UITypeEditor.cs
- InertiaExpansionBehavior.cs
- WindowsFormsHelpers.cs
- WindowsSlider.cs
- QueryNode.cs
- MemoryRecordBuffer.cs
- SQLConvert.cs
- Relationship.cs
- ByteConverter.cs
- TextBoxLine.cs
- Funcletizer.cs
- NotCondition.cs
- LifetimeServices.cs
- HttpModuleAction.cs
- XmlSignificantWhitespace.cs
- OperationDescription.cs
- LoginNameDesigner.cs
- MinMaxParagraphWidth.cs
- ExpressionBuilder.cs
- DtdParser.cs
- UpdateProgress.cs
- BufferedGenericXmlSecurityToken.cs
- DetailsViewAutoFormat.cs
- SequenceQuery.cs
- RawStylusSystemGestureInputReport.cs
- Style.cs
- XsltLoader.cs
- XmlSchemaGroupRef.cs
- PointCollection.cs
- Deflater.cs
- PageHandlerFactory.cs
- HorizontalAlignConverter.cs
- AsymmetricKeyExchangeFormatter.cs
- ProtocolsConfigurationEntry.cs
- XmlSchemaExporter.cs
- HwndAppCommandInputProvider.cs
- XsltContext.cs
- OdbcError.cs
- LogFlushAsyncResult.cs
- SrgsElementFactory.cs
- MenuItemCollection.cs
- ChannelServices.cs
- WebPartDescription.cs
- CatalogPart.cs
- KeyInterop.cs
- GridEntryCollection.cs
- Pts.cs
- EntityDataSourceWrapper.cs
- Vector3DIndependentAnimationStorage.cs
- TextParentUndoUnit.cs
- EntityDataSourceValidationException.cs
- ArgumentNullException.cs
- DesignerSerializationVisibilityAttribute.cs
- externdll.cs
- BuildResult.cs
- AnnotationResourceChangedEventArgs.cs
- BinaryCommonClasses.cs
- SettingsProperty.cs
- WebBrowserContainer.cs
- ClientSession.cs
- MembershipValidatePasswordEventArgs.cs
- externdll.cs
- Process.cs
- VisualTarget.cs
- Variable.cs
- VisualBrush.cs
- Int32Rect.cs
- AutomationProperties.cs
- UIInitializationException.cs
- OleDbErrorCollection.cs
- TraceSource.cs
- SafeNativeMemoryHandle.cs
- ChildTable.cs
- ActivitySurrogate.cs
- Soap.cs
- LicFileLicenseProvider.cs
- RefreshEventArgs.cs
- Certificate.cs
- TemplateBuilder.cs
- StrokeCollectionConverter.cs
- SystemColors.cs
- _LocalDataStore.cs
- SqlNotificationEventArgs.cs
- AuthenticationConfig.cs
- FixedSOMTableRow.cs
- Win32.cs