Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / EncryptedData.cs / 1 / EncryptedData.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Xml; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class is used by the encryption utility to encrypt the security token // internal class EncryptedData { string m_type; EncryptionMethodElement m_encryptionMethod; CipherData m_cipherData; SecurityKeyIdentifier m_keyIdentifier; SecurityTokenSerializer m_tokenSerializer; public EncryptedData() { this.m_cipherData = new CipherData(); this.m_encryptionMethod = new EncryptionMethodElement(); } public string EncryptionMethod { set { this.m_encryptionMethod.algorithm = value; } } public SecurityKeyIdentifier KeyIdentifier { set { this.m_keyIdentifier = value; } } public string Type { set { this.m_type = value; } } public SecurityTokenSerializer TokenSerializer { set { this.m_tokenSerializer = value; } } // // Summary // Set up the encryption // // Parameters // algorithm - The symmetric algorithm to use. // buffer - Data to encrypt // offset - The offset into the byte array from which to begin using data. // length - The number of bytes in the byte array to use as data. // public void SetUpEncryption( SymmetricAlgorithm algorithm, byte[ ] buffer, int offset, int length ) { IDT.ThrowInvalidArgumentConditional( null == algorithm, "algorithm" ); IDT.TraceDebug( "Setting up the encryption for {0}", algorithm.ToString() ); byte[ ] iv; byte[ ] cipherText; GenerateIVAndEncrypt( algorithm, buffer, offset, length, out iv, out cipherText ); this.m_cipherData.SetCipherValueFragments( iv, cipherText ); } // // Summary // Write the encrypted data to a writer // // Parameters // writer - The XmlWriter to which the encrypted data object is written. // public void WriteTo( XmlWriter writer ) { writer.WriteStartElement( XmlNames.XmlEnc.DefaultPrefix, XmlNames.XmlEnc.EncryptedData, XmlNames.XmlEnc.Namespace ); if( !String.IsNullOrEmpty( this.m_type ) ) { writer.WriteAttributeString( XmlNames.XmlEnc.Type, null, this.m_type ); } if( !String.IsNullOrEmpty( this.m_encryptionMethod.algorithm ) ) { this.m_encryptionMethod.WriteTo( writer ); } if( this.m_keyIdentifier != null ) { m_tokenSerializer.WriteKeyIdentifier(XmlDictionaryWriter.CreateDictionaryWriter(writer), this.m_keyIdentifier); } this.m_cipherData.WriteTo( writer ); writer.WriteEndElement(); // EncryptedData } // // Summary // Generate the IV and encrypt the data // // Parameters // algorithm - The symmetric algorithm to use. // plainText - The input for which to compute the transform. // offset - The offset into the byte array from which to begin using data. // length - The number of bytes in the byte array to use as data. // iv - The IV value returned. // cipherText - The cipher text returned. // void GenerateIVAndEncrypt( SymmetricAlgorithm algorithm, byte[ ] plainText, int offset, int length, out byte[ ] iv, out byte[ ] cipherText ) { IDT.TraceDebug( "Generate the IV and encrypt the data" ); RandomNumberGenerator random = new RNGCryptoServiceProvider(); int ivSize = algorithm.BlockSize / 8; iv = new byte[ ivSize ]; random.GetBytes( iv ); algorithm.Padding = PaddingMode.PKCS7; algorithm.Mode = CipherMode.CBC; using ( ICryptoTransform encrTransform = algorithm.CreateEncryptor( algorithm.Key, iv ) ) { cipherText = encrTransform.TransformFinalBlock( plainText, offset, length ); } } // // Summary // Cipher data struct to be used to encrypt the token // struct CipherData { byte[ ] m_iv; byte[ ] m_cipherText; public byte[ ] CipherValue { get { return this.m_cipherText; } } public void SetCipherValueFragments( byte[ ] iv, byte[ ] cipherText ) { m_iv = iv; m_cipherText = cipherText; } public void WriteTo( XmlWriter writer ) { writer.WriteStartElement( XmlNames.XmlEnc.DefaultPrefix, XmlNames.XmlEnc.CipherData, XmlNames.XmlEnc.Namespace ); writer.WriteStartElement( XmlNames.XmlEnc.DefaultPrefix, XmlNames.XmlEnc.CipherValue, XmlNames.XmlEnc.Namespace ); if( null != m_iv ) { writer.WriteBase64( m_iv, 0, m_iv.Length ); } writer.WriteBase64( m_cipherText, 0, m_cipherText.Length ); writer.WriteEndElement(); // CipherValue writer.WriteEndElement(); // CipherData } } // // Summary // The encryption element to be written to the XML // struct EncryptionMethodElement { internal string algorithm; public void WriteTo( XmlWriter writer ) { writer.WriteStartElement( XmlNames.XmlEnc.DefaultPrefix, XmlNames.XmlEnc.EncryptionMethod, XmlNames.XmlEnc.Namespace ); writer.WriteAttributeString( XmlNames.XmlEnc.Algorithm, null, this.algorithm ); writer.WriteEndElement(); // EncryptionMethod } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
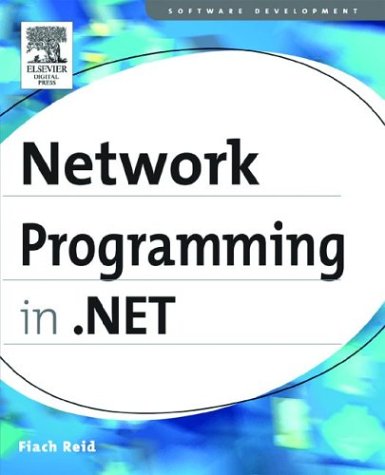
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResolvedKeyFrameEntry.cs
- SqlFacetAttribute.cs
- ActivityXamlServices.cs
- DynamicPropertyReader.cs
- AutomationPropertyInfo.cs
- StringBlob.cs
- StyleXamlParser.cs
- CryptoKeySecurity.cs
- FormatterConverter.cs
- UnsafeMethods.cs
- Terminate.cs
- EntityParameter.cs
- TabControl.cs
- BuildResult.cs
- XMLUtil.cs
- COM2Properties.cs
- _AutoWebProxyScriptHelper.cs
- WebPartCatalogCloseVerb.cs
- XmlComment.cs
- XmlQualifiedNameTest.cs
- ApplicationGesture.cs
- Literal.cs
- TraceContextEventArgs.cs
- precedingquery.cs
- BamlLocalizabilityResolver.cs
- DrawingContextWalker.cs
- UrlMapping.cs
- DnsEndPoint.cs
- EnumerableRowCollection.cs
- EdmFunctions.cs
- ScriptIgnoreAttribute.cs
- ChannelManager.cs
- SelectionUIService.cs
- XmlWriterDelegator.cs
- HandlerWithFactory.cs
- XmlSchemaAll.cs
- ParseHttpDate.cs
- PerformanceCounterPermission.cs
- SimpleMailWebEventProvider.cs
- ContentFilePart.cs
- RuntimeTransactionHandle.cs
- CharEntityEncoderFallback.cs
- Hashtable.cs
- ByteStack.cs
- InfoCardCryptoHelper.cs
- BaseUriWithWildcard.cs
- DbModificationClause.cs
- NetworkStream.cs
- EntityModelBuildProvider.cs
- CapabilitiesUse.cs
- PackWebResponse.cs
- WebHeaderCollection.cs
- Message.cs
- QilXmlReader.cs
- RenderContext.cs
- LambdaCompiler.Lambda.cs
- RegexFCD.cs
- NameTable.cs
- HtmlInputSubmit.cs
- EpmContentDeSerializerBase.cs
- MetaTableHelper.cs
- WindowsRichEditRange.cs
- XmlIncludeAttribute.cs
- WindowsRegion.cs
- SchemaCollectionCompiler.cs
- TypeBuilder.cs
- SharedStatics.cs
- CollectionBuilder.cs
- _BasicClient.cs
- OleDbConnectionInternal.cs
- DefaultProxySection.cs
- safemediahandle.cs
- FolderBrowserDialog.cs
- SudsParser.cs
- ProxyElement.cs
- PerformanceCountersElement.cs
- BufferedGraphicsContext.cs
- CalendarDateRange.cs
- WCFBuildProvider.cs
- InkCanvasSelectionAdorner.cs
- OleDbErrorCollection.cs
- VoiceSynthesis.cs
- ConnectionDemuxer.cs
- XmlAttributeAttribute.cs
- RequestResizeEvent.cs
- _NegotiateClient.cs
- ChangeBlockUndoRecord.cs
- Preprocessor.cs
- DirectoryRedirect.cs
- AddInToken.cs
- HttpTransportBindingElement.cs
- TextReader.cs
- ToolStripMenuItem.cs
- IDReferencePropertyAttribute.cs
- WorkerRequest.cs
- WebPartVerb.cs
- DataRecord.cs
- UpdateManifestForBrowserApplication.cs
- DragDeltaEventArgs.cs
- WpfXamlType.cs