Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / HttpTransportBindingElement.cs / 2 / HttpTransportBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Description; using System.Configuration; using System.Net; using System.Net.Security; using System.Runtime.Serialization; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Security; using System.Xml; using WsdlNS = System.Web.Services.Description; public class HttpTransportBindingElement : TransportBindingElement, IWsdlExportExtension, IPolicyExportExtension, ITransportPolicyImport { bool allowCookies; AuthenticationSchemes authenticationScheme; bool bypassProxyOnLocal; HostNameComparisonMode hostNameComparisonMode; bool keepAliveEnabled; bool inheritBaseAddressSettings; int maxBufferSize; bool maxBufferSizeInitialized; string method; Uri proxyAddress; AuthenticationSchemes proxyAuthenticationScheme; string realm; TransferMode transferMode; bool unsafeConnectionNtlmAuthentication; bool useDefaultWebProxy; IWebProxy webProxy; public HttpTransportBindingElement() : base() { this.allowCookies = HttpTransportDefaults.AllowCookies; this.authenticationScheme = HttpTransportDefaults.AuthenticationScheme; this.bypassProxyOnLocal = HttpTransportDefaults.BypassProxyOnLocal; this.hostNameComparisonMode = HttpTransportDefaults.HostNameComparisonMode; this.keepAliveEnabled = HttpTransportDefaults.KeepAliveEnabled; this.maxBufferSize = TransportDefaults.MaxBufferSize; this.method = string.Empty; this.proxyAuthenticationScheme = HttpTransportDefaults.ProxyAuthenticationScheme; this.proxyAddress = HttpTransportDefaults.ProxyAddress; this.realm = HttpTransportDefaults.Realm; this.transferMode = HttpTransportDefaults.TransferMode; this.unsafeConnectionNtlmAuthentication = HttpTransportDefaults.UnsafeConnectionNtlmAuthentication; this.useDefaultWebProxy = HttpTransportDefaults.UseDefaultWebProxy; this.webProxy = null; } protected HttpTransportBindingElement(HttpTransportBindingElement elementToBeCloned) : base(elementToBeCloned) { this.allowCookies = elementToBeCloned.allowCookies; this.authenticationScheme = elementToBeCloned.authenticationScheme; this.bypassProxyOnLocal = elementToBeCloned.bypassProxyOnLocal; this.hostNameComparisonMode = elementToBeCloned.hostNameComparisonMode; this.inheritBaseAddressSettings = elementToBeCloned.InheritBaseAddressSettings; this.keepAliveEnabled = elementToBeCloned.keepAliveEnabled; this.maxBufferSize = elementToBeCloned.maxBufferSize; this.maxBufferSizeInitialized = elementToBeCloned.maxBufferSizeInitialized; this.method = elementToBeCloned.method; this.proxyAddress = elementToBeCloned.proxyAddress; this.proxyAuthenticationScheme = elementToBeCloned.proxyAuthenticationScheme; this.realm = elementToBeCloned.realm; this.transferMode = elementToBeCloned.transferMode; this.unsafeConnectionNtlmAuthentication = elementToBeCloned.unsafeConnectionNtlmAuthentication; this.useDefaultWebProxy = elementToBeCloned.useDefaultWebProxy; this.webProxy = elementToBeCloned.webProxy; } public bool AllowCookies { get { return this.allowCookies; } set { this.allowCookies = value; } } public AuthenticationSchemes AuthenticationScheme { get { return this.authenticationScheme; } set { if (!AuthenticationSchemesHelper.IsSingleton(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("value", SR.GetString(SR.HttpRequiresSingleAuthScheme, value)); } this.authenticationScheme = value; } } public bool BypassProxyOnLocal { get { return this.bypassProxyOnLocal; } set { this.bypassProxyOnLocal = value; } } public HostNameComparisonMode HostNameComparisonMode { get { return this.hostNameComparisonMode; } set { HostNameComparisonModeHelper.Validate(value); this.hostNameComparisonMode = value; } } // MB#26970: used by MEX to ensure that we don't conflict on base-address scoped settings internal bool InheritBaseAddressSettings { get { return this.inheritBaseAddressSettings; } set { this.inheritBaseAddressSettings = value; } } public bool KeepAliveEnabled { get { return this.keepAliveEnabled; } set { this.keepAliveEnabled = value; } } // client // server public int MaxBufferSize { get { if (maxBufferSizeInitialized || TransferMode != TransferMode.Buffered) return maxBufferSize; long maxReceivedMessageSize = MaxReceivedMessageSize; if (maxReceivedMessageSize > int.MaxValue) return int.MaxValue; else return (int)maxReceivedMessageSize; } set { if (value <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.ValueMustBePositive))); } maxBufferSizeInitialized = true; this.maxBufferSize = value; } } // string.Empty == wildcard internal string Method { get { return this.method; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.method = value; } } public Uri ProxyAddress { get { return this.proxyAddress; } set { this.proxyAddress = value; } } public AuthenticationSchemes ProxyAuthenticationScheme { get { return this.proxyAuthenticationScheme; } set { if (!AuthenticationSchemesHelper.IsSingleton(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("value", SR.GetString(SR.HttpProxyRequiresSingleAuthScheme, value)); } this.proxyAuthenticationScheme = value; } } // CSDMain#17853: used by cardspace to ensure that the correct proxy is picked up internal IWebProxy Proxy { set { this.webProxy = value; } get { return this.webProxy; } } public string Realm { get { return this.realm; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.realm = value; } } public override string Scheme { get { return "http"; } } // client // server public TransferMode TransferMode { get { return this.transferMode; } set { TransferModeHelper.Validate(value); this.transferMode = value; } } internal virtual bool SupportsClientAuthenticationImpl { get { return (this.authenticationScheme != AuthenticationSchemes.Anonymous); } } internal virtual bool SupportsClientWindowsIdentityImpl { get { return (this.authenticationScheme != AuthenticationSchemes.Anonymous); } } public bool UnsafeConnectionNtlmAuthentication { get { return this.unsafeConnectionNtlmAuthentication; } set { this.unsafeConnectionNtlmAuthentication = value; } } public bool UseDefaultWebProxy { get { return this.useDefaultWebProxy; } set { this.useDefaultWebProxy = value; } } internal virtual string WsdlTransportUri { get { return TransportPolicyConstants.HttpTransportUri; } } public override BindingElement Clone() { return new HttpTransportBindingElement(this); } public override T GetProperty(BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(ISecurityCapabilities)) { return (T)(object)new SecurityCapabilities(SupportsClientAuthenticationImpl, AuthenticationScheme == AuthenticationSchemes.Negotiate, SupportsClientWindowsIdentityImpl, ProtectionLevel.None, ProtectionLevel.None); } else if (typeof(T) == typeof(IBindingDeliveryCapabilities)) { return (T)(object)new BindingDeliveryCapabilitiesHelper(); } else if (typeof(T) == typeof(TransferMode)) { return (T)(object)this.TransferMode; } else { #pragma warning suppress 56506 // [....], BindingContext.BindingParameters cannot be null if (context.BindingParameters.Find () == null) { context.BindingParameters.Add(new TextMessageEncodingBindingElement()); } return base.GetProperty (context); } } public override bool CanBuildChannelFactory (BindingContext context) { return (typeof(TChannel) == typeof(IRequestChannel)); } public override bool CanBuildChannelListener (BindingContext context) { return (typeof(TChannel) == typeof(IReplyChannel)); } public override IChannelFactory BuildChannelFactory (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (!this.CanBuildChannelFactory (context)) { #pragma warning suppress 56506 // [....], context.Binding will never be null. throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.CouldnTCreateChannelForChannelType2, context.Binding.Name, typeof(TChannel))); } return (IChannelFactory )(object)new HttpChannelFactory(this, context); } public override IChannelListener BuildChannelListener (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (!this.CanBuildChannelListener (context)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument( #pragma warning suppress 56506 // [....], context.Binding will never be null. "TChannel", SR.GetString(SR.CouldnTCreateChannelForChannelType2, context.Binding.Name, typeof(TChannel))); } HttpChannelListener listener = new HttpChannelListener(this, context); VirtualPathExtension.ApplyHostedContext(listener, context); return (IChannelListener )(object)listener; } void IPolicyExportExtension.ExportPolicy(MetadataExporter exporter, PolicyConversionContext context) { if (exporter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("exporter"); } if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } this.OnExportPolicy(exporter, context); bool createdNew; MessageEncodingBindingElement encodingBindingElement = FindMessageEncodingBindingElement(context.BindingElements, out createdNew); if (createdNew && encodingBindingElement is IPolicyExportExtension) { ((IPolicyExportExtension)encodingBindingElement).ExportPolicy(exporter, context); } WsdlExporter.WSAddressingHelper.AddWSAddressingAssertion(exporter, context, encodingBindingElement.MessageVersion.Addressing); } internal virtual void OnExportPolicy(MetadataExporter exporter, PolicyConversionContext policyContext) { string assertionName = null; switch (this.AuthenticationScheme) { case AuthenticationSchemes.Anonymous: break; case AuthenticationSchemes.Basic: assertionName = TransportPolicyConstants.BasicHttpAuthenticationName; break; case AuthenticationSchemes.Digest: assertionName = TransportPolicyConstants.DigestHttpAuthenticationName; break; case AuthenticationSchemes.Negotiate: assertionName = TransportPolicyConstants.NegotiateHttpAuthenticationName; break; case AuthenticationSchemes.Ntlm: assertionName = TransportPolicyConstants.NtlmHttpAuthenticationName; break; } if (assertionName != null) { policyContext.GetBindingAssertions().Add(new XmlDocument().CreateElement(TransportPolicyConstants.HttpAuthPrefix, assertionName, TransportPolicyConstants.HttpAuthNamespace)); } } internal virtual void OnImportPolicy(MetadataImporter importer, PolicyConversionContext policyContext) { } void ITransportPolicyImport.ImportPolicy(MetadataImporter importer, PolicyConversionContext policyContext) { ICollection bindingAssertions = policyContext.GetBindingAssertions(); List httpAuthAssertions = new List (); bool foundAssertion = false; foreach (XmlElement assertion in bindingAssertions) { if (assertion.NamespaceURI != TransportPolicyConstants.HttpAuthNamespace) { continue; } switch (assertion.LocalName) { case TransportPolicyConstants.BasicHttpAuthenticationName: this.AuthenticationScheme = AuthenticationSchemes.Basic; break; case TransportPolicyConstants.DigestHttpAuthenticationName: this.AuthenticationScheme = AuthenticationSchemes.Digest; break; case TransportPolicyConstants.NegotiateHttpAuthenticationName: this.AuthenticationScheme = AuthenticationSchemes.Negotiate; break; case TransportPolicyConstants.NtlmHttpAuthenticationName: this.AuthenticationScheme = AuthenticationSchemes.Ntlm; break; default: continue; } if (foundAssertion) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException( SR.GetString(SR.HttpTransportCannotHaveMultipleAuthenticationSchemes, policyContext.Contract.Namespace, policyContext.Contract.Name))); } foundAssertion = true; httpAuthAssertions.Add(assertion); } httpAuthAssertions.ForEach(delegate(XmlElement element) { bindingAssertions.Remove(element); }); OnImportPolicy(importer, policyContext); } void IWsdlExportExtension.ExportContract(WsdlExporter exporter, WsdlContractConversionContext context) { } void IWsdlExportExtension.ExportEndpoint(WsdlExporter exporter, WsdlEndpointConversionContext endpointContext) { bool createdNew; MessageEncodingBindingElement encodingBindingElement = FindMessageEncodingBindingElement(endpointContext, out createdNew); TransportBindingElement.ExportWsdlEndpoint(exporter, endpointContext, this.WsdlTransportUri, encodingBindingElement.MessageVersion.Addressing); } internal override bool IsMatch(BindingElement b) { if (!base.IsMatch(b)) return false; HttpTransportBindingElement http = b as HttpTransportBindingElement; if (http == null) return false; if (this.allowCookies != http.allowCookies) return false; if (this.authenticationScheme != http.authenticationScheme) return false; if (this.hostNameComparisonMode != http.hostNameComparisonMode) return false; if (this.inheritBaseAddressSettings != http.inheritBaseAddressSettings) return false; if (this.keepAliveEnabled != http.keepAliveEnabled) return false; if (this.maxBufferSize != http.maxBufferSize) return false; if (this.method != http.method) return false; if (this.proxyAddress != http.proxyAddress) return false; if (this.proxyAuthenticationScheme != http.proxyAuthenticationScheme) return false; if (this.realm != http.realm) return false; if (this.transferMode != http.transferMode) return false; if (this.unsafeConnectionNtlmAuthentication != http.unsafeConnectionNtlmAuthentication) return false; if (this.useDefaultWebProxy != http.useDefaultWebProxy) return false; if (this.webProxy != http.webProxy) return false; return true; } MessageEncodingBindingElement FindMessageEncodingBindingElement(BindingElementCollection bindingElements, out bool createdNew) { createdNew = false; MessageEncodingBindingElement encodingBindingElement = bindingElements.Find (); if (encodingBindingElement == null) { createdNew = true; encodingBindingElement = new TextMessageEncodingBindingElement(); } return encodingBindingElement; } MessageEncodingBindingElement FindMessageEncodingBindingElement(WsdlEndpointConversionContext endpointContext, out bool createdNew) { BindingElementCollection bindingElements = endpointContext.Endpoint.Binding.CreateBindingElements(); return FindMessageEncodingBindingElement(bindingElements, out createdNew); } class BindingDeliveryCapabilitiesHelper : IBindingDeliveryCapabilities { internal BindingDeliveryCapabilitiesHelper() { } bool IBindingDeliveryCapabilities.AssuresOrderedDelivery { get { return false; } } bool IBindingDeliveryCapabilities.QueuedDelivery { get { return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
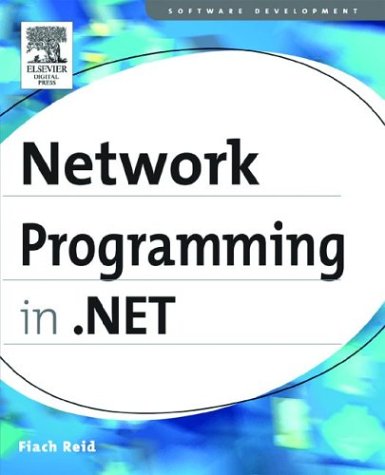
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageEntitySetMapping.cs
- SimpleWebHandlerParser.cs
- SqlTopReducer.cs
- PageRequestManager.cs
- ImageCodecInfo.cs
- HelpProvider.cs
- SQLRoleProvider.cs
- IERequestCache.cs
- LineUtil.cs
- PointConverter.cs
- PenThread.cs
- DefaultParameterValueAttribute.cs
- Identity.cs
- NameValueConfigurationElement.cs
- XmlIlTypeHelper.cs
- TableTextElementCollectionInternal.cs
- DesignerDataStoredProcedure.cs
- RepeaterItemEventArgs.cs
- HebrewNumber.cs
- XmlSyndicationContent.cs
- CallbackValidatorAttribute.cs
- Int16AnimationUsingKeyFrames.cs
- GridItemPattern.cs
- DetailsViewModeEventArgs.cs
- ControlBuilder.cs
- TriggerCollection.cs
- CaseInsensitiveOrdinalStringComparer.cs
- WebResponse.cs
- IpcPort.cs
- InitializationEventAttribute.cs
- SHA256.cs
- CollectionViewProxy.cs
- TracePayload.cs
- SHA1.cs
- LabelInfo.cs
- TripleDES.cs
- ColorConvertedBitmap.cs
- Transform3DGroup.cs
- Calendar.cs
- FocusManager.cs
- MetadataItemSerializer.cs
- designeractionlistschangedeventargs.cs
- ConnectionPoolManager.cs
- DataSourceCollectionBase.cs
- StrongTypingException.cs
- safelink.cs
- NameTable.cs
- DetailsViewUpdatedEventArgs.cs
- UpdateManifestForBrowserApplication.cs
- EUCJPEncoding.cs
- AdornerDecorator.cs
- FocusChangedEventArgs.cs
- PageAsyncTask.cs
- InputScopeAttribute.cs
- SpellerInterop.cs
- PackWebRequestFactory.cs
- DataGrid.cs
- HiddenField.cs
- SecurityAccessDeniedException.cs
- ArraySubsetEnumerator.cs
- InvocationExpression.cs
- ProcessProtocolHandler.cs
- UrlMappingCollection.cs
- OSFeature.cs
- InstallerTypeAttribute.cs
- RequestCachePolicy.cs
- CharacterBuffer.cs
- RowToFieldTransformer.cs
- EmissiveMaterial.cs
- GridViewColumn.cs
- PinProtectionHelper.cs
- RegexReplacement.cs
- AccessDataSource.cs
- VBIdentifierName.cs
- BamlResourceContent.cs
- RectAnimationClockResource.cs
- MaskPropertyEditor.cs
- oledbmetadatacolumnnames.cs
- ExpressionPrefixAttribute.cs
- OutputScope.cs
- BulletDecorator.cs
- RtfControlWordInfo.cs
- SiteIdentityPermission.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ProviderCollection.cs
- AssemblyResourceLoader.cs
- CheckBox.cs
- MimeObjectFactory.cs
- ContainerVisual.cs
- SchemaCollectionPreprocessor.cs
- IndicFontClient.cs
- SafeNativeMethodsMilCoreApi.cs
- CommentEmitter.cs
- Attributes.cs
- ResourceSet.cs
- PriorityRange.cs
- AnnotationComponentChooser.cs
- Processor.cs
- HttpRuntime.cs
- SmtpTransport.cs