Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / Localizer / BamlLocalizer.cs / 3 / BamlLocalizer.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizer.cs // // Contents: BamlLocalizer class, part of Baml Localization API // // Created: 3/17/2004 Garyyang // History: 8/3/2004 Move to System.Windows namespace // 11/29/2004 Garyyang Move to System.Windows.Markup.Localization namespace // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace // // //----------------------------------------------------------------------- using System; using System.IO; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Windows.Markup; using System.Diagnostics; using System.Text; using System.Reflection; using System.Windows; using MS.Internal.Globalization; namespace System.Windows.Markup.Localizer { ////// BamlLocalizer class localizes a baml stram. /// public class BamlLocalizer { //----------------------------------- // constructor //----------------------------------- ////// Constructor /// /// source baml stream to be localized public BamlLocalizer(Stream source) : this (source, null) { } ////// Constructor /// /// source baml stream to be localized /// Localizability resolver implemented by client public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver ) : this (source, resolver, null) { } ////// constructor /// /// source baml straem to be localized /// Localizability resolver implemented by client /// TextReader to read localization comments XML public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver, TextReader comments ) { if (source == null) { throw new ArgumentNullException("source"); } _tree = BamlResourceDeserializer.LoadBaml(source); // create a Baml Localization Enumerator _bamlTreeMap = new BamlTreeMap(this, _tree, resolver, comments); } ////// Extract localizable resources from the source baml. /// ///Localizable resources returned in the form of BamlLoaclizationDictionary public BamlLocalizationDictionary ExtractResources() { return _bamlTreeMap.LocalizationDictionary.Copy(); } ////// Udpate the source baml into a target stream with all the resource udpates applied. /// /// target stream /// resource updates to be applied when generating the localized baml public void UpdateBaml( Stream target, BamlLocalizationDictionary updates ) { if (target == null) { throw new ArgumentNullException("target"); } if (updates == null) { throw new ArgumentNullException("updates"); } // we duplicate the internal baml tree here because // we will need to modify the tree to do generation // UpdateBaml can be called multiple times BamlTree _duplicateTree = _tree.Copy(); _bamlTreeMap.EnsureMap(); // Udpate the tree BamlTreeUpdater.UpdateTree( _duplicateTree, _bamlTreeMap, updates ); // Serialize the tree into Baml BamlResourceSerializer.Serialize( this, _duplicateTree, target ); } ////// Raised by BamlLocalizer when it encounters any abnormal conditions. /// ////// BamlLocalizer can normally ignore the errors and continue the localization. /// As a result, the output baml will only be partially localized. The client /// can choose to stop the BamlLocalizer on error by throw exceptions in their /// event handlers. /// public event BamlLocalizerErrorNotifyEventHandler ErrorNotify; ////// Raise ErrorNotify event /// protected virtual void OnErrorNotify(BamlLocalizerErrorNotifyEventArgs e) { BamlLocalizerErrorNotifyEventHandler handler = ErrorNotify; if (handler != null) { handler(this, e); } } //---------------------------------- // Internal method //---------------------------------- internal void RaiseErrorNotifyEvent(BamlLocalizerErrorNotifyEventArgs e) { OnErrorNotify(e); } //---------------------------------- // Private member //---------------------------------- private BamlTreeMap _bamlTreeMap; private BamlTree _tree; } ////// The delegate for the BamlLocalizer.ErrorNotify event /// public delegate void BamlLocalizerErrorNotifyEventHandler(object sender, BamlLocalizerErrorNotifyEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizer.cs // // Contents: BamlLocalizer class, part of Baml Localization API // // Created: 3/17/2004 Garyyang // History: 8/3/2004 Move to System.Windows namespace // 11/29/2004 Garyyang Move to System.Windows.Markup.Localization namespace // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace // // //----------------------------------------------------------------------- using System; using System.IO; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Windows.Markup; using System.Diagnostics; using System.Text; using System.Reflection; using System.Windows; using MS.Internal.Globalization; namespace System.Windows.Markup.Localizer { ////// BamlLocalizer class localizes a baml stram. /// public class BamlLocalizer { //----------------------------------- // constructor //----------------------------------- ////// Constructor /// /// source baml stream to be localized public BamlLocalizer(Stream source) : this (source, null) { } ////// Constructor /// /// source baml stream to be localized /// Localizability resolver implemented by client public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver ) : this (source, resolver, null) { } ////// constructor /// /// source baml straem to be localized /// Localizability resolver implemented by client /// TextReader to read localization comments XML public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver, TextReader comments ) { if (source == null) { throw new ArgumentNullException("source"); } _tree = BamlResourceDeserializer.LoadBaml(source); // create a Baml Localization Enumerator _bamlTreeMap = new BamlTreeMap(this, _tree, resolver, comments); } ////// Extract localizable resources from the source baml. /// ///Localizable resources returned in the form of BamlLoaclizationDictionary public BamlLocalizationDictionary ExtractResources() { return _bamlTreeMap.LocalizationDictionary.Copy(); } ////// Udpate the source baml into a target stream with all the resource udpates applied. /// /// target stream /// resource updates to be applied when generating the localized baml public void UpdateBaml( Stream target, BamlLocalizationDictionary updates ) { if (target == null) { throw new ArgumentNullException("target"); } if (updates == null) { throw new ArgumentNullException("updates"); } // we duplicate the internal baml tree here because // we will need to modify the tree to do generation // UpdateBaml can be called multiple times BamlTree _duplicateTree = _tree.Copy(); _bamlTreeMap.EnsureMap(); // Udpate the tree BamlTreeUpdater.UpdateTree( _duplicateTree, _bamlTreeMap, updates ); // Serialize the tree into Baml BamlResourceSerializer.Serialize( this, _duplicateTree, target ); } ////// Raised by BamlLocalizer when it encounters any abnormal conditions. /// ////// BamlLocalizer can normally ignore the errors and continue the localization. /// As a result, the output baml will only be partially localized. The client /// can choose to stop the BamlLocalizer on error by throw exceptions in their /// event handlers. /// public event BamlLocalizerErrorNotifyEventHandler ErrorNotify; ////// Raise ErrorNotify event /// protected virtual void OnErrorNotify(BamlLocalizerErrorNotifyEventArgs e) { BamlLocalizerErrorNotifyEventHandler handler = ErrorNotify; if (handler != null) { handler(this, e); } } //---------------------------------- // Internal method //---------------------------------- internal void RaiseErrorNotifyEvent(BamlLocalizerErrorNotifyEventArgs e) { OnErrorNotify(e); } //---------------------------------- // Private member //---------------------------------- private BamlTreeMap _bamlTreeMap; private BamlTree _tree; } ////// The delegate for the BamlLocalizer.ErrorNotify event /// public delegate void BamlLocalizerErrorNotifyEventHandler(object sender, BamlLocalizerErrorNotifyEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
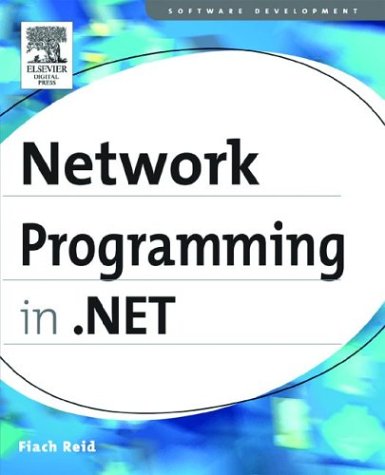
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityStoreSchemaFilterEntry.cs
- BindingMAnagerBase.cs
- UrlMappingsSection.cs
- CharacterString.cs
- XmlSchemaDatatype.cs
- CancellationTokenRegistration.cs
- OdbcConnectionHandle.cs
- EditorZoneBase.cs
- DependentList.cs
- Style.cs
- ServiceNameCollection.cs
- TextDecorationCollection.cs
- HttpResponseHeader.cs
- TreeNodeBindingCollection.cs
- DataGridViewRowEventArgs.cs
- InfoCardSymmetricAlgorithm.cs
- DescendantQuery.cs
- PerformanceCounterPermissionAttribute.cs
- elementinformation.cs
- JulianCalendar.cs
- GPPOINTF.cs
- ResourcePool.cs
- ConditionalAttribute.cs
- BitmapData.cs
- ElementUtil.cs
- ResourceKey.cs
- Column.cs
- ToolZoneDesigner.cs
- BitmapEffectState.cs
- HttpCapabilitiesEvaluator.cs
- InheritablePropertyChangeInfo.cs
- CatalogZone.cs
- WSHttpBindingCollectionElement.cs
- MembershipValidatePasswordEventArgs.cs
- SerializationEventsCache.cs
- FixedSOMLineCollection.cs
- XmlUtilWriter.cs
- CheckedListBox.cs
- DispatcherHooks.cs
- LineServicesRun.cs
- EntityAdapter.cs
- RuntimeArgumentHandle.cs
- LayoutTable.cs
- OrderByExpression.cs
- StringFormat.cs
- ProfileService.cs
- PathFigure.cs
- WindowsPen.cs
- FormatConvertedBitmap.cs
- DataGridClipboardHelper.cs
- AssertUtility.cs
- InfoCardHelper.cs
- CommandHelpers.cs
- GlyphInfoList.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- NetDataContractSerializer.cs
- WebPartManagerInternals.cs
- XamlSerializer.cs
- CustomWebEventKey.cs
- XmlUtil.cs
- MaterialCollection.cs
- CheckBoxFlatAdapter.cs
- SoundPlayer.cs
- XmlEncodedRawTextWriter.cs
- DataComponentMethodGenerator.cs
- TriggerAction.cs
- WebConfigurationHost.cs
- EdgeProfileValidation.cs
- PackWebRequestFactory.cs
- SqlException.cs
- _TransmitFileOverlappedAsyncResult.cs
- CommunicationObject.cs
- Positioning.cs
- TreeViewImageKeyConverter.cs
- LayoutManager.cs
- EditorZone.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- ListViewSelectEventArgs.cs
- TextSelectionHighlightLayer.cs
- DataViewSettingCollection.cs
- ComUdtElementCollection.cs
- WebServiceFault.cs
- PropertyTab.cs
- ObjectListCommandsPage.cs
- LinqTreeNodeEvaluator.cs
- ApplicationGesture.cs
- DataFormats.cs
- XamlReaderHelper.cs
- ContextMenu.cs
- ClientSettings.cs
- DescendantBaseQuery.cs
- TripleDESCryptoServiceProvider.cs
- ToolStripSystemRenderer.cs
- sitestring.cs
- XmlTextEncoder.cs
- SystemIPInterfaceProperties.cs
- UrlMappingCollection.cs
- OleDbDataAdapter.cs
- JournalEntryStack.cs
- Paragraph.cs