Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / security / system / security / authentication / ExtendedProtection / ServiceNameCollection.cs / 1 / ServiceNameCollection.cs
using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Collections; using System.Diagnostics; namespace System.Security.Authentication.ExtendedProtection { // derived from ReadOnlyCollectionBase because it needs to be back ported to .Net 1.x public class ServiceNameCollection : ReadOnlyCollectionBase { public ServiceNameCollection(ICollection items) { if (items == null) { throw new ArgumentNullException("items"); } InnerList.AddRange(items); } public ServiceNameCollection Merge(string serviceName) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); AddIfNew(newServiceNames, serviceName); ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } public ServiceNameCollection Merge(IEnumerable serviceNames) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); // we have a pretty bad performance here: O(n^2), but since service name lists should // be small (<<50) and Merge() should not be called frequently, this shouldn't be an issue foreach (object item in serviceNames) { AddIfNew(newServiceNames, item as string); } ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } private void AddIfNew(ArrayList newServiceNames, string serviceName) { if (String.IsNullOrEmpty(serviceName)) { throw new ArgumentException(SR.GetString(SR.security_ServiceNameCollection_EmptyServiceName)); } if (!Contains(serviceName, newServiceNames)) { newServiceNames.Add(serviceName); } } private bool Contains(string searchServiceName, ICollection serviceNames) { Debug.Assert(serviceNames != null); Debug.Assert(!String.IsNullOrEmpty(searchServiceName)); bool found = false; foreach (string serviceName in serviceNames) { if (String.Compare(serviceName, searchServiceName, StringComparison.InvariantCultureIgnoreCase) == 0) { found = true; break; } } return found; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Collections; using System.Diagnostics; namespace System.Security.Authentication.ExtendedProtection { // derived from ReadOnlyCollectionBase because it needs to be back ported to .Net 1.x public class ServiceNameCollection : ReadOnlyCollectionBase { public ServiceNameCollection(ICollection items) { if (items == null) { throw new ArgumentNullException("items"); } InnerList.AddRange(items); } public ServiceNameCollection Merge(string serviceName) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); AddIfNew(newServiceNames, serviceName); ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } public ServiceNameCollection Merge(IEnumerable serviceNames) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); // we have a pretty bad performance here: O(n^2), but since service name lists should // be small (<<50) and Merge() should not be called frequently, this shouldn't be an issue foreach (object item in serviceNames) { AddIfNew(newServiceNames, item as string); } ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } private void AddIfNew(ArrayList newServiceNames, string serviceName) { if (String.IsNullOrEmpty(serviceName)) { throw new ArgumentException(SR.GetString(SR.security_ServiceNameCollection_EmptyServiceName)); } if (!Contains(serviceName, newServiceNames)) { newServiceNames.Add(serviceName); } } private bool Contains(string searchServiceName, ICollection serviceNames) { Debug.Assert(serviceNames != null); Debug.Assert(!String.IsNullOrEmpty(searchServiceName)); bool found = false; foreach (string serviceName in serviceNames) { if (String.Compare(serviceName, searchServiceName, StringComparison.InvariantCultureIgnoreCase) == 0) { found = true; break; } } return found; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
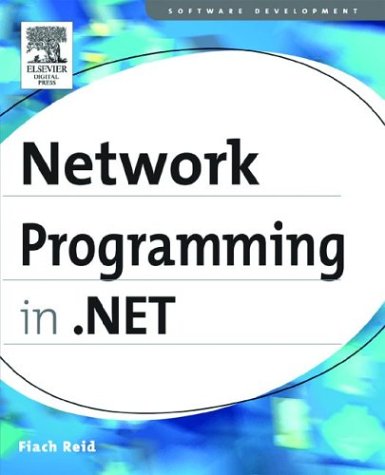
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlQualifiedName.cs
- SystemWebSectionGroup.cs
- Win32MouseDevice.cs
- InheritanceRules.cs
- WinEventTracker.cs
- RadioButton.cs
- Encoder.cs
- BitmapEffectDrawingContextState.cs
- ResolveMatchesMessageCD1.cs
- EncodingNLS.cs
- NumberFunctions.cs
- ActiveXHelper.cs
- FakeModelItemImpl.cs
- XsdBuildProvider.cs
- DiscoveryDocumentSearchPattern.cs
- TrackingProfile.cs
- SystemIPAddressInformation.cs
- ProxyHwnd.cs
- TextRangeEditLists.cs
- DetailsViewModeEventArgs.cs
- OdbcInfoMessageEvent.cs
- DecimalSumAggregationOperator.cs
- activationcontext.cs
- MailMessageEventArgs.cs
- TypeExtensionSerializer.cs
- TdsParserSafeHandles.cs
- TypeFieldSchema.cs
- TypedDatasetGenerator.cs
- HashMembershipCondition.cs
- XmlSerializerNamespaces.cs
- Vector3DAnimationBase.cs
- FixedSOMFixedBlock.cs
- Line.cs
- DetailsViewUpdatedEventArgs.cs
- XmlComplianceUtil.cs
- IntSecurity.cs
- SqlConnectionString.cs
- QueryContext.cs
- ApplicationDirectoryMembershipCondition.cs
- AppDomainProtocolHandler.cs
- GroupBox.cs
- XmlChildNodes.cs
- TripleDES.cs
- Rotation3DAnimationBase.cs
- CollectionConverter.cs
- VBIdentifierDesigner.xaml.cs
- DeploymentSection.cs
- _NetRes.cs
- MachineKeySection.cs
- SequenceDesigner.xaml.cs
- UnorderedHashRepartitionStream.cs
- _RequestCacheProtocol.cs
- DropDownList.cs
- CompositeClientFormatter.cs
- DoubleAverageAggregationOperator.cs
- WebPartActionVerb.cs
- StyleTypedPropertyAttribute.cs
- OrderedDictionaryStateHelper.cs
- PenThread.cs
- BindingListCollectionView.cs
- WMICapabilities.cs
- Codec.cs
- BitConverter.cs
- TransformConverter.cs
- ReadOnlyPropertyMetadata.cs
- CommandHelpers.cs
- ObservableDictionary.cs
- StylusPlugInCollection.cs
- SynchronizedInputAdaptor.cs
- ToolStripItemImageRenderEventArgs.cs
- WebPartCatalogAddVerb.cs
- ExpressionNormalizer.cs
- StickyNote.cs
- TraceHandlerErrorFormatter.cs
- TerminatorSinks.cs
- PageWrapper.cs
- SiteIdentityPermission.cs
- SHA1.cs
- ValidatedControlConverter.cs
- DependencyPropertyConverter.cs
- Int32Animation.cs
- BlobPersonalizationState.cs
- SimpleType.cs
- NameSpaceExtractor.cs
- Cursors.cs
- Pts.cs
- SystemThemeKey.cs
- DtrList.cs
- Section.cs
- DesignerValidatorAdapter.cs
- TextOutput.cs
- basenumberconverter.cs
- StatusBarItem.cs
- ImageAnimator.cs
- TextTreeRootTextBlock.cs
- FormViewRow.cs
- StylusLogic.cs
- GridViewUpdateEventArgs.cs
- LambdaCompiler.Address.cs
- SingleAnimationUsingKeyFrames.cs