Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / XamlIntegration / ActivityXamlServices.cs / 1305376 / ActivityXamlServices.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.XamlIntegration { using System; using System.Collections.Generic; using System.ComponentModel; using System.IO; using System.Reflection; using System.Xaml; using System.Xml; public static class ActivityXamlServices { static readonly XamlSchemaContext dynamicActivityReaderSchemaContext = new DynamicActivityReaderSchemaContext(); public static Activity Load(Stream stream) { if (stream == null) { throw FxTrace.Exception.ArgumentNull("stream"); } using (XmlReader xmlReader = XmlReader.Create(stream)) { return Load(xmlReader); } } public static Activity Load(string fileName) { if (fileName == null) { throw FxTrace.Exception.ArgumentNull("fileName"); } using (XmlReader xmlReader = XmlReader.Create(fileName)) { return Load(xmlReader); } } public static Activity Load(TextReader textReader) { if (textReader == null) { throw FxTrace.Exception.ArgumentNull("textReader"); } using (XmlReader xmlReader = XmlReader.Create(textReader)) { return Load(xmlReader); } } public static Activity Load(XmlReader xmlReader) { if (xmlReader == null) { throw FxTrace.Exception.ArgumentNull("xmlReader"); } using (XamlXmlReader xamlReader = new XamlXmlReader(xmlReader, dynamicActivityReaderSchemaContext)) { return Load(xamlReader); } } public static Activity Load(XamlReader xamlReader) { if (xamlReader == null) { throw FxTrace.Exception.ArgumentNull("xamlReader"); } DynamicActivityXamlReader dynamicActivityReader = new DynamicActivityXamlReader(xamlReader); object xamlObject = XamlServices.Load(dynamicActivityReader); Activity result = xamlObject as Activity; if (result == null) { throw FxTrace.Exception.Argument("reader", SR.ActivityXamlServicesRequiresActivity( xamlObject != null ? xamlObject.GetType().FullName : string.Empty)); } return result; } public static XamlReader CreateReader(Stream stream) { if (stream == null) { throw FxTrace.Exception.ArgumentNull("stream"); } return CreateReader(new XamlXmlReader(XmlReader.Create(stream), dynamicActivityReaderSchemaContext), dynamicActivityReaderSchemaContext); } public static XamlReader CreateReader(XamlReader innerReader) { if (innerReader == null) { throw FxTrace.Exception.ArgumentNull("innerReader"); } return new DynamicActivityXamlReader(innerReader); } public static XamlReader CreateReader(XamlReader innerReader, XamlSchemaContext schemaContext) { if (innerReader == null) { throw FxTrace.Exception.ArgumentNull("innerReader"); } if (schemaContext == null) { throw FxTrace.Exception.ArgumentNull("schemaContext"); } return new DynamicActivityXamlReader(innerReader, schemaContext); } public static XamlReader CreateBuilderReader(XamlReader innerReader) { if (innerReader == null) { throw FxTrace.Exception.ArgumentNull("innerReader"); } return new DynamicActivityXamlReader(true, innerReader, null); } public static XamlReader CreateBuilderReader(XamlReader innerReader, XamlSchemaContext schemaContext) { if (innerReader == null) { throw FxTrace.Exception.ArgumentNull("innerReader"); } if (schemaContext == null) { throw FxTrace.Exception.ArgumentNull("schemaContext"); } return new DynamicActivityXamlReader(true, innerReader, schemaContext); } public static XamlWriter CreateBuilderWriter(XamlWriter innerWriter) { if (innerWriter == null) { throw FxTrace.Exception.ArgumentNull("innerWriter"); } return new ActivityBuilderXamlWriter(innerWriter); } class DynamicActivityReaderSchemaContext : XamlSchemaContext { static bool serviceModelLoaded; const string serviceModelDll = "System.ServiceModel, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"; const string serviceModelActivitiesDll = "System.ServiceModel.Activities, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35"; const string serviceModelNamespace = "http://schemas.microsoft.com/netfx/2009/xaml/servicemodel"; // Eventually this will be unnecessary since XAML team has changed the default behavior public DynamicActivityReaderSchemaContext() : base(new XamlSchemaContextSettings()) { } protected override XamlType GetXamlType(string xamlNamespace, string name, params XamlType[] typeArguments) { XamlType xamlType = base.GetXamlType(xamlNamespace, name, typeArguments); if (xamlType == null) { if (xamlNamespace == serviceModelNamespace && !serviceModelLoaded) { Assembly.Load(serviceModelDll); Assembly.Load(serviceModelActivitiesDll); serviceModelLoaded = true; xamlType = base.GetXamlType(xamlNamespace, name, typeArguments); } } return xamlType; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
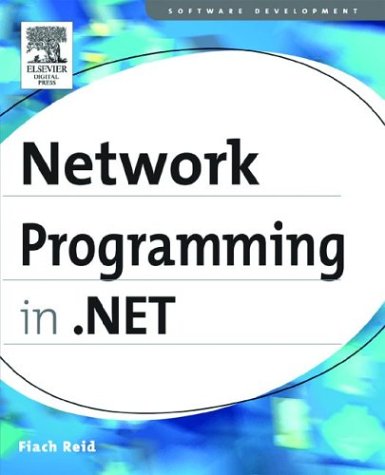
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VerificationException.cs
- LineGeometry.cs
- XmlSchemaCollection.cs
- DocumentAutomationPeer.cs
- WinFormsSecurity.cs
- HttpCacheVaryByContentEncodings.cs
- ValidatorCompatibilityHelper.cs
- LayoutTableCell.cs
- BrowsableAttribute.cs
- RSAPKCS1SignatureFormatter.cs
- GridViewSortEventArgs.cs
- ExtensionWindow.cs
- EdmToObjectNamespaceMap.cs
- EventLogHandle.cs
- LoginUtil.cs
- DescriptionAttribute.cs
- WindowsListViewItemStartMenu.cs
- InternalCache.cs
- SecureEnvironment.cs
- SqlDataSourceStatusEventArgs.cs
- SystemDropShadowChrome.cs
- ProviderException.cs
- ContextBase.cs
- BaseAppDomainProtocolHandler.cs
- PaintEvent.cs
- SecondaryIndex.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- BitmapEffectDrawingContent.cs
- CryptoApi.cs
- GraphicsPath.cs
- ResolveMatches11.cs
- SpellCheck.cs
- unitconverter.cs
- SiteMembershipCondition.cs
- SolidBrush.cs
- TextElementEnumerator.cs
- PropertyInfoSet.cs
- XhtmlTextWriter.cs
- TextEffect.cs
- EventHandlerList.cs
- SafeHandle.cs
- CompilationSection.cs
- MetadataItemEmitter.cs
- BamlRecords.cs
- ManagementOperationWatcher.cs
- DataGridViewCellCollection.cs
- ParseElementCollection.cs
- DependencyStoreSurrogate.cs
- RemoveStoryboard.cs
- PartialList.cs
- OrthographicCamera.cs
- DiscoveryInnerClientAdhoc11.cs
- PointAnimationClockResource.cs
- SQLCharsStorage.cs
- DuplicateWaitObjectException.cs
- AssemblySettingAttributes.cs
- HighlightComponent.cs
- RegexStringValidatorAttribute.cs
- Cell.cs
- COM2ExtendedTypeConverter.cs
- ModelItem.cs
- _PooledStream.cs
- Point3DCollectionValueSerializer.cs
- TrackingMemoryStream.cs
- Transform3DGroup.cs
- SchemaDeclBase.cs
- ObjectPropertyMapping.cs
- CodeAttributeArgumentCollection.cs
- HttpHandlerAction.cs
- BinaryConverter.cs
- FileDialogCustomPlace.cs
- DelayDesigner.cs
- Trace.cs
- WindowsTitleBar.cs
- ConditionalAttribute.cs
- Gdiplus.cs
- smtpconnection.cs
- DocumentXPathNavigator.cs
- ObjectSet.cs
- SQLMoney.cs
- ExceptionHelpers.cs
- DataGridViewAdvancedBorderStyle.cs
- DesignerDataColumn.cs
- ReachSerializerAsync.cs
- SqlConnectionPoolGroupProviderInfo.cs
- JsonFormatGeneratorStatics.cs
- Membership.cs
- DesignerSerializerAttribute.cs
- DynamicScriptObject.cs
- FlowDocument.cs
- DSACryptoServiceProvider.cs
- ManagementQuery.cs
- ConfigurationElement.cs
- TypeBrowserDialog.cs
- RootBrowserWindowAutomationPeer.cs
- JsonByteArrayDataContract.cs
- ArraySubsetEnumerator.cs
- SByte.cs
- XamlParser.cs
- PersonalizationStateInfoCollection.cs