Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2ExtendedTypeConverter.cs / 1 / COM2ExtendedTypeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Diagnostics; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using Microsoft.Win32; using System.Globalization; using System.Collections; ////// /// Base class for value editors that extend basic functionality. /// calls will be delegated to the "base value editor". /// internal class Com2ExtendedTypeConverter : TypeConverter { private TypeConverter innerConverter; public Com2ExtendedTypeConverter(TypeConverter innerConverter) { this.innerConverter = innerConverter; } public Com2ExtendedTypeConverter(Type baseType) { this.innerConverter = TypeDescriptor.GetConverter(baseType); } public TypeConverter InnerConverter { get { return innerConverter; } } public TypeConverter GetWrappedConverter(Type t) { TypeConverter converter = innerConverter; while (converter != null) { if (t.IsInstanceOfType(converter)) { return converter; } if (converter is Com2ExtendedTypeConverter) { converter = ((Com2ExtendedTypeConverter)converter).InnerConverter; } else { break; } } return null; } ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (innerConverter != null) { return innerConverter.CanConvertFrom(context, sourceType); } return base.CanConvertFrom(context, sourceType); } ////// /// Determines if this converter can convert an object to the given destination /// type. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (innerConverter != null) { return innerConverter.CanConvertTo(context, destinationType); } return base.CanConvertTo(context, destinationType); } ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (innerConverter != null) { return innerConverter.ConvertFrom(context, culture, value); } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (innerConverter != null) { return innerConverter.ConvertTo(context, culture, value, destinationType); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Creates an instance of this type given a set of property values /// for the object. This is useful for objects that are immutable, but still /// want to provide changable properties. /// public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { if (innerConverter != null) { return innerConverter.CreateInstance(context, propertyValues); } return base.CreateInstance(context, propertyValues); } ////// /// Determines if changing a value on this object should require a call to /// CreateInstance to create a new value. /// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { if (innerConverter != null) { return innerConverter.GetCreateInstanceSupported(context); } return base.GetCreateInstanceSupported(context); } ////// /// Retrieves the set of properties for this type. By default, a type has /// does not return any properties. An easy implementation of this method /// can just call TypeDescriptor.GetProperties for the correct data type. /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { if (innerConverter != null) { return innerConverter.GetProperties(context, value, attributes); } return base.GetProperties(context, value, attributes); } ////// /// Determines if this object supports properties. By default, this /// is false. /// public override bool GetPropertiesSupported(ITypeDescriptorContext context) { if (innerConverter != null) { return innerConverter.GetPropertiesSupported(context); } return base.GetPropertiesSupported(context); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (innerConverter != null) { return innerConverter.GetStandardValues(context); } return base.GetStandardValues(context); } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { if (innerConverter != null) { return innerConverter.GetStandardValuesExclusive(context); } return base.GetStandardValuesExclusive(context); } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { if (innerConverter != null) { return innerConverter.GetStandardValuesSupported(context); } return base.GetStandardValuesSupported(context); } ////// /// Determines if the given object value is valid for this type. /// public override bool IsValid(ITypeDescriptorContext context, object value) { if (innerConverter != null) { return innerConverter.IsValid(context, value); } return base.IsValid(context, value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
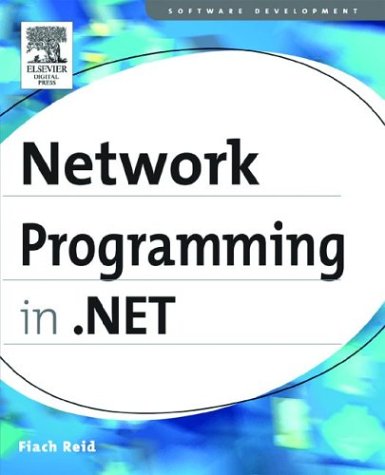
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputBinder.cs
- ClientBuildManagerCallback.cs
- PbrsForward.cs
- AxisAngleRotation3D.cs
- CFGGrammar.cs
- PasswordTextContainer.cs
- TransformPattern.cs
- KeyEventArgs.cs
- ellipse.cs
- EventlogProvider.cs
- CollectionCodeDomSerializer.cs
- GestureRecognizer.cs
- Evidence.cs
- FontFamilyValueSerializer.cs
- QilGenerator.cs
- WebConfigurationHostFileChange.cs
- CachedPathData.cs
- FaultDesigner.cs
- CounterCreationDataCollection.cs
- AttributeQuery.cs
- SchemaTableOptionalColumn.cs
- UnitControl.cs
- PriorityRange.cs
- ExpressionPrefixAttribute.cs
- CompleteWizardStep.cs
- BitmapEffectGroup.cs
- QilReplaceVisitor.cs
- RawContentTypeMapper.cs
- DrawingCollection.cs
- EntityDataSourceUtil.cs
- ComNativeDescriptor.cs
- Table.cs
- DurableInstanceManager.cs
- AssociationProvider.cs
- CodeVariableReferenceExpression.cs
- MsmqInputChannelListener.cs
- KeyValuePairs.cs
- _SSPIWrapper.cs
- GlobalItem.cs
- UnsafeNativeMethods.cs
- DataGridLengthConverter.cs
- TextRunTypographyProperties.cs
- LinearKeyFrames.cs
- ToolStripGrip.cs
- AdditionalEntityFunctions.cs
- coordinator.cs
- ModulesEntry.cs
- AttachmentService.cs
- DataConnectionHelper.cs
- InvalidProgramException.cs
- UnsafeCollabNativeMethods.cs
- HandlerFactoryCache.cs
- WpfMemberInvoker.cs
- ThrowOnMultipleAssignment.cs
- ExpressionNormalizer.cs
- WindowsListViewScroll.cs
- SimpleApplicationHost.cs
- Stacktrace.cs
- UxThemeWrapper.cs
- TypeConstant.cs
- SpeechSeg.cs
- UiaCoreApi.cs
- ContentPresenter.cs
- ClientOperationFormatterProvider.cs
- TypeUnloadedException.cs
- DnsPermission.cs
- AssemblyNameProxy.cs
- TextElement.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- DataGridTablesFactory.cs
- SafeRegistryHandle.cs
- AffineTransform3D.cs
- FontDialog.cs
- SchemaNotation.cs
- ConfigurationStrings.cs
- ReadContentAsBinaryHelper.cs
- WorkflowElementDialog.cs
- XmlNotation.cs
- RegistryPermission.cs
- ObjectAnimationUsingKeyFrames.cs
- CheckoutException.cs
- WebPartUserCapability.cs
- CursorInteropHelper.cs
- EventLogPermissionEntry.cs
- Dispatcher.cs
- TabletCollection.cs
- EmulateRecognizeCompletedEventArgs.cs
- RawTextInputReport.cs
- WebBrowserNavigatedEventHandler.cs
- HostProtectionPermission.cs
- HashAlgorithm.cs
- BamlReader.cs
- ItemChangedEventArgs.cs
- UserControlFileEditor.cs
- NumberSubstitution.cs
- RotateTransform3D.cs
- MimeParameters.cs
- TextStore.cs
- PermissionSetEnumerator.cs
- ViewGenResults.cs