Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / SolidBrush.cs / 1305376 / SolidBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; using System.Runtime.Versioning; ////// /// public sealed class SolidBrush : Brush, ISystemColorTracker { // GDI+ doesn't understand system colors, so we need to cache the value here private Color color = Color.Empty; private bool immutable; /** * Create a new solid fill brush object */ ////// Defines a brush made up of a single color. Brushes are /// used to fill graphics shapes such as rectangles, ellipses, pies, polygons, and paths. /// ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public SolidBrush(Color color) { this.color = color; IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateSolidFill(this.color.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); if (color.IsSystemColor) SystemColorTracker.Add(this); } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] internal SolidBrush(Color color, bool immutable) : this(color) { this.immutable = immutable; } ////// Initializes a new instance of the ///class of the specified /// color. /// /// Constructor to initialized this object from a GDI+ Brush native pointer. /// internal SolidBrush( IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrushInternal( nativeBrush ); } ////// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // We intentionally lose the "immutable" bit. return new SolidBrush(cloneBrush); } ///. /// protected override void Dispose(bool disposing) { if (!disposing) { immutable = false; } else if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } base.Dispose(disposing); } /// /// /// public Color Color { get { if (this.color == Color.Empty) { int colorARGB = 0; int status = SafeNativeMethods.Gdip.GdipGetSolidFillColor(new HandleRef(this, this.NativeBrush), out colorARGB); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = Color.FromArgb(colorARGB); } // GDI+ doesn't understand system colors, so we can't use GdipGetSolidFillColor in the general case return this.color; } set { if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } if( this.color != value ) { Color oldColor = this.color; InternalSetColor(value); // if (value.IsSystemColor && !oldColor.IsSystemColor) { SystemColorTracker.Add(this); } } } } // Sets the color even if the brush is considered immutable private void InternalSetColor(Color value) { int status = SafeNativeMethods.Gdip.GdipSetSolidFillColor(new HandleRef(this, this.NativeBrush), value.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = value; } ////// The color of this ///. /// /// void ISystemColorTracker.OnSystemColorChanged() { if( this.NativeBrush != IntPtr.Zero ){ InternalSetColor(color); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
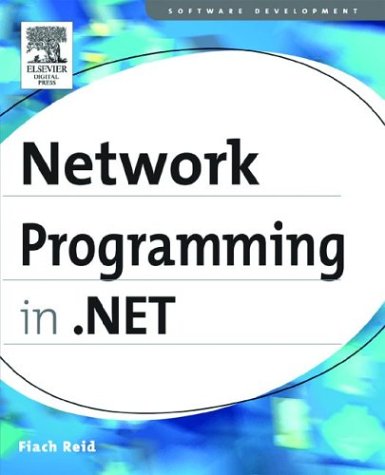
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConvertTextFrag.cs
- _NTAuthentication.cs
- FtpWebRequest.cs
- PrintSchema.cs
- DataGridViewRowPrePaintEventArgs.cs
- OneWayBindingElementImporter.cs
- ButtonAutomationPeer.cs
- SerializationEventsCache.cs
- TimeEnumHelper.cs
- IisTraceWebEventProvider.cs
- XmlDataSourceNodeDescriptor.cs
- UserUseLicenseDictionaryLoader.cs
- _ProxyChain.cs
- MissingSatelliteAssemblyException.cs
- BitmapCacheBrush.cs
- InvokeHandlers.cs
- DataGridViewRowHeaderCell.cs
- URL.cs
- DirtyTextRange.cs
- Form.cs
- Renderer.cs
- Double.cs
- ConcurrentDictionary.cs
- EntityTypeBase.cs
- DeflateStream.cs
- MemberDescriptor.cs
- NavigatingCancelEventArgs.cs
- CollectionCodeDomSerializer.cs
- SecurityTokenInclusionMode.cs
- StoreItemCollection.cs
- HwndKeyboardInputProvider.cs
- Wildcard.cs
- SafeFileHandle.cs
- SubclassTypeValidatorAttribute.cs
- String.cs
- CanonicalFontFamilyReference.cs
- WebRequestModuleElementCollection.cs
- ApplicationBuildProvider.cs
- RelationshipManager.cs
- HttpWriter.cs
- ClientConfigurationHost.cs
- RoutedEvent.cs
- RoutingChannelExtension.cs
- DataGridViewBand.cs
- EventsTab.cs
- GradientBrush.cs
- _HTTPDateParse.cs
- SupportsEventValidationAttribute.cs
- WebBrowserPermission.cs
- MissingSatelliteAssemblyException.cs
- PartialCachingControl.cs
- IconConverter.cs
- DocumentEventArgs.cs
- VisualTreeHelper.cs
- DataTableMapping.cs
- TextModifierScope.cs
- XmlEntityReference.cs
- mediaeventshelper.cs
- TemplateKeyConverter.cs
- RelationshipNavigation.cs
- SpanIndex.cs
- EncoderFallback.cs
- ConfigurationHandlersInstallComponent.cs
- MatchNoneMessageFilter.cs
- ObjectConverter.cs
- ConnectionInterfaceCollection.cs
- MobileUITypeEditor.cs
- WebCategoryAttribute.cs
- BinaryMethodMessage.cs
- HandlerFactoryCache.cs
- ResourceExpression.cs
- ChtmlCommandAdapter.cs
- MDIClient.cs
- SendingRequestEventArgs.cs
- Int32CollectionConverter.cs
- VerticalAlignConverter.cs
- CommandExpr.cs
- ElementNotEnabledException.cs
- OrderedDictionaryStateHelper.cs
- HMACSHA1.cs
- StreamGeometryContext.cs
- DataPager.cs
- CompiledIdentityConstraint.cs
- ActiveXHelper.cs
- BreakRecordTable.cs
- SqlBuilder.cs
- RuleSetDialog.cs
- versioninfo.cs
- future.cs
- SqlDataSourceWizardForm.cs
- HiddenFieldPageStatePersister.cs
- HttpApplicationFactory.cs
- CodeDOMProvider.cs
- mansign.cs
- Matrix3D.cs
- TextEditorContextMenu.cs
- StoragePropertyMapping.cs
- DataTemplateKey.cs
- DropTarget.cs
- ClientUtils.cs