Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / ObjectConverter.cs / 1305376 / ObjectConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * An object that can convert from one object type to another, potentially using a format * string if the conversion is to a string. * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System.Runtime.Serialization.Formatters; using System.ComponentModel; using System.Globalization; using System; using System.Security.Permissions; // ////// /// [Obsolete("The recommended alternative is System.Convert and String.Format. http://go.microsoft.com/fwlink/?linkid=14202")] public sealed class ObjectConverter { internal static readonly char [] formatSeparator = new char[] { ','}; public ObjectConverter() { } ////// /// public static object ConvertValue(object value, Type toType, string formatString) { // Workaround for now to handle inability of reflection to deal with Null. if (value == null || Convert.IsDBNull(value)) { return value; } Type fromType = value.GetType(); if (toType.IsAssignableFrom(fromType)) { return value; } // for now, just hit the sweet spots. if (typeof(string).IsAssignableFrom(fromType)) { if (typeof(int).IsAssignableFrom(toType)) { return Convert.ToInt32((string)value, CultureInfo.InvariantCulture); } else if (typeof(bool).IsAssignableFrom(toType)) { return Convert.ToBoolean((string)value, CultureInfo.InvariantCulture); } else if (typeof(DateTime).IsAssignableFrom(toType)) { return Convert.ToDateTime((string)value, CultureInfo.InvariantCulture); } else if (typeof(Decimal).IsAssignableFrom(toType)) { TypeConverter tc = new DecimalConverter(); return tc.ConvertFromInvariantString((string)value); } else if (typeof(Double).IsAssignableFrom(toType)) { return Convert.ToDouble((string)value, CultureInfo.InvariantCulture); } else if (typeof(Int16).IsAssignableFrom(toType)) { return Convert.ToInt16((Int16)value, CultureInfo.InvariantCulture); } else { throw new ArgumentException( SR.GetString(SR.Cannot_convert_from_to, fromType.ToString(), toType.ToString())); } } else if (typeof(string).IsAssignableFrom(toType)) { if (typeof(int).IsAssignableFrom(fromType)) { return ((int)value).ToString(formatString, CultureInfo.InvariantCulture); } else if (typeof(bool).IsAssignableFrom(fromType)) { string [] tokens=null; if (formatString != null) { tokens = formatString.Split(formatSeparator); if (tokens.Length != 2) { tokens = null; } } if ((bool)value) { if (tokens != null) { return tokens[0]; } else { return "true"; } } else { if (tokens != null) { return tokens[1]; } else { return "false"; } } } else if (typeof(DateTime).IsAssignableFrom(fromType)) { return((DateTime)value).ToString(formatString, CultureInfo.InvariantCulture); } else if (typeof(Decimal).IsAssignableFrom(fromType)) { return ((Decimal)value).ToString(formatString, CultureInfo.InvariantCulture); } else if (typeof(Double).IsAssignableFrom(fromType)) { return ((Double)value).ToString(formatString, CultureInfo.InvariantCulture); } else if (typeof(Single).IsAssignableFrom(fromType)) { return ((Single)value).ToString(formatString, CultureInfo.InvariantCulture); } else if (typeof(Int16).IsAssignableFrom(fromType)) { return ((Int16)value).ToString(formatString, CultureInfo.InvariantCulture); } else { throw new ArgumentException( SR.GetString(SR.Cannot_convert_from_to, fromType.ToString(), toType.ToString())); } } else { throw new ArgumentException( SR.GetString(SR.Cannot_convert_from_to, fromType.ToString(), toType.ToString())); } } /* string t1; int t2; bool t3; DateTime t4; bool t5; Currency t6; string t7, t8, t9, t10, t11; string t12; void Test() { t1 = (string)ObjectConverter.ConvertValue("Should be a string", typeof(string), null); t2 = (int)ObjectConverter.ConvertValue("32", typeof(int), null); t3 = (bool)ObjectConverter.ConvertValue("true", typeof(bool), null); t4 = (DateTime)ObjectConverter.ConvertValue("11/14/62", typeof(DateTime), null); t5 = (bool)ObjectConverter.ConvertValue("false", typeof(bool), null); t6 = (Currency)ObjectConverter.ConvertValue("$12.50", typeof(Currency), null); t7 = (string)ObjectConverter.ConvertValue(32, typeof(string), "#"); t8 = (string)ObjectConverter.ConvertValue(true, typeof(string), "yes;no"); t9 = (string)ObjectConverter.ConvertValue(false, typeof(string), "yes;no"); t10 = (string)ObjectConverter.ConvertValue(DateTime.Now, typeof(string), "hh:mm"); t11 = (string)ObjectConverter.ConvertValue(new Currency(12), typeof(string), "C"); } public static void Main(string[] args) { new ObjectConverter().Test(); } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
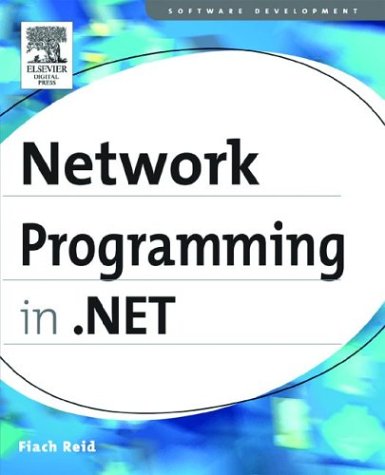
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DropTarget.cs
- EditingMode.cs
- XmlChoiceIdentifierAttribute.cs
- EditBehavior.cs
- DesignerCalendarAdapter.cs
- SchemaNamespaceManager.cs
- RepeaterCommandEventArgs.cs
- followingsibling.cs
- UriParserTemplates.cs
- PersonalizationProviderCollection.cs
- ThemeDirectoryCompiler.cs
- DesignerToolStripControlHost.cs
- BoundField.cs
- EtwTrace.cs
- CallId.cs
- TextTreeTextBlock.cs
- ListViewTableRow.cs
- Size.cs
- TableParagraph.cs
- PrintPageEvent.cs
- WebPartExportVerb.cs
- InteropEnvironment.cs
- WindowsPrincipal.cs
- PointLight.cs
- WorkflowElementDialog.cs
- CollectionViewSource.cs
- Matrix3D.cs
- TreeViewCancelEvent.cs
- PropVariant.cs
- BamlLocalizer.cs
- listitem.cs
- ThicknessConverter.cs
- NotifyIcon.cs
- BehaviorEditorPart.cs
- DesignBindingPropertyDescriptor.cs
- SurrogateDataContract.cs
- httpstaticobjectscollection.cs
- Label.cs
- ContentDefinition.cs
- Parallel.cs
- XmlWriter.cs
- ReflectPropertyDescriptor.cs
- Console.cs
- InstanceHandleConflictException.cs
- LinearGradientBrush.cs
- ObjectHandle.cs
- QilPatternVisitor.cs
- FormViewInsertEventArgs.cs
- StringReader.cs
- DataContext.cs
- JournalNavigationScope.cs
- complextypematerializer.cs
- SessionPageStatePersister.cs
- SelectedCellsChangedEventArgs.cs
- AgileSafeNativeMemoryHandle.cs
- EmptyEnumerator.cs
- DocumentXPathNavigator.cs
- TextTreePropertyUndoUnit.cs
- DbConnectionStringBuilder.cs
- TransformerInfoCollection.cs
- ThrowHelper.cs
- ListViewSelectEventArgs.cs
- NameValueCollection.cs
- CodeSnippetExpression.cs
- Aggregates.cs
- QuaternionValueSerializer.cs
- Image.cs
- RadioButtonList.cs
- SwitchLevelAttribute.cs
- TypeConverter.cs
- GifBitmapEncoder.cs
- StrongNameUtility.cs
- HttpListenerException.cs
- XmlSchemaValidationException.cs
- FakeModelItemImpl.cs
- controlskin.cs
- UrlEncodedParameterWriter.cs
- NetMsmqBinding.cs
- smtppermission.cs
- SqlVersion.cs
- CrossSiteScriptingValidation.cs
- TraceData.cs
- VisualTransition.cs
- VisualStyleInformation.cs
- ControlValuePropertyAttribute.cs
- tibetanshape.cs
- ReflectionTypeLoadException.cs
- NativeMethods.cs
- ToolStripPanel.cs
- SystemTcpConnection.cs
- ViewCellRelation.cs
- TypeUtils.cs
- StringCollection.cs
- LogRecordSequence.cs
- ButtonBaseAdapter.cs
- ZipIOCentralDirectoryFileHeader.cs
- LocalizationComments.cs
- MarkupCompilePass2.cs
- SqlNotificationEventArgs.cs
- MetadataSource.cs