Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / VisualStyles / VisualStyleInformation.cs / 1 / VisualStyleInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA905:SystemAndMicrosoftNamespacesRequireApproval", Scope="namespace", Target="System.Windows.Forms.VisualStyles")] namespace System.Windows.Forms.VisualStyles { using System; using System.Text; using System.Drawing; using System.Windows.Forms; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; ////// /// public static class VisualStyleInformation { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; ////// Provides information about the current visual style. /// NOTE: /// 1) These properties (except SupportByOS, which is always meaningful) are meaningful only /// if visual styles are supported and have currently been applied by the user. /// 2) A subset of these use VisualStyleRenderer objects, so they are /// not meaningful unless VisualStyleRenderer.IsSupported is true. /// ////// /// public static bool IsSupportedByOS { get { return (OSFeature.Feature.IsPresent(OSFeature.Themes)); } } ////// Used to find whether visual styles are supported by the current OS. Same as /// using the OSFeature class to see if themes are supported. /// ////// /// public static bool IsEnabledByUser { get { if (!IsSupportedByOS) { return false; } return (SafeNativeMethods.IsAppThemed()); } } internal static string ThemeFilename { get { if (IsEnabledByUser) { StringBuilder filename = new StringBuilder(512); SafeNativeMethods.GetCurrentThemeName(filename, filename.Capacity, null, 0, null, 0); return (filename.ToString()); } return String.Empty; } } ////// Returns true if a visual style has currently been applied by the user, else false. /// ////// /// The current visual style's color scheme name. /// public static string ColorScheme { get { if (IsEnabledByUser) { StringBuilder colorScheme = new StringBuilder(512); SafeNativeMethods.GetCurrentThemeName(null, 0, colorScheme, colorScheme.Capacity, null, 0); return (colorScheme.ToString()); } return String.Empty; } } ////// /// The current visual style's size name. /// public static string Size { get { if (IsEnabledByUser) { StringBuilder size = new StringBuilder(512); SafeNativeMethods.GetCurrentThemeName(null, 0, null, 0, size, size.Capacity); return (size.ToString()); } return String.Empty; } } ////// /// The current visual style's display name. /// public static string DisplayName { get { if (IsEnabledByUser) { StringBuilder name = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.DisplayName, name, name.Capacity); return name.ToString(); } return String.Empty; } } ////// /// The current visual style's company. /// public static string Company { get { if (IsEnabledByUser) { StringBuilder company = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Company, company, company.Capacity); return company.ToString(); } return String.Empty; } } ////// /// The name of the current visual style's author. /// public static string Author { get { if (IsEnabledByUser) { StringBuilder author = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Author, author, author.Capacity); return author.ToString(); } return String.Empty; } } ////// /// The current visual style's copyright information. /// public static string Copyright { get { if (IsEnabledByUser) { StringBuilder copyright = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Copyright, copyright, copyright.Capacity); return copyright.ToString(); } return String.Empty; } } ////// /// The current visual style's url. /// [SuppressMessage("Microsoft.Design", "CA1056:UriPropertiesShouldNotBeStrings")] public static string Url { [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings")] get { if (IsEnabledByUser) { StringBuilder url = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Url, url, url.Capacity); return url.ToString(); } return String.Empty; } } ////// /// The current visual style's version. /// public static string Version { get { if (IsEnabledByUser) { StringBuilder version = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Version, version, version.Capacity); return version.ToString(); } return String.Empty; } } ////// /// The current visual style's description. /// public static string Description { get { if (IsEnabledByUser) { StringBuilder description = new StringBuilder(512); SafeNativeMethods.GetThemeDocumentationProperty(ThemeFilename, VisualStyleDocProperty.Description, description, description.Capacity); return description.ToString(); } return String.Empty; } } ////// /// Returns true if the current theme supports flat menus, else false. /// public static bool SupportsFlatMenus { get { if (Application.RenderWithVisualStyles) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(VisualStyleElement.Window.Caption.Active); } else { visualStyleRenderer.SetParameters(VisualStyleElement.Window.Caption.Active); } return (SafeNativeMethods.GetThemeSysBool(new HandleRef(null, visualStyleRenderer.Handle), VisualStyleSystemProperty.SupportsFlatMenus)); } return false; } } ////// /// The minimum color depth supported by the current visual style. /// public static int MinimumColorDepth { get { if (Application.RenderWithVisualStyles) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(VisualStyleElement.Window.Caption.Active); } else { visualStyleRenderer.SetParameters(VisualStyleElement.Window.Caption.Active); } int mcDepth = 0; SafeNativeMethods.GetThemeSysInt(new HandleRef(null, visualStyleRenderer.Handle), VisualStyleSystemProperty.MinimumColorDepth, ref mcDepth); return mcDepth; } return 0; } } ////// /// Border Color that Windows XP renders for controls like TextBox and ComboBox. /// public static Color TextControlBorder { get { if (Application.RenderWithVisualStyles) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(VisualStyleElement.TextBox.TextEdit.Normal); } else { visualStyleRenderer.SetParameters(VisualStyleElement.TextBox.TextEdit.Normal); } Color borderColor = visualStyleRenderer.GetColor(ColorProperty.BorderColor); return borderColor; } return SystemColors.WindowFrame; } } ////// /// This is the color buttons and tab pages are highlighted with when they are moused over on themed OS. /// public static Color ControlHighlightHot { get { if (Application.RenderWithVisualStyles) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(VisualStyleElement.Button.PushButton.Normal); } else { visualStyleRenderer.SetParameters(VisualStyleElement.Button.PushButton.Normal); } Color accentColor = visualStyleRenderer.GetColor(ColorProperty.AccentColorHint); return accentColor; } return SystemColors.ButtonHighlight; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
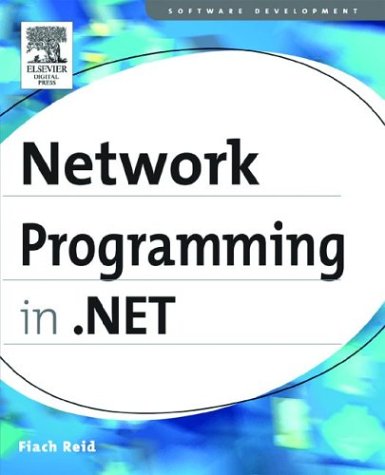
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonBaseAutomationPeer.cs
- Properties.cs
- GradientBrush.cs
- LocalizeDesigner.cs
- SerializationInfoEnumerator.cs
- MultiByteCodec.cs
- SmiGettersStream.cs
- DragEvent.cs
- DBConcurrencyException.cs
- SoapBinding.cs
- COM2FontConverter.cs
- DSASignatureFormatter.cs
- AbsoluteQuery.cs
- ListMarkerLine.cs
- SingleObjectCollection.cs
- DataGridViewTextBoxCell.cs
- XmlAttributeProperties.cs
- SqlDataSourceSelectingEventArgs.cs
- PackWebRequest.cs
- ViewKeyConstraint.cs
- Light.cs
- FacetChecker.cs
- Span.cs
- SettingsProperty.cs
- ValidationEventArgs.cs
- CharacterShapingProperties.cs
- _OverlappedAsyncResult.cs
- ISCIIEncoding.cs
- KeyedCollection.cs
- ClassValidator.cs
- DragCompletedEventArgs.cs
- ScrollChangedEventArgs.cs
- ProfileService.cs
- DynamicAttribute.cs
- BigInt.cs
- VersionPair.cs
- TextViewBase.cs
- EventLogEntry.cs
- ToolbarAUtomationPeer.cs
- Renderer.cs
- NativeMethods.cs
- ArraySegment.cs
- RecipientInfo.cs
- EditingCoordinator.cs
- ServiceNotStartedException.cs
- HttpSysSettings.cs
- WorkflowOperationFault.cs
- GlobalizationSection.cs
- DecoratedNameAttribute.cs
- PagedControl.cs
- SecurityState.cs
- MethodCallConverter.cs
- HuffModule.cs
- SymLanguageType.cs
- IdentityModelDictionary.cs
- DirectoryGroupQuery.cs
- HostingEnvironment.cs
- DropShadowEffect.cs
- ProgressBarHighlightConverter.cs
- LocalizableResourceBuilder.cs
- DateTimeFormatInfo.cs
- VSWCFServiceContractGenerator.cs
- WebControlAdapter.cs
- ValueTable.cs
- ShaderRenderModeValidation.cs
- WindowsSpinner.cs
- ToolTipService.cs
- MediaTimeline.cs
- HighContrastHelper.cs
- TextServicesHost.cs
- SessionParameter.cs
- ContravarianceAdapter.cs
- EditCommandColumn.cs
- MSHTMLHost.cs
- DBNull.cs
- COM2AboutBoxPropertyDescriptor.cs
- Slider.cs
- EntryWrittenEventArgs.cs
- ExpressionStringBuilder.cs
- ObsoleteAttribute.cs
- PermissionSetTriple.cs
- TextComposition.cs
- XPathAxisIterator.cs
- XDRSchema.cs
- ScrollEvent.cs
- LocalizableResourceBuilder.cs
- DispatchOperationRuntime.cs
- RemotingException.cs
- ExtensionDataObject.cs
- GridPattern.cs
- Int32CollectionConverter.cs
- ScrollEvent.cs
- ReachPageContentCollectionSerializer.cs
- PropertyEntry.cs
- StaticSiteMapProvider.cs
- FileInfo.cs
- FilterEventArgs.cs
- EventDescriptorCollection.cs
- wgx_render.cs
- GeometryValueSerializer.cs