Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / IconConverter.cs / 1305376 / IconConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.IO; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.ComponentModel.Design.Serialization; using System.Diagnostics.CodeAnalysis; using System.Runtime.Versioning; ////// /// IconConverter is a class that can be used to convert /// Icon from one data type to another. Access this /// class through the TypeDescriptor. /// public class IconConverter : ExpandableObjectConverter { ////// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(byte[])) { return true; } if(sourceType == typeof(InstanceDescriptor)){ return false; } return base.CanConvertFrom(context, sourceType); } ///Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { return true; } if (destinationType == typeof(byte[])) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is byte[]) { MemoryStream ms = new MemoryStream((byte[])value); return new Icon(ms); } return base.ConvertFrom(context, culture, value); } ///Converts the given object to the converter's native type. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] [SuppressMessage("Microsoft.Reliability", "CA2000:DisposeObjectsBeforeLosingScope")] [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(Image) || destinationType == typeof(Bitmap)) { Icon icon = value as Icon; if( icon != null ) { return icon.ToBitmap(); } } if (destinationType == typeof(string)) { if (value != null) { return value.ToString(); } else { return SR.GetString(SR.toStringNone); } } if (destinationType == typeof(byte[])) { if (value != null) { MemoryStream ms = null; try { ms = new MemoryStream(); Icon icon = value as Icon; if( icon != null ) { icon.Save(ms); } } finally { if (ms != null) { ms.Close(); } } if (ms != null) { return ms.ToArray(); } else { return null; } } else { return new byte[0]; } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
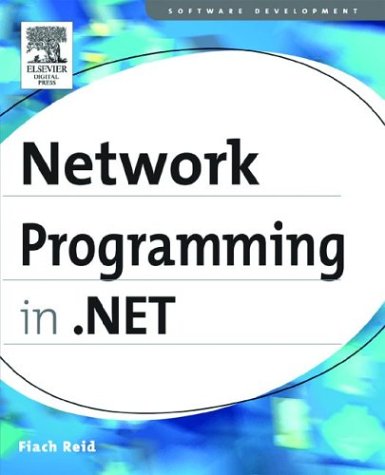
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPipelineRuntime.cs
- DataGridToolTip.cs
- FormatterConverter.cs
- Aggregates.cs
- Token.cs
- CompilerErrorCollection.cs
- SettingsAttributeDictionary.cs
- NativeMethodsOther.cs
- PointLight.cs
- AutoCompleteStringCollection.cs
- PrimitiveOperationFormatter.cs
- XpsFixedDocumentReaderWriter.cs
- OleDbSchemaGuid.cs
- GC.cs
- MULTI_QI.cs
- TablePatternIdentifiers.cs
- SqlConnectionHelper.cs
- ResourceReferenceExpression.cs
- GeneratedView.cs
- CompiledELinqQueryState.cs
- BeginStoryboard.cs
- AtomServiceDocumentSerializer.cs
- AdRotator.cs
- IOThreadTimer.cs
- SqlDataSourceTableQuery.cs
- PassportAuthenticationModule.cs
- Material.cs
- ObjectView.cs
- Trace.cs
- DisplayClaim.cs
- Resources.Designer.cs
- ButtonBaseAdapter.cs
- DesignerAdRotatorAdapter.cs
- ExceptionWrapper.cs
- EntityDataSourceChangingEventArgs.cs
- Accessible.cs
- XmlSchemaGroupRef.cs
- InheritedPropertyChangedEventArgs.cs
- ReadOnlyState.cs
- ProxyHelper.cs
- RelationshipEndCollection.cs
- ToolBar.cs
- BaseComponentEditor.cs
- JsonServiceDocumentSerializer.cs
- BlobPersonalizationState.cs
- XmlNavigatorFilter.cs
- ReadContentAsBinaryHelper.cs
- DetailsViewPageEventArgs.cs
- TraceInternal.cs
- FrameworkTemplate.cs
- RequestCacheValidator.cs
- DatePickerTextBox.cs
- VirtualPath.cs
- SecurityHeaderLayout.cs
- RuleProcessor.cs
- ToolStripDropTargetManager.cs
- RequestTimeoutManager.cs
- NodeFunctions.cs
- ContextMarshalException.cs
- UIElementCollection.cs
- TransformValueSerializer.cs
- ADMembershipProvider.cs
- AssemblyFilter.cs
- DropShadowEffect.cs
- ListViewGroupCollectionEditor.cs
- BindingManagerDataErrorEventArgs.cs
- DriveNotFoundException.cs
- LockCookie.cs
- InheritanceAttribute.cs
- HexParser.cs
- TextRange.cs
- Geometry3D.cs
- ControlUtil.cs
- FilterException.cs
- FigureParaClient.cs
- DataGridViewColumnHeaderCell.cs
- RijndaelManagedTransform.cs
- OleDbConnectionInternal.cs
- NumberSubstitution.cs
- VectorConverter.cs
- ServiceHttpHandlerFactory.cs
- PingReply.cs
- TriggerBase.cs
- PrintController.cs
- CaseKeyBox.ViewModel.cs
- SQLBinary.cs
- KeyValuePairs.cs
- Graph.cs
- dbenumerator.cs
- ToolStripDropDownClosedEventArgs.cs
- ParsedAttributeCollection.cs
- ResourceCategoryAttribute.cs
- WebPartZoneBase.cs
- NullReferenceException.cs
- Zone.cs
- EventItfInfo.cs
- ConfigurationException.cs
- Vector3DCollection.cs
- ColumnMapTranslator.cs
- GenericIdentity.cs