Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / BufferedGraphics.cs / 1 / BufferedGraphics.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.ComponentModel; using System.Collections; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Text; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; ////// /// The BufferedGraphics class can be thought of as a "Token" or "Reference" to the /// buffer that a BufferedGraphicsContext creates. While a BufferedGraphics is /// outstanding, the memory associated with the buffer is locked. The general design /// is such that under normal conditions a single BufferedGraphics will be in use at /// one time for a given BufferedGraphicsContext. /// [SuppressMessage("Microsoft.Usage", "CA2216:DisposableTypesShouldDeclareFinalizer")] public sealed class BufferedGraphics : IDisposable { private Graphics bufferedGraphicsSurface; private Graphics targetGraphics; private BufferedGraphicsContext context; private IntPtr targetDC; private Point targetLoc; private Size virtualSize; private bool disposeContext; private static int rop = 0xcc0020; // RasterOp.SOURCE.GetRop(); ////// /// Internal constructor, this class is created by the BufferedGraphicsContext. /// internal BufferedGraphics(Graphics bufferedGraphicsSurface, BufferedGraphicsContext context, Graphics targetGraphics, IntPtr targetDC, Point targetLoc, Size virtualSize) { this.context = context; this.bufferedGraphicsSurface = bufferedGraphicsSurface; this.targetDC = targetDC; this.targetGraphics = targetGraphics; this.targetLoc = targetLoc; this.virtualSize = virtualSize; } ~BufferedGraphics() { Dispose(false); } ////// /// Disposes the object and releases the lock on the memory. /// public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { if (context != null) { context.ReleaseBuffer(this); if (DisposeContext) { context.Dispose(); context = null; } } if (bufferedGraphicsSurface != null) { bufferedGraphicsSurface.Dispose(); bufferedGraphicsSurface = null; } } } ////// /// Internal property - determines if we need to dispose of the Context when this is disposed /// internal bool DisposeContext { get { return disposeContext; } set { disposeContext = value; } } ////// /// Allows access to the Graphics wrapper for the buffer. /// public Graphics Graphics { get { Debug.Assert(bufferedGraphicsSurface != null, "The BufferedGraphicsSurface is null!"); return bufferedGraphicsSurface; } } ////// /// Renders the buffer to the original graphics used to allocate the buffer. /// public void Render() { if (targetGraphics != null) { Render(targetGraphics); } else { RenderInternal(new HandleRef(Graphics, targetDC), this); } } ////// /// Renders the buffer to the specified target graphics. /// public void Render(Graphics target) { if (target != null) { IntPtr targetDC = target.GetHdc(); try { RenderInternal(new HandleRef(target, targetDC), this); } finally { target.ReleaseHdcInternal(targetDC); } } } ////// /// Renders the buffer to the specified target HDC. /// public void Render(IntPtr targetDC) { IntSecurity.UnmanagedCode.Demand(); RenderInternal(new HandleRef(null, targetDC), this); } ////// /// Internal method that renders the specified buffer into the target. /// private void RenderInternal(HandleRef refTargetDC, BufferedGraphics buffer) { IntPtr sourceDC = buffer.Graphics.GetHdc(); try { SafeNativeMethods.BitBlt(refTargetDC, targetLoc.X, targetLoc.Y, virtualSize.Width, virtualSize.Height, new HandleRef(buffer.Graphics, sourceDC), 0, 0, rop); } finally { buffer.Graphics.ReleaseHdcInternal(sourceDC); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
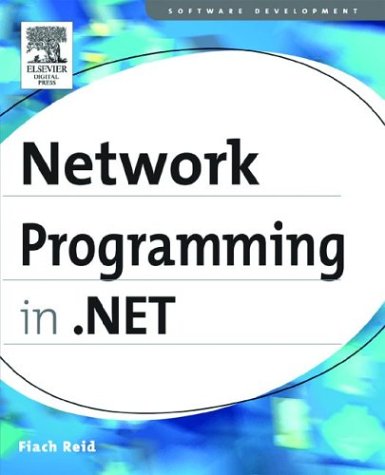
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlServer2KCompatibilityCheck.cs
- CheckableControlBaseAdapter.cs
- InputDevice.cs
- Rect3D.cs
- CacheChildrenQuery.cs
- ThreadStaticAttribute.cs
- LineBreakRecord.cs
- TextEditorThreadLocalStore.cs
- AppDomain.cs
- TextProperties.cs
- SoapSchemaMember.cs
- HttpConfigurationContext.cs
- AsyncPostBackErrorEventArgs.cs
- AbstractSvcMapFileLoader.cs
- FormViewDeletedEventArgs.cs
- RegexCompilationInfo.cs
- SystemTcpConnection.cs
- ProjectionPlan.cs
- NavigatorOutput.cs
- Events.cs
- Control.cs
- Application.cs
- PropertyMappingExceptionEventArgs.cs
- MemoryMappedFile.cs
- SqlProviderManifest.cs
- FixedSOMElement.cs
- Style.cs
- HtmlInputButton.cs
- InteropDesigner.xaml.cs
- AssemblyInfo.cs
- listitem.cs
- LoginViewDesigner.cs
- AppModelKnownContentFactory.cs
- DataTableMappingCollection.cs
- COM2PictureConverter.cs
- ConnectionInterfaceCollection.cs
- TextParentUndoUnit.cs
- ContextStaticAttribute.cs
- GeometryConverter.cs
- DataGridViewColumnCollection.cs
- ArithmeticLiteral.cs
- CompiledAction.cs
- ReverseInheritProperty.cs
- AutomationPeer.cs
- SinglePageViewer.cs
- RuntimeArgumentHandle.cs
- PropertyPushdownHelper.cs
- Hex.cs
- ConstNode.cs
- LocatorManager.cs
- DataGridRowHeaderAutomationPeer.cs
- DynamicPropertyReader.cs
- Control.cs
- mda.cs
- ModifyActivitiesPropertyDescriptor.cs
- ModelItemDictionaryImpl.cs
- Html32TextWriter.cs
- GuidelineCollection.cs
- TlsSspiNegotiation.cs
- StoreAnnotationsMap.cs
- UnicodeEncoding.cs
- FrameworkContentElement.cs
- XmlSchemaFacet.cs
- CodeLinePragma.cs
- BinaryMethodMessage.cs
- ShaperBuffers.cs
- Rotation3DAnimation.cs
- BlockCollection.cs
- OrderedDictionary.cs
- CodeSnippetTypeMember.cs
- BindingGraph.cs
- LoaderAllocator.cs
- XmlSchemaAttributeGroup.cs
- MsmqOutputMessage.cs
- SByte.cs
- ScrollProviderWrapper.cs
- CancelAsyncOperationRequest.cs
- PersonalizationStateQuery.cs
- CodeObject.cs
- tooltip.cs
- Registry.cs
- WindowsSolidBrush.cs
- RowBinding.cs
- VolatileEnlistmentState.cs
- DataGridViewRow.cs
- ConstraintManager.cs
- DateBoldEvent.cs
- Compiler.cs
- DataGridViewRowCancelEventArgs.cs
- UdpMessageProperty.cs
- InfoCardListRequest.cs
- OLEDB_Enum.cs
- ReleaseInstanceMode.cs
- CalendarTable.cs
- UIElement3D.cs
- TextEditorDragDrop.cs
- DataRow.cs
- EntityContainerEntitySetDefiningQuery.cs
- OptimizedTemplateContentHelper.cs
- SchemeSettingElementCollection.cs