Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilList.cs / 1 / QilList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil operator having N children. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilList : QilNode { private int count; private QilNode[] members; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new (empty) QilList /// public QilList(QilNodeType nodeType) : base(nodeType) { this.members = new QilNode[4]; this.xmlType = null; } //----------------------------------------------- // QilNode methods //----------------------------------------------- ////// Lazily create the XmlQueryType. /// public override XmlQueryType XmlType { get { if (this.xmlType == null) { XmlQueryType xt = XmlQueryTypeFactory.Empty; if (this.count > 0) { if (this.nodeType == QilNodeType.Sequence) { for (int i = 0; i < this.count; i++) xt = XmlQueryTypeFactory.Sequence(xt, this.members[i].XmlType); // Sequences do not preserve DocOrderDistinct if (xt.IsDod) xt = XmlQueryTypeFactory.PrimeProduct(XmlQueryTypeFactory.NodeNotRtfS, xt.Cardinality); } else if (this.nodeType == QilNodeType.BranchList) { xt = this.members[0].XmlType; for (int i = 1; i < this.count; i++) xt = XmlQueryTypeFactory.Choice(xt, this.members[i].XmlType); } } this.xmlType = xt; } return this.xmlType; } } ////// Override in order to clone the "members" array. /// public override QilNode ShallowClone(QilFactory f) { QilList n = (QilList) MemberwiseClone(); n.members = (QilNode[]) this.members.Clone(); f.TraceNode(n); return n; } //----------------------------------------------- // IListmethods -- override //----------------------------------------------- public override int Count { get { return this.count; } } public override QilNode this[int index] { get { if (index >= 0 && index < this.count) return this.members[index]; throw new IndexOutOfRangeException(); } set { if (index >= 0 && index < this.count) this.members[index] = value; else throw new IndexOutOfRangeException(); // Invalidate XmlType this.xmlType = null; } } public override void Insert(int index, QilNode node) { if (index < 0 || index > this.count) throw new IndexOutOfRangeException(); if (this.count == this.members.Length) { QilNode[] membersNew = new QilNode[this.count * 2]; Array.Copy(this.members, membersNew, this.count); this.members = membersNew; } if (index < this.count) Array.Copy(this.members, index, this.members, index + 1, this.count - index); this.count++; this.members[index] = node; // Invalidate XmlType this.xmlType = null; } public override void RemoveAt(int index) { if (index < 0 || index >= this.count) throw new IndexOutOfRangeException(); this.count--; if (index < this.count) Array.Copy(this.members, index + 1, this.members, index, this.count - index); this.members[this.count] = null; // Invalidate XmlType this.xmlType = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil operator having N children. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilList : QilNode { private int count; private QilNode[] members; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new (empty) QilList /// public QilList(QilNodeType nodeType) : base(nodeType) { this.members = new QilNode[4]; this.xmlType = null; } //----------------------------------------------- // QilNode methods //----------------------------------------------- ////// Lazily create the XmlQueryType. /// public override XmlQueryType XmlType { get { if (this.xmlType == null) { XmlQueryType xt = XmlQueryTypeFactory.Empty; if (this.count > 0) { if (this.nodeType == QilNodeType.Sequence) { for (int i = 0; i < this.count; i++) xt = XmlQueryTypeFactory.Sequence(xt, this.members[i].XmlType); // Sequences do not preserve DocOrderDistinct if (xt.IsDod) xt = XmlQueryTypeFactory.PrimeProduct(XmlQueryTypeFactory.NodeNotRtfS, xt.Cardinality); } else if (this.nodeType == QilNodeType.BranchList) { xt = this.members[0].XmlType; for (int i = 1; i < this.count; i++) xt = XmlQueryTypeFactory.Choice(xt, this.members[i].XmlType); } } this.xmlType = xt; } return this.xmlType; } } ////// Override in order to clone the "members" array. /// public override QilNode ShallowClone(QilFactory f) { QilList n = (QilList) MemberwiseClone(); n.members = (QilNode[]) this.members.Clone(); f.TraceNode(n); return n; } //----------------------------------------------- // IListmethods -- override //----------------------------------------------- public override int Count { get { return this.count; } } public override QilNode this[int index] { get { if (index >= 0 && index < this.count) return this.members[index]; throw new IndexOutOfRangeException(); } set { if (index >= 0 && index < this.count) this.members[index] = value; else throw new IndexOutOfRangeException(); // Invalidate XmlType this.xmlType = null; } } public override void Insert(int index, QilNode node) { if (index < 0 || index > this.count) throw new IndexOutOfRangeException(); if (this.count == this.members.Length) { QilNode[] membersNew = new QilNode[this.count * 2]; Array.Copy(this.members, membersNew, this.count); this.members = membersNew; } if (index < this.count) Array.Copy(this.members, index, this.members, index + 1, this.count - index); this.count++; this.members[index] = node; // Invalidate XmlType this.xmlType = null; } public override void RemoveAt(int index) { if (index < 0 || index >= this.count) throw new IndexOutOfRangeException(); this.count--; if (index < this.count) Array.Copy(this.members, index + 1, this.members, index, this.count - index); this.members[this.count] = null; // Invalidate XmlType this.xmlType = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
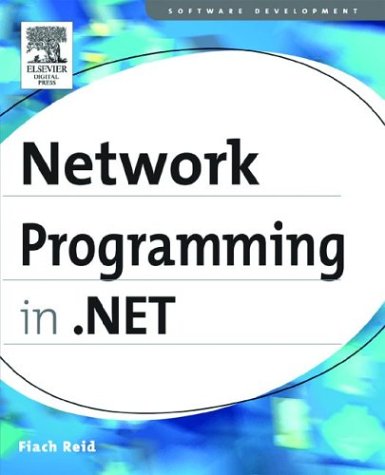
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataDocumentXPathNavigator.cs
- PersistChildrenAttribute.cs
- MemoryRecordBuffer.cs
- smtpconnection.cs
- XmlCharCheckingWriter.cs
- DockProviderWrapper.cs
- _LoggingObject.cs
- PolicyException.cs
- IconConverter.cs
- FontResourceCache.cs
- x509utils.cs
- StreamWriter.cs
- ModuleElement.cs
- ArrayExtension.cs
- XmlSchemaDatatype.cs
- SubMenuStyle.cs
- IssuedTokenClientCredential.cs
- MimeMultiPart.cs
- cookieexception.cs
- OAVariantLib.cs
- WSSecureConversation.cs
- BoolLiteral.cs
- MemoryPressure.cs
- _LocalDataStore.cs
- ProgressBarRenderer.cs
- GridView.cs
- TableLayoutRowStyleCollection.cs
- OleDbConnection.cs
- QilName.cs
- ByteConverter.cs
- DocumentViewerAutomationPeer.cs
- MarshalByValueComponent.cs
- AncestorChangedEventArgs.cs
- RoleGroup.cs
- ControlTemplate.cs
- RowSpanVector.cs
- ValidationErrorEventArgs.cs
- TdsParserStateObject.cs
- SslStream.cs
- Size.cs
- ThumbButtonInfo.cs
- DeviceContexts.cs
- TextCompositionEventArgs.cs
- XmlArrayItemAttribute.cs
- NameNode.cs
- CompatibleComparer.cs
- PlaceHolder.cs
- ProcessProtocolHandler.cs
- GridViewDeletedEventArgs.cs
- CharacterBufferReference.cs
- SevenBitStream.cs
- DataGridRowHeader.cs
- MissingSatelliteAssemblyException.cs
- WinCategoryAttribute.cs
- PropertyMapper.cs
- SQLSingleStorage.cs
- RelationshipConstraintValidator.cs
- ListViewInsertEventArgs.cs
- TemplateControl.cs
- ReadingWritingEntityEventArgs.cs
- CheckBoxRenderer.cs
- SecurityStandardsManager.cs
- TagPrefixAttribute.cs
- TreeNodeBinding.cs
- CodeDomSerializerException.cs
- AssemblyFilter.cs
- ItemContainerGenerator.cs
- AutomationEvent.cs
- KeyManager.cs
- RegisteredArrayDeclaration.cs
- Win32.cs
- SoapIncludeAttribute.cs
- ReachSerializer.cs
- RecognizerStateChangedEventArgs.cs
- figurelength.cs
- XmlIlVisitor.cs
- OdbcHandle.cs
- OdbcParameter.cs
- NativeMethods.cs
- ErrorHandlerModule.cs
- HtmlInputCheckBox.cs
- PowerStatus.cs
- DataGridViewCellValueEventArgs.cs
- CodeVariableReferenceExpression.cs
- ScriptControlDescriptor.cs
- X500Name.cs
- Vector.cs
- ThicknessConverter.cs
- ClientProxyGenerator.cs
- UdpTransportSettingsElement.cs
- RunInstallerAttribute.cs
- SyndicationSerializer.cs
- WriteTimeStream.cs
- ChannelManagerBase.cs
- BuildProvidersCompiler.cs
- PenThreadPool.cs
- OracleNumber.cs
- DrawingGroupDrawingContext.cs
- ServiceX509SecurityTokenProvider.cs
- PersistenceTypeAttribute.cs