Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewGroupConverter.cs / 1 / ListViewGroupConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// ListViewGroupConverter is a class that can be used to convert /// ListViewGroup objects from one data type to another. Access this /// class through the TypeDescriptor. /// internal class ListViewGroupConverter : TypeConverter { ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string) && context != null && context.Instance is ListViewItem) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } if (destinationType == typeof(string) && context != null && context.Instance is ListViewItem) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Trim(); if (context != null && context.Instance != null) { ListViewItem item = context.Instance as ListViewItem; if (item != null && item.ListView != null) { foreach(ListViewGroup group in item.ListView.Groups) { if (group.Header == text) { return group; } } } } } if (value == null || value.Equals(SR.GetString(SR.toStringNone))) { return null; } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is ListViewGroup) { ListViewGroup group = (ListViewGroup)value; ConstructorInfo ctor; // Header // ctor = typeof(ListViewGroup).GetConstructor(new Type[] {typeof(string), typeof(HorizontalAlignment)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { group.Header, group.HeaderAlignment }, false); } } if (destinationType == typeof(string) && value == null) { return SR.GetString(SR.toStringNone); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { ListViewItem item = context.Instance as ListViewItem; if (item != null && item.ListView != null) { ArrayList list = new ArrayList(); foreach (ListViewGroup group in item.ListView.Groups) { list.Add(group); } list.Add(null); return new StandardValuesCollection(list); } } return null; } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return true; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
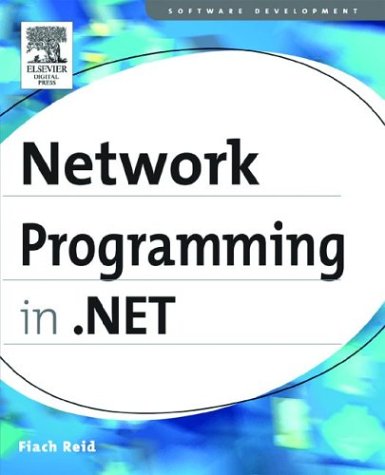
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridGeneralPage.cs
- UnsignedPublishLicense.cs
- DrawingImage.cs
- Size.cs
- LocalizedNameDescriptionPair.cs
- TextFormatter.cs
- OpenTypeCommon.cs
- SessionStateUtil.cs
- DataContractSerializer.cs
- TableItemStyle.cs
- CategoryAttribute.cs
- NameValueFileSectionHandler.cs
- RetriableClipboard.cs
- UnauthorizedAccessException.cs
- CrossAppDomainChannel.cs
- SectionRecord.cs
- TextEditorParagraphs.cs
- JapaneseCalendar.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- EntityContainer.cs
- ValidatingPropertiesEventArgs.cs
- CapabilitiesSection.cs
- SID.cs
- UICuesEvent.cs
- MenuItem.cs
- EntitySqlQueryState.cs
- WmlControlAdapter.cs
- AppSettingsReader.cs
- CategoryGridEntry.cs
- TextBoxBaseDesigner.cs
- DynamicValueConverter.cs
- InitializationEventAttribute.cs
- SQLGuidStorage.cs
- AddInStore.cs
- AsyncOperationManager.cs
- PanelStyle.cs
- WorkflowTraceTransfer.cs
- CompressedStack.cs
- SessionStateSection.cs
- BamlResourceContent.cs
- SynchronousReceiveBehavior.cs
- TdsParserSessionPool.cs
- SecurityTokenSerializer.cs
- Model3DCollection.cs
- EventDescriptorCollection.cs
- DataGridViewColumnEventArgs.cs
- ObjectStateManagerMetadata.cs
- SafeNativeMethods.cs
- IPEndPointCollection.cs
- XmlEncoding.cs
- FixedLineResult.cs
- SafeBitVector32.cs
- MergeLocalizationDirectives.cs
- NavigateUrlConverter.cs
- Cloud.cs
- MdiWindowListStrip.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- SafeRegistryKey.cs
- PtsContext.cs
- NavigationPropertyEmitter.cs
- ToolStripComboBox.cs
- ZipPackage.cs
- DefaultValidator.cs
- Parameter.cs
- SerializationException.cs
- ToolStripScrollButton.cs
- BitVector32.cs
- CustomMenuItemCollection.cs
- LinearQuaternionKeyFrame.cs
- EncoderBestFitFallback.cs
- PerformanceCounterPermission.cs
- ToolStripRenderer.cs
- Base64Encoder.cs
- HostedAspNetEnvironment.cs
- XmlQueryCardinality.cs
- DtdParser.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- WaitHandleCannotBeOpenedException.cs
- DbModificationCommandTree.cs
- PassportAuthenticationEventArgs.cs
- ParseHttpDate.cs
- RemotingConfigParser.cs
- CalendarDateChangedEventArgs.cs
- DictionaryBase.cs
- RenameRuleObjectDialog.cs
- Sequence.cs
- URIFormatException.cs
- EditorZoneBase.cs
- Point3DCollectionValueSerializer.cs
- XPathDocumentBuilder.cs
- storepermissionattribute.cs
- SelfIssuedAuthRSACryptoProvider.cs
- ZipIOModeEnforcingStream.cs
- DictionaryEntry.cs
- EntryPointNotFoundException.cs
- WindowsTab.cs
- ConfigurationPermission.cs
- MergePropertyDescriptor.cs
- FixedPageProcessor.cs
- TraceContext.cs