Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Base64Encoder.cs / 1305376 / Base64Encoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; namespace System.Xml { internal abstract class Base64Encoder { byte[] leftOverBytes; int leftOverBytesCount; char[] charsLine; internal const int Base64LineSize = 76; internal const int LineSizeInBytes = Base64LineSize/4*3; internal Base64Encoder() { charsLine = new char[Base64LineSize]; } internal abstract void WriteChars( char[] chars, int index, int count ); internal void Encode( byte[] buffer, int index, int count ) { if ( buffer == null ) { throw new ArgumentNullException( "buffer" ); } if ( index < 0 ) { throw new ArgumentOutOfRangeException( "index" ); } if ( count < 0 ) { throw new ArgumentOutOfRangeException( "count" ); } if ( count > buffer.Length - index ) { throw new ArgumentOutOfRangeException( "count" ); } // encode left-over buffer if( leftOverBytesCount > 0 ) { int i = leftOverBytesCount; while ( i < 3 && count > 0 ) { leftOverBytes[i++] = buffer[index++]; count--; } // the total number of buffer we have is less than 3 -> return if ( count == 0 && i < 3 ) { leftOverBytesCount = i; return; } // encode the left-over buffer and write out int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, 3, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); } // store new left-over buffer leftOverBytesCount = count % 3; if ( leftOverBytesCount > 0 ) { count -= leftOverBytesCount; if ( leftOverBytes == null ) { leftOverBytes = new byte[3]; } for( int i = 0; i < leftOverBytesCount; i++ ) { leftOverBytes[i] = buffer[ index + count + i ]; } } // encode buffer in 76 character long chunks int endIndex = index + count; int chunkSize = LineSizeInBytes; while( index < endIndex ) { if ( index + chunkSize > endIndex ) { chunkSize = endIndex - index; } int charCount = Convert.ToBase64CharArray( buffer, index, chunkSize, charsLine, 0 ); WriteChars( charsLine, 0, charCount ); index += chunkSize; } } internal void Flush() { if ( leftOverBytesCount > 0 ) { int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, leftOverBytesCount, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); leftOverBytesCount = 0; } } } internal class XmlRawWriterBase64Encoder : Base64Encoder { XmlRawWriter rawWriter; internal XmlRawWriterBase64Encoder( XmlRawWriter rawWriter ) { this.rawWriter = rawWriter; } internal override void WriteChars( char[] chars, int index, int count ) { rawWriter.WriteRaw( chars, index, count ); } } #if !SILVERLIGHT internal class XmlTextWriterBase64Encoder : Base64Encoder { XmlTextEncoder xmlTextEncoder; internal XmlTextWriterBase64Encoder( XmlTextEncoder xmlTextEncoder ) { this.xmlTextEncoder = xmlTextEncoder; } internal override void WriteChars( char[] chars, int index, int count ) { xmlTextEncoder.WriteRaw( chars, index, count ); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
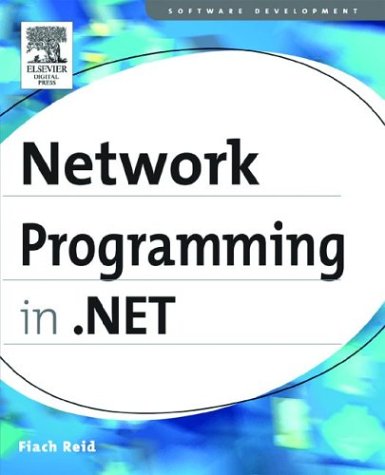
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReflectionServiceProvider.cs
- ClrPerspective.cs
- TextRangeEditTables.cs
- DocumentGridPage.cs
- Int32AnimationUsingKeyFrames.cs
- WebPartConnectionsCancelEventArgs.cs
- LocalTransaction.cs
- OpacityConverter.cs
- Directory.cs
- SafeFindHandle.cs
- Graphics.cs
- EnumMemberAttribute.cs
- GetWinFXPath.cs
- WebPartConnectionsConnectVerb.cs
- MembershipSection.cs
- TreeNodeStyleCollection.cs
- HtmlShimManager.cs
- WebPartsPersonalization.cs
- WmlLabelAdapter.cs
- Char.cs
- CustomTrackingRecord.cs
- RectAnimation.cs
- MimeMultiPart.cs
- ActiveXSite.cs
- NativeMethodsCLR.cs
- FullTextState.cs
- ExcCanonicalXml.cs
- TypedDataSetSchemaImporterExtension.cs
- RichTextBoxConstants.cs
- MeshGeometry3D.cs
- ActivityIdHeader.cs
- IndexedString.cs
- TranslateTransform3D.cs
- WorkflowNamespace.cs
- TransportContext.cs
- ContractInstanceProvider.cs
- SerializableAttribute.cs
- SecurityCriticalDataForSet.cs
- NeedSkipTokenVisitor.cs
- FrameworkContextData.cs
- FormDocumentDesigner.cs
- TextRangeBase.cs
- _UncName.cs
- _DisconnectOverlappedAsyncResult.cs
- SafeHandles.cs
- KeyToListMap.cs
- TypedTableBaseExtensions.cs
- WebPartChrome.cs
- Timer.cs
- ValidationSummary.cs
- Visual3D.cs
- Tracer.cs
- InboundActivityHelper.cs
- HtmlContainerControl.cs
- WindowsTokenRoleProvider.cs
- Wildcard.cs
- Hex.cs
- PermissionAttributes.cs
- BrowserCapabilitiesCodeGenerator.cs
- InfoCardRSACryptoProvider.cs
- ResourceReferenceExpressionConverter.cs
- CreateUserWizard.cs
- ImageList.cs
- ASCIIEncoding.cs
- UdpRetransmissionSettings.cs
- EventProvider.cs
- StylusPlugin.cs
- ColorDialog.cs
- SqlVersion.cs
- XPathArrayIterator.cs
- WindowsScrollBarBits.cs
- ExportOptions.cs
- BatchWriter.cs
- MatrixTransform3D.cs
- SerialStream.cs
- PartialCachingAttribute.cs
- FacetEnabledSchemaElement.cs
- X509Certificate.cs
- DataExpression.cs
- FontSource.cs
- SqlHelper.cs
- TreeViewBindingsEditor.cs
- TypeResolver.cs
- ComUdtElementCollection.cs
- WebPartMinimizeVerb.cs
- StatusBarPanelClickEvent.cs
- Button.cs
- ToolBarPanel.cs
- StringConverter.cs
- InstanceCreationEditor.cs
- HttpResponseHeader.cs
- NameValueSectionHandler.cs
- Monitor.cs
- CodeGen.cs
- StringBuilder.cs
- SplitContainer.cs
- SqlMethodAttribute.cs
- TableLayoutCellPaintEventArgs.cs
- Converter.cs
- RewritingValidator.cs