Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / BuildTasks / Microsoft / Build / Tasks / Windows / GetWinFXPath.cs / 1 / GetWinFXPath.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Return the path for current WinFX runtime. // // // History: // 11/16/2005 weibz Created // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Globalization; using System.Diagnostics; using System.Reflection; using System.Resources; using System.Runtime.InteropServices; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using MS.Utility; using MS.Internal.Tasks; // Since we disable PreSharp warnings in this file, PreSharp warning is unknown to C# compiler. // We first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace Microsoft.Build.Tasks.Windows { #region GetWinFXPath Task class ////// Return the directory of current WinFX run time. /// public sealed class GetWinFXPath : Task { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constrcutor /// public GetWinFXPath() : base(SR.ResourceManager) { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// ITask Execute method /// ///public override bool Execute() { bool ret = true; try { TaskHelper.DisplayLogo(Log, SR.Get(SRID.GetWinFXPathTask)); bool isWow64; isWow64 = IsWow64CLRRun(); if (isWow64) { WinFXPath = WinFXWowPath; } else { WinFXPath = WinFXNativePath; } } catch (Exception e) { // PreSharp Complaint 6500 - do not handle null-ref or SEH exceptions. if (e is NullReferenceException || e is SEHException) { throw; } else { string message; string errorId; errorId = Log.ExtractMessageCode(e.Message, out message); if (String.IsNullOrEmpty(errorId)) { errorId = UnknownErrorID; message = SR.Get(SRID.UnknownBuildError, message); } Log.LogError(null, errorId, null, null, 0, 0, 0, 0, message, null); } ret = false; } #pragma warning disable 6500 catch // Non-CLS compliant errors { Log.LogErrorWithCodeFromResources(SRID.NonClsError); ret = false; } #pragma warning restore 6500 return ret; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties /// /// The path for native WinFX runtime. /// [Required] public string WinFXNativePath { get { return _winFXNativePath; } set { _winFXNativePath = value; } } ////// The path for WoW WinFX run time. /// [Required] public string WinFXWowPath { get { return _winFXWowPath; } set { _winFXWowPath = value; } } ////// The real path for the WinFX runtime /// [Output] public string WinFXPath { get { return _winFXPath; } set { _winFXPath = value; } } #endregion Public Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods // PInvoke delegate for IsWow64Process private delegate bool IsWow64ProcessDelegate([In] IntPtr hProcess, [Out] out bool Wow64Process); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr LoadLibrary(string fileName); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern bool FreeLibrary([In] IntPtr module); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr GetProcAddress(IntPtr module, string procName); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr GetCurrentProcess(); //// Detect if the msbuild is running under WOW64 environment. // static private bool IsWow64CLRRun() { bool isWow64 = false; IntPtr NullIntPtr = new IntPtr(0); IntPtr kernel32Dll = LoadLibrary(Kernel32Dll); if (kernel32Dll != NullIntPtr) { try { IntPtr isWow64ProcessHandle = GetProcAddress(kernel32Dll, IsWow64ProcessMethodName); // if the entry point is missing, it cannot be Wow64 if (isWow64ProcessHandle != NullIntPtr) { // entry point present, check if running in WOW64 IsWow64ProcessDelegate isWow64Process = (IsWow64ProcessDelegate)Marshal.GetDelegateForFunctionPointer(isWow64ProcessHandle, typeof(IsWow64ProcessDelegate)); isWow64Process(GetCurrentProcess(), out isWow64); } } finally { FreeLibrary(kernel32Dll); } } return isWow64; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private string _winFXNativePath = string.Empty; private string _winFXWowPath = string.Empty; private string _winFXPath = string.Empty; private const string UnknownErrorID = "FX1000"; private const string Kernel32Dll = "kernel32.dll"; private const string IsWow64ProcessMethodName = "IsWow64Process"; #endregion Private Fields } #endregion GetWinFXPath Task class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Return the path for current WinFX runtime. // // // History: // 11/16/2005 weibz Created // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Globalization; using System.Diagnostics; using System.Reflection; using System.Resources; using System.Runtime.InteropServices; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using MS.Utility; using MS.Internal.Tasks; // Since we disable PreSharp warnings in this file, PreSharp warning is unknown to C# compiler. // We first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace Microsoft.Build.Tasks.Windows { #region GetWinFXPath Task class ////// Return the directory of current WinFX run time. /// public sealed class GetWinFXPath : Task { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constrcutor /// public GetWinFXPath() : base(SR.ResourceManager) { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// ITask Execute method /// ///public override bool Execute() { bool ret = true; try { TaskHelper.DisplayLogo(Log, SR.Get(SRID.GetWinFXPathTask)); bool isWow64; isWow64 = IsWow64CLRRun(); if (isWow64) { WinFXPath = WinFXWowPath; } else { WinFXPath = WinFXNativePath; } } catch (Exception e) { // PreSharp Complaint 6500 - do not handle null-ref or SEH exceptions. if (e is NullReferenceException || e is SEHException) { throw; } else { string message; string errorId; errorId = Log.ExtractMessageCode(e.Message, out message); if (String.IsNullOrEmpty(errorId)) { errorId = UnknownErrorID; message = SR.Get(SRID.UnknownBuildError, message); } Log.LogError(null, errorId, null, null, 0, 0, 0, 0, message, null); } ret = false; } #pragma warning disable 6500 catch // Non-CLS compliant errors { Log.LogErrorWithCodeFromResources(SRID.NonClsError); ret = false; } #pragma warning restore 6500 return ret; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties /// /// The path for native WinFX runtime. /// [Required] public string WinFXNativePath { get { return _winFXNativePath; } set { _winFXNativePath = value; } } ////// The path for WoW WinFX run time. /// [Required] public string WinFXWowPath { get { return _winFXWowPath; } set { _winFXWowPath = value; } } ////// The real path for the WinFX runtime /// [Output] public string WinFXPath { get { return _winFXPath; } set { _winFXPath = value; } } #endregion Public Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods // PInvoke delegate for IsWow64Process private delegate bool IsWow64ProcessDelegate([In] IntPtr hProcess, [Out] out bool Wow64Process); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr LoadLibrary(string fileName); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern bool FreeLibrary([In] IntPtr module); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr GetProcAddress(IntPtr module, string procName); [DllImport(Kernel32Dll, PreserveSig = true)] private static extern IntPtr GetCurrentProcess(); //// Detect if the msbuild is running under WOW64 environment. // static private bool IsWow64CLRRun() { bool isWow64 = false; IntPtr NullIntPtr = new IntPtr(0); IntPtr kernel32Dll = LoadLibrary(Kernel32Dll); if (kernel32Dll != NullIntPtr) { try { IntPtr isWow64ProcessHandle = GetProcAddress(kernel32Dll, IsWow64ProcessMethodName); // if the entry point is missing, it cannot be Wow64 if (isWow64ProcessHandle != NullIntPtr) { // entry point present, check if running in WOW64 IsWow64ProcessDelegate isWow64Process = (IsWow64ProcessDelegate)Marshal.GetDelegateForFunctionPointer(isWow64ProcessHandle, typeof(IsWow64ProcessDelegate)); isWow64Process(GetCurrentProcess(), out isWow64); } } finally { FreeLibrary(kernel32Dll); } } return isWow64; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private string _winFXNativePath = string.Empty; private string _winFXWowPath = string.Empty; private string _winFXPath = string.Empty; private const string UnknownErrorID = "FX1000"; private const string Kernel32Dll = "kernel32.dll"; private const string IsWow64ProcessMethodName = "IsWow64Process"; #endregion Private Fields } #endregion GetWinFXPath Task class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
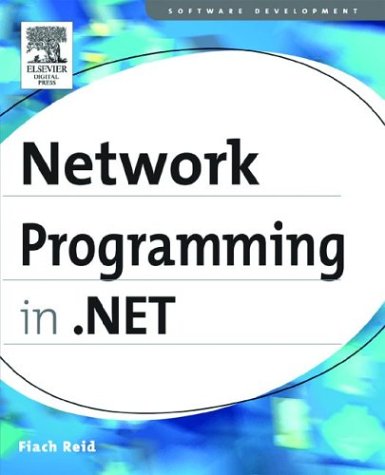
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSource.cs
- XmlSchemaAttributeGroup.cs
- ListViewGroup.cs
- XPathPatternBuilder.cs
- TypeListConverter.cs
- SafeNativeMethods.cs
- MultiAsyncResult.cs
- XmlAnyElementAttribute.cs
- EmptyEnumerator.cs
- ColorConvertedBitmap.cs
- MembershipValidatePasswordEventArgs.cs
- EntityDesignerBuildProvider.cs
- KnownAssembliesSet.cs
- PerfService.cs
- AutoResizedEvent.cs
- DocumentOrderComparer.cs
- NavigationEventArgs.cs
- securestring.cs
- PropertyDescriptor.cs
- InputLanguageCollection.cs
- RegisteredHiddenField.cs
- SoapTypeAttribute.cs
- WebRequestModuleElementCollection.cs
- DropSource.cs
- XhtmlConformanceSection.cs
- XmlSerializerFactory.cs
- StylusPlugInCollection.cs
- PrintDocument.cs
- SessionStateUtil.cs
- DocumentOrderComparer.cs
- CalendarDateRangeChangingEventArgs.cs
- OneOfTypeConst.cs
- ItemsPanelTemplate.cs
- ImageUrlEditor.cs
- SecurityTokenAuthenticator.cs
- WebPartRestoreVerb.cs
- TimelineClockCollection.cs
- WebPartTransformerCollection.cs
- IPEndPoint.cs
- TableParaClient.cs
- COM2Enum.cs
- WebPartCatalogCloseVerb.cs
- _SSPIWrapper.cs
- GeometryGroup.cs
- RuleSettingsCollection.cs
- ExecutionEngineException.cs
- DiscoveryService.cs
- SessionEndedEventArgs.cs
- HttpListenerRequest.cs
- RelativeSource.cs
- UnsupportedPolicyOptionsException.cs
- DependencyPropertyAttribute.cs
- TypedColumnHandler.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- AtomServiceDocumentSerializer.cs
- DataGridViewDataErrorEventArgs.cs
- CqlBlock.cs
- SelectionRangeConverter.cs
- WebPartDisplayModeCollection.cs
- BrowserCapabilitiesFactory.cs
- GraphicsPath.cs
- ObjectDataSourceFilteringEventArgs.cs
- GcSettings.cs
- HwndStylusInputProvider.cs
- RayHitTestParameters.cs
- PermissionListSet.cs
- MetadataHelper.cs
- UrlAuthFailedErrorFormatter.cs
- CommandField.cs
- RotateTransform.cs
- GeneralTransform3DGroup.cs
- CoTaskMemHandle.cs
- DataPagerFieldCollection.cs
- DataTemplateSelector.cs
- recordstatefactory.cs
- ArraySegment.cs
- UpdateRecord.cs
- xsdvalidator.cs
- Keywords.cs
- SecureStringHasher.cs
- BindingExpressionBase.cs
- HostedController.cs
- TextUtf8RawTextWriter.cs
- FrameSecurityDescriptor.cs
- DtdParser.cs
- EventWaitHandleSecurity.cs
- BasicCellRelation.cs
- DataGridAutomationPeer.cs
- DurationConverter.cs
- ErrorHandler.cs
- ProcessThreadDesigner.cs
- XamlSerializerUtil.cs
- Msec.cs
- XmlNamespaceDeclarationsAttribute.cs
- ChangeToolStripParentVerb.cs
- LowerCaseStringConverter.cs
- UnmanagedMemoryStream.cs
- Rfc4050KeyFormatter.cs
- DataGridCellAutomationPeer.cs
- InvokeWebServiceDesigner.cs