Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextLineBreak.cs / 1305600 / TextLineBreak.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextLineBreak.cs // // Contents: Text properties and state at the point where text is broken // by the line breaking process, which may need to be carried over // when formatting the next line. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Security; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using MS.Internal; using MS.Internal.TextFormatting; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// Text properties and state at the point where text is broken /// by the line breaking process. /// public sealed class TextLineBreak : IDisposable { private TextModifierScope _currentScope; private SecurityCriticalDataForSet_breakRecord; #region Constructors /// /// Internallly construct the line break /// internal TextLineBreak( TextModifierScope currentScope, SecurityCriticalDataForSetbreakRecord ) { _currentScope = currentScope; _breakRecord = breakRecord; if (breakRecord.Value == IntPtr.Zero) { // this object does not hold unmanaged resource, // remove it from the finalizer queue. GC.SuppressFinalize(this); } } #endregion /// /// Finalize the line break /// ~TextLineBreak() { DisposeInternal(true); } ////// Dispose the line break /// public void Dispose() { DisposeInternal(false); GC.SuppressFinalize(this); } ////// Clone a new instance of TextLineBreak /// ////// Critical - as this calls unmanaged API LoCloneBreakRecord. /// PublicOK - as it takes no parameter and retain no additional unmanaged resource. /// [SecurityCritical] public TextLineBreak Clone() { IntPtr pbreakrec = IntPtr.Zero; if (_breakRecord.Value != IntPtr.Zero) { LsErr lserr = UnsafeNativeMethods.LoCloneBreakRecord(_breakRecord.Value, out pbreakrec); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.CloneBreakRecordFailure, lserr), lserr); } } return new TextLineBreak(_currentScope, new SecurityCriticalDataForSet(pbreakrec)); } /// /// Destroy LS unmanaged break records object inside the line break /// managed object. The parameter flag indicates whether the call is /// from finalizer thread or the main UI thread. /// ////// Critical - as this calls the setter of _breakRecord.Value which is type SecurityCriticalDataForSet. /// _breakRecord is the value received from call to LoCreateBreaks and being passed back in /// when building the next break. No code should have access to set it otherwise. /// Safe - as this does not take any parameter that it passes directly to the critical function. /// [SecurityCritical, SecurityTreatAsSafe] private void DisposeInternal(bool finalizing) { if (_breakRecord.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposeBreakRecord(_breakRecord.Value, finalizing); _breakRecord.Value = IntPtr.Zero; GC.KeepAlive(this); } } ////// Current text modifier scope, which can be null. /// internal TextModifierScope TextModifierScope { get { return _currentScope; } } ////// Unmanaged pointer to LS break records structure /// internal SecurityCriticalDataForSetBreakRecord { get { return _breakRecord; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextLineBreak.cs // // Contents: Text properties and state at the point where text is broken // by the line breaking process, which may need to be carried over // when formatting the next line. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Security; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using MS.Internal; using MS.Internal.TextFormatting; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { /// /// Text properties and state at the point where text is broken /// by the line breaking process. /// public sealed class TextLineBreak : IDisposable { private TextModifierScope _currentScope; private SecurityCriticalDataForSet_breakRecord; #region Constructors /// /// Internallly construct the line break /// internal TextLineBreak( TextModifierScope currentScope, SecurityCriticalDataForSetbreakRecord ) { _currentScope = currentScope; _breakRecord = breakRecord; if (breakRecord.Value == IntPtr.Zero) { // this object does not hold unmanaged resource, // remove it from the finalizer queue. GC.SuppressFinalize(this); } } #endregion /// /// Finalize the line break /// ~TextLineBreak() { DisposeInternal(true); } ////// Dispose the line break /// public void Dispose() { DisposeInternal(false); GC.SuppressFinalize(this); } ////// Clone a new instance of TextLineBreak /// ////// Critical - as this calls unmanaged API LoCloneBreakRecord. /// PublicOK - as it takes no parameter and retain no additional unmanaged resource. /// [SecurityCritical] public TextLineBreak Clone() { IntPtr pbreakrec = IntPtr.Zero; if (_breakRecord.Value != IntPtr.Zero) { LsErr lserr = UnsafeNativeMethods.LoCloneBreakRecord(_breakRecord.Value, out pbreakrec); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.CloneBreakRecordFailure, lserr), lserr); } } return new TextLineBreak(_currentScope, new SecurityCriticalDataForSet(pbreakrec)); } /// /// Destroy LS unmanaged break records object inside the line break /// managed object. The parameter flag indicates whether the call is /// from finalizer thread or the main UI thread. /// ////// Critical - as this calls the setter of _breakRecord.Value which is type SecurityCriticalDataForSet. /// _breakRecord is the value received from call to LoCreateBreaks and being passed back in /// when building the next break. No code should have access to set it otherwise. /// Safe - as this does not take any parameter that it passes directly to the critical function. /// [SecurityCritical, SecurityTreatAsSafe] private void DisposeInternal(bool finalizing) { if (_breakRecord.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposeBreakRecord(_breakRecord.Value, finalizing); _breakRecord.Value = IntPtr.Zero; GC.KeepAlive(this); } } ////// Current text modifier scope, which can be null. /// internal TextModifierScope TextModifierScope { get { return _currentScope; } } ////// Unmanaged pointer to LS break records structure /// internal SecurityCriticalDataForSetBreakRecord { get { return _breakRecord; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
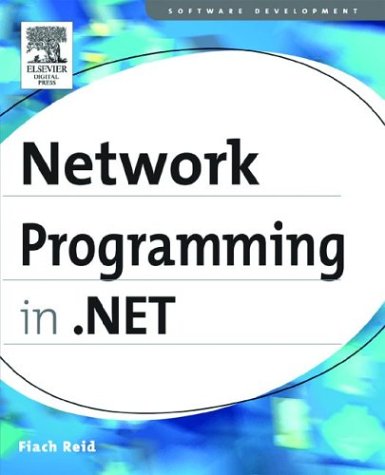
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA384Managed.cs
- Margins.cs
- PropertyEmitter.cs
- InheritanceContextChangedEventManager.cs
- _LoggingObject.cs
- SearchForVirtualItemEventArgs.cs
- SqlConnectionStringBuilder.cs
- compensatingcollection.cs
- TaskbarItemInfo.cs
- GraphicsPathIterator.cs
- PasswordRecovery.cs
- MultiAsyncResult.cs
- Matrix3DConverter.cs
- RegexParser.cs
- HtmlFormWrapper.cs
- SqlTypeSystemProvider.cs
- LinqDataSourceEditData.cs
- SubpageParaClient.cs
- XmlWriterTraceListener.cs
- ApplicationDirectoryMembershipCondition.cs
- IDispatchConstantAttribute.cs
- TileBrush.cs
- DecoderExceptionFallback.cs
- AesCryptoServiceProvider.cs
- ReflectionTypeLoadException.cs
- RequestCacheValidator.cs
- DependentList.cs
- CounterSample.cs
- CommandField.cs
- FreezableDefaultValueFactory.cs
- InternalDispatchObject.cs
- OleDbDataAdapter.cs
- DescendantOverDescendantQuery.cs
- ChangeTracker.cs
- ResourceManager.cs
- Registry.cs
- smtppermission.cs
- DirectionalLight.cs
- ScriptingJsonSerializationSection.cs
- ScriptReferenceBase.cs
- OuterProxyWrapper.cs
- BindingExpression.cs
- PaperSize.cs
- XmlWellformedWriterHelpers.cs
- HostedImpersonationContext.cs
- BamlStream.cs
- ListViewDeleteEventArgs.cs
- ParenthesizePropertyNameAttribute.cs
- Route.cs
- LinkLabel.cs
- CodeExporter.cs
- PageBreakRecord.cs
- SqlClientWrapperSmiStreamChars.cs
- HashCodeCombiner.cs
- Sentence.cs
- XmlSchemaIdentityConstraint.cs
- TransformerInfoCollection.cs
- wgx_exports.cs
- BindingList.cs
- Track.cs
- Adorner.cs
- CheckPair.cs
- DbExpressionBuilder.cs
- StatusBarItemAutomationPeer.cs
- PolicyException.cs
- mansign.cs
- SqlResolver.cs
- RegexGroupCollection.cs
- SpnegoTokenProvider.cs
- DataPointer.cs
- XamlPoint3DCollectionSerializer.cs
- Int32CollectionConverter.cs
- JpegBitmapEncoder.cs
- ExtensionQuery.cs
- Conditional.cs
- AutomationElementCollection.cs
- MailSettingsSection.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- SchemaImporterExtensionElement.cs
- CaseStatementProjectedSlot.cs
- IIS7WorkerRequest.cs
- UnsafeNativeMethods.cs
- Membership.cs
- MexNamedPipeBindingElement.cs
- CommandHelpers.cs
- TextBox.cs
- DCSafeHandle.cs
- UnsafePeerToPeerMethods.cs
- DeclarationUpdate.cs
- TagNameToTypeMapper.cs
- ReservationCollection.cs
- URLString.cs
- CustomTrackingQuery.cs
- ModelFactory.cs
- BitmapEffectInput.cs
- PrintPreviewGraphics.cs
- TextAdaptor.cs
- CommonRemoteMemoryBlock.cs
- SparseMemoryStream.cs
- TimeZoneNotFoundException.cs