Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / KeyToListMap.cs / 1305376 / KeyToListMap.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Collections.ObjectModel; namespace System.Data.Common.Utils { // This class contains an abstraction that map a key of type TKey to a // list of values (of type TValue). This is really a convenience abstraction internal class KeyToListMap: InternalBase { #region Constructors // effects: Creates an empty map with keys compared using comparer internal KeyToListMap(IEqualityComparer comparer) { Debug.Assert(null != comparer); m_map = new Dictionary >(comparer); } #endregion #region Fields // Just a regular dictionary private Dictionary > m_map; #endregion #region Properties // effects: Yields all the keys in this internal IEnumerable Keys { get { return m_map.Keys; } } // effects: Returns all the values for all keys with all the values // of a particular key adjacent to each other internal IEnumerable AllValues { get { foreach (TKey key in Keys) { foreach (TValue value in ListForKey(key)) { yield return value; } } } } // effects: Returns all the Dictionary Entries in this Map. internal IEnumerable >> KeyValuePairs { get { return m_map; } } #endregion #region Methods internal bool ContainsKey(TKey key) { return m_map.ContainsKey(key); } // effects: Adds to this. If the entry already exists, another one is added internal void Add(TKey key, TValue value) { // If entry for key already exists, add value to the list, else // create a new list and add the value to it List valueList; if (!m_map.TryGetValue(key, out valueList)) { valueList = new List (); m_map[key] = valueList; } valueList.Add(value); } // effects: Adds for each value in values to this. If the entry already exists, another one is added internal void AddRange(TKey key, IEnumerable values) { foreach (TValue value in values) { Add(key, value); } } // effects: Removes all entries corresponding to key // Returns true iff the key was removed internal bool RemoveKey(TKey key) { return m_map.Remove(key); } // requires: key exist in this // effects: Returns the values associated with key internal System.Collections.ObjectModel.ReadOnlyCollection ListForKey(TKey key) { Debug.Assert(m_map.ContainsKey(key), "key not registered in map"); return new System.Collections.ObjectModel.ReadOnlyCollection (m_map[key]); } // effects: Returns true if the key exists and false if not. // In case the Key exists, the out parameter is assigned the List for that key, // otherwise it is assigned a null value internal bool TryGetListForKey(TKey key, out System.Collections.ObjectModel.ReadOnlyCollection valueCollection) { List list; valueCollection = null; if (m_map.TryGetValue(key, out list)) { valueCollection = new System.Collections.ObjectModel.ReadOnlyCollection (list); return true; } return false; } // Returns all values for the given key. If no values have been added for the key, // yields no values. internal IEnumerable EnumerateValues(TKey key) { List values; if (m_map.TryGetValue(key, out values)) { foreach (TValue value in values) { yield return value; } } } internal override void ToCompactString(StringBuilder builder) { foreach (TKey key in Keys) { // Calling key's ToString here StringUtil.FormatStringBuilder(builder, "{0}", key); builder.Append(": "); IEnumerable values = ListForKey(key); StringUtil.ToSeparatedString(builder, values, ",", "null"); builder.Append("; "); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Collections.ObjectModel; namespace System.Data.Common.Utils { // This class contains an abstraction that map a key of type TKey to a // list of values (of type TValue). This is really a convenience abstraction internal class KeyToListMap: InternalBase { #region Constructors // effects: Creates an empty map with keys compared using comparer internal KeyToListMap(IEqualityComparer comparer) { Debug.Assert(null != comparer); m_map = new Dictionary >(comparer); } #endregion #region Fields // Just a regular dictionary private Dictionary > m_map; #endregion #region Properties // effects: Yields all the keys in this internal IEnumerable Keys { get { return m_map.Keys; } } // effects: Returns all the values for all keys with all the values // of a particular key adjacent to each other internal IEnumerable AllValues { get { foreach (TKey key in Keys) { foreach (TValue value in ListForKey(key)) { yield return value; } } } } // effects: Returns all the Dictionary Entries in this Map. internal IEnumerable >> KeyValuePairs { get { return m_map; } } #endregion #region Methods internal bool ContainsKey(TKey key) { return m_map.ContainsKey(key); } // effects: Adds to this. If the entry already exists, another one is added internal void Add(TKey key, TValue value) { // If entry for key already exists, add value to the list, else // create a new list and add the value to it List valueList; if (!m_map.TryGetValue(key, out valueList)) { valueList = new List (); m_map[key] = valueList; } valueList.Add(value); } // effects: Adds for each value in values to this. If the entry already exists, another one is added internal void AddRange(TKey key, IEnumerable values) { foreach (TValue value in values) { Add(key, value); } } // effects: Removes all entries corresponding to key // Returns true iff the key was removed internal bool RemoveKey(TKey key) { return m_map.Remove(key); } // requires: key exist in this // effects: Returns the values associated with key internal System.Collections.ObjectModel.ReadOnlyCollection ListForKey(TKey key) { Debug.Assert(m_map.ContainsKey(key), "key not registered in map"); return new System.Collections.ObjectModel.ReadOnlyCollection (m_map[key]); } // effects: Returns true if the key exists and false if not. // In case the Key exists, the out parameter is assigned the List for that key, // otherwise it is assigned a null value internal bool TryGetListForKey(TKey key, out System.Collections.ObjectModel.ReadOnlyCollection valueCollection) { List list; valueCollection = null; if (m_map.TryGetValue(key, out list)) { valueCollection = new System.Collections.ObjectModel.ReadOnlyCollection (list); return true; } return false; } // Returns all values for the given key. If no values have been added for the key, // yields no values. internal IEnumerable EnumerateValues(TKey key) { List values; if (m_map.TryGetValue(key, out values)) { foreach (TValue value in values) { yield return value; } } } internal override void ToCompactString(StringBuilder builder) { foreach (TKey key in Keys) { // Calling key's ToString here StringUtil.FormatStringBuilder(builder, "{0}", key); builder.Append(": "); IEnumerable values = ListForKey(key); StringUtil.ToSeparatedString(builder, values, ",", "null"); builder.Append("; "); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
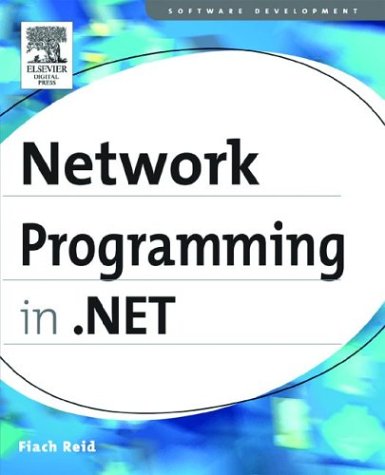
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MissingSatelliteAssemblyException.cs
- QueryResults.cs
- InputManager.cs
- XmlLanguage.cs
- Label.cs
- SortDescriptionCollection.cs
- CodeRemoveEventStatement.cs
- OpenTypeLayout.cs
- ArraySegment.cs
- ConsoleTraceListener.cs
- MailMessageEventArgs.cs
- EventQueueState.cs
- ScrollChrome.cs
- EntityProviderServices.cs
- RequestCachePolicyConverter.cs
- embossbitmapeffect.cs
- DataGridPageChangedEventArgs.cs
- IgnoreFileBuildProvider.cs
- IgnoreDataMemberAttribute.cs
- TypeConverterBase.cs
- Point3DCollectionConverter.cs
- DesignerTransaction.cs
- EventMap.cs
- Int32CollectionConverter.cs
- OptimizedTemplateContent.cs
- UrlMappingsSection.cs
- ObjectTag.cs
- WebControl.cs
- ManualResetEvent.cs
- ManipulationVelocities.cs
- DataError.cs
- ProcessHost.cs
- SiteMapNodeCollection.cs
- PropertiesTab.cs
- StrokeSerializer.cs
- HandleCollector.cs
- CreateDataSourceDialog.cs
- TypeUtil.cs
- LineBreak.cs
- EnumMember.cs
- EventHandlerList.cs
- SqlDuplicator.cs
- PerspectiveCamera.cs
- BevelBitmapEffect.cs
- URL.cs
- UIElementParaClient.cs
- XamlRtfConverter.cs
- HashMembershipCondition.cs
- QilExpression.cs
- TextElement.cs
- SnapLine.cs
- SessionSwitchEventArgs.cs
- MembershipAdapter.cs
- RadioButtonAutomationPeer.cs
- AppDomainManager.cs
- GridViewRowPresenter.cs
- DataGridViewTopRowAccessibleObject.cs
- Point3DIndependentAnimationStorage.cs
- SelectorAutomationPeer.cs
- FormViewDesigner.cs
- StdValidatorsAndConverters.cs
- ErrorInfoXmlDocument.cs
- DataTable.cs
- DataServiceExpressionVisitor.cs
- SBCSCodePageEncoding.cs
- SchemaTypeEmitter.cs
- TableLayoutRowStyleCollection.cs
- COM2ComponentEditor.cs
- Dispatcher.cs
- MarkupExtensionParser.cs
- DiscoveryDocument.cs
- SRGSCompiler.cs
- BamlResourceSerializer.cs
- BitVector32.cs
- ContractUtils.cs
- HttpCacheVaryByContentEncodings.cs
- RequiredFieldValidator.cs
- GuidelineCollection.cs
- Utils.cs
- OpCopier.cs
- Stylus.cs
- FreezableDefaultValueFactory.cs
- SystemWebExtensionsSectionGroup.cs
- ServiceAuthorizationElement.cs
- SafeProcessHandle.cs
- OperationResponse.cs
- Rect3DConverter.cs
- PartialList.cs
- XamlRtfConverter.cs
- MenuScrollingVisibilityConverter.cs
- Emitter.cs
- RSAPKCS1SignatureDeformatter.cs
- ProxyHwnd.cs
- LambdaCompiler.Address.cs
- CodeMemberMethod.cs
- Misc.cs
- ArgIterator.cs
- DataGridViewCheckBoxCell.cs
- TextRunProperties.cs
- UdpDuplexChannel.cs