Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / EventHandlerList.cs / 1 / EventHandlerList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public sealed class EventHandlerList : IDisposable { ListEntry head; Component parent; ///Provides a simple list of delegates. This class cannot be inherited. ////// Creates a new event handler list. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public EventHandlerList() { } ////// Creates a new event handler list. The parent component is used to check the component's /// CanRaiseEvents property. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] internal EventHandlerList(Component parent) { this.parent = parent; } ////// public Delegate this[object key] { [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] get { ListEntry e = null; if (parent == null || parent.CanRaiseEventsInternal) { e = Find(key); } if (e != null) { return e.handler; } else { return null; } } set { ListEntry e = Find(key); if (e != null) { e.handler = value; } else { head = new ListEntry(key, value, head); } } } ///Gets or sets the delegate for the specified key. ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public void AddHandler(object key, Delegate value) { ListEntry e = Find(key); if (e != null) { e.handler = Delegate.Combine(e.handler, value); } else { head = new ListEntry(key, value, head); } } ///[To be supplied.] ///allows you to add a list of events to this list public void AddHandlers(EventHandlerList listToAddFrom) { ListEntry currentListEntry = listToAddFrom.head; while (currentListEntry != null) { AddHandler(currentListEntry.key, currentListEntry.handler); currentListEntry = currentListEntry.next; } } ////// public void Dispose() { head = null; } private ListEntry Find(object key) { ListEntry found = head; while (found != null) { if (found.key == key) { break; } found = found.next; } return found; } ///[To be supplied.] ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public void RemoveHandler(object key, Delegate value) { ListEntry e = Find(key); if (e != null) { e.handler = Delegate.Remove(e.handler, value); } // else... no error for removal of non-existant delegate // } private sealed class ListEntry { internal ListEntry next; internal object key; internal Delegate handler; public ListEntry(object key, Delegate handler, ListEntry next) { this.next = next; this.key = key; this.handler = handler; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public sealed class EventHandlerList : IDisposable { ListEntry head; Component parent; ///Provides a simple list of delegates. This class cannot be inherited. ////// Creates a new event handler list. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public EventHandlerList() { } ////// Creates a new event handler list. The parent component is used to check the component's /// CanRaiseEvents property. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] internal EventHandlerList(Component parent) { this.parent = parent; } ////// public Delegate this[object key] { [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] get { ListEntry e = null; if (parent == null || parent.CanRaiseEventsInternal) { e = Find(key); } if (e != null) { return e.handler; } else { return null; } } set { ListEntry e = Find(key); if (e != null) { e.handler = value; } else { head = new ListEntry(key, value, head); } } } ///Gets or sets the delegate for the specified key. ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public void AddHandler(object key, Delegate value) { ListEntry e = Find(key); if (e != null) { e.handler = Delegate.Combine(e.handler, value); } else { head = new ListEntry(key, value, head); } } ///[To be supplied.] ///allows you to add a list of events to this list public void AddHandlers(EventHandlerList listToAddFrom) { ListEntry currentListEntry = listToAddFrom.head; while (currentListEntry != null) { AddHandler(currentListEntry.key, currentListEntry.handler); currentListEntry = currentListEntry.next; } } ////// public void Dispose() { head = null; } private ListEntry Find(object key) { ListEntry found = head; while (found != null) { if (found.key == key) { break; } found = found.next; } return found; } ///[To be supplied.] ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public void RemoveHandler(object key, Delegate value) { ListEntry e = Find(key); if (e != null) { e.handler = Delegate.Remove(e.handler, value); } // else... no error for removal of non-existant delegate // } private sealed class ListEntry { internal ListEntry next; internal object key; internal Delegate handler; public ListEntry(object key, Delegate handler, ListEntry next) { this.next = next; this.key = key; this.handler = handler; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
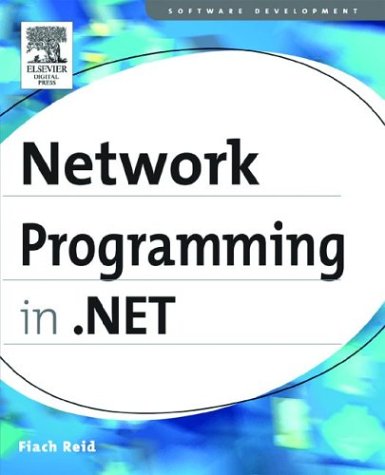
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartTracker.cs
- FormViewPagerRow.cs
- DocumentApplicationJournalEntry.cs
- XmlSchemaAll.cs
- ListViewUpdateEventArgs.cs
- WmlPhoneCallAdapter.cs
- RuntimeEnvironment.cs
- OptimisticConcurrencyException.cs
- OwnerDrawPropertyBag.cs
- AccessorTable.cs
- EpmCustomContentDeSerializer.cs
- ListBindableAttribute.cs
- NonSerializedAttribute.cs
- GeometryConverter.cs
- AuthorizationRule.cs
- Debug.cs
- DesignerDataView.cs
- TypeToken.cs
- EventRecordWrittenEventArgs.cs
- Timeline.cs
- CornerRadiusConverter.cs
- TaiwanLunisolarCalendar.cs
- X509IssuerSerialKeyIdentifierClause.cs
- EllipseGeometry.cs
- FilteredDataSetHelper.cs
- WebPartManager.cs
- TypeGeneratedEventArgs.cs
- serverconfig.cs
- UIElementHelper.cs
- Matrix3DValueSerializer.cs
- HandleCollector.cs
- WebZoneDesigner.cs
- Timeline.cs
- ProjectionRewriter.cs
- CompilationSection.cs
- DataGridRowAutomationPeer.cs
- ResourceReferenceExpressionConverter.cs
- FixedSOMSemanticBox.cs
- DateTimeConverter.cs
- TextBox.cs
- ApplicationFileParser.cs
- EntityDataSourceSelectingEventArgs.cs
- AsyncStreamReader.cs
- FormConverter.cs
- ErrorsHelper.cs
- EmissiveMaterial.cs
- IERequestCache.cs
- PermissionSetEnumerator.cs
- ErrorFormatterPage.cs
- httpapplicationstate.cs
- ServiceModelExtensionCollectionElement.cs
- DBPropSet.cs
- _PooledStream.cs
- CompilationUtil.cs
- MasterPageBuildProvider.cs
- SoapIncludeAttribute.cs
- CssClassPropertyAttribute.cs
- SchemaImporter.cs
- HandlerFactoryCache.cs
- TypeExtensionConverter.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- AppDomainProtocolHandler.cs
- IndividualDeviceConfig.cs
- ISFClipboardData.cs
- StringValidator.cs
- Control.cs
- SqlDuplicator.cs
- TemplateAction.cs
- SectionUpdates.cs
- WCFBuildProvider.cs
- SessionEndingCancelEventArgs.cs
- PassportAuthentication.cs
- XPathChildIterator.cs
- XmlSignificantWhitespace.cs
- DocumentOrderComparer.cs
- Pkcs7Signer.cs
- XomlCompiler.cs
- IPHostEntry.cs
- ImpersonateTokenRef.cs
- TableCell.cs
- FixedSOMGroup.cs
- RegularExpressionValidator.cs
- GridViewSortEventArgs.cs
- ReadOnlyPropertyMetadata.cs
- WebPartZoneCollection.cs
- GeneratedView.cs
- BuildDependencySet.cs
- ProfileGroupSettings.cs
- Point3DKeyFrameCollection.cs
- XmlUtil.cs
- WpfKnownMemberInvoker.cs
- ModelUIElement3D.cs
- DefaultMemberAttribute.cs
- XmlBoundElement.cs
- TypeUtils.cs
- PrintDocument.cs
- DetailsViewRowCollection.cs
- DataGridColumnCollection.cs
- DataControlFieldHeaderCell.cs
- WebPartConnectionsCancelVerb.cs