Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / SRGSCompiler.cs / 1 / SRGSCompiler.cs
//// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 6/1/2004 [....] Converted from the managed code // 10/1/2004 [....] Added Custom grammar support //---------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Reflection; using System.Runtime.InteropServices; using System.Speech.Internal.SrgsParser; using System.Speech.Recognition; using System.Speech.Recognition.SrgsGrammar; using System.Text; using System.Xml; namespace System.Speech.Internal.SrgsCompiler { internal static class SrgsCompiler { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods ////// Loads the SRGS XML grammar and produces the binary grammar format. /// /// Source SRGS XML streams /// filename to compile to /// stream to compile to /// Compile for CFG or DLL /// in xmlReader.Count == 1, name of the originel file /// List of referenced assemblies /// Strong name internal static void CompileStream (XmlReader [] xmlReaders, string filename, Stream stream, bool fOutputCfg, Uri originalUri, string [] referencedAssemblies, string keyFile) { // raft of files to compiler is only available for class library System.Diagnostics.Debug.Assert (!fOutputCfg || xmlReaders.Length == 1); int cReaders = xmlReaders.Length; #if !NO_STG ListcfgResources = new List (); CustomGrammar cgCombined = new CustomGrammar (); #else object cfgResources = null; #endif for (int iReader = 0; iReader < cReaders; iReader++) { // Set the current directory to the location where is the grammar string srgsPath = null; Uri uri = originalUri; if (uri == null) { if (xmlReaders [iReader].BaseURI != null && xmlReaders [iReader].BaseURI.Length > 0) { uri = new Uri (xmlReaders [iReader].BaseURI); } } if (uri != null && (!uri.IsAbsoluteUri || uri.IsFile)) { srgsPath = Path.GetDirectoryName (uri.IsAbsoluteUri ? uri.AbsolutePath : uri.OriginalString); } CultureInfo culture; StringBuilder innerCode = new StringBuilder (); ISrgsParser srgsParser = (ISrgsParser) new XmlParser (xmlReaders [iReader], uri); object cg = CompileStream (iReader + 1, srgsParser, srgsPath, filename, stream, fOutputCfg, innerCode, cfgResources, out culture, referencedAssemblies, keyFile); #if !NO_STG if (!fOutputCfg) { cgCombined.Combine ((CustomGrammar) cg, innerCode.ToString ()); } #endif } #if !NO_STG // Create the DLL if this needs to be done if (!fOutputCfg) { cgCombined.CreateAssembly (filename, cfgResources); } #endif } /// /// Produces the binary grammar format. /// /// Source SRGS XML streams /// filename to compile to /// stream to compile to /// Compile for CFG or DLL /// List of referenced assemblies /// Strong name internal static void CompileStream (SrgsDocument srgsGrammar, string filename, Stream stream, bool fOutputCfg, string [] referencedAssemblies, string keyFile) { ISrgsParser srgsParser = (ISrgsParser) new SrgsDocumentParser (srgsGrammar.Grammar); #if !NO_STG ListcfgResources = new List (); #else object cfgResources = null; #endif StringBuilder innerCode = new StringBuilder (); CultureInfo culture; // Validate the grammar before compiling it. Set the tag-format and sapi flags too. srgsGrammar.Grammar.Validate (); object cg = CompileStream (1, srgsParser, null, filename, stream, fOutputCfg, innerCode, cfgResources, out culture, referencedAssemblies, keyFile); #if !NO_STG // Create the DLL if this needs to be done if (!fOutputCfg) { CustomGrammar cgCombined = new CustomGrammar (); cgCombined.Combine ((CustomGrammar) cg, innerCode.ToString ()); cgCombined.CreateAssembly (filename, cfgResources); } #endif } #endregion static private object CompileStream (int iCfg, ISrgsParser srgsParser, string srgsPath, string filename, Stream stream, bool fOutputCfg, StringBuilder innerCode, object cfgResources, out CultureInfo culture, string [] referencedAssemblies, string keyFile) { Backend backend = new Backend (); #if !NO_STG CustomGrammar cg = new CustomGrammar (); SrgsElementCompilerFactory elementFactory = new SrgsElementCompilerFactory (backend, cg); #else SrgsElementCompilerFactory elementFactory = new SrgsElementCompilerFactory (backend); #endif srgsParser.ElementFactory = elementFactory; srgsParser.Parse (); // Optimize in-memory graph representation of the grammar. backend.Optimize (); // culture = backend.LangId == 0x540A ? new CultureInfo ("es-us") : new CultureInfo (backend.LangId); #if !NO_STG // A grammar may contains references to other files in codebehind. // Set the current directory to the location where is the grammar if (cg._codebehind.Count > 0 && !string.IsNullOrEmpty (srgsPath)) { for (int i = 0; i < cg._codebehind.Count; i++) { if (!File.Exists (cg._codebehind [i])) { cg._codebehind [i] = srgsPath + "\\" + cg._codebehind [i]; } } } // Add the referenced assemblies if (referencedAssemblies != null) { foreach (string assembly in referencedAssemblies) { cg._assemblyReferences.Add (assembly); } } // Assign the key file cg._keyFile = keyFile; // Assign the Scripts to the backend backend.ScriptRefs = cg._scriptRefs; // If the target is a dll, then create first the CFG and stuff it as an embedded resource if (!fOutputCfg) { // Creates a DLL with the CFG is a resource // Create the CFG CustomGrammar.CfgResource resource = new CustomGrammar.CfgResource (); resource.data = BuildCfg (backend).ToArray (); resource.name = iCfg.ToString (CultureInfo.InvariantCulture) + ".CFG"; ((List )cfgResources).Add (resource); // Sanity check, create the DLL and checks that all the methods can be called // Combine all the scripts innerCode.Append (cg.CreateAssembly (iCfg, filename, culture)); } else { //if semantic processing for a rule is defined, a script needs to be defined if (cg._scriptRefs.Count > 0 && !cg.HasScript) { XmlParser.ThrowSrgsException (SRID.NoScriptsForRules); } // Creates a CFG with IL embedded CreateAssembly (backend, cg); // Save binary grammar to dest if (!string.IsNullOrEmpty (filename)) { // Create a stream if a filename was given stream = new FileStream (filename, FileMode.Create, FileAccess.Write); } #endif try { using (StreamMarshaler streamHelper = new StreamMarshaler (stream)) { backend.Commit (streamHelper); } } finally { if (!string.IsNullOrEmpty (filename)) { stream.Close (); } } #if !NO_STG } return cg; #else return null; #endif } #if !SPEECHSERVER /// /// Build the state machine for a grammar and returns it in a Memory Stream. /// /// ///static private MemoryStream BuildCfg (Backend backend) { // Creates a DLL MemoryStream cfgStream = new MemoryStream (); // Save binary grammar to dest using (StreamMarshaler streamHelper = new StreamMarshaler (cfgStream)) { #if !NO_STG // no IL backend.IL = null; backend.PDB = null; #endif // Generate the binary blob backend.Commit (streamHelper); } return cfgStream; } #endif #if !NO_STG /// /// Generate the assembly code for a back. The scripts are defined in custom /// grammars. /// /// /// static void CreateAssembly (Backend backend, CustomGrammar cg) { if (cg.HasScript) { // Create the IL and checks that the signature for all the methods for scriptRefs are ok // Pass a reference to the IL and scriptRefs to the backend. byte [] il; byte [] symbols; cg.CreateAssembly (out il, out symbols); backend.IL = il; backend.PDB = symbols; } } #endif } internal enum RuleScope { PublicRule, PrivateRule } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
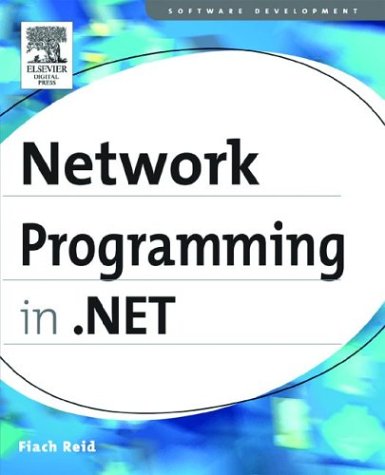
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrugalList.cs
- BreakRecordTable.cs
- SchemaTableOptionalColumn.cs
- UIElement.cs
- SimpleTextLine.cs
- TimeManager.cs
- TableRow.cs
- MultipleViewProviderWrapper.cs
- SQLUtility.cs
- CountdownEvent.cs
- CustomExpressionEventArgs.cs
- AllMembershipCondition.cs
- FixUp.cs
- EnvironmentPermission.cs
- TextTreeDeleteContentUndoUnit.cs
- MembershipSection.cs
- UrlPath.cs
- MemoryFailPoint.cs
- ActivityTypeDesigner.xaml.cs
- CodeNamespaceImport.cs
- Number.cs
- XmlQueryStaticData.cs
- TableParaClient.cs
- PermissionToken.cs
- SecuritySessionFilter.cs
- InfiniteTimeSpanConverter.cs
- EdmEntityTypeAttribute.cs
- SiteMembershipCondition.cs
- AuthorizationRuleCollection.cs
- MetadataCache.cs
- Vector3DAnimationBase.cs
- OdbcRowUpdatingEvent.cs
- RuntimeConfig.cs
- ReadWriteObjectLock.cs
- TextEditorParagraphs.cs
- MobileSysDescriptionAttribute.cs
- ScriptHandlerFactory.cs
- StdRegProviderWrapper.cs
- ApplicationSecurityManager.cs
- NumericExpr.cs
- EndEvent.cs
- XpsPackagingPolicy.cs
- SoapSchemaImporter.cs
- TypeContext.cs
- DataSourceCollectionBase.cs
- XmlMemberMapping.cs
- HighlightComponent.cs
- SerializationFieldInfo.cs
- PageCatalogPart.cs
- IEnumerable.cs
- ExchangeUtilities.cs
- XhtmlBasicValidationSummaryAdapter.cs
- CurrentTimeZone.cs
- DecimalFormatter.cs
- AuthorizationRule.cs
- SafeEventLogWriteHandle.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- TextEffectResolver.cs
- AutoGeneratedField.cs
- Renderer.cs
- RegexMatchCollection.cs
- QuadraticBezierSegment.cs
- WebPartDescription.cs
- ControlValuePropertyAttribute.cs
- AvTraceDetails.cs
- UxThemeWrapper.cs
- BinaryMethodMessage.cs
- ContainsRowNumberChecker.cs
- BindingCollectionElement.cs
- PointAnimationBase.cs
- ObjectHandle.cs
- BitmapImage.cs
- PatternMatcher.cs
- HebrewCalendar.cs
- PieceDirectory.cs
- XmlNotation.cs
- TriState.cs
- AppliedDeviceFiltersDialog.cs
- SafeEventLogReadHandle.cs
- HwndSourceKeyboardInputSite.cs
- oledbconnectionstring.cs
- PropertyGeneratedEventArgs.cs
- GPRECT.cs
- InvokePatternIdentifiers.cs
- MsmqNonTransactedPoisonHandler.cs
- RadioButtonFlatAdapter.cs
- DbExpressionVisitor.cs
- MetadataUtil.cs
- DiscoveryRequestHandler.cs
- ByteArrayHelperWithString.cs
- IImplicitResourceProvider.cs
- FreezableDefaultValueFactory.cs
- PropertyChangeTracker.cs
- WsatAdminException.cs
- GregorianCalendar.cs
- XmlSchemaProviderAttribute.cs
- ConnectionsZone.cs
- CryptoApi.cs
- FeatureManager.cs
- DataBindingsDialog.cs