Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / DataSourceCollectionBase.cs / 1 / DataSourceCollectionBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design{ using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; ////// internal abstract class DataSourceCollectionBase : CollectionBase, INamedObjectCollection, IObjectWithParent { private DataSourceComponent collectionHost; internal DataSourceCollectionBase(DataSourceComponent collectionHost) { this.collectionHost = collectionHost; } internal virtual DataSourceComponent CollectionHost { get { return this.collectionHost; } set { this.collectionHost = value; } } protected virtual Type ItemType { get { return typeof(IDataSourceNamedObject); } } protected abstract INameService NameService { get; } [Browsable(false)] object IObjectWithParent.Parent { get { return this.collectionHost; } } protected virtual string CreateUniqueName(IDataSourceNamedObject value) { string suggestedName = StringUtil.NotEmpty(value.Name) ? value.Name : value.PublicTypeName; return NameService.CreateUniqueName(this, suggestedName, 1); } ////// This function will check to see namedObject have a name conflict with the names /// used in the collection and fix the name for namedObject if there is a conflict /// /// internal protected virtual void EnsureUniqueName(IDataSourceNamedObject namedObject) { if (namedObject.Name == null || namedObject.Name.Length == 0 || this.FindObject(namedObject.Name) != null) { namedObject.Name = CreateUniqueName(namedObject); } } internal virtual protected IDataSourceNamedObject FindObject(string name) { IEnumerator e = this.InnerList.GetEnumerator(); while( e.MoveNext() ) { IDataSourceNamedObject existing = (IDataSourceNamedObject) e.Current; if (StringUtil.EqualValue(existing.Name, name)) { return existing; } } return null; } public void InsertBefore(object value, object refObject) { int index = List.IndexOf(refObject); if (index >= 0) { List.Insert(index, value); } else { List.Add(value); } } protected override void OnValidate( object value ) { base.OnValidate( value ); ValidateType( value ); } public void Remove(string name) { INamedObject obj = NamedObjectUtil.Find( this, name ); if( obj != null ) { this.List.Remove( obj ); } } ////// This function only check the name to be valid, typically used by Insert function, which already ensure the name is unique /// /// internal protected virtual void ValidateName(IDataSourceNamedObject obj) { this.NameService.ValidateName(obj.Name); } ////// This function checks the name to be unique and valid, typically used by rename /// /// /// internal protected virtual void ValidateUniqueName(IDataSourceNamedObject obj, string proposedName) { this.NameService.ValidateUniqueName(this, obj, proposedName); } protected void ValidateType( object value ) { if (!ItemType.IsInstanceOfType(value)) { throw new InternalException(VSDExceptions.DataSource.INVALID_COLLECTIONTYPE_MSG, VSDExceptions.DataSource.INVALID_COLLECTIONTYPE_CODE, true); } } public INameService GetNameService() { return NameService; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
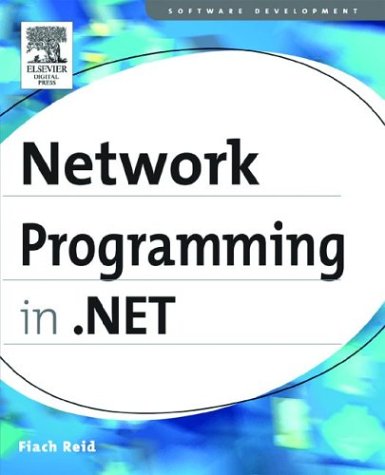
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlphabeticalEnumConverter.cs
- DataServiceRequest.cs
- ScrollEventArgs.cs
- ApplicationDirectoryMembershipCondition.cs
- ChannelSinkStacks.cs
- ProfileServiceManager.cs
- PointConverter.cs
- DummyDataSource.cs
- XpsSerializationException.cs
- FlowDocumentReader.cs
- DecimalAnimationBase.cs
- GridViewRow.cs
- BackStopAuthenticationModule.cs
- EventInfo.cs
- PartDesigner.cs
- TypeUsage.cs
- ValidationErrorEventArgs.cs
- HttpContext.cs
- NumberFormatter.cs
- DesignBindingPropertyDescriptor.cs
- BufferedWebEventProvider.cs
- HashHelper.cs
- UnsafeNetInfoNativeMethods.cs
- ClusterSafeNativeMethods.cs
- PageAdapter.cs
- CapabilitiesState.cs
- ParseElementCollection.cs
- securitycriticaldataClass.cs
- Matrix3DConverter.cs
- WMICapabilities.cs
- XmlSerializationGeneratedCode.cs
- StyleCollection.cs
- CustomAssemblyResolver.cs
- AttributedMetaModel.cs
- TypeExtensionSerializer.cs
- Console.cs
- RealProxy.cs
- XmlTextEncoder.cs
- DataFieldEditor.cs
- RegexGroup.cs
- IncomingWebResponseContext.cs
- ServiceModelConfiguration.cs
- ValidatorCompatibilityHelper.cs
- Cursor.cs
- TriggerCollection.cs
- DiscoveryRequestHandler.cs
- VerificationException.cs
- CompatibleComparer.cs
- DataGridViewCellStyleConverter.cs
- Unit.cs
- ControlParameter.cs
- InvokePattern.cs
- ComponentRenameEvent.cs
- SqlFactory.cs
- ApplyHostConfigurationBehavior.cs
- ValidatorUtils.cs
- MergablePropertyAttribute.cs
- PersonalizationEntry.cs
- EvidenceBase.cs
- TagPrefixCollection.cs
- ParseNumbers.cs
- DbConnectionInternal.cs
- StringResourceManager.cs
- TextParaClient.cs
- TcpStreams.cs
- XmlSchemaAll.cs
- WmpBitmapEncoder.cs
- ChangesetResponse.cs
- PersonalizableTypeEntry.cs
- RootBrowserWindowProxy.cs
- StructuredTypeEmitter.cs
- JoinTreeNode.cs
- Button.cs
- WasHostedComPlusFactory.cs
- PartDesigner.cs
- SoapExtension.cs
- DataGridAutoFormat.cs
- SchemaImporterExtension.cs
- LineServicesRun.cs
- Control.cs
- SettingsPropertyValue.cs
- TreeNodeBinding.cs
- ConfigurationSectionGroup.cs
- CollectionViewProxy.cs
- QilIterator.cs
- ActivityExecutor.cs
- PrintDialogException.cs
- MenuItemCollection.cs
- SignatureGenerator.cs
- Splitter.cs
- InternalTypeHelper.cs
- ArgumentException.cs
- ListContractAdapter.cs
- SettingsPropertyWrongTypeException.cs
- MultidimensionalArrayItemReference.cs
- OptimizerPatterns.cs
- DesignerRegionCollection.cs
- XmlDeclaration.cs
- SoapIncludeAttribute.cs
- XmlWrappingReader.cs