Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceRequest.cs / 4 / DataServiceRequest.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Xml; ///non-generic placeholder for generic implementation public abstract class DataServiceRequest { ///internal constructor so that only our assembly can provide an implementation internal DataServiceRequest() { } ///Element Type public abstract Type ElementType { get; } ///Gets the URI for a the query public abstract Uri RequestUri { get; } ///Gets the URI for a the query ///a string with the URI public override string ToString() { return this.RequestUri.ToString(); } ////// get an enumerable materializes the objects the response /// ///element type /// context /// reader /// elementType ///an enumerable internal static object CreateMaterializer(DataServiceContext context, XmlReader reader, Type elementType) { Debug.Assert(null == elementType, "non-null elementType with strongly typed create delegate"); return new MaterializeAtom.MaterializeAtomT (context, reader); } /// /// get an enumerable materializes the objects the response /// ///element type /// context /// contentType /// method to get http response stream ///an enumerable internal static IEnumerableMaterialize (DataServiceContext context, string contentType, Func response) { Func create = CreateMaterializer ; return (IEnumerable )context.GetMaterializer(null, contentType, response, create); } /// /// Creates a instance of strongly typed DataServiceRequest with the given element type. /// /// element type for the DataServiceRequest. /// constructor parameter. ///returns the strongly typed DataServiceRequest instance. internal static DataServiceRequest GetInstance(Type elementType, Uri requestUri) { Type genericType = typeof(DataServiceRequest<>).MakeGenericType(elementType); return (DataServiceRequest)Activator.CreateInstance(genericType, new object[] { requestUri }); } #if !ASTORIA_LIGHT // Synchronous methods not available ////// execute uri and materialize result /// ///element type /// context /// uri to execute ///enumerable of results internal IEnumerableExecute (DataServiceContext context, Uri requestUri) { Debug.Assert(null != context, "context is null"); IEnumerable result = null; QueryAsyncResult response = null; try { DataServiceRequest serviceRequest = new DataServiceRequest (requestUri); HttpWebRequest request = context.CreateRequest(requestUri, XmlConstants.HttpMethodGet, false, null); response = new QueryAsyncResult(this, "Execute", serviceRequest, request, null, null); response.Execute(null); if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } catch (InvalidOperationException ex) { QueryOperationResponse operationResponse = response.GetResponse(result, typeof(TElement)); if (null != operationResponse) { operationResponse.Error = ex; throw new DataServiceQueryException(Strings.DataServiceException_GeneralError, ex, operationResponse); } throw; } } #endif /// enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal abstract System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse); /// /// Begins an asynchronous request to an Internet resource. /// /// source of execute (DataServiceQuery or DataServiceContext /// context /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. internal IAsyncResult BeginExecute(object source, DataServiceContext context, AsyncCallback callback, object state) { Debug.Assert(null != context, "context is null"); HttpWebRequest request = context.CreateRequest(this.RequestUri, XmlConstants.HttpMethodGet, false, null); QueryAsyncResult result = new QueryAsyncResult(source, "Execute", this, request, callback, state); result.BeginExecute(null); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Xml; ///non-generic placeholder for generic implementation public abstract class DataServiceRequest { ///internal constructor so that only our assembly can provide an implementation internal DataServiceRequest() { } ///Element Type public abstract Type ElementType { get; } ///Gets the URI for a the query public abstract Uri RequestUri { get; } ///Gets the URI for a the query ///a string with the URI public override string ToString() { return this.RequestUri.ToString(); } ////// get an enumerable materializes the objects the response /// ///element type /// context /// reader /// elementType ///an enumerable internal static object CreateMaterializer(DataServiceContext context, XmlReader reader, Type elementType) { Debug.Assert(null == elementType, "non-null elementType with strongly typed create delegate"); return new MaterializeAtom.MaterializeAtomT (context, reader); } /// /// get an enumerable materializes the objects the response /// ///element type /// context /// contentType /// method to get http response stream ///an enumerable internal static IEnumerableMaterialize (DataServiceContext context, string contentType, Func response) { Func create = CreateMaterializer ; return (IEnumerable )context.GetMaterializer(null, contentType, response, create); } /// /// Creates a instance of strongly typed DataServiceRequest with the given element type. /// /// element type for the DataServiceRequest. /// constructor parameter. ///returns the strongly typed DataServiceRequest instance. internal static DataServiceRequest GetInstance(Type elementType, Uri requestUri) { Type genericType = typeof(DataServiceRequest<>).MakeGenericType(elementType); return (DataServiceRequest)Activator.CreateInstance(genericType, new object[] { requestUri }); } #if !ASTORIA_LIGHT // Synchronous methods not available ////// execute uri and materialize result /// ///element type /// context /// uri to execute ///enumerable of results internal IEnumerableExecute (DataServiceContext context, Uri requestUri) { Debug.Assert(null != context, "context is null"); IEnumerable result = null; QueryAsyncResult response = null; try { DataServiceRequest serviceRequest = new DataServiceRequest (requestUri); HttpWebRequest request = context.CreateRequest(requestUri, XmlConstants.HttpMethodGet, false, null); response = new QueryAsyncResult(this, "Execute", serviceRequest, request, null, null); response.Execute(null); if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } catch (InvalidOperationException ex) { QueryOperationResponse operationResponse = response.GetResponse(result, typeof(TElement)); if (null != operationResponse) { operationResponse.Error = ex; throw new DataServiceQueryException(Strings.DataServiceException_GeneralError, ex, operationResponse); } throw; } } #endif /// enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal abstract System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse); /// /// Begins an asynchronous request to an Internet resource. /// /// source of execute (DataServiceQuery or DataServiceContext /// context /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. internal IAsyncResult BeginExecute(object source, DataServiceContext context, AsyncCallback callback, object state) { Debug.Assert(null != context, "context is null"); HttpWebRequest request = context.CreateRequest(this.RequestUri, XmlConstants.HttpMethodGet, false, null); QueryAsyncResult result = new QueryAsyncResult(source, "Execute", this, request, callback, state); result.BeginExecute(null); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
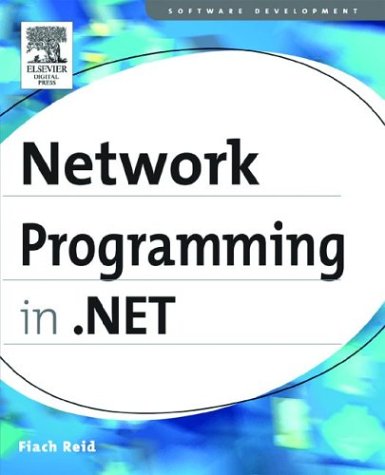
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsGraphicsCacheManager.cs
- Event.cs
- Span.cs
- ResourcesBuildProvider.cs
- RuleSet.cs
- ADConnectionHelper.cs
- XhtmlBasicImageAdapter.cs
- ObjRef.cs
- DataMemberAttribute.cs
- KeyValuePairs.cs
- Preprocessor.cs
- MethodImplAttribute.cs
- Choices.cs
- ListBox.cs
- AmbiguousMatchException.cs
- xmlsaver.cs
- MarshalByRefObject.cs
- Rfc4050KeyFormatter.cs
- UnsafeNativeMethods.cs
- SelectedDatesCollection.cs
- ObjectDataSourceSelectingEventArgs.cs
- InteropTrackingRecord.cs
- DropSource.cs
- ToolboxItemWrapper.cs
- EntityKey.cs
- TableItemStyle.cs
- EntityDataSourceChangingEventArgs.cs
- UInt64.cs
- Context.cs
- DataGridViewCellEventArgs.cs
- PasswordPropertyTextAttribute.cs
- UxThemeWrapper.cs
- ValidationEventArgs.cs
- Constants.cs
- Stylesheet.cs
- ComplexLine.cs
- RegexRunnerFactory.cs
- AdPostCacheSubstitution.cs
- UIntPtr.cs
- HMAC.cs
- Vector3dCollection.cs
- CommandValueSerializer.cs
- ToolStripSeparatorRenderEventArgs.cs
- IBuiltInEvidence.cs
- ToolStripItemImageRenderEventArgs.cs
- UnionExpr.cs
- WorkflowApplicationCompletedException.cs
- ListDesigner.cs
- StylusPointPropertyUnit.cs
- InputQueueChannelAcceptor.cs
- DesignerVerb.cs
- WindowsStreamSecurityUpgradeProvider.cs
- PieceNameHelper.cs
- PerformanceCounterPermission.cs
- TemplateInstanceAttribute.cs
- Vector3DAnimationBase.cs
- TextParagraph.cs
- FixedSOMImage.cs
- TranslateTransform3D.cs
- _HTTPDateParse.cs
- DataServiceRequestArgs.cs
- Grant.cs
- PersonalizationDictionary.cs
- SecurityUniqueId.cs
- HtmlEncodedRawTextWriter.cs
- ConnectionStringsExpressionBuilder.cs
- OracleLob.cs
- HMACSHA256.cs
- Assembly.cs
- ErrorWebPart.cs
- PathTooLongException.cs
- XmlSchemaSubstitutionGroup.cs
- PKCS1MaskGenerationMethod.cs
- KeyConverter.cs
- DataColumnMappingCollection.cs
- SizeFConverter.cs
- CqlGenerator.cs
- ItemMap.cs
- ToolStripContainer.cs
- IRCollection.cs
- DataSourceXmlTextReader.cs
- MouseButton.cs
- NotificationContext.cs
- CommandLibraryHelper.cs
- KernelTypeValidation.cs
- EventArgs.cs
- BitmapEffect.cs
- KeysConverter.cs
- WmlPanelAdapter.cs
- CommonXSendMessage.cs
- ObjectResult.cs
- WebPartConnectionsCancelVerb.cs
- MenuBase.cs
- SemanticResolver.cs
- QueryExpression.cs
- QueryableDataSourceEditData.cs
- FocusManager.cs
- InsufficientMemoryException.cs
- IsolatedStorageFilePermission.cs
- WebPartEditVerb.cs