Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / ComponentModel / SortDescriptionCollection.cs / 1305600 / SortDescriptionCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: dynamic collection of SortDescriptions // // See spec at http://avalon/connecteddata/Specs/CollectionView.mht // // History: // 03/24/2005 : [....] - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows; using MS.Utility; namespace System.ComponentModel { ////// Implementation of a dynamic data collection of SortDescriptions. /// public class SortDescriptionCollection : Collection, INotifyCollectionChanged { //----------------------------------------------------- // // Public Events // //----------------------------------------------------- #region Public Events /// /// Occurs when the collection changes, either by adding or removing an item. /// ////// see event NotifyCollectionChangedEventHandler INotifyCollectionChanged.CollectionChanged { add { CollectionChanged += value; } remove { CollectionChanged -= value; } } ////// /// Occurs when the collection changes, either by adding or removing an item. /// protected event NotifyCollectionChangedEventHandler CollectionChanged; #endregion Public Events //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { base.ClearItems(); OnCollectionChanged(NotifyCollectionChangedAction.Reset); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { SortDescription removedItem = this[index]; base.RemoveItem(index); OnCollectionChanged(NotifyCollectionChangedAction.Remove, removedItem, index); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { item.Seal(); base.InsertItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// called by base class Collection<T> when an item is set in the list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { item.Seal(); SortDescription originalItem = this[index]; base.SetItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Remove, originalItem, index); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// raise CollectionChanged event to any listeners /// private void OnCollectionChanged(NotifyCollectionChangedAction action, object item, int index) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action, item, index)); } } // raise CollectionChanged event to any listeners void OnCollectionChanged(NotifyCollectionChangedAction action) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action)); } } #endregion Protected Methods ////// Immutable, read-only SortDescriptionCollection /// class EmptySortDescriptionCollection : SortDescriptionCollection, IList { //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is set in list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { throw new NotSupportedException(); } #endregion Protected Methods #region IList Implementations // explicit implementation to override the IsReadOnly and IsFixedSize properties bool IList.IsFixedSize { get { return true; } } bool IList.IsReadOnly { get { return true; } } #endregion IList Implementations } ////// returns an empty and non-modifiable SortDescriptionCollection /// public static readonly SortDescriptionCollection Empty = new EmptySortDescriptionCollection(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: dynamic collection of SortDescriptions // // See spec at http://avalon/connecteddata/Specs/CollectionView.mht // // History: // 03/24/2005 : [....] - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows; using MS.Utility; namespace System.ComponentModel { ////// Implementation of a dynamic data collection of SortDescriptions. /// public class SortDescriptionCollection : Collection, INotifyCollectionChanged { //----------------------------------------------------- // // Public Events // //----------------------------------------------------- #region Public Events /// /// Occurs when the collection changes, either by adding or removing an item. /// ////// see event NotifyCollectionChangedEventHandler INotifyCollectionChanged.CollectionChanged { add { CollectionChanged += value; } remove { CollectionChanged -= value; } } ////// /// Occurs when the collection changes, either by adding or removing an item. /// protected event NotifyCollectionChangedEventHandler CollectionChanged; #endregion Public Events //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { base.ClearItems(); OnCollectionChanged(NotifyCollectionChangedAction.Reset); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { SortDescription removedItem = this[index]; base.RemoveItem(index); OnCollectionChanged(NotifyCollectionChangedAction.Remove, removedItem, index); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { item.Seal(); base.InsertItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// called by base class Collection<T> when an item is set in the list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { item.Seal(); SortDescription originalItem = this[index]; base.SetItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Remove, originalItem, index); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// raise CollectionChanged event to any listeners /// private void OnCollectionChanged(NotifyCollectionChangedAction action, object item, int index) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action, item, index)); } } // raise CollectionChanged event to any listeners void OnCollectionChanged(NotifyCollectionChangedAction action) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action)); } } #endregion Protected Methods ////// Immutable, read-only SortDescriptionCollection /// class EmptySortDescriptionCollection : SortDescriptionCollection, IList { //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is set in list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { throw new NotSupportedException(); } #endregion Protected Methods #region IList Implementations // explicit implementation to override the IsReadOnly and IsFixedSize properties bool IList.IsFixedSize { get { return true; } } bool IList.IsReadOnly { get { return true; } } #endregion IList Implementations } ////// returns an empty and non-modifiable SortDescriptionCollection /// public static readonly SortDescriptionCollection Empty = new EmptySortDescriptionCollection(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
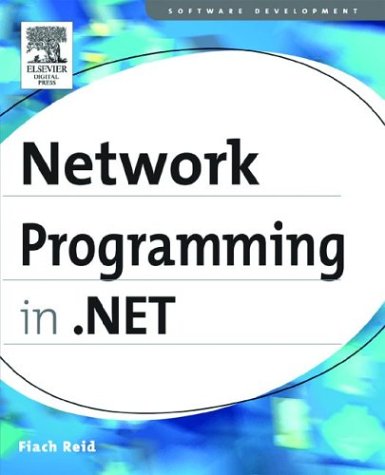
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicenseManager.cs
- Operand.cs
- TypedTableBase.cs
- GiveFeedbackEvent.cs
- InputMethodStateChangeEventArgs.cs
- FirstMatchCodeGroup.cs
- NavigationService.cs
- MappingMetadataHelper.cs
- EdmToObjectNamespaceMap.cs
- FrameworkElementAutomationPeer.cs
- BindingElementCollection.cs
- CodeRegionDirective.cs
- ReceiveActivityDesignerTheme.cs
- NavigationPropertySingletonExpression.cs
- CallbackHandler.cs
- Validator.cs
- CompilerScopeManager.cs
- ColorContextHelper.cs
- ObjRef.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- CodePageEncoding.cs
- LineVisual.cs
- ButtonAutomationPeer.cs
- RegisteredHiddenField.cs
- MSAAWinEventWrap.cs
- BitHelper.cs
- WebPartsPersonalizationAuthorization.cs
- CopyAction.cs
- CaretElement.cs
- TypeHelper.cs
- HtmlGenericControl.cs
- SqlDataSourceParameterParser.cs
- ContentDisposition.cs
- StorageMappingItemCollection.cs
- CopyNodeSetAction.cs
- Regex.cs
- PrintDialog.cs
- WindowsSolidBrush.cs
- CompositeScriptReference.cs
- BrushConverter.cs
- SettingsPropertyCollection.cs
- XmlTextEncoder.cs
- StyleSheetRefUrlEditor.cs
- OleCmdHelper.cs
- IteratorFilter.cs
- TearOffProxy.cs
- FunctionImportElement.cs
- BaseValidator.cs
- _DigestClient.cs
- ListView.cs
- AttributeCollection.cs
- AssociationTypeEmitter.cs
- OleDbInfoMessageEvent.cs
- XmlExpressionDumper.cs
- ModulesEntry.cs
- HtmlListAdapter.cs
- DataTableReader.cs
- SqlBuilder.cs
- Pool.cs
- Attributes.cs
- ClientUrlResolverWrapper.cs
- TypeBuilder.cs
- Expander.cs
- TextParagraphProperties.cs
- ConfigXmlAttribute.cs
- RadioButtonBaseAdapter.cs
- DelegatedStream.cs
- BuildManager.cs
- HyperlinkAutomationPeer.cs
- TableLayoutSettings.cs
- ReliableReplySessionChannel.cs
- PageContent.cs
- DataControlButton.cs
- CodeLinePragma.cs
- Debug.cs
- SqlEnums.cs
- FrameworkTemplate.cs
- XmlAttributeHolder.cs
- AmbientLight.cs
- ObjectRef.cs
- InputProviderSite.cs
- DayRenderEvent.cs
- SerializableAttribute.cs
- SizeAnimationClockResource.cs
- PartialCachingControl.cs
- xsdvalidator.cs
- WindowsHyperlink.cs
- EntryPointNotFoundException.cs
- TimeoutStream.cs
- CollectionBuilder.cs
- PrivilegeNotHeldException.cs
- String.cs
- WebPartAddingEventArgs.cs
- ItemList.cs
- ImageMap.cs
- SafeNativeMethods.cs
- DataGridViewAdvancedBorderStyle.cs
- RuleInfoComparer.cs
- FileDialogCustomPlaces.cs
- LinqToSqlWrapper.cs