Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / TabRenderer.cs / 1 / TabRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TabRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private TabRenderer() { } ////// This is a rendering class for the Tab control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabItem(Graphics g, Rectangle bounds, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, false, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageRectangle, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabPage(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.Tab.Pane.Normal, 0); visualStyleRenderer.DrawBackground(g, bounds); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a TabPage. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TabRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private TabRenderer() { } ////// This is a rendering class for the Tab control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabItem(Graphics g, Rectangle bounds, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, false, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageRectangle, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabPage(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.Tab.Pane.Normal, 0); visualStyleRenderer.DrawBackground(g, bounds); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a TabPage. /// ///
Link Menu
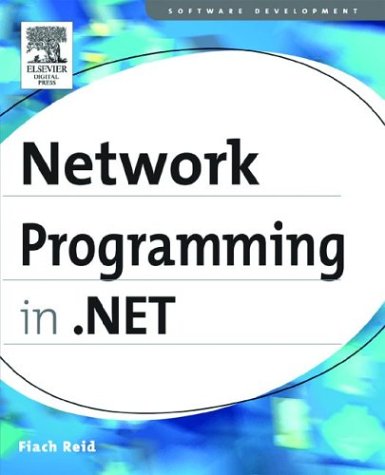
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeQuery.cs
- DBDataPermission.cs
- ConfigXmlElement.cs
- WorkflowTraceTransfer.cs
- LookupNode.cs
- CodePageEncoding.cs
- SingleSelectRootGridEntry.cs
- AlignmentYValidation.cs
- SafeWaitHandle.cs
- ValueProviderWrapper.cs
- IisTraceWebEventProvider.cs
- HiddenFieldPageStatePersister.cs
- XmlIlTypeHelper.cs
- XamlTypeMapper.cs
- KerberosRequestorSecurityTokenAuthenticator.cs
- ReliabilityContractAttribute.cs
- CornerRadiusConverter.cs
- DataServiceException.cs
- COSERVERINFO.cs
- RectKeyFrameCollection.cs
- MetadataItemCollectionFactory.cs
- MD5Cng.cs
- SurrogateEncoder.cs
- SiteMapProvider.cs
- WebBrowserProgressChangedEventHandler.cs
- CharUnicodeInfo.cs
- ManualResetEvent.cs
- TrackingSection.cs
- NativeMethodsOther.cs
- DataContractFormatAttribute.cs
- LogicalExpressionEditor.cs
- NativeMethodsCLR.cs
- MemberProjectionIndex.cs
- BuilderPropertyEntry.cs
- MailMessageEventArgs.cs
- ScaleTransform3D.cs
- SqlDataReaderSmi.cs
- LineBreak.cs
- PageSettings.cs
- RequestSecurityTokenForGetBrowserToken.cs
- StorageRoot.cs
- COM2ExtendedBrowsingHandler.cs
- TrackingProfile.cs
- DocumentSchemaValidator.cs
- BufferedStream.cs
- LookupBindingPropertiesAttribute.cs
- TransactedReceiveScope.cs
- SqlUserDefinedAggregateAttribute.cs
- Effect.cs
- HttpRuntime.cs
- XmlRawWriter.cs
- CurrencyManager.cs
- XhtmlBasicListAdapter.cs
- ThicknessAnimationBase.cs
- CurrentChangedEventManager.cs
- RichTextBoxAutomationPeer.cs
- ClientFormsIdentity.cs
- MonthCalendar.cs
- SplayTreeNode.cs
- NativeMethodsOther.cs
- SQLRoleProvider.cs
- _TransmitFileOverlappedAsyncResult.cs
- BlobPersonalizationState.cs
- HyperLink.cs
- PartDesigner.cs
- SaveFileDialog.cs
- SparseMemoryStream.cs
- AnnotationAuthorChangedEventArgs.cs
- SqlDeflator.cs
- securitymgrsite.cs
- StdRegProviderWrapper.cs
- CodeEventReferenceExpression.cs
- GroupItemAutomationPeer.cs
- ContextConfiguration.cs
- NestedContainer.cs
- XmlTextReaderImpl.cs
- PersistenceProviderDirectory.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- CacheForPrimitiveTypes.cs
- GeneralTransform3DCollection.cs
- DocumentAutomationPeer.cs
- HyperLinkColumn.cs
- ImportContext.cs
- HttpHostedTransportConfiguration.cs
- XmlStreamNodeWriter.cs
- PageThemeCodeDomTreeGenerator.cs
- GeneratedView.cs
- RadioButtonFlatAdapter.cs
- InputManager.cs
- ClrProviderManifest.cs
- ISAPIRuntime.cs
- StreamGeometryContext.cs
- RNGCryptoServiceProvider.cs
- NativeMethodsCLR.cs
- SoapIncludeAttribute.cs
- CommonRemoteMemoryBlock.cs
- TraceUtility.cs
- DragSelectionMessageFilter.cs
- PtsHost.cs
- Panel.cs