Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / AdornerDecorator.cs / 1 / AdornerDecorator.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // AdornerDecorator class. // See spec at: http://avalon/uis/Specs/AdornerLayer%20Spec.htm // // History: // 1/29/2004 psarrett: Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Threading; namespace System.Windows.Documents { ////// This AdornerDecorator does not hookup its child in the logical tree. It's being /// used by PopupRoot and FixedDocument. /// internal class NonLogicalAdornerDecorator : AdornerDecorator { public override UIElement Child { get { return IntChild; } set { if (IntChild != value) { this.RemoveVisualChild(IntChild); this.RemoveVisualChild(AdornerLayer); IntChild = value; if(value != null) { this.AddVisualChild(value); this.AddVisualChild(AdornerLayer); } InvalidateMeasure(); } } } } ////// Object which allows elements beneath it in the visual tree to be adorned. /// AdornerDecorator has two children. /// The first child is the parent of the rest of the visual tree below the AdornerDecorator. /// The second child is the AdornerLayer on which adorners are rendered. /// /// AdornerDecorator is intended to be used as part of an object's Style. /// public class AdornerDecorator : Decorator { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public AdornerDecorator() : base() { _adornerLayer = new AdornerLayer(); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// AdornerLayer on which adorners are rendered. /// public AdornerLayer AdornerLayer { get { return _adornerLayer; } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Measurement override. Implement your size-to-content logic here. /// /// /// Sizing constraint. /// protected override Size MeasureOverride(Size constraint) { Size desiredSize = base.MeasureOverride(constraint); if (VisualTreeHelper.GetParent(_adornerLayer) != null) { // We don't really care about the size of the AdornerLayer-- we'll // always just make the AdornerDecorator the full desiredSize. But // we need to measure it anyway, to make sure Adorners render. _adornerLayer.Measure(constraint); } return desiredSize; } ////// Override for /// The size reserved for this element by the parent ////// The actual ink area of the element, typically the same as finalSize protected override Size ArrangeOverride(Size finalSize) { Size inkSize = base.ArrangeOverride(finalSize); if (VisualTreeHelper.GetParent(_adornerLayer) != null) { _adornerLayer.Arrange(new Rect(finalSize)); } return (inkSize); } ////// Gets or sets the child of the AdornerDecorator. /// public override UIElement Child { get { return base.Child; } set { Visual old = base.Child; if (old == value) { return; } if (value == null) { base.Child = null; RemoveVisualChild(_adornerLayer); } else { base.Child = value; AddVisualChild(_adornerLayer); } } } ////// Returns the Visual children count. /// protected override int VisualChildrenCount { get { if (base.Child != null) { return 2; // One for the child and one for the adorner layer. } else { return 0; } } } ////// Returns the child at the specified index. /// protected override Visual GetVisualChild(int index) { if (base.Child == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } else { switch (index) { case 0: return base.Child; case 1: return _adornerLayer; default: throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } } } #endregion Protected Methods //----------------------------------------------------- // // Private Members // //----------------------------------------------------- #region Private Members // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 6; } } readonly AdornerLayer _adornerLayer; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // AdornerDecorator class. // See spec at: http://avalon/uis/Specs/AdornerLayer%20Spec.htm // // History: // 1/29/2004 psarrett: Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Threading; namespace System.Windows.Documents { ////// This AdornerDecorator does not hookup its child in the logical tree. It's being /// used by PopupRoot and FixedDocument. /// internal class NonLogicalAdornerDecorator : AdornerDecorator { public override UIElement Child { get { return IntChild; } set { if (IntChild != value) { this.RemoveVisualChild(IntChild); this.RemoveVisualChild(AdornerLayer); IntChild = value; if(value != null) { this.AddVisualChild(value); this.AddVisualChild(AdornerLayer); } InvalidateMeasure(); } } } } ////// Object which allows elements beneath it in the visual tree to be adorned. /// AdornerDecorator has two children. /// The first child is the parent of the rest of the visual tree below the AdornerDecorator. /// The second child is the AdornerLayer on which adorners are rendered. /// /// AdornerDecorator is intended to be used as part of an object's Style. /// public class AdornerDecorator : Decorator { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public AdornerDecorator() : base() { _adornerLayer = new AdornerLayer(); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// AdornerLayer on which adorners are rendered. /// public AdornerLayer AdornerLayer { get { return _adornerLayer; } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Measurement override. Implement your size-to-content logic here. /// /// /// Sizing constraint. /// protected override Size MeasureOverride(Size constraint) { Size desiredSize = base.MeasureOverride(constraint); if (VisualTreeHelper.GetParent(_adornerLayer) != null) { // We don't really care about the size of the AdornerLayer-- we'll // always just make the AdornerDecorator the full desiredSize. But // we need to measure it anyway, to make sure Adorners render. _adornerLayer.Measure(constraint); } return desiredSize; } ////// Override for /// The size reserved for this element by the parent ////// The actual ink area of the element, typically the same as finalSize protected override Size ArrangeOverride(Size finalSize) { Size inkSize = base.ArrangeOverride(finalSize); if (VisualTreeHelper.GetParent(_adornerLayer) != null) { _adornerLayer.Arrange(new Rect(finalSize)); } return (inkSize); } ////// Gets or sets the child of the AdornerDecorator. /// public override UIElement Child { get { return base.Child; } set { Visual old = base.Child; if (old == value) { return; } if (value == null) { base.Child = null; RemoveVisualChild(_adornerLayer); } else { base.Child = value; AddVisualChild(_adornerLayer); } } } ////// Returns the Visual children count. /// protected override int VisualChildrenCount { get { if (base.Child != null) { return 2; // One for the child and one for the adorner layer. } else { return 0; } } } ////// Returns the child at the specified index. /// protected override Visual GetVisualChild(int index) { if (base.Child == null) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } else { switch (index) { case 0: return base.Child; case 1: return _adornerLayer; default: throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } } } #endregion Protected Methods //----------------------------------------------------- // // Private Members // //----------------------------------------------------- #region Private Members // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 6; } } readonly AdornerLayer _adornerLayer; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
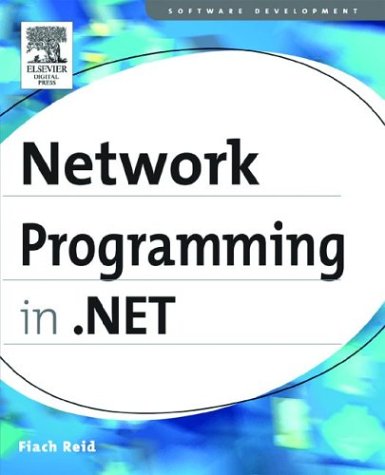
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InternalReceiveMessage.cs
- DesignerUtility.cs
- ObjectListShowCommandsEventArgs.cs
- JsonFormatGeneratorStatics.cs
- ModelPropertyCollectionImpl.cs
- IndexerNameAttribute.cs
- OneWayChannelListener.cs
- NetStream.cs
- FunctionImportElement.cs
- TypedTableBase.cs
- HttpPostedFileWrapper.cs
- Resources.Designer.cs
- Attachment.cs
- ToolStripDesignerAvailabilityAttribute.cs
- Query.cs
- WorkflowQueue.cs
- ServiceReference.cs
- ProviderConnectionPoint.cs
- LinearGradientBrush.cs
- FilterQueryOptionExpression.cs
- XPathSelectionIterator.cs
- HttpCacheVaryByContentEncodings.cs
- SerializationTrace.cs
- DatagridviewDisplayedBandsData.cs
- ManagementEventArgs.cs
- IntranetCredentialPolicy.cs
- RuntimeWrappedException.cs
- SoapReflectionImporter.cs
- PropertyPathConverter.cs
- SectionInformation.cs
- FixedPageAutomationPeer.cs
- TableParaClient.cs
- Int64AnimationUsingKeyFrames.cs
- RetrieveVirtualItemEventArgs.cs
- ValidatingPropertiesEventArgs.cs
- NativeActivityTransactionContext.cs
- OdbcHandle.cs
- LogExtentCollection.cs
- ItemsControlAutomationPeer.cs
- HMACMD5.cs
- CLRBindingWorker.cs
- EdmError.cs
- AnnotationDocumentPaginator.cs
- log.cs
- PrintPreviewDialog.cs
- ComboBoxRenderer.cs
- XmlUTF8TextWriter.cs
- CaretElement.cs
- OrthographicCamera.cs
- TrackingMemoryStream.cs
- TagPrefixInfo.cs
- _SSPISessionCache.cs
- IntSecurity.cs
- WorkerRequest.cs
- FieldToken.cs
- UIPermission.cs
- Mutex.cs
- AssemblyInfo.cs
- OperationCanceledException.cs
- HttpRequest.cs
- TerminatorSinks.cs
- ViewManagerAttribute.cs
- ScrollBarAutomationPeer.cs
- ValidationHelper.cs
- FontCollection.cs
- SqlCommandSet.cs
- SocketException.cs
- WindowsGraphicsCacheManager.cs
- RuleSetCollection.cs
- CachedCompositeFamily.cs
- LoadMessageLogger.cs
- ClientType.cs
- UrlPropertyAttribute.cs
- DifferencingCollection.cs
- AnnotationAuthorChangedEventArgs.cs
- SingleStorage.cs
- ReadonlyMessageFilter.cs
- XDeferredAxisSource.cs
- Validator.cs
- SystemTcpConnection.cs
- ActiveXHelper.cs
- NamedServiceModelExtensionCollectionElement.cs
- DataObject.cs
- PageAdapter.cs
- FacetDescriptionElement.cs
- StrokeCollection.cs
- CollectionViewGroupRoot.cs
- HttpModuleCollection.cs
- BindingBase.cs
- QueryableFilterUserControl.cs
- DefaultProxySection.cs
- FloaterBaseParagraph.cs
- DoubleKeyFrameCollection.cs
- HybridObjectCache.cs
- FileNotFoundException.cs
- WebBaseEventKeyComparer.cs
- XmlAnyElementAttributes.cs
- TraceContextEventArgs.cs
- httpserverutility.cs
- AgileSafeNativeMemoryHandle.cs