Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / Figure.cs / 1305600 / Figure.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Figure element. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Controls; // TextBlock using MS.Internal; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Figure element. /// public class Figure : AnchoredBlock { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initialized the new instance of a Figure /// public Figure() : this(null) { } ////// Initialized the new instance of a Figure specifying a Block added /// to a Figure as its first child. /// /// /// Block added as a first initial child of the Figure. /// public Figure(Block childBlock) : this(childBlock, null) { } ////// Creates a new Figure instance. /// /// /// Optional child of the new Figure, may be null. /// /// /// Optional position at which to insert the new Figure. May /// be null. /// public Figure(Block childBlock, TextPointer insertionPosition) : base(childBlock, insertionPosition) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// DependencyProperty for public static readonly DependencyProperty HorizontalAnchorProperty = DependencyProperty.Register( "HorizontalAnchor", typeof(FigureHorizontalAnchor), typeof(Figure), new FrameworkPropertyMetadata( FigureHorizontalAnchor.ColumnRight, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidHorizontalAnchor)); ///property. /// /// /// public FigureHorizontalAnchor HorizontalAnchor { get { return (FigureHorizontalAnchor)GetValue(HorizontalAnchorProperty); } set { SetValue(HorizontalAnchorProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty VerticalAnchorProperty = DependencyProperty.Register( "VerticalAnchor", typeof(FigureVerticalAnchor), typeof(Figure), new FrameworkPropertyMetadata( FigureVerticalAnchor.ParagraphTop, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidVerticalAnchor)); ///property. /// /// /// public FigureVerticalAnchor VerticalAnchor { get { return (FigureVerticalAnchor)GetValue(VerticalAnchorProperty); } set { SetValue(VerticalAnchorProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty HorizontalOffsetProperty = DependencyProperty.Register( "HorizontalOffset", typeof(double), typeof(Figure), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidOffset)); ///property. /// /// /// [TypeConverter(typeof(LengthConverter))] public double HorizontalOffset { get { return (double)GetValue(HorizontalOffsetProperty); } set { SetValue(HorizontalOffsetProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty VerticalOffsetProperty = DependencyProperty.Register( "VerticalOffset", typeof(double), typeof(Figure), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidOffset)); ///property. /// /// /// [TypeConverter(typeof(LengthConverter))] public double VerticalOffset { get { return (double)GetValue(VerticalOffsetProperty); } set { SetValue(VerticalOffsetProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty CanDelayPlacementProperty = DependencyProperty.Register( "CanDelayPlacement", typeof(bool), typeof(Figure), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// /// public bool CanDelayPlacement { get { return (bool)GetValue(CanDelayPlacementProperty); } set { SetValue(CanDelayPlacementProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty WrapDirectionProperty = DependencyProperty.Register( "WrapDirection", typeof(WrapDirection), typeof(Figure), new FrameworkPropertyMetadata( WrapDirection.Both, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidWrapDirection)); ///property. /// /// /// public WrapDirection WrapDirection { get { return (WrapDirection)GetValue(WrapDirectionProperty); } set { SetValue(WrapDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty WidthProperty = DependencyProperty.Register( "Width", typeof(FigureLength), typeof(Figure), new FrameworkPropertyMetadata( new FigureLength(1.0, FigureUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Width property specifies the width of the element. /// public FigureLength Width { get { return (FigureLength)GetValue(WidthProperty); } set { SetValue(WidthProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty HeightProperty = DependencyProperty.Register( "Height", typeof(FigureLength), typeof(Figure), new FrameworkPropertyMetadata( new FigureLength(1.0, FigureUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Height property specifies the height of the element. /// public FigureLength Height { get { return (FigureLength)GetValue(HeightProperty); } set { SetValue(HeightProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidHorizontalAnchor(object o) { FigureHorizontalAnchor value = (FigureHorizontalAnchor)o; return value == FigureHorizontalAnchor.ContentCenter || value == FigureHorizontalAnchor.ContentLeft || value == FigureHorizontalAnchor.ContentRight || value == FigureHorizontalAnchor.PageCenter || value == FigureHorizontalAnchor.PageLeft || value == FigureHorizontalAnchor.PageRight || value == FigureHorizontalAnchor.ColumnCenter || value == FigureHorizontalAnchor.ColumnLeft || value == FigureHorizontalAnchor.ColumnRight; // || value == FigureHorizontalAnchor.CharacterCenter // || value == FigureHorizontalAnchor.CharacterLeft // || value == FigureHorizontalAnchor.CharacterRight; } private static bool IsValidVerticalAnchor(object o) { FigureVerticalAnchor value = (FigureVerticalAnchor)o; return value == FigureVerticalAnchor.ContentBottom || value == FigureVerticalAnchor.ContentCenter || value == FigureVerticalAnchor.ContentTop || value == FigureVerticalAnchor.PageBottom || value == FigureVerticalAnchor.PageCenter || value == FigureVerticalAnchor.PageTop || value == FigureVerticalAnchor.ParagraphTop; // || value == FigureVerticalAnchor.CharacterBottom // || value == FigureVerticalAnchor.CharacterCenter // || value == FigureVerticalAnchor.CharacterTop; } private static bool IsValidWrapDirection(object o) { WrapDirection value = (WrapDirection)o; return value == WrapDirection.Both || value == WrapDirection.None || value == WrapDirection.Left || value == WrapDirection.Right; } private static bool IsValidOffset(object o) { double offset = (double)o; double maxOffset = Math.Min(1000000, PTS.MaxPageSize); double minOffset = -maxOffset; if (Double.IsNaN(offset)) { return false; } if (offset < minOffset || offset > maxOffset) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Figure element. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Controls; // TextBlock using MS.Internal; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Figure element. /// public class Figure : AnchoredBlock { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initialized the new instance of a Figure /// public Figure() : this(null) { } ////// Initialized the new instance of a Figure specifying a Block added /// to a Figure as its first child. /// /// /// Block added as a first initial child of the Figure. /// public Figure(Block childBlock) : this(childBlock, null) { } ////// Creates a new Figure instance. /// /// /// Optional child of the new Figure, may be null. /// /// /// Optional position at which to insert the new Figure. May /// be null. /// public Figure(Block childBlock, TextPointer insertionPosition) : base(childBlock, insertionPosition) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// DependencyProperty for public static readonly DependencyProperty HorizontalAnchorProperty = DependencyProperty.Register( "HorizontalAnchor", typeof(FigureHorizontalAnchor), typeof(Figure), new FrameworkPropertyMetadata( FigureHorizontalAnchor.ColumnRight, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidHorizontalAnchor)); ///property. /// /// /// public FigureHorizontalAnchor HorizontalAnchor { get { return (FigureHorizontalAnchor)GetValue(HorizontalAnchorProperty); } set { SetValue(HorizontalAnchorProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty VerticalAnchorProperty = DependencyProperty.Register( "VerticalAnchor", typeof(FigureVerticalAnchor), typeof(Figure), new FrameworkPropertyMetadata( FigureVerticalAnchor.ParagraphTop, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidVerticalAnchor)); ///property. /// /// /// public FigureVerticalAnchor VerticalAnchor { get { return (FigureVerticalAnchor)GetValue(VerticalAnchorProperty); } set { SetValue(VerticalAnchorProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty HorizontalOffsetProperty = DependencyProperty.Register( "HorizontalOffset", typeof(double), typeof(Figure), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidOffset)); ///property. /// /// /// [TypeConverter(typeof(LengthConverter))] public double HorizontalOffset { get { return (double)GetValue(HorizontalOffsetProperty); } set { SetValue(HorizontalOffsetProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty VerticalOffsetProperty = DependencyProperty.Register( "VerticalOffset", typeof(double), typeof(Figure), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidOffset)); ///property. /// /// /// [TypeConverter(typeof(LengthConverter))] public double VerticalOffset { get { return (double)GetValue(VerticalOffsetProperty); } set { SetValue(VerticalOffsetProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty CanDelayPlacementProperty = DependencyProperty.Register( "CanDelayPlacement", typeof(bool), typeof(Figure), new FrameworkPropertyMetadata( true, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// /// public bool CanDelayPlacement { get { return (bool)GetValue(CanDelayPlacementProperty); } set { SetValue(CanDelayPlacementProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty WrapDirectionProperty = DependencyProperty.Register( "WrapDirection", typeof(WrapDirection), typeof(Figure), new FrameworkPropertyMetadata( WrapDirection.Both, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidWrapDirection)); ///property. /// /// /// public WrapDirection WrapDirection { get { return (WrapDirection)GetValue(WrapDirectionProperty); } set { SetValue(WrapDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty WidthProperty = DependencyProperty.Register( "Width", typeof(FigureLength), typeof(Figure), new FrameworkPropertyMetadata( new FigureLength(1.0, FigureUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Width property specifies the width of the element. /// public FigureLength Width { get { return (FigureLength)GetValue(WidthProperty); } set { SetValue(WidthProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty HeightProperty = DependencyProperty.Register( "Height", typeof(FigureLength), typeof(Figure), new FrameworkPropertyMetadata( new FigureLength(1.0, FigureUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Height property specifies the height of the element. /// public FigureLength Height { get { return (FigureLength)GetValue(HeightProperty); } set { SetValue(HeightProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidHorizontalAnchor(object o) { FigureHorizontalAnchor value = (FigureHorizontalAnchor)o; return value == FigureHorizontalAnchor.ContentCenter || value == FigureHorizontalAnchor.ContentLeft || value == FigureHorizontalAnchor.ContentRight || value == FigureHorizontalAnchor.PageCenter || value == FigureHorizontalAnchor.PageLeft || value == FigureHorizontalAnchor.PageRight || value == FigureHorizontalAnchor.ColumnCenter || value == FigureHorizontalAnchor.ColumnLeft || value == FigureHorizontalAnchor.ColumnRight; // || value == FigureHorizontalAnchor.CharacterCenter // || value == FigureHorizontalAnchor.CharacterLeft // || value == FigureHorizontalAnchor.CharacterRight; } private static bool IsValidVerticalAnchor(object o) { FigureVerticalAnchor value = (FigureVerticalAnchor)o; return value == FigureVerticalAnchor.ContentBottom || value == FigureVerticalAnchor.ContentCenter || value == FigureVerticalAnchor.ContentTop || value == FigureVerticalAnchor.PageBottom || value == FigureVerticalAnchor.PageCenter || value == FigureVerticalAnchor.PageTop || value == FigureVerticalAnchor.ParagraphTop; // || value == FigureVerticalAnchor.CharacterBottom // || value == FigureVerticalAnchor.CharacterCenter // || value == FigureVerticalAnchor.CharacterTop; } private static bool IsValidWrapDirection(object o) { WrapDirection value = (WrapDirection)o; return value == WrapDirection.Both || value == WrapDirection.None || value == WrapDirection.Left || value == WrapDirection.Right; } private static bool IsValidOffset(object o) { double offset = (double)o; double maxOffset = Math.Min(1000000, PTS.MaxPageSize); double minOffset = -maxOffset; if (Double.IsNaN(offset)) { return false; } if (offset < minOffset || offset > maxOffset) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
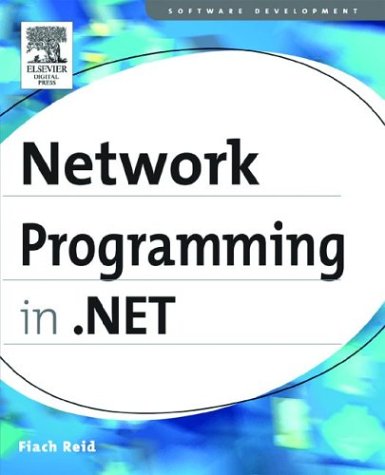
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MatrixTransform.cs
- Sorting.cs
- CodeMemberMethod.cs
- SqlFunctionAttribute.cs
- LinkLabelLinkClickedEvent.cs
- DataGridViewComboBoxColumn.cs
- ConnectionManagementElementCollection.cs
- figurelengthconverter.cs
- ExpressionConverter.cs
- SQLBinary.cs
- SpeechRecognizer.cs
- ZipIORawDataFileBlock.cs
- WeakReferenceList.cs
- FigureParaClient.cs
- InvalidWMPVersionException.cs
- DefaultDiscoveryService.cs
- SspiSecurityTokenParameters.cs
- CounterSampleCalculator.cs
- DataRowCollection.cs
- HtmlInputControl.cs
- Select.cs
- XpsImageSerializationService.cs
- ScrollItemPatternIdentifiers.cs
- ISSmlParser.cs
- EncoderParameters.cs
- ConsoleKeyInfo.cs
- SerializationAttributes.cs
- Normalization.cs
- HttpChannelListener.cs
- ProtocolsConfiguration.cs
- DataServiceEntityAttribute.cs
- FaultHandlingFilter.cs
- MethodSet.cs
- TextRangeBase.cs
- XmlWriterSettings.cs
- ImageList.cs
- InputLanguage.cs
- ToolStripItemRenderEventArgs.cs
- ProcessModelSection.cs
- SchemaInfo.cs
- EventProviderWriter.cs
- XmlUtf8RawTextWriter.cs
- TextBox.cs
- SourceInterpreter.cs
- XmlResolver.cs
- EtwTrace.cs
- Nodes.cs
- File.cs
- TreeView.cs
- TemplatedWizardStep.cs
- SimpleTypeResolver.cs
- GuidConverter.cs
- Util.cs
- Rectangle.cs
- MobileRedirect.cs
- XamlStyleSerializer.cs
- DataBinding.cs
- InvalidAsynchronousStateException.cs
- DataControlField.cs
- TextRangeSerialization.cs
- ProxyManager.cs
- ButtonBaseAutomationPeer.cs
- RootBrowserWindowProxy.cs
- SystemFonts.cs
- COAUTHIDENTITY.cs
- LicenseException.cs
- safex509handles.cs
- TimeSpanConverter.cs
- HttpServerVarsCollection.cs
- StylusPointProperty.cs
- ReferenceService.cs
- EventLogEntryCollection.cs
- CellParagraph.cs
- NamedPipeHostedTransportConfiguration.cs
- UITypeEditor.cs
- Exceptions.cs
- NetDataContractSerializer.cs
- TableHeaderCell.cs
- AttachedPropertyBrowsableAttribute.cs
- ToolBarButton.cs
- DrawingBrush.cs
- InputLangChangeRequestEvent.cs
- IteratorFilter.cs
- DrawingImage.cs
- IntPtr.cs
- EncryptedHeader.cs
- ZoomingMessageFilter.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- EncryptedXml.cs
- NavigateEvent.cs
- TextBox.cs
- ToolStripHighContrastRenderer.cs
- ToolStripDropDown.cs
- QilFactory.cs
- TimelineClockCollection.cs
- ActivityXRefConverter.cs
- FlowNode.cs
- SoapSchemaExporter.cs
- QueryContinueDragEventArgs.cs
- UserNamePasswordServiceCredential.cs