Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / EntityKeyElement.cs / 2 / EntityKeyElement.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an Key element in an EntityType element. /// internal sealed class EntityKeyElement : SchemaElement { private List_keyProperties; /// /// Constructs an EntityContainerAssociationSetEnd /// /// Reference to the schema element. public EntityKeyElement( SchemaEntityType parentElement ) : base( parentElement ) { } public IListKeyProperties { get { if (_keyProperties == null) { _keyProperties = new List (); } return _keyProperties; } } protected override bool HandleAttribute(XmlReader reader) { return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.PropertyRef)) { HandlePropertyRefElement(reader); return true; } return false; } /// /// /// /// private void HandlePropertyRefElement(XmlReader reader) { PropertyRefElement property = new PropertyRefElement((SchemaEntityType)ParentElement); property.Parse(reader); this.KeyProperties.Add(property); } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); foreach (PropertyRefElement property in _keyProperties) { if (!property.ResolveNames((SchemaEntityType)this.ParentElement)) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNoProperty(this.ParentElement.FQName, property.Name)); } } } ////// Validate all the key properties /// internal override void Validate() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); DictionarypropertyLookUp = new Dictionary (StringComparer.Ordinal); foreach (PropertyRefElement keyProperty in _keyProperties) { StructuredProperty property = keyProperty.Property; Debug.Assert(property != null, "This should never be null, since if we were not able to resolve, we should have never reached to this point"); if (propertyLookUp.ContainsKey(property.Name)) { AddError(ErrorCode.DuplicatePropertySpecifiedInEntityKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.DuplicatePropertyNameSpecifiedInEntityKey(this.ParentElement.FQName, property.Name)); continue; } propertyLookUp.Add(property.Name, keyProperty); if (property.Nullable) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNullablePart(property.Name, this.ParentElement.Name)); } // currently we only support key properties of scalar type if ((!(property.Type is ScalarType)) || (property.CollectionKind != CollectionKind.None)) { AddError(ErrorCode.EntityKeyMustBeScalar, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntityKeyMustBeScalar(property.Name, this.ParentElement.Name)); continue; } Debug.Assert(property.TypeUsage != null, "For scalar type, typeusage must be initialized"); // Bug 484200: Binary type key properties are currently not supported. PrimitiveTypeKind kind = ((PrimitiveType)property.TypeUsage.EdmType).PrimitiveTypeKind; if (!Helper.IsValidKeyType(kind)) { if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupported(property.Name, this.ParentElement.FQName, kind)); } else { Debug.Assert(SchemaDataModelOption.ProviderDataModel == Schema.DataModel, "Invalid DataModel encountered"); AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupportedInSSDL(property.Name, this.ParentElement.FQName, property.TypeUsage.EdmType.Name, property.TypeUsage.EdmType.BaseType.FullName, kind)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an Key element in an EntityType element. /// internal sealed class EntityKeyElement : SchemaElement { private List_keyProperties; /// /// Constructs an EntityContainerAssociationSetEnd /// /// Reference to the schema element. public EntityKeyElement( SchemaEntityType parentElement ) : base( parentElement ) { } public IListKeyProperties { get { if (_keyProperties == null) { _keyProperties = new List (); } return _keyProperties; } } protected override bool HandleAttribute(XmlReader reader) { return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.PropertyRef)) { HandlePropertyRefElement(reader); return true; } return false; } /// /// /// /// private void HandlePropertyRefElement(XmlReader reader) { PropertyRefElement property = new PropertyRefElement((SchemaEntityType)ParentElement); property.Parse(reader); this.KeyProperties.Add(property); } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); foreach (PropertyRefElement property in _keyProperties) { if (!property.ResolveNames((SchemaEntityType)this.ParentElement)) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNoProperty(this.ParentElement.FQName, property.Name)); } } } ////// Validate all the key properties /// internal override void Validate() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); DictionarypropertyLookUp = new Dictionary (StringComparer.Ordinal); foreach (PropertyRefElement keyProperty in _keyProperties) { StructuredProperty property = keyProperty.Property; Debug.Assert(property != null, "This should never be null, since if we were not able to resolve, we should have never reached to this point"); if (propertyLookUp.ContainsKey(property.Name)) { AddError(ErrorCode.DuplicatePropertySpecifiedInEntityKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.DuplicatePropertyNameSpecifiedInEntityKey(this.ParentElement.FQName, property.Name)); continue; } propertyLookUp.Add(property.Name, keyProperty); if (property.Nullable) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNullablePart(property.Name, this.ParentElement.Name)); } // currently we only support key properties of scalar type if ((!(property.Type is ScalarType)) || (property.CollectionKind != CollectionKind.None)) { AddError(ErrorCode.EntityKeyMustBeScalar, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntityKeyMustBeScalar(property.Name, this.ParentElement.Name)); continue; } Debug.Assert(property.TypeUsage != null, "For scalar type, typeusage must be initialized"); // Bug 484200: Binary type key properties are currently not supported. PrimitiveTypeKind kind = ((PrimitiveType)property.TypeUsage.EdmType).PrimitiveTypeKind; if (!Helper.IsValidKeyType(kind)) { if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupported(property.Name, this.ParentElement.FQName, kind)); } else { Debug.Assert(SchemaDataModelOption.ProviderDataModel == Schema.DataModel, "Invalid DataModel encountered"); AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupportedInSSDL(property.Name, this.ParentElement.FQName, property.TypeUsage.EdmType.Name, property.TypeUsage.EdmType.BaseType.FullName, kind)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
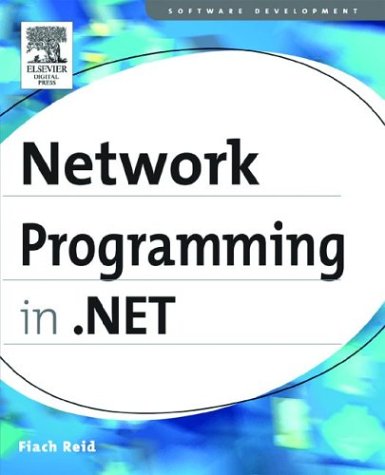
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- rsa.cs
- CodeDomLoader.cs
- FileLogRecordEnumerator.cs
- DataListItemCollection.cs
- GlyphingCache.cs
- AutomationPropertyInfo.cs
- List.cs
- WebBrowserNavigatingEventHandler.cs
- assemblycache.cs
- ReadOnlyDictionary.cs
- clipboard.cs
- SrgsGrammarCompiler.cs
- WmlPageAdapter.cs
- RegexTree.cs
- ProfessionalColorTable.cs
- Size3D.cs
- SmiSettersStream.cs
- ToolStripContainerActionList.cs
- PseudoWebRequest.cs
- ProtocolViolationException.cs
- DataSourceConverter.cs
- FrameworkContentElement.cs
- PreloadHost.cs
- BezierSegment.cs
- BuildResult.cs
- IntSecurity.cs
- NullableConverter.cs
- LinqDataSourceStatusEventArgs.cs
- XmlSchemaFacet.cs
- RawAppCommandInputReport.cs
- UTF8Encoding.cs
- QuarticEase.cs
- ListViewInsertEventArgs.cs
- SQLInt64Storage.cs
- IMembershipProvider.cs
- SqlConnectionManager.cs
- TiffBitmapDecoder.cs
- FakeModelPropertyImpl.cs
- InvokeGenerator.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- ThrowHelper.cs
- OrthographicCamera.cs
- TemplateControlParser.cs
- HttpModuleCollection.cs
- EmbeddedObject.cs
- BoolExpression.cs
- Timer.cs
- DeferredElementTreeState.cs
- MetafileHeader.cs
- InheritedPropertyChangedEventArgs.cs
- MemoryFailPoint.cs
- QueryResult.cs
- UrlMappingsModule.cs
- StrokeCollection2.cs
- UrlAuthorizationModule.cs
- RotateTransform.cs
- ComponentCommands.cs
- StateManagedCollection.cs
- UnmanagedMemoryStreamWrapper.cs
- SectionUpdates.cs
- PhysicalOps.cs
- DispatcherExceptionFilterEventArgs.cs
- KeyGesture.cs
- MaskPropertyEditor.cs
- ZipIOBlockManager.cs
- DetailsViewUpdateEventArgs.cs
- SourceFileBuildProvider.cs
- Metafile.cs
- XmlSchemaSimpleContent.cs
- XmlSchemaImport.cs
- SafeLibraryHandle.cs
- BlockUIContainer.cs
- CompilationSection.cs
- TextDecorationCollection.cs
- SystemParameters.cs
- MethodRental.cs
- WebSysDescriptionAttribute.cs
- altserialization.cs
- ISessionStateStore.cs
- AffineTransform3D.cs
- metadatamappinghashervisitor.cs
- ObjectDisposedException.cs
- XmlIlVisitor.cs
- ResourcesGenerator.cs
- XmlSchemaInfo.cs
- ContainerControl.cs
- BaseTemplateParser.cs
- BindingOperations.cs
- Set.cs
- ControlType.cs
- LinqDataSourceEditData.cs
- X509Extension.cs
- DataServiceCollectionOfT.cs
- TextCompositionEventArgs.cs
- FileSystemInfo.cs
- TypeValidationEventArgs.cs
- SqlDataAdapter.cs
- DelegateHelpers.cs
- UserUseLicenseDictionaryLoader.cs
- RealizedColumnsBlock.cs