Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Security / IMembershipProvider.cs / 1 / IMembershipProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Web; using System.Security.Principal; using System.Collections.Specialized; using System.Web.Configuration; using System.Security.Permissions; using System.Globalization; using System.Security.Cryptography; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Text; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class MembershipProvider : ProviderBase { private const int SALT_SIZE_IN_BYTES = 16; // // Property Section // // Public properties public abstract bool EnablePasswordRetrieval { get; } public abstract bool EnablePasswordReset { get; } public abstract bool RequiresQuestionAndAnswer { get; } public abstract string ApplicationName { get; set; } public abstract int MaxInvalidPasswordAttempts { get; } public abstract int PasswordAttemptWindow { get; } public abstract bool RequiresUniqueEmail { get; } public abstract MembershipPasswordFormat PasswordFormat { get; } public abstract int MinRequiredPasswordLength { get; } public abstract int MinRequiredNonAlphanumericCharacters { get; } public abstract string PasswordStrengthRegularExpression { get; } // // Method Section // public abstract MembershipUser CreateUser( string username, string password, string email, string passwordQuestion, string passwordAnswer, bool isApproved, object providerUserKey, out MembershipCreateStatus status ); public abstract bool ChangePasswordQuestionAndAnswer(string username, string password, string newPasswordQuestion, string newPasswordAnswer); public abstract string GetPassword(string username, string answer); public abstract bool ChangePassword(string username, string oldPassword, string newPassword); public abstract string ResetPassword(string username, string answer); public abstract void UpdateUser(MembershipUser user); public abstract bool ValidateUser(string username, string password); public abstract bool UnlockUser( string userName ); public abstract MembershipUser GetUser( object providerUserKey, bool userIsOnline ); public abstract MembershipUser GetUser(string username, bool userIsOnline); // GetUser() can throw 1 type of exception: // 1. ArgumentException is thrown if: // A. Username is null, is empty, contains commas, or is longer than 256 characters internal MembershipUser GetUser(string username, bool userIsOnline, bool throwOnError) { MembershipUser user = null; try { user = GetUser(username, userIsOnline); } catch (ArgumentException) { if (throwOnError) throw; } return user; } public abstract string GetUserNameByEmail(string email); public abstract bool DeleteUser(string username, bool deleteAllRelatedData); public abstract MembershipUserCollection GetAllUsers(int pageIndex, int pageSize, out int totalRecords); public abstract int GetNumberOfUsersOnline(); public abstract MembershipUserCollection FindUsersByName(string usernameToMatch, int pageIndex, int pageSize, out int totalRecords); public abstract MembershipUserCollection FindUsersByEmail(string emailToMatch, int pageIndex, int pageSize, out int totalRecords); protected virtual byte[] EncryptPassword( byte[] password ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); return MachineKeySection.EncryptOrDecryptData(true, password, null, 0, password.Length); } protected virtual byte[] DecryptPassword( byte[] encodedPassword ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); return MachineKeySection.EncryptOrDecryptData(false, encodedPassword, null, 0, encodedPassword.Length); } internal string EncodePassword(string pass, int passwordFormat, string salt) { if (passwordFormat == 0) // MembershipPasswordFormat.Clear return pass; byte[] bIn = Encoding.Unicode.GetBytes(pass); byte[] bSalt = Convert.FromBase64String(salt); byte[] bAll = new byte[bSalt.Length + bIn.Length]; byte[] bRet = null; Buffer.BlockCopy(bSalt, 0, bAll, 0, bSalt.Length); Buffer.BlockCopy(bIn, 0, bAll, bSalt.Length, bIn.Length); if (passwordFormat == 1) { // MembershipPasswordFormat.Hashed #if !FEATURE_PAL // FEATURE_PAL does not enable cryptography HashAlgorithm s = HashAlgorithm.Create( Membership.HashAlgorithmType ); // If the hash algorithm is null (and came from config), throw a config exception if (s == null) { if ( Membership.IsHashAlgorithmFromMembershipConfig ) { MembershipSection settings = RuntimeConfig.GetAppConfig().Membership; settings.ThrowHashAlgorithmException(); } } bRet = s.ComputeHash(bAll); #endif // !FEATURE_PAL } else { bRet = EncryptPassword( bAll ); } return Convert.ToBase64String(bRet); } internal string UnEncodePassword(string pass, int passwordFormat) { switch (passwordFormat) { case 0: // MembershipPasswordFormat.Clear: return pass; case 1: // MembershipPasswordFormat.Hashed: throw new ProviderException(SR.GetString(SR.Provider_can_not_decode_hashed_password)); default: byte[] bIn = Convert.FromBase64String(pass); byte[] bRet = DecryptPassword( bIn ); if (bRet == null) return null; return Encoding.Unicode.GetString(bRet, SALT_SIZE_IN_BYTES, bRet.Length - SALT_SIZE_IN_BYTES); } } internal string GenerateSalt() { byte[] buf = new byte[SALT_SIZE_IN_BYTES]; (new RNGCryptoServiceProvider()).GetBytes(buf); return Convert.ToBase64String(buf); } // // Event Section // public event MembershipValidatePasswordEventHandler ValidatingPassword { add { _EventHandler += value; } remove { _EventHandler -= value; } } protected virtual void OnValidatingPassword( ValidatePasswordEventArgs e ) { if( _EventHandler != null ) { _EventHandler( this, e ); } } private MembershipValidatePasswordEventHandler _EventHandler; } }[To be supplied.] ///
Link Menu
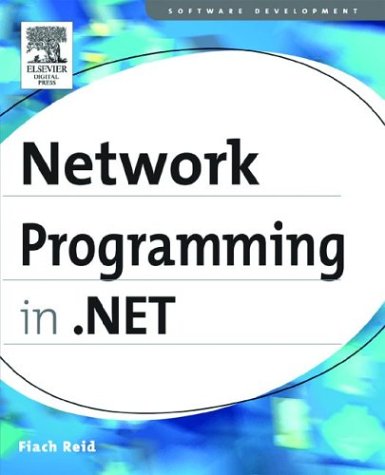
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CreateUserErrorEventArgs.cs
- FixedSOMPageConstructor.cs
- Ref.cs
- FixedSOMSemanticBox.cs
- TypeElement.cs
- VectorAnimationBase.cs
- MetaType.cs
- DataGridPageChangedEventArgs.cs
- DomainLiteralReader.cs
- XmlReflectionMember.cs
- ReferentialConstraintRoleElement.cs
- WebPartConnectionsConfigureVerb.cs
- BoolExpr.cs
- ClickablePoint.cs
- MetadataArtifactLoader.cs
- UnsafeNativeMethods.cs
- EntityTransaction.cs
- QueryExecutionOption.cs
- QueryFunctions.cs
- ADMembershipProvider.cs
- Repeater.cs
- CopyNamespacesAction.cs
- DateRangeEvent.cs
- CompositeFontParser.cs
- OleDbDataAdapter.cs
- ToolTipService.cs
- CapabilitiesAssignment.cs
- XmlJsonWriter.cs
- TextServicesPropertyRanges.cs
- WrapPanel.cs
- ChangePasswordAutoFormat.cs
- CustomError.cs
- RegularExpressionValidator.cs
- ExpressionEditorAttribute.cs
- IImplicitResourceProvider.cs
- Int32Collection.cs
- Accessors.cs
- SiteMapDataSource.cs
- HatchBrush.cs
- DesignerActionMethodItem.cs
- Regex.cs
- FacetValues.cs
- AnnotationAuthorChangedEventArgs.cs
- ReversePositionQuery.cs
- DesignerVerb.cs
- EdmMember.cs
- PropertyToken.cs
- XsltOutput.cs
- ExpressionWriter.cs
- VirtualPathData.cs
- DateRangeEvent.cs
- BoundColumn.cs
- WindowsFormsHostPropertyMap.cs
- TextSchema.cs
- _NetRes.cs
- Int64Storage.cs
- ControlBuilderAttribute.cs
- CommaDelimitedStringAttributeCollectionConverter.cs
- X509ChainElement.cs
- OwnerDrawPropertyBag.cs
- Roles.cs
- XDRSchema.cs
- OracleInternalConnection.cs
- ScrollViewer.cs
- MsmqBindingBase.cs
- ComPlusServiceHost.cs
- _SslStream.cs
- NetPeerTcpBindingElement.cs
- ColorTranslator.cs
- ToolStripSplitButton.cs
- SystemWebSectionGroup.cs
- Logging.cs
- InputChannel.cs
- HttpHandlerActionCollection.cs
- OptimalTextSource.cs
- CodeCommentStatementCollection.cs
- Pen.cs
- RequestTimeoutManager.cs
- CustomTypeDescriptor.cs
- EtwTrace.cs
- XPathAxisIterator.cs
- ServiceDocument.cs
- PerformanceCounterCategory.cs
- XmlDictionaryReaderQuotas.cs
- TypedAsyncResult.cs
- JoinQueryOperator.cs
- DocumentPageTextView.cs
- MailMessage.cs
- PrtCap_Reader.cs
- InputLanguageManager.cs
- List.cs
- SubclassTypeValidatorAttribute.cs
- Content.cs
- KnownBoxes.cs
- CounterNameConverter.cs
- CompilerError.cs
- ButtonBase.cs
- ErrorWrapper.cs
- PanelDesigner.cs
- ApplicationId.cs