Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / ServiceDocument.cs / 1 / ServiceDocument.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System.Collections.ObjectModel; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Collections.Generic; using System.Xml; public class ServiceDocument : IExtensibleSyndicationObject { Uri baseUri; ExtensibleSyndicationObject extensions = new ExtensibleSyndicationObject(); string language; Collectionworkspaces; public ServiceDocument() : this(null) { } public ServiceDocument(IEnumerable workspaces) { if (workspaces != null) { this.workspaces = new NullNotAllowedCollection (); foreach (Workspace workspace in workspaces) { this.workspaces.Add(workspace); } } } public Dictionary AttributeExtensions { get { return this.extensions.AttributeExtensions; } } public Uri BaseUri { get { return this.baseUri; } set { this.baseUri = value; } } public SyndicationElementExtensionCollection ElementExtensions { get { return this.extensions.ElementExtensions; } } public string Language { get { return this.language; } set { this.language = value; } } public Collection Workspaces { get { if (this.workspaces == null) { this.workspaces = new NullNotAllowedCollection (); } return this.workspaces; } } public static ServiceDocument Load(XmlReader reader) { return Load (reader); } public static TServiceDocument Load (XmlReader reader) where TServiceDocument : ServiceDocument, new () { AtomPub10ServiceDocumentFormatter formatter = new AtomPub10ServiceDocumentFormatter (); formatter.ReadFrom(reader); return (TServiceDocument)(object) formatter.Document; } public ServiceDocumentFormatter GetFormatter() { return new AtomPub10ServiceDocumentFormatter(this); } public void Save(XmlWriter writer) { new AtomPub10ServiceDocumentFormatter(this).WriteTo(writer); } protected internal virtual Workspace CreateWorkspace() { return new Workspace(); } protected internal virtual bool TryParseAttribute(string name, string ns, string value, string version) { return false; } protected internal virtual bool TryParseElement(XmlReader reader, string version) { return false; } protected internal virtual void WriteAttributeExtensions(XmlWriter writer, string version) { this.extensions.WriteAttributeExtensions(writer); } protected internal virtual void WriteElementExtensions(XmlWriter writer, string version) { this.extensions.WriteElementExtensions(writer); } internal void LoadElementExtensions(XmlReader readerOverUnparsedExtensions, int maxExtensionSize) { this.extensions.LoadElementExtensions(readerOverUnparsedExtensions, maxExtensionSize); } internal void LoadElementExtensions(XmlBuffer buffer) { this.extensions.LoadElementExtensions(buffer); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
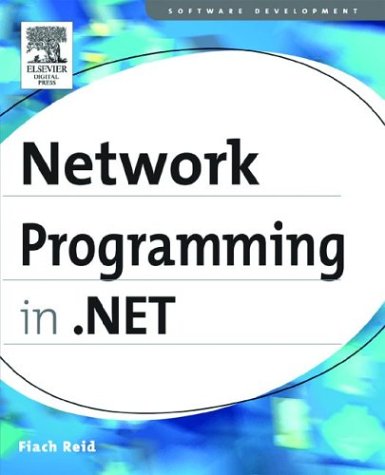
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScriptingSectionGroup.cs
- ParentUndoUnit.cs
- EditCommandColumn.cs
- RectAnimationUsingKeyFrames.cs
- WebEvents.cs
- LineBreak.cs
- PerformanceCounterTraceRecord.cs
- GridViewColumnHeaderAutomationPeer.cs
- AttributeAction.cs
- InkPresenterAutomationPeer.cs
- Model3DCollection.cs
- Thumb.cs
- HoistedLocals.cs
- DatagridviewDisplayedBandsData.cs
- FlagsAttribute.cs
- UserControlAutomationPeer.cs
- DrawingBrush.cs
- TextLineResult.cs
- EditorPartChrome.cs
- NullReferenceException.cs
- StylusPointPropertyInfoDefaults.cs
- PageRanges.cs
- ComplexType.cs
- FileUpload.cs
- ProgressChangedEventArgs.cs
- CodeDelegateCreateExpression.cs
- Color.cs
- MethodCallConverter.cs
- TimeSpanMinutesConverter.cs
- InfocardInteractiveChannelInitializer.cs
- XmlSecureResolver.cs
- AdjustableArrowCap.cs
- MetadataItem_Static.cs
- WmlLiteralTextAdapter.cs
- FieldAccessException.cs
- ChildTable.cs
- ArglessEventHandlerProxy.cs
- ServiceDesigner.xaml.cs
- ListViewHitTestInfo.cs
- _ProxyRegBlob.cs
- List.cs
- ReadWriteSpinLock.cs
- CurrencyManager.cs
- PeerTransportBindingElement.cs
- HttpModulesInstallComponent.cs
- CompilerTypeWithParams.cs
- EventMap.cs
- Misc.cs
- CommandLibraryHelper.cs
- ColumnMap.cs
- EdmValidator.cs
- CustomAttribute.cs
- shaper.cs
- SelectionRangeConverter.cs
- Repeater.cs
- GridViewUpdateEventArgs.cs
- FixedSOMImage.cs
- MenuCommand.cs
- OrderedDictionary.cs
- COAUTHINFO.cs
- XPathAxisIterator.cs
- OpCellTreeNode.cs
- CoreSwitches.cs
- AggregateNode.cs
- BitStack.cs
- MimeMultiPart.cs
- BinaryKeyIdentifierClause.cs
- ListItemConverter.cs
- PersonalizationState.cs
- ShaderEffect.cs
- XmlStringTable.cs
- WriteFileContext.cs
- Margins.cs
- _Events.cs
- SecurityTokenAuthenticator.cs
- DesignerActionKeyboardBehavior.cs
- StrokeCollectionDefaultValueFactory.cs
- MarkupExtensionParser.cs
- MaskedTextBoxDesignerActionList.cs
- HwndProxyElementProvider.cs
- ToolStripSystemRenderer.cs
- XsltContext.cs
- XsltContext.cs
- VersionedStream.cs
- PersonalizationEntry.cs
- HGlobalSafeHandle.cs
- SchemaCollectionPreprocessor.cs
- ControlParameter.cs
- TextDecorationCollection.cs
- TrackBarRenderer.cs
- TransformGroup.cs
- Registry.cs
- WebPartsSection.cs
- HttpHandlerActionCollection.cs
- SimpleHandlerFactory.cs
- QueryOutputWriter.cs
- CachedFontFamily.cs
- COM2Enum.cs
- ServiceControllerDesigner.cs
- TextBox.cs