Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / System.Runtime.DurableInstancing / System / Runtime / ReadOnlyDictionary.cs / 1305376 / ReadOnlyDictionary.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Runtime { using System.Collections; using System.Collections.Generic; [Serializable] class ReadOnlyDictionary: IDictionary { IDictionary dictionary; public ReadOnlyDictionary(IDictionary dictionary) : this(dictionary, true) { } public ReadOnlyDictionary(IDictionary dictionary, bool makeCopy) { if (makeCopy) { this.dictionary = new Dictionary (dictionary); } else { this.dictionary = dictionary; } } public int Count { get { return this.dictionary.Count; } } public bool IsReadOnly { get { return true; } } public ICollection Keys { get { return this.dictionary.Keys; } } public ICollection Values { get { return this.dictionary.Values; } } public TValue this[TKey key] { get { return this.dictionary[key]; } set { throw Fx.Exception.AsError(CreateReadOnlyException()); } } public static IDictionary Create(IDictionary dictionary) { if (dictionary.IsReadOnly) { return dictionary; } else { return new ReadOnlyDictionary (dictionary); } } Exception CreateReadOnlyException() { return new InvalidOperationException(SRCore.DictionaryIsReadOnly); } public void Add(TKey key, TValue value) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public void Add(KeyValuePair item) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public void Clear() { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool Contains(KeyValuePair item) { return this.dictionary.Contains(item); } public bool ContainsKey(TKey key) { return this.dictionary.ContainsKey(key); } public void CopyTo(KeyValuePair [] array, int arrayIndex) { this.dictionary.CopyTo(array, arrayIndex); } public IEnumerator > GetEnumerator() { return this.dictionary.GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public bool Remove(TKey key) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool Remove(KeyValuePair item) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool TryGetValue(TKey key, out TValue value) { return this.dictionary.TryGetValue(key, out value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Runtime { using System.Collections; using System.Collections.Generic; [Serializable] class ReadOnlyDictionary : IDictionary { IDictionary dictionary; public ReadOnlyDictionary(IDictionary dictionary) : this(dictionary, true) { } public ReadOnlyDictionary(IDictionary dictionary, bool makeCopy) { if (makeCopy) { this.dictionary = new Dictionary (dictionary); } else { this.dictionary = dictionary; } } public int Count { get { return this.dictionary.Count; } } public bool IsReadOnly { get { return true; } } public ICollection Keys { get { return this.dictionary.Keys; } } public ICollection Values { get { return this.dictionary.Values; } } public TValue this[TKey key] { get { return this.dictionary[key]; } set { throw Fx.Exception.AsError(CreateReadOnlyException()); } } public static IDictionary Create(IDictionary dictionary) { if (dictionary.IsReadOnly) { return dictionary; } else { return new ReadOnlyDictionary (dictionary); } } Exception CreateReadOnlyException() { return new InvalidOperationException(SRCore.DictionaryIsReadOnly); } public void Add(TKey key, TValue value) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public void Add(KeyValuePair item) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public void Clear() { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool Contains(KeyValuePair item) { return this.dictionary.Contains(item); } public bool ContainsKey(TKey key) { return this.dictionary.ContainsKey(key); } public void CopyTo(KeyValuePair [] array, int arrayIndex) { this.dictionary.CopyTo(array, arrayIndex); } public IEnumerator > GetEnumerator() { return this.dictionary.GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public bool Remove(TKey key) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool Remove(KeyValuePair item) { throw Fx.Exception.AsError(CreateReadOnlyException()); } public bool TryGetValue(TKey key, out TValue value) { return this.dictionary.TryGetValue(key, out value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
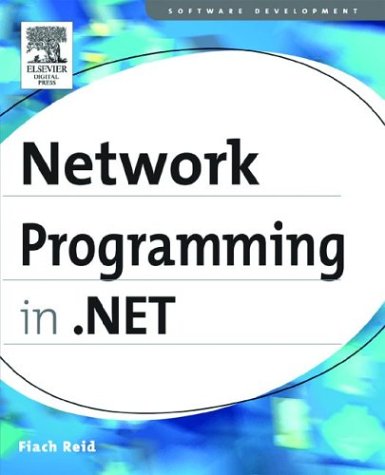
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionChangeEventArgs.cs
- SystemIPAddressInformation.cs
- InfoCardMasterKey.cs
- NavigateEvent.cs
- SecurityResources.cs
- Compilation.cs
- DigestTraceRecordHelper.cs
- BamlBinaryWriter.cs
- HttpRuntime.cs
- Wizard.cs
- SocketCache.cs
- HttpListenerException.cs
- InstanceData.cs
- CommandExpr.cs
- RectAnimationBase.cs
- DrawingGroup.cs
- AuthenticatingEventArgs.cs
- Thread.cs
- EntityProviderFactory.cs
- Rfc2898DeriveBytes.cs
- ObjectDataSourceFilteringEventArgs.cs
- DesignerLoader.cs
- CustomAttribute.cs
- ActivityBuilderXamlWriter.cs
- X509Utils.cs
- StandardBindingElementCollection.cs
- ChooseAction.cs
- WebMessageEncoderFactory.cs
- ConcurrentQueue.cs
- TypeContext.cs
- ToolStripSettings.cs
- TcpConnectionPoolSettingsElement.cs
- AggregateNode.cs
- XXXOnTypeBuilderInstantiation.cs
- DetailsViewPageEventArgs.cs
- SecurityResources.cs
- FormsAuthenticationCredentials.cs
- KoreanCalendar.cs
- MailBnfHelper.cs
- XmlReflectionMember.cs
- PathStreamGeometryContext.cs
- ImageListImage.cs
- ToolbarAUtomationPeer.cs
- PrintDialogDesigner.cs
- RegexCode.cs
- TableLayoutSettings.cs
- JsonEncodingStreamWrapper.cs
- Pair.cs
- TimeSpanOrInfiniteConverter.cs
- AuthorizationSection.cs
- BooleanConverter.cs
- ItemCollection.cs
- Context.cs
- GestureRecognitionResult.cs
- StylusPlugInCollection.cs
- Debug.cs
- HitTestParameters.cs
- LocalFileSettingsProvider.cs
- VolatileEnlistmentMultiplexing.cs
- WinEventHandler.cs
- ConfigurationPermission.cs
- IOThreadScheduler.cs
- PostBackTrigger.cs
- DataGridCellsPanel.cs
- SafeSystemMetrics.cs
- Delay.cs
- TypeDescriptionProviderAttribute.cs
- ReferentialConstraintRoleElement.cs
- FamilyMapCollection.cs
- SqlUserDefinedAggregateAttribute.cs
- PixelFormatConverter.cs
- DataGridParentRows.cs
- SqlCaseSimplifier.cs
- TextPenaltyModule.cs
- StrokeSerializer.cs
- ReverseComparer.cs
- PostBackOptions.cs
- DataGridViewComboBoxColumn.cs
- DataGridAutoFormat.cs
- StrokeCollection.cs
- ServiceModelSectionGroup.cs
- TextDecorationCollection.cs
- LoadedEvent.cs
- SerializationInfoEnumerator.cs
- ComEventsInfo.cs
- cache.cs
- SqlDataSourceFilteringEventArgs.cs
- ObjectTypeMapping.cs
- OleDbCommandBuilder.cs
- HorizontalAlignConverter.cs
- SqlBulkCopy.cs
- ChannelCredentials.cs
- XmlDataCollection.cs
- ArgumentException.cs
- IssuanceTokenProviderState.cs
- ReachSerializer.cs
- ZipIOExtraFieldElement.cs
- ArglessEventHandlerProxy.cs
- ListSurrogate.cs
- FillRuleValidation.cs