Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / SystemParameters.cs / 1305600 / SystemParameters.cs
using System; using System.Collections; using System.ComponentModel; using System.Runtime.InteropServices; using System.Windows.Media; using System.Security; using System.Windows.Controls.Primitives; using Microsoft.Win32; using MS.Win32; using MS.Internal; using MS.Internal.Interop; using MS.Internal.KnownBoxes; // Disable pragma warnings to enable PREsharp pragmas #pragma warning disable 1634, 1691 namespace System.Windows { ////// Indicates whether the system power is online, or that the system power status is unknown. /// public enum PowerLineStatus { ////// The system is offline. /// Offline = 0x00, ////// The system is online. /// Online = 0x01, ////// The power status of the system is unknown. /// Unknown = 0xFF, } ////// Contains properties that are queries into the system's various settings. /// public static class SystemParameters { // Disable Warning 6503 Property get methods should not throw exceptions. // By design properties below throw Win32Exception if there is an error when calling the native method #pragma warning disable 6503 // Win32Exception will get the last Win32 error code in case of errors, so we don't have to. #pragma warning disable 6523 #region Accessibility Parameters ////// Maps to SPI_GETFOCUSBORDERWIDTH /// ////// PublicOK -- Determined safe: getting the size of the dotted rectangle around a selected obj /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusBorderWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusBorderWidth]) { int focusBorderWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOCUSBORDERWIDTH, 0, ref focusBorderWidth, 0)) { _focusBorderWidth = ConvertPixel(focusBorderWidth); _cacheValid[(int)CacheSlot.FocusBorderWidth] = true; } else { throw new Win32Exception(); } } } return _focusBorderWidth; } } ////// Maps to SPI_GETFOCUSBORDERHEIGHT /// ////// PublicOK -- Determined safe: getting the size of the dotted rectangle around a selected obj /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusBorderHeight { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusBorderHeight]) { int focusBorderHeight = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOCUSBORDERHEIGHT, 0, ref focusBorderHeight, 0)) { _focusBorderHeight = ConvertPixel(focusBorderHeight); _cacheValid[(int)CacheSlot.FocusBorderHeight] = true; } else { throw new Win32Exception(); } } } return _focusBorderHeight; } } ////// Maps to SPI_GETHIGHCONTRAST -> HCF_HIGHCONTRASTON /// ////// Critical as this code does an elevation. /// PublicOK - considered ok to expose since the method doesn't take user input and only /// returns a boolean value which indicates the current high contrast mode. /// public static bool HighContrast { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HighContrast]) { NativeMethods.HIGHCONTRAST_I highContrast = new NativeMethods.HIGHCONTRAST_I(); highContrast.cbSize = Marshal.SizeOf(typeof(NativeMethods.HIGHCONTRAST_I)); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHIGHCONTRAST, highContrast.cbSize, ref highContrast, 0)) { _highContrast = (highContrast.dwFlags & NativeMethods.HCF_HIGHCONTRASTON) == NativeMethods.HCF_HIGHCONTRASTON; _cacheValid[(int)CacheSlot.HighContrast] = true; } else { throw new Win32Exception(); } } } return _highContrast; } } ////// Maps to SPI_GETMOUSEVANISH. /// ////// Critical -- calling UnsafeNativeMethods /// PublicOK - considered ok to expose. /// // internal static bool MouseVanish { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseVanish]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEVANISH, 0, ref _mouseVanish, 0)) { _cacheValid[(int)CacheSlot.MouseVanish] = true; } else { throw new Win32Exception(); } } } return _mouseVanish; } } #endregion [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static SystemResourceKey CreateInstance(SystemResourceKeyID KeyId) { return new SystemResourceKey(KeyId); } #region Accessibility Keys ////// FocusBorderWidth System Resource Key /// public static ResourceKey FocusBorderWidthKey { get { if (_cacheFocusBorderWidth == null) { _cacheFocusBorderWidth = CreateInstance(SystemResourceKeyID.FocusBorderWidth); } return _cacheFocusBorderWidth; } } ////// FocusBorderHeight System Resource Key /// public static ResourceKey FocusBorderHeightKey { get { if (_cacheFocusBorderHeight == null) { _cacheFocusBorderHeight = CreateInstance(SystemResourceKeyID.FocusBorderHeight); } return _cacheFocusBorderHeight; } } ////// HighContrast System Resource Key /// public static ResourceKey HighContrastKey { get { if (_cacheHighContrast == null) { _cacheHighContrast = CreateInstance(SystemResourceKeyID.HighContrast); } return _cacheHighContrast; } } #endregion #region Desktop Parameters ////// Maps to SPI_GETDROPSHADOW /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool DropShadow { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.DropShadow]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDROPSHADOW, 0, ref _dropShadow, 0)) { _cacheValid[(int)CacheSlot.DropShadow] = true; } else { throw new Win32Exception(); } } } return _dropShadow; } } ////// Maps to SPI_GETFLATMENU /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool FlatMenu { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FlatMenu]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFLATMENU, 0, ref _flatMenu, 0)) { _cacheValid[(int)CacheSlot.FlatMenu] = true; } else { throw new Win32Exception(); } } } return _flatMenu; } } ////// Maps to SPI_GETWORKAREA /// ////// SecurityCritical because it calls an unsafe native method. /// TreatAsSafe - Okay to expose info to internet callers. /// internal static NativeMethods.RECT WorkAreaInternal { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WorkAreaInternal]) { _workAreaInternal = new NativeMethods.RECT(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWORKAREA, 0, ref _workAreaInternal, 0)) { _cacheValid[(int)CacheSlot.WorkAreaInternal] = true; } else { throw new Win32Exception(); } } } return _workAreaInternal; } } ////// Maps to SPI_GETWORKAREA /// public static Rect WorkArea { get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WorkArea]) { NativeMethods.RECT workArea = WorkAreaInternal; _workArea = new Rect(ConvertPixel(workArea.left), ConvertPixel(workArea.top), ConvertPixel(workArea.right - workArea.left + 1), ConvertPixel(workArea.bottom - workArea.top + 1)); _cacheValid[(int)CacheSlot.WorkArea] = true; } } return _workArea; } } #endregion #region Desktop Keys ////// DropShadow System Resource Key /// public static ResourceKey DropShadowKey { get { if (_cacheDropShadow == null) { _cacheDropShadow = CreateInstance(SystemResourceKeyID.DropShadow); } return _cacheDropShadow; } } ////// FlatMenu System Resource Key /// public static ResourceKey FlatMenuKey { get { if (_cacheFlatMenu == null) { _cacheFlatMenu = CreateInstance(SystemResourceKeyID.FlatMenu); } return _cacheFlatMenu; } } ////// WorkArea System Resource Key /// public static ResourceKey WorkAreaKey { get { if (_cacheWorkArea == null) { _cacheWorkArea = CreateInstance(SystemResourceKeyID.WorkArea); } return _cacheWorkArea; } } #endregion #region Icon Parameters ////// Maps to SPI_GETICONMETRICS /// ////// SecurityCritical because it calls an unsafe native method. /// TreatAsSafe - Okay to expose info to internet callers. /// internal static NativeMethods.ICONMETRICS IconMetrics { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconMetrics]) { _iconMetrics = new NativeMethods.ICONMETRICS(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETICONMETRICS, _iconMetrics.cbSize, _iconMetrics, 0)) { _cacheValid[(int)CacheSlot.IconMetrics] = true; } else { throw new Win32Exception(); } } } return _iconMetrics; } } ////// Maps to SPI_GETICONMETRICS -> iHorzSpacing or SPI_ICONHORIZONTALSPACING /// public static double IconHorizontalSpacing { get { return ConvertPixel(IconMetrics.iHorzSpacing); } } ////// Maps to SPI_GETICONMETRICS -> iVertSpacing or SPI_ICONVERTICALSPACING /// public static double IconVerticalSpacing { get { return ConvertPixel(IconMetrics.iVertSpacing); } } ////// Maps to SPI_GETICONMETRICS -> iTitleWrap or SPI_GETICONTITLEWRAP /// public static bool IconTitleWrap { get { return IconMetrics.iTitleWrap != 0; } } #endregion #region Icon Keys ////// IconHorizontalSpacing System Resource Key /// public static ResourceKey IconHorizontalSpacingKey { get { if (_cacheIconHorizontalSpacing == null) { _cacheIconHorizontalSpacing = CreateInstance(SystemResourceKeyID.IconHorizontalSpacing); } return _cacheIconHorizontalSpacing; } } ////// IconVerticalSpacing System Resource Key /// public static ResourceKey IconVerticalSpacingKey { get { if (_cacheIconVerticalSpacing == null) { _cacheIconVerticalSpacing = CreateInstance(SystemResourceKeyID.IconVerticalSpacing); } return _cacheIconVerticalSpacing; } } ////// IconTitleWrap System Resource Key /// public static ResourceKey IconTitleWrapKey { get { if (_cacheIconTitleWrap == null) { _cacheIconTitleWrap = CreateInstance(SystemResourceKeyID.IconTitleWrap); } return _cacheIconTitleWrap; } } #endregion #region Input Parameters ////// Maps to SPI_GETKEYBOARDCUES /// /// ////// Demanding unmanaged code permission because calling an unsafe native method. /// SecurityCritical because it calls an unsafe native method. PublicOK because is demanding unmanaged code perm. /// PublicOK: This information is ok to give out /// public static bool KeyboardCues { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardCues]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDCUES, 0, ref _keyboardCues, 0)) { _cacheValid[(int)CacheSlot.KeyboardCues] = true; } else { throw new Win32Exception(); } } } return _keyboardCues; } } ////// Maps to SPI_GETKEYBOARDDELAY /// ////// PublicOK -- Determined safe: getting keyboard repeat delay /// Security Critical -- Calling UnsafeNativeMethods /// public static int KeyboardDelay { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardDelay]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDDELAY, 0, ref _keyboardDelay, 0)) { _cacheValid[(int)CacheSlot.KeyboardDelay] = true; } else { throw new Win32Exception(); } } } return _keyboardDelay; } } ////// Maps to SPI_GETKEYBOARDPREF /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool KeyboardPreference { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardPreference]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDPREF, 0, ref _keyboardPref, 0)) { _cacheValid[(int)CacheSlot.KeyboardPreference] = true; } else { throw new Win32Exception(); } } } return _keyboardPref; } } ////// Maps to SPI_GETKEYBOARDSPEED /// ////// PublicOK -- Determined safe: getting keyboard repeat-speed /// Security Critical -- Calling UnsafeNativeMethods /// public static int KeyboardSpeed { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardSpeed]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDSPEED, 0, ref _keyboardSpeed, 0)) { _cacheValid[(int)CacheSlot.KeyboardSpeed] = true; } else { throw new Win32Exception(); } } } return _keyboardSpeed; } } ////// Maps to SPI_GETSNAPTODEFBUTTON /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool SnapToDefaultButton { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SnapToDefaultButton]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSNAPTODEFBUTTON, 0, ref _snapToDefButton, 0)) { _cacheValid[(int)CacheSlot.SnapToDefaultButton] = true; } else { throw new Win32Exception(); } } } return _snapToDefButton; } } ////// Maps to SPI_GETWHEELSCROLLLINES /// ////// Get is PublicOK -- Determined safe: Geting the number of lines to scroll when the mouse wheel is rotated. \ /// Get is Critical -- Calling unsafe native methods. /// public static int WheelScrollLines { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WheelScrollLines]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWHEELSCROLLLINES, 0, ref _wheelScrollLines, 0)) { _cacheValid[(int)CacheSlot.WheelScrollLines] = true; } else { throw new Win32Exception(); } } } return _wheelScrollLines; } } ////// Maps to SPI_GETMOUSEHOVERTIME. /// public static TimeSpan MouseHoverTime { get { return TimeSpan.FromMilliseconds(MouseHoverTimeMilliseconds); } } ////// TreatAsSafe -- Determined safe: getting time mouse pointer has to stay in the hover rectangle for TrackMouseEvent to generate a WM_MOUSEHOVER message. /// Security Critical -- Calling UnsafeNativeMethods /// internal static int MouseHoverTimeMilliseconds { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverTime]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERTIME, 0, ref _mouseHoverTime, 0)) { _cacheValid[(int)CacheSlot.MouseHoverTime] = true; } else { throw new Win32Exception(); } } } return _mouseHoverTime; } } ////// Maps to SPI_GETMOUSEHOVERHEIGHT. /// ////// PublicOK -- Determined safe: gettingthe height, in pixels, of the rectangle within which the mouse pointer has to stay for TrackMouseEvent to generate a WM_MOUSEHOVER message /// Security Critical -- Calling UnsafeNativeMethods /// public static double MouseHoverHeight { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverHeight]) { int mouseHoverHeight = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERHEIGHT, 0, ref mouseHoverHeight, 0)) { _mouseHoverHeight = ConvertPixel(mouseHoverHeight); _cacheValid[(int)CacheSlot.MouseHoverHeight] = true; } else { throw new Win32Exception(); } } } return _mouseHoverHeight; } } ////// Maps to SPI_GETMOUSEHOVERWIDTH. /// /// ////// PublicOK -- Determined safe: getting the width, in pixels, of the rectangle within which the mouse pointer has to stay for TrackMouseEvent to generate a WM_MOUSEHOVER message /// Security Critical -- Calling UnsafeNativeMethods /// public static double MouseHoverWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverWidth]) { int mouseHoverWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERWIDTH, 0, ref mouseHoverWidth, 0)) { _mouseHoverWidth = ConvertPixel(mouseHoverWidth); _cacheValid[(int)CacheSlot.MouseHoverWidth] = true; } else { throw new Win32Exception(); } } } return _mouseHoverWidth; } } #endregion #region Input Keys ////// KeyboardCues System Resource Key /// public static ResourceKey KeyboardCuesKey { get { if (_cacheKeyboardCues == null) { _cacheKeyboardCues = CreateInstance(SystemResourceKeyID.KeyboardCues); } return _cacheKeyboardCues; } } ////// KeyboardDelay System Resource Key /// public static ResourceKey KeyboardDelayKey { get { if (_cacheKeyboardDelay == null) { _cacheKeyboardDelay = CreateInstance(SystemResourceKeyID.KeyboardDelay); } return _cacheKeyboardDelay; } } ////// KeyboardPreference System Resource Key /// public static ResourceKey KeyboardPreferenceKey { get { if (_cacheKeyboardPreference == null) { _cacheKeyboardPreference = CreateInstance(SystemResourceKeyID.KeyboardPreference); } return _cacheKeyboardPreference; } } ////// KeyboardSpeed System Resource Key /// public static ResourceKey KeyboardSpeedKey { get { if (_cacheKeyboardSpeed == null) { _cacheKeyboardSpeed = CreateInstance(SystemResourceKeyID.KeyboardSpeed); } return _cacheKeyboardSpeed; } } ////// SnapToDefaultButton System Resource Key /// public static ResourceKey SnapToDefaultButtonKey { get { if (_cacheSnapToDefaultButton == null) { _cacheSnapToDefaultButton = CreateInstance(SystemResourceKeyID.SnapToDefaultButton); } return _cacheSnapToDefaultButton; } } ////// WheelScrollLines System Resource Key /// public static ResourceKey WheelScrollLinesKey { get { if (_cacheWheelScrollLines == null) { _cacheWheelScrollLines = CreateInstance(SystemResourceKeyID.WheelScrollLines); } return _cacheWheelScrollLines; } } ////// MouseHoverTime System Resource Key /// public static ResourceKey MouseHoverTimeKey { get { if (_cacheMouseHoverTime == null) { _cacheMouseHoverTime = CreateInstance(SystemResourceKeyID.MouseHoverTime); } return _cacheMouseHoverTime; } } ////// MouseHoverHeight System Resource Key /// public static ResourceKey MouseHoverHeightKey { get { if (_cacheMouseHoverHeight == null) { _cacheMouseHoverHeight = CreateInstance(SystemResourceKeyID.MouseHoverHeight); } return _cacheMouseHoverHeight; } } ////// MouseHoverWidth System Resource Key /// public static ResourceKey MouseHoverWidthKey { get { if (_cacheMouseHoverWidth == null) { _cacheMouseHoverWidth = CreateInstance(SystemResourceKeyID.MouseHoverWidth); } return _cacheMouseHoverWidth; } } #endregion #region Menu Parameters ////// Maps to SPI_GETMENUDROPALIGNMENT /// ////// Demanding unmanaged code permission because calling an unsafe native method. /// Critical - get: it calls an unsafe native method /// PublicOK - get: it's safe to expose a menu drop alignment of a system. /// public static bool MenuDropAlignment { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuDropAlignment]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUDROPALIGNMENT, 0, ref _menuDropAlignment, 0)) { _cacheValid[(int)CacheSlot.MenuDropAlignment] = true; } else { throw new Win32Exception(); } } } return _menuDropAlignment; } } ////// Maps to SPI_GETMENUFADE /// ////// Critical - because it calls an unsafe native method /// PublicOK - ok to return menu fade data /// public static bool MenuFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUFADE, 0, ref _menuFade, 0)) { _cacheValid[(int)CacheSlot.MenuFade] = true; } else { throw new Win32Exception(); } } } return _menuFade; } } ////// Maps to SPI_GETMENUSHOWDELAY /// ////// Critical - calls a method that perfoms an elevation. /// PublicOK - considered ok to expose in partial trust. /// public static int MenuShowDelay { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuShowDelay]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUSHOWDELAY, 0, ref _menuShowDelay, 0)) { _cacheValid[(int)CacheSlot.MenuShowDelay] = true; } else { throw new Win32Exception(); } } } return _menuShowDelay; } } #endregion #region Menu Keys ////// MenuDropAlignment System Resource Key /// public static ResourceKey MenuDropAlignmentKey { get { if (_cacheMenuDropAlignment == null) { _cacheMenuDropAlignment = CreateInstance(SystemResourceKeyID.MenuDropAlignment); } return _cacheMenuDropAlignment; } } ////// MenuFade System Resource Key /// public static ResourceKey MenuFadeKey { get { if (_cacheMenuFade == null) { _cacheMenuFade = CreateInstance(SystemResourceKeyID.MenuFade); } return _cacheMenuFade; } } ////// MenuShowDelay System Resource Key /// public static ResourceKey MenuShowDelayKey { get { if (_cacheMenuShowDelay == null) { _cacheMenuShowDelay = CreateInstance(SystemResourceKeyID.MenuShowDelay); } return _cacheMenuShowDelay; } } #endregion #region UI Effects Parameters ////// Returns the system value of PopupAnimation for ComboBoxes. /// public static PopupAnimation ComboBoxPopupAnimation { get { if (ComboBoxAnimation) { return PopupAnimation.Slide; } return PopupAnimation.None; } } ////// Maps to SPI_GETCOMBOBOXANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool ComboBoxAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ComboBoxAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCOMBOBOXANIMATION, 0, ref _comboBoxAnimation, 0)) { _cacheValid[(int)CacheSlot.ComboBoxAnimation] = true; } else { throw new Win32Exception(); } } } return _comboBoxAnimation; } } ////// Maps to SPI_GETCLIENTAREAANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool ClientAreaAnimation { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ClientAreaAnimation]) { // This parameter is only available on Windows Versions >= 0x0600 (Vista) if (System.Environment.OSVersion.Version.Major >= 6) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCLIENTAREAANIMATION, 0, ref _clientAreaAnimation, 0)) { _cacheValid[(int)CacheSlot.ClientAreaAnimation] = true; } else { throw new Win32Exception(); } } else // Windows XP, assume value is true { _clientAreaAnimation = true; _cacheValid[(int)CacheSlot.ClientAreaAnimation] = true; } } } return _clientAreaAnimation; } } ////// Maps to SPI_GETCURSORSHADOW /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool CursorShadow { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorShadow]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCURSORSHADOW, 0, ref _cursorShadow, 0)) { _cacheValid[(int)CacheSlot.CursorShadow] = true; } else { throw new Win32Exception(); } } } return _cursorShadow; } } ////// Maps to SPI_GETGRADIENTCAPTIONS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool GradientCaptions { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.GradientCaptions]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETGRADIENTCAPTIONS, 0, ref _gradientCaptions, 0)) { _cacheValid[(int)CacheSlot.GradientCaptions] = true; } else { throw new Win32Exception(); } } } return _gradientCaptions; } } ////// Maps to SPI_GETHOTTRACKING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool HotTracking { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HotTracking]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHOTTRACKING, 0, ref _hotTracking, 0)) { _cacheValid[(int)CacheSlot.HotTracking] = true; } else { throw new Win32Exception(); } } } return _hotTracking; } } ////// Maps to SPI_GETLISTBOXSMOOTHSCROLLING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool ListBoxSmoothScrolling { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ListBoxSmoothScrolling]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETLISTBOXSMOOTHSCROLLING, 0, ref _listBoxSmoothScrolling, 0)) { _cacheValid[(int)CacheSlot.ListBoxSmoothScrolling] = true; } else { throw new Win32Exception(); } } } return _listBoxSmoothScrolling; } } ////// Returns the PopupAnimation value for Menus. /// public static PopupAnimation MenuPopupAnimation { get { if (MenuAnimation) { if (MenuFade) { return PopupAnimation.Fade; } else { return PopupAnimation.Scroll; } } return PopupAnimation.None; } } ////// Maps to SPI_GETMENUANIMATION /// ////// Critical - calls SystemParametersInfo /// PublicOK - net information returned is whether menu-animation is enabled. Considered safe. /// public static bool MenuAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUANIMATION, 0, ref _menuAnimation, 0)) { _cacheValid[(int)CacheSlot.MenuAnimation] = true; } else { throw new Win32Exception(); } } } return _menuAnimation; } } ////// Maps to SPI_GETSELECTIONFADE /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool SelectionFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SelectionFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSELECTIONFADE, 0, ref _selectionFade, 0)) { _cacheValid[(int)CacheSlot.SelectionFade] = true; } else { throw new Win32Exception(); } } } return _selectionFade; } } ////// Maps to SPI_GETSTYLUSHOTTRACKING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool StylusHotTracking { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.StylusHotTracking]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSTYLUSHOTTRACKING, 0, ref _stylusHotTracking, 0)) { _cacheValid[(int)CacheSlot.StylusHotTracking] = true; } else { throw new Win32Exception(); } } } return _stylusHotTracking; } } ////// Returns the PopupAnimation value for ToolTips. /// public static PopupAnimation ToolTipPopupAnimation { get { // Win32 ToolTips do not appear to scroll, only fade if (ToolTipAnimation && ToolTipFade) { return PopupAnimation.Fade; } return PopupAnimation.None; } } ////// Maps to SPI_GETTOOLTIPANIMATION /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static bool ToolTipAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ToolTipAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETTOOLTIPANIMATION, 0, ref _toolTipAnimation, 0)) { _cacheValid[(int)CacheSlot.ToolTipAnimation] = true; } else { throw new Win32Exception(); } } } return _toolTipAnimation; } } ////// Maps to SPI_GETTOOLTIPFADE /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static bool ToolTipFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ToolTipFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETTOOLTIPFADE, 0, ref _tooltipFade, 0)) { _cacheValid[(int)CacheSlot.ToolTipFade] = true; } else { throw new Win32Exception(); } } } return _tooltipFade; } } ////// Maps to SPI_GETUIEFFECTS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool UIEffects { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.UIEffects]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETUIEFFECTS, 0, ref _uiEffects, 0)) { _cacheValid[(int)CacheSlot.UIEffects] = true; } else { throw new Win32Exception(); } } } return _uiEffects; } } #endregion #region UI Effects Keys ////// ComboBoxAnimation System Resource Key /// public static ResourceKey ComboBoxAnimationKey { get { if (_cacheComboBoxAnimation == null) { _cacheComboBoxAnimation = CreateInstance(SystemResourceKeyID.ComboBoxAnimation); } return _cacheComboBoxAnimation; } } ////// ClientAreaAnimation System Resource Key /// public static ResourceKey ClientAreaAnimationKey { get { if (_cacheClientAreaAnimation == null) { _cacheClientAreaAnimation = CreateInstance(SystemResourceKeyID.ClientAreaAnimation); } return _cacheClientAreaAnimation; } } ////// CursorShadow System Resource Key /// public static ResourceKey CursorShadowKey { get { if (_cacheCursorShadow == null) { _cacheCursorShadow = CreateInstance(SystemResourceKeyID.CursorShadow); } return _cacheCursorShadow; } } ////// GradientCaptions System Resource Key /// public static ResourceKey GradientCaptionsKey { get { if (_cacheGradientCaptions == null) { _cacheGradientCaptions = CreateInstance(SystemResourceKeyID.GradientCaptions); } return _cacheGradientCaptions; } } ////// HotTracking System Resource Key /// public static ResourceKey HotTrackingKey { get { if (_cacheHotTracking == null) { _cacheHotTracking = CreateInstance(SystemResourceKeyID.HotTracking); } return _cacheHotTracking; } } ////// ListBoxSmoothScrolling System Resource Key /// public static ResourceKey ListBoxSmoothScrollingKey { get { if (_cacheListBoxSmoothScrolling == null) { _cacheListBoxSmoothScrolling = CreateInstance(SystemResourceKeyID.ListBoxSmoothScrolling); } return _cacheListBoxSmoothScrolling; } } ////// MenuAnimation System Resource Key /// public static ResourceKey MenuAnimationKey { get { if (_cacheMenuAnimation == null) { _cacheMenuAnimation = CreateInstance(SystemResourceKeyID.MenuAnimation); } return _cacheMenuAnimation; } } ////// SelectionFade System Resource Key /// public static ResourceKey SelectionFadeKey { get { if (_cacheSelectionFade == null) { _cacheSelectionFade = CreateInstance(SystemResourceKeyID.SelectionFade); } return _cacheSelectionFade; } } ////// StylusHotTracking System Resource Key /// public static ResourceKey StylusHotTrackingKey { get { if (_cacheStylusHotTracking == null) { _cacheStylusHotTracking = CreateInstance(SystemResourceKeyID.StylusHotTracking); } return _cacheStylusHotTracking; } } ////// ToolTipAnimation System Resource Key /// public static ResourceKey ToolTipAnimationKey { get { if (_cacheToolTipAnimation == null) { _cacheToolTipAnimation = CreateInstance(SystemResourceKeyID.ToolTipAnimation); } return _cacheToolTipAnimation; } } ////// ToolTipFade System Resource Key /// public static ResourceKey ToolTipFadeKey { get { if (_cacheToolTipFade == null) { _cacheToolTipFade = CreateInstance(SystemResourceKeyID.ToolTipFade); } return _cacheToolTipFade; } } ////// UIEffects System Resource Key /// public static ResourceKey UIEffectsKey { get { if (_cacheUIEffects == null) { _cacheUIEffects = CreateInstance(SystemResourceKeyID.UIEffects); } return _cacheUIEffects; } } ////// ComboBoxPopupAnimation System Resource Key /// public static ResourceKey ComboBoxPopupAnimationKey { get { if (_cacheComboBoxPopupAnimation == null) { _cacheComboBoxPopupAnimation = CreateInstance(SystemResourceKeyID.ComboBoxPopupAnimation); } return _cacheComboBoxPopupAnimation; } } ////// MenuPopupAnimation System Resource Key /// public static ResourceKey MenuPopupAnimationKey { get { if (_cacheMenuPopupAnimation == null) { _cacheMenuPopupAnimation = CreateInstance(SystemResourceKeyID.MenuPopupAnimation); } return _cacheMenuPopupAnimation; } } ////// ToolTipPopupAnimation System Resource Key /// public static ResourceKey ToolTipPopupAnimationKey { get { if (_cacheToolTipPopupAnimation == null) { _cacheToolTipPopupAnimation = CreateInstance(SystemResourceKeyID.ToolTipPopupAnimation); } return _cacheToolTipPopupAnimation; } } #endregion #region Window Parameters ////// Maps to SPI_GETANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool MinimizeAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizeAnimation]) { NativeMethods.ANIMATIONINFO animInfo = new NativeMethods.ANIMATIONINFO(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETANIMATION, animInfo.cbSize, animInfo, 0)) { _minAnimation = animInfo.iMinAnimate != 0; _cacheValid[(int)CacheSlot.MinimizeAnimation] = true; } else { throw new Win32Exception(); } } } return _minAnimation; } } ////// Maps to SPI_GETBORDER /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static int Border { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.Border]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETBORDER, 0, ref _border, 0)) { _cacheValid[(int)CacheSlot.Border] = true; } else { throw new Win32Exception(); } } } return _border; } } ////// Maps to SPI_GETCARETWIDTH /// ////// PublicOK -- Determined safe: getting width of caret /// Security Critical -- Calling UnsafeNativeMethods /// public static double CaretWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CaretWidth]) { int caretWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCARETWIDTH, 0, ref caretWidth, 0)) { _caretWidth = ConvertPixel(caretWidth); _cacheValid[(int)CacheSlot.CaretWidth] = true; } else { throw new Win32Exception(); } } } return _caretWidth; } } ////// Maps to SPI_GETDRAGFULLWINDOWS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool DragFullWindows { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.DragFullWindows]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDRAGFULLWINDOWS, 0, ref _dragFullWindows, 0)) { _cacheValid[(int)CacheSlot.DragFullWindows] = true; } else { throw new Win32Exception(); } } } return _dragFullWindows; } } ////// Maps to SPI_GETFOREGROUNDFLASHCOUNT /// ////// Get is PublicOK -- Getting # of times taskbar button will flash when rejecting a forecground switch request. /// Get is Security Critical -- Calling UnsafeNativeMethods /// public static int ForegroundFlashCount { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ForegroundFlashCount]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOREGROUNDFLASHCOUNT, 0, ref _foregroundFlashCount, 0)) { _cacheValid[(int)CacheSlot.ForegroundFlashCount] = true; } else { throw new Win32Exception(); } } } return _foregroundFlashCount; } } ////// Maps to SPI_GETNONCLIENTMETRICS /// ////// SecurityCritical because it calls an unsafe native method. /// SecurityTreatAsSafe as we think this would be ok to expose publically - and this is ok for consumption in partial trust. /// internal static NativeMethods.NONCLIENTMETRICS NonClientMetrics { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.NonClientMetrics]) { _ncm = new NativeMethods.NONCLIENTMETRICS(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, _ncm.cbSize, _ncm, 0)) { _cacheValid[(int)CacheSlot.NonClientMetrics] = true; } else { throw new Win32Exception(); } } } return _ncm; } } ////// From SPI_GETNONCLIENTMETRICS /// public static double BorderWidth { get { return ConvertPixel(NonClientMetrics.iBorderWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double ScrollWidth { get { return ConvertPixel(NonClientMetrics.iScrollWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double ScrollHeight { get { return ConvertPixel(NonClientMetrics.iScrollHeight); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double CaptionWidth { get { return ConvertPixel(NonClientMetrics.iCaptionWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double CaptionHeight { get { return ConvertPixel(NonClientMetrics.iCaptionHeight); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double SmallCaptionWidth { get { return ConvertPixel(NonClientMetrics.iSmCaptionWidth); } } ////// From SPI_NONCLIENTMETRICS /// public static double SmallCaptionHeight { get { return ConvertPixel(NonClientMetrics.iSmCaptionHeight); } } ////// From SPI_NONCLIENTMETRICS /// public static double MenuWidth { get { return ConvertPixel(NonClientMetrics.iMenuWidth); } } ////// From SPI_NONCLIENTMETRICS /// public static double MenuHeight { get { return ConvertPixel(NonClientMetrics.iMenuHeight); } } #endregion #region Window Parameters Keys ////// MinimizeAnimation System Resource Key /// public static ResourceKey MinimizeAnimationKey { get { if (_cacheMinimizeAnimation == null) { _cacheMinimizeAnimation = CreateInstance(SystemResourceKeyID.MinimizeAnimation); } return _cacheMinimizeAnimation; } } ////// Border System Resource Key /// public static ResourceKey BorderKey { get { if (_cacheBorder == null) { _cacheBorder = CreateInstance(SystemResourceKeyID.Border); } return _cacheBorder; } } ////// CaretWidth System Resource Key /// public static ResourceKey CaretWidthKey { get { if (_cacheCaretWidth == null) { _cacheCaretWidth = CreateInstance(SystemResourceKeyID.CaretWidth); } return _cacheCaretWidth; } } ////// ForegroundFlashCount System Resource Key /// public static ResourceKey ForegroundFlashCountKey { get { if (_cacheForegroundFlashCount == null) { _cacheForegroundFlashCount = CreateInstance(SystemResourceKeyID.ForegroundFlashCount); } return _cacheForegroundFlashCount; } } ////// DragFullWindows System Resource Key /// public static ResourceKey DragFullWindowsKey { get { if (_cacheDragFullWindows == null) { _cacheDragFullWindows = CreateInstance(SystemResourceKeyID.DragFullWindows); } return _cacheDragFullWindows; } } ////// BorderWidth System Resource Key /// public static ResourceKey BorderWidthKey { get { if (_cacheBorderWidth == null) { _cacheBorderWidth = CreateInstance(SystemResourceKeyID.BorderWidth); } return _cacheBorderWidth; } } ////// ScrollWidth System Resource Key /// public static ResourceKey ScrollWidthKey { get { if (_cacheScrollWidth == null) { _cacheScrollWidth = CreateInstance(SystemResourceKeyID.ScrollWidth); } return _cacheScrollWidth; } } ////// ScrollHeight System Resource Key /// public static ResourceKey ScrollHeightKey { get { if (_cacheScrollHeight == null) { _cacheScrollHeight = CreateInstance(SystemResourceKeyID.ScrollHeight); } return _cacheScrollHeight; } } ////// CaptionWidth System Resource Key /// public static ResourceKey CaptionWidthKey { get { if (_cacheCaptionWidth == null) { _cacheCaptionWidth = CreateInstance(SystemResourceKeyID.CaptionWidth); } return _cacheCaptionWidth; } } ////// CaptionHeight System Resource Key /// public static ResourceKey CaptionHeightKey { get { if (_cacheCaptionHeight == null) { _cacheCaptionHeight = CreateInstance(SystemResourceKeyID.CaptionHeight); } return _cacheCaptionHeight; } } ////// SmallCaptionWidth System Resource Key /// public static ResourceKey SmallCaptionWidthKey { get { if (_cacheSmallCaptionWidth == null) { _cacheSmallCaptionWidth = CreateInstance(SystemResourceKeyID.SmallCaptionWidth); } return _cacheSmallCaptionWidth; } } ////// MenuWidth System Resource Key /// public static ResourceKey MenuWidthKey { get { if (_cacheMenuWidth == null) { _cacheMenuWidth = CreateInstance(SystemResourceKeyID.MenuWidth); } return _cacheMenuWidth; } } ////// MenuHeight System Resource Key /// public static ResourceKey MenuHeightKey { get { if (_cacheMenuHeight == null) { _cacheMenuHeight = CreateInstance(SystemResourceKeyID.MenuHeight); } return _cacheMenuHeight; } } #endregion #region Metrics ////// Maps to SM_CXBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThinHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThinHorizontalBorderHeight]) { _thinHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXBORDER)); _cacheValid[(int)CacheSlot.ThinHorizontalBorderHeight] = true; } } return _thinHorizontalBorderHeight; } } ////// Maps to SM_CYBORDER /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThinVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThinVerticalBorderWidth]) { _thinVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYBORDER)); _cacheValid[(int)CacheSlot.ThinVerticalBorderWidth] = true; } } return _thinVerticalBorderWidth; } } ////// Maps to SM_CXCURSOR /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double CursorWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorWidth]) { _cursorWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXCURSOR)); _cacheValid[(int)CacheSlot.CursorWidth] = true; } } return _cursorWidth; } } ////// Maps to SM_CYCURSOR /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double CursorHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorHeight]) { _cursorHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYCURSOR)); _cacheValid[(int)CacheSlot.CursorHeight] = true; } } return _cursorHeight; } } ////// Maps to SM_CXEDGE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThickHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThickHorizontalBorderHeight]) { _thickHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXEDGE)); _cacheValid[(int)CacheSlot.ThickHorizontalBorderHeight] = true; } } return _thickHorizontalBorderHeight; } } ////// Maps to SM_CYEDGE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThickVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThickVerticalBorderWidth]) { _thickVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYEDGE)); _cacheValid[(int)CacheSlot.ThickVerticalBorderWidth] = true; } } return _thickVerticalBorderWidth; } } ////// Maps to SM_CXDRAG /// ////// Critical - calls into native code (GetSystemMetrics) /// PublicOK - Safe data to expose /// public static double MinimumHorizontalDragDistance { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumHorizontalDragDistance]) { _minimumHorizontalDragDistance = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXDRAG)); _cacheValid[(int)CacheSlot.MinimumHorizontalDragDistance] = true; } } return _minimumHorizontalDragDistance; } } ////// Maps to SM_CYDRAG /// ////// Critical - calls into native code (GetSystemMetrics) /// PublicOK - Safe data to expose /// public static double MinimumVerticalDragDistance { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumVerticalDragDistance]) { _minimumVerticalDragDistance = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYDRAG)); _cacheValid[(int)CacheSlot.MinimumVerticalDragDistance] = true; } } return _minimumVerticalDragDistance; } } ////// Maps to SM_CXFIXEDFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FixedFrameHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FixedFrameHorizontalBorderHeight]) { _fixedFrameHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFIXEDFRAME)); _cacheValid[(int)CacheSlot.FixedFrameHorizontalBorderHeight] = true; } } return _fixedFrameHorizontalBorderHeight; } } ////// Maps to SM_CYFIXEDFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FixedFrameVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FixedFrameVerticalBorderWidth]) { _fixedFrameVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFIXEDFRAME)); _cacheValid[(int)CacheSlot.FixedFrameVerticalBorderWidth] = true; } } return _fixedFrameVerticalBorderWidth; } } ////// Maps to SM_CXFOCUSBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusHorizontalBorderHeight]) { _focusHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFOCUSBORDER)); _cacheValid[(int)CacheSlot.FocusHorizontalBorderHeight] = true; } } return _focusHorizontalBorderHeight; } } ////// Maps to SM_CYFOCUSBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusVerticalBorderWidth]) { _focusVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFOCUSBORDER)); _cacheValid[(int)CacheSlot.FocusVerticalBorderWidth] = true; } } return _focusVerticalBorderWidth; } } ////// Maps to SM_CXFULLSCREEN /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double FullPrimaryScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FullPrimaryScreenWidth]) { _fullPrimaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFULLSCREEN)); _cacheValid[(int)CacheSlot.FullPrimaryScreenWidth] = true; } } return _fullPrimaryScreenWidth; } } ////// Maps to SM_CYFULLSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double FullPrimaryScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FullPrimaryScreenHeight]) { _fullPrimaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFULLSCREEN)); _cacheValid[(int)CacheSlot.FullPrimaryScreenHeight] = true; } } return _fullPrimaryScreenHeight; } } ////// Maps to SM_CXHSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarButtonWidth]) { _horizontalScrollBarButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXHSCROLL)); _cacheValid[(int)CacheSlot.HorizontalScrollBarButtonWidth] = true; } } return _horizontalScrollBarButtonWidth; } } ////// Maps to SM_CYHSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarHeight]) { _horizontalScrollBarHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYHSCROLL)); _cacheValid[(int)CacheSlot.HorizontalScrollBarHeight] = true; } } return _horizontalScrollBarHeight; } } ////// Maps to SM_CXHTHUMB /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarThumbWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarThumbWidth]) { _horizontalScrollBarThumbWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXHTHUMB)); _cacheValid[(int)CacheSlot.HorizontalScrollBarThumbWidth] = true; } } return _horizontalScrollBarThumbWidth; } } ////// Maps to SM_CXICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconWidth]) { _iconWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXICON)); _cacheValid[(int)CacheSlot.IconWidth] = true; } } return _iconWidth; } } ////// Maps to SM_CYICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconHeight]) { _iconHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYICON)); _cacheValid[(int)CacheSlot.IconHeight] = true; } } return _iconHeight; } } ////// Maps to SM_CXICONSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconGridWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconGridWidth]) { _iconGridWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXICONSPACING)); _cacheValid[(int)CacheSlot.IconGridWidth] = true; } } return _iconGridWidth; } } ////// Maps to SM_CYICONSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconGridHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconGridHeight]) { _iconGridHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYICONSPACING)); _cacheValid[(int)CacheSlot.IconGridHeight] = true; } } return _iconGridHeight; } } ////// Maps to SM_CXMAXIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximizedPrimaryScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximizedPrimaryScreenWidth]) { _maximizedPrimaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMAXIMIZED)); _cacheValid[(int)CacheSlot.MaximizedPrimaryScreenWidth] = true; } } return _maximizedPrimaryScreenWidth; } } ////// Maps to SM_CYMAXIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximizedPrimaryScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximizedPrimaryScreenHeight]) { _maximizedPrimaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMAXIMIZED)); _cacheValid[(int)CacheSlot.MaximizedPrimaryScreenHeight] = true; } } return _maximizedPrimaryScreenHeight; } } ////// Maps to SM_CXMAXTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximumWindowTrackWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximumWindowTrackWidth]) { _maximumWindowTrackWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMAXTRACK)); _cacheValid[(int)CacheSlot.MaximumWindowTrackWidth] = true; } } return _maximumWindowTrackWidth; } } ////// Maps to SM_CYMAXTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximumWindowTrackHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximumWindowTrackHeight]) { _maximumWindowTrackHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMAXTRACK)); _cacheValid[(int)CacheSlot.MaximumWindowTrackHeight] = true; } } return _maximumWindowTrackHeight; } } ////// Maps to SM_CXMENUCHECK /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuCheckmarkWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuCheckmarkWidth]) { _menuCheckmarkWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMENUCHECK)); _cacheValid[(int)CacheSlot.MenuCheckmarkWidth] = true; } } return _menuCheckmarkWidth; } } ////// Maps to SM_CYMENUCHECK /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuCheckmarkHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuCheckmarkHeight]) { _menuCheckmarkHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENUCHECK)); _cacheValid[(int)CacheSlot.MenuCheckmarkHeight] = true; } } return _menuCheckmarkHeight; } } ////// Maps to SM_CXMENUSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuButtonWidth]) { _menuButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMENUSIZE)); _cacheValid[(int)CacheSlot.MenuButtonWidth] = true; } } return _menuButtonWidth; } } ////// Maps to SM_CYMENUSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuButtonHeight]) { _menuButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENUSIZE)); _cacheValid[(int)CacheSlot.MenuButtonHeight] = true; } } return _menuButtonHeight; } } ////// Maps to SM_CXMIN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowWidth]) { _minimumWindowWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMIN)); _cacheValid[(int)CacheSlot.MinimumWindowWidth] = true; } } return _minimumWindowWidth; } } ////// Maps to SM_CYMIN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowHeight]) { _minimumWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMIN)); _cacheValid[(int)CacheSlot.MinimumWindowHeight] = true; } } return _minimumWindowHeight; } } ////// Maps to SM_CXMINIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK -- There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedWindowWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedWindowWidth]) { _minimizedWindowWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINIMIZED)); _cacheValid[(int)CacheSlot.MinimizedWindowWidth] = true; } } return _minimizedWindowWidth; } } ////// Maps to SM_CYMINIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedWindowHeight]) { _minimizedWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINIMIZED)); _cacheValid[(int)CacheSlot.MinimizedWindowHeight] = true; } } return _minimizedWindowHeight; } } ////// Maps to SM_CXMINSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedGridWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedGridWidth]) { _minimizedGridWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINSPACING)); _cacheValid[(int)CacheSlot.MinimizedGridWidth] = true; } } return _minimizedGridWidth; } } ////// Maps to SM_CYMINSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedGridHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedGridHeight]) { _minimizedGridHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINSPACING)); _cacheValid[(int)CacheSlot.MinimizedGridHeight] = true; } } return _minimizedGridHeight; } } ////// Maps to SM_CXMINTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exist a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowTrackWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowTrackWidth]) { _minimumWindowTrackWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINTRACK)); _cacheValid[(int)CacheSlot.MinimumWindowTrackWidth] = true; } } return _minimumWindowTrackWidth; } } ////// Maps to SM_CYMINTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowTrackHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowTrackHeight]) { _minimumWindowTrackHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINTRACK)); _cacheValid[(int)CacheSlot.MinimumWindowTrackHeight] = true; } } return _minimumWindowTrackHeight; } } ////// Maps to SM_CXSCREEN /// ////// PublicOK -- This is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// public static double PrimaryScreenWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PrimaryScreenWidth]) { _primaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSCREEN)); _cacheValid[(int)CacheSlot.PrimaryScreenWidth] = true; } } return _primaryScreenWidth; } } ////// Maps to SM_CYSCREEN /// ////// PublicOK --This is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// public static double PrimaryScreenHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PrimaryScreenHeight]) { _primaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSCREEN)); _cacheValid[(int)CacheSlot.PrimaryScreenHeight] = true; } } return _primaryScreenHeight; } } ////// Maps to SM_CXSIZE /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionButtonWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionButtonWidth]) { _windowCaptionButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSIZE)); _cacheValid[(int)CacheSlot.WindowCaptionButtonWidth] = true; } } return _windowCaptionButtonWidth; } } ////// Maps to SM_CYSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionButtonHeight]) { _windowCaptionButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSIZE)); _cacheValid[(int)CacheSlot.WindowCaptionButtonHeight] = true; } } return _windowCaptionButtonHeight; } } ////// Maps to SM_CXSIZEFRAME /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ResizeFrameHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ResizeFrameHorizontalBorderHeight]) { _resizeFrameHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSIZEFRAME)); _cacheValid[(int)CacheSlot.ResizeFrameHorizontalBorderHeight] = true; } } return _resizeFrameHorizontalBorderHeight; } } ////// Maps to SM_CYSIZEFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ResizeFrameVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ResizeFrameVerticalBorderWidth]) { _resizeFrameVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSIZEFRAME)); _cacheValid[(int)CacheSlot.ResizeFrameVerticalBorderWidth] = true; } } return _resizeFrameVerticalBorderWidth; } } ////// Maps to SM_CXSMICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallIconWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallIconWidth]) { _smallIconWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSMICON)); _cacheValid[(int)CacheSlot.SmallIconWidth] = true; } } return _smallIconWidth; } } ////// Maps to SM_CYSMICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallIconHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallIconHeight]) { _smallIconHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSMICON)); _cacheValid[(int)CacheSlot.SmallIconHeight] = true; } } return _smallIconHeight; } } ////// Maps to SM_CXSMSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallWindowCaptionButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallWindowCaptionButtonWidth]) { _smallWindowCaptionButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSMSIZE)); _cacheValid[(int)CacheSlot.SmallWindowCaptionButtonWidth] = true; } } return _smallWindowCaptionButtonWidth; } } ////// Maps to SM_CYSMSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallWindowCaptionButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallWindowCaptionButtonHeight]) { _smallWindowCaptionButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSMSIZE)); _cacheValid[(int)CacheSlot.SmallWindowCaptionButtonHeight] = true; } } return _smallWindowCaptionButtonHeight; } } ////// Maps to SM_CXVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenWidth]) { _virtualScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenWidth] = true; } } return _virtualScreenWidth; } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenHeight]) { _virtualScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenHeight] = true; } } return _virtualScreenHeight; } } ////// Maps to SM_CXVSCROLL /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarWidth]) { _verticalScrollBarWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXVSCROLL)); _cacheValid[(int)CacheSlot.VerticalScrollBarWidth] = true; } } return _verticalScrollBarWidth; } } ////// Maps to SM_CYVSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarButtonHeight]) { _verticalScrollBarButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVSCROLL)); _cacheValid[(int)CacheSlot.VerticalScrollBarButtonHeight] = true; } } return _verticalScrollBarButtonHeight; } } ////// Maps to SM_CYCAPTION /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionHeight]) { _windowCaptionHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYCAPTION)); _cacheValid[(int)CacheSlot.WindowCaptionHeight] = true; } } return _windowCaptionHeight; } } ////// Maps to SM_CYKANJIWINDOW /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double KanjiWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KanjiWindowHeight]) { _kanjiWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYKANJIWINDOW)); _cacheValid[(int)CacheSlot.KanjiWindowHeight] = true; } } return _kanjiWindowHeight; } } ////// Maps to SM_CYMENU /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuBarHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuBarHeight]) { _menuBarHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENU)); _cacheValid[(int)CacheSlot.MenuBarHeight] = true; } } return _menuBarHeight; } } ////// Maps to SM_CYVTHUMB /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarThumbHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarThumbHeight]) { _verticalScrollBarThumbHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVTHUMB)); _cacheValid[(int)CacheSlot.VerticalScrollBarThumbHeight] = true; } } return _verticalScrollBarThumbHeight; } } ////// Maps to SM_IMMENABLED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand in this code. /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsImmEnabled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsImmEnabled]) { _isImmEnabled = UnsafeNativeMethods.GetSystemMetrics(SM.IMMENABLED) != 0; _cacheValid[(int)CacheSlot.IsImmEnabled] = true; } } return _isImmEnabled; } } ////// Maps to SM_MEDIACENTER /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMediaCenter { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMediaCenter]) { _isMediaCenter = UnsafeNativeMethods.GetSystemMetrics(SM.MEDIACENTER) != 0; _cacheValid[(int)CacheSlot.IsMediaCenter] = true; } } return _isMediaCenter; } } ////// Maps to SM_MENUDROPALIGNMENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMenuDropRightAligned { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMenuDropRightAligned]) { _isMenuDropRightAligned = UnsafeNativeMethods.GetSystemMetrics(SM.MENUDROPALIGNMENT) != 0; _cacheValid[(int)CacheSlot.IsMenuDropRightAligned] = true; } } return _isMenuDropRightAligned; } } ////// Maps to SM_MIDEASTENABLED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMiddleEastEnabled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMiddleEastEnabled]) { _isMiddleEastEnabled = UnsafeNativeMethods.GetSystemMetrics(SM.MIDEASTENABLED) != 0; _cacheValid[(int)CacheSlot.IsMiddleEastEnabled] = true; } } return _isMiddleEastEnabled; } } ////// Maps to SM_MOUSEPRESENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMousePresent { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMousePresent]) { _isMousePresent = UnsafeNativeMethods.GetSystemMetrics(SM.MOUSEPRESENT) != 0; _cacheValid[(int)CacheSlot.IsMousePresent] = true; } } return _isMousePresent; } } ////// Maps to SM_MOUSEWHEELPRESENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMouseWheelPresent { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMouseWheelPresent]) { _isMouseWheelPresent = UnsafeNativeMethods.GetSystemMetrics(SM.MOUSEWHEELPRESENT) != 0; _cacheValid[(int)CacheSlot.IsMouseWheelPresent] = true; } } return _isMouseWheelPresent; } } ////// Maps to SM_PENWINDOWS /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Deemed as unsafe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsPenWindows { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsPenWindows]) { _isPenWindows = UnsafeNativeMethods.GetSystemMetrics(SM.PENWINDOWS) != 0; _cacheValid[(int)CacheSlot.IsPenWindows] = true; } } return _isPenWindows; } } ////// Maps to SM_REMOTECONTROL /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsRemotelyControlled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsRemotelyControlled]) { _isRemotelyControlled = UnsafeNativeMethods.GetSystemMetrics(SM.REMOTECONTROL) != 0; _cacheValid[(int)CacheSlot.IsRemotelyControlled] = true; } } return _isRemotelyControlled; } } ////// Maps to SM_REMOTESESSION /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demand Unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsRemoteSession { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsRemoteSession]) { _isRemoteSession = UnsafeNativeMethods.GetSystemMetrics(SM.REMOTESESSION) != 0; _cacheValid[(int)CacheSlot.IsRemoteSession] = true; } } return _isRemoteSession; } } ////// Maps to SM_SHOWSOUNDS /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demand Unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool ShowSounds { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ShowSounds]) { _showSounds = UnsafeNativeMethods.GetSystemMetrics(SM.SHOWSOUNDS) != 0; _cacheValid[(int)CacheSlot.ShowSounds] = true; } } return _showSounds; } } ////// Maps to SM_SLOWMACHINE /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsSlowMachine { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsSlowMachine]) { _isSlowMachine = UnsafeNativeMethods.GetSystemMetrics(SM.SLOWMACHINE) != 0; _cacheValid[(int)CacheSlot.IsSlowMachine] = true; } } return _isSlowMachine; } } ////// Maps to SM_SWAPBUTTON /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool SwapButtons { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SwapButtons]) { _swapButtons = UnsafeNativeMethods.GetSystemMetrics(SM.SWAPBUTTON) != 0; _cacheValid[(int)CacheSlot.SwapButtons] = true; } } return _swapButtons; } } ////// Maps to SM_TABLETPC /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK -- Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsTabletPC { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsTabletPC]) { _isTabletPC = UnsafeNativeMethods.GetSystemMetrics(SM.TABLETPC) != 0; _cacheValid[(int)CacheSlot.IsTabletPC] = true; } } return _isTabletPC; } } ////// Maps to SM_XVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenLeft { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenLeft]) { _virtualScreenLeft = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.XVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenLeft] = true; } } return _virtualScreenLeft; } } ////// Maps to SM_YVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenTop { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenTop]) { _virtualScreenTop = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.YVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenTop] = true; } } return _virtualScreenTop; } } #endregion #region Metrics Keys ////// ThinHorizontalBorderHeight System Resource Key /// public static ResourceKey ThinHorizontalBorderHeightKey { get { if (_cacheThinHorizontalBorderHeight == null) { _cacheThinHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ThinHorizontalBorderHeight); } return _cacheThinHorizontalBorderHeight; } } ////// ThinVerticalBorderWidth System Resource Key /// public static ResourceKey ThinVerticalBorderWidthKey { get { if (_cacheThinVerticalBorderWidth == null) { _cacheThinVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ThinVerticalBorderWidth); } return _cacheThinVerticalBorderWidth; } } ////// CursorWidth System Resource Key /// public static ResourceKey CursorWidthKey { get { if (_cacheCursorWidth == null) { _cacheCursorWidth = CreateInstance(SystemResourceKeyID.CursorWidth); } return _cacheCursorWidth; } } ////// CursorHeight System Resource Key /// public static ResourceKey CursorHeightKey { get { if (_cacheCursorHeight == null) { _cacheCursorHeight = CreateInstance(SystemResourceKeyID.CursorHeight); } return _cacheCursorHeight; } } ////// ThickHorizontalBorderHeight System Resource Key /// public static ResourceKey ThickHorizontalBorderHeightKey { get { if (_cacheThickHorizontalBorderHeight == null) { _cacheThickHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ThickHorizontalBorderHeight); } return _cacheThickHorizontalBorderHeight; } } ////// ThickVerticalBorderWidth System Resource Key /// public static ResourceKey ThickVerticalBorderWidthKey { get { if (_cacheThickVerticalBorderWidth == null) { _cacheThickVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ThickVerticalBorderWidth); } return _cacheThickVerticalBorderWidth; } } ////// FixedFrameHorizontalBorderHeight System Resource Key /// public static ResourceKey FixedFrameHorizontalBorderHeightKey { get { if (_cacheFixedFrameHorizontalBorderHeight == null) { _cacheFixedFrameHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.FixedFrameHorizontalBorderHeight); } return _cacheFixedFrameHorizontalBorderHeight; } } ////// FixedFrameVerticalBorderWidth System Resource Key /// public static ResourceKey FixedFrameVerticalBorderWidthKey { get { if (_cacheFixedFrameVerticalBorderWidth == null) { _cacheFixedFrameVerticalBorderWidth = CreateInstance(SystemResourceKeyID.FixedFrameVerticalBorderWidth); } return _cacheFixedFrameVerticalBorderWidth; } } ////// FocusHorizontalBorderHeight System Resource Key /// public static ResourceKey FocusHorizontalBorderHeightKey { get { if (_cacheFocusHorizontalBorderHeight == null) { _cacheFocusHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.FocusHorizontalBorderHeight); } return _cacheFocusHorizontalBorderHeight; } } ////// FocusVerticalBorderWidth System Resource Key /// public static ResourceKey FocusVerticalBorderWidthKey { get { if (_cacheFocusVerticalBorderWidth == null) { _cacheFocusVerticalBorderWidth = CreateInstance(SystemResourceKeyID.FocusVerticalBorderWidth); } return _cacheFocusVerticalBorderWidth; } } ////// FullPrimaryScreenWidth System Resource Key /// public static ResourceKey FullPrimaryScreenWidthKey { get { if (_cacheFullPrimaryScreenWidth == null) { _cacheFullPrimaryScreenWidth = CreateInstance(SystemResourceKeyID.FullPrimaryScreenWidth); } return _cacheFullPrimaryScreenWidth; } } ////// FullPrimaryScreenHeight System Resource Key /// public static ResourceKey FullPrimaryScreenHeightKey { get { if (_cacheFullPrimaryScreenHeight == null) { _cacheFullPrimaryScreenHeight = CreateInstance(SystemResourceKeyID.FullPrimaryScreenHeight); } return _cacheFullPrimaryScreenHeight; } } ////// HorizontalScrollBarButtonWidth System Resource Key /// public static ResourceKey HorizontalScrollBarButtonWidthKey { get { if (_cacheHorizontalScrollBarButtonWidth == null) { _cacheHorizontalScrollBarButtonWidth = CreateInstance(SystemResourceKeyID.HorizontalScrollBarButtonWidth); } return _cacheHorizontalScrollBarButtonWidth; } } ////// HorizontalScrollBarHeight System Resource Key /// public static ResourceKey HorizontalScrollBarHeightKey { get { if (_cacheHorizontalScrollBarHeight == null) { _cacheHorizontalScrollBarHeight = CreateInstance(SystemResourceKeyID.HorizontalScrollBarHeight); } return _cacheHorizontalScrollBarHeight; } } ////// HorizontalScrollBarThumbWidth System Resource Key /// public static ResourceKey HorizontalScrollBarThumbWidthKey { get { if (_cacheHorizontalScrollBarThumbWidth == null) { _cacheHorizontalScrollBarThumbWidth = CreateInstance(SystemResourceKeyID.HorizontalScrollBarThumbWidth); } return _cacheHorizontalScrollBarThumbWidth; } } ////// IconWidth System Resource Key /// public static ResourceKey IconWidthKey { get { if (_cacheIconWidth == null) { _cacheIconWidth = CreateInstance(SystemResourceKeyID.IconWidth); } return _cacheIconWidth; } } ////// IconHeight System Resource Key /// public static ResourceKey IconHeightKey { get { if (_cacheIconHeight == null) { _cacheIconHeight = CreateInstance(SystemResourceKeyID.IconHeight); } return _cacheIconHeight; } } ////// IconGridWidth System Resource Key /// public static ResourceKey IconGridWidthKey { get { if (_cacheIconGridWidth == null) { _cacheIconGridWidth = CreateInstance(SystemResourceKeyID.IconGridWidth); } return _cacheIconGridWidth; } } ////// IconGridHeight System Resource Key /// public static ResourceKey IconGridHeightKey { get { if (_cacheIconGridHeight == null) { _cacheIconGridHeight = CreateInstance(SystemResourceKeyID.IconGridHeight); } return _cacheIconGridHeight; } } ////// MaximizedPrimaryScreenWidth System Resource Key /// public static ResourceKey MaximizedPrimaryScreenWidthKey { get { if (_cacheMaximizedPrimaryScreenWidth == null) { _cacheMaximizedPrimaryScreenWidth = CreateInstance(SystemResourceKeyID.MaximizedPrimaryScreenWidth); } return _cacheMaximizedPrimaryScreenWidth; } } ////// MaximizedPrimaryScreenHeight System Resource Key /// public static ResourceKey MaximizedPrimaryScreenHeightKey { get { if (_cacheMaximizedPrimaryScreenHeight == null) { _cacheMaximizedPrimaryScreenHeight = CreateInstance(SystemResourceKeyID.MaximizedPrimaryScreenHeight); } return _cacheMaximizedPrimaryScreenHeight; } } ////// MaximumWindowTrackWidth System Resource Key /// public static ResourceKey MaximumWindowTrackWidthKey { get { if (_cacheMaximumWindowTrackWidth == null) { _cacheMaximumWindowTrackWidth = CreateInstance(SystemResourceKeyID.MaximumWindowTrackWidth); } return _cacheMaximumWindowTrackWidth; } } ////// MaximumWindowTrackHeight System Resource Key /// public static ResourceKey MaximumWindowTrackHeightKey { get { if (_cacheMaximumWindowTrackHeight == null) { _cacheMaximumWindowTrackHeight = CreateInstance(SystemResourceKeyID.MaximumWindowTrackHeight); } return _cacheMaximumWindowTrackHeight; } } ////// MenuCheckmarkWidth System Resource Key /// public static ResourceKey MenuCheckmarkWidthKey { get { if (_cacheMenuCheckmarkWidth == null) { _cacheMenuCheckmarkWidth = CreateInstance(SystemResourceKeyID.MenuCheckmarkWidth); } return _cacheMenuCheckmarkWidth; } } ////// MenuCheckmarkHeight System Resource Key /// public static ResourceKey MenuCheckmarkHeightKey { get { if (_cacheMenuCheckmarkHeight == null) { _cacheMenuCheckmarkHeight = CreateInstance(SystemResourceKeyID.MenuCheckmarkHeight); } return _cacheMenuCheckmarkHeight; } } ////// MenuButtonWidth System Resource Key /// public static ResourceKey MenuButtonWidthKey { get { if (_cacheMenuButtonWidth == null) { _cacheMenuButtonWidth = CreateInstance(SystemResourceKeyID.MenuButtonWidth); } return _cacheMenuButtonWidth; } } ////// MenuButtonHeight System Resource Key /// public static ResourceKey MenuButtonHeightKey { get { if (_cacheMenuButtonHeight == null) { _cacheMenuButtonHeight = CreateInstance(SystemResourceKeyID.MenuButtonHeight); } return _cacheMenuButtonHeight; } } ////// MinimumWindowWidth System Resource Key /// public static ResourceKey MinimumWindowWidthKey { get { if (_cacheMinimumWindowWidth == null) { _cacheMinimumWindowWidth = CreateInstance(SystemResourceKeyID.MinimumWindowWidth); } return _cacheMinimumWindowWidth; } } ////// MinimumWindowHeight System Resource Key /// public static ResourceKey MinimumWindowHeightKey { get { if (_cacheMinimumWindowHeight == null) { _cacheMinimumWindowHeight = CreateInstance(SystemResourceKeyID.MinimumWindowHeight); } return _cacheMinimumWindowHeight; } } ////// MinimizedWindowWidth System Resource Key /// public static ResourceKey MinimizedWindowWidthKey { get { if (_cacheMinimizedWindowWidth == null) { _cacheMinimizedWindowWidth = CreateInstance(SystemResourceKeyID.MinimizedWindowWidth); } return _cacheMinimizedWindowWidth; } } ////// MinimizedWindowHeight System Resource Key /// public static ResourceKey MinimizedWindowHeightKey { get { if (_cacheMinimizedWindowHeight == null) { _cacheMinimizedWindowHeight = CreateInstance(SystemResourceKeyID.MinimizedWindowHeight); } return _cacheMinimizedWindowHeight; } } ////// MinimizedGridWidth System Resource Key /// public static ResourceKey MinimizedGridWidthKey { get { if (_cacheMinimizedGridWidth == null) { _cacheMinimizedGridWidth = CreateInstance(SystemResourceKeyID.MinimizedGridWidth); } return _cacheMinimizedGridWidth; } } ////// MinimizedGridHeight System Resource Key /// public static ResourceKey MinimizedGridHeightKey { get { if (_cacheMinimizedGridHeight == null) { _cacheMinimizedGridHeight = CreateInstance(SystemResourceKeyID.MinimizedGridHeight); } return _cacheMinimizedGridHeight; } } ////// MinimumWindowTrackWidth System Resource Key /// public static ResourceKey MinimumWindowTrackWidthKey { get { if (_cacheMinimumWindowTrackWidth == null) { _cacheMinimumWindowTrackWidth = CreateInstance(SystemResourceKeyID.MinimumWindowTrackWidth); } return _cacheMinimumWindowTrackWidth; } } ////// MinimumWindowTrackHeight System Resource Key /// public static ResourceKey MinimumWindowTrackHeightKey { get { if (_cacheMinimumWindowTrackHeight == null) { _cacheMinimumWindowTrackHeight = CreateInstance(SystemResourceKeyID.MinimumWindowTrackHeight); } return _cacheMinimumWindowTrackHeight; } } ////// PrimaryScreenWidth System Resource Key /// public static ResourceKey PrimaryScreenWidthKey { get { if (_cachePrimaryScreenWidth == null) { _cachePrimaryScreenWidth = CreateInstance(SystemResourceKeyID.PrimaryScreenWidth); } return _cachePrimaryScreenWidth; } } ////// PrimaryScreenHeight System Resource Key /// public static ResourceKey PrimaryScreenHeightKey { get { if (_cachePrimaryScreenHeight == null) { _cachePrimaryScreenHeight = CreateInstance(SystemResourceKeyID.PrimaryScreenHeight); } return _cachePrimaryScreenHeight; } } ////// WindowCaptionButtonWidth System Resource Key /// public static ResourceKey WindowCaptionButtonWidthKey { get { if (_cacheWindowCaptionButtonWidth == null) { _cacheWindowCaptionButtonWidth = CreateInstance(SystemResourceKeyID.WindowCaptionButtonWidth); } return _cacheWindowCaptionButtonWidth; } } ////// WindowCaptionButtonHeight System Resource Key /// public static ResourceKey WindowCaptionButtonHeightKey { get { if (_cacheWindowCaptionButtonHeight == null) { _cacheWindowCaptionButtonHeight = CreateInstance(SystemResourceKeyID.WindowCaptionButtonHeight); } return _cacheWindowCaptionButtonHeight; } } ////// ResizeFrameHorizontalBorderHeight System Resource Key /// public static ResourceKey ResizeFrameHorizontalBorderHeightKey { get { if (_cacheResizeFrameHorizontalBorderHeight == null) { _cacheResizeFrameHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ResizeFrameHorizontalBorderHeight); } return _cacheResizeFrameHorizontalBorderHeight; } } ////// ResizeFrameVerticalBorderWidth System Resource Key /// public static ResourceKey ResizeFrameVerticalBorderWidthKey { get { if (_cacheResizeFrameVerticalBorderWidth == null) { _cacheResizeFrameVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ResizeFrameVerticalBorderWidth); } return _cacheResizeFrameVerticalBorderWidth; } } ////// SmallIconWidth System Resource Key /// public static ResourceKey SmallIconWidthKey { get { if (_cacheSmallIconWidth == null) { _cacheSmallIconWidth = CreateInstance(SystemResourceKeyID.SmallIconWidth); } return _cacheSmallIconWidth; } } ////// SmallIconHeight System Resource Key /// public static ResourceKey SmallIconHeightKey { get { if (_cacheSmallIconHeight == null) { _cacheSmallIconHeight = CreateInstance(SystemResourceKeyID.SmallIconHeight); } return _cacheSmallIconHeight; } } ////// SmallWindowCaptionButtonWidth System Resource Key /// public static ResourceKey SmallWindowCaptionButtonWidthKey { get { if (_cacheSmallWindowCaptionButtonWidth == null) { _cacheSmallWindowCaptionButtonWidth = CreateInstance(SystemResourceKeyID.SmallWindowCaptionButtonWidth); } return _cacheSmallWindowCaptionButtonWidth; } } ////// SmallWindowCaptionButtonHeight System Resource Key /// public static ResourceKey SmallWindowCaptionButtonHeightKey { get { if (_cacheSmallWindowCaptionButtonHeight == null) { _cacheSmallWindowCaptionButtonHeight = CreateInstance(SystemResourceKeyID.SmallWindowCaptionButtonHeight); } return _cacheSmallWindowCaptionButtonHeight; } } ////// VirtualScreenWidth System Resource Key /// public static ResourceKey VirtualScreenWidthKey { get { if (_cacheVirtualScreenWidth == null) { _cacheVirtualScreenWidth = CreateInstance(SystemResourceKeyID.VirtualScreenWidth); } return _cacheVirtualScreenWidth; } } ////// VirtualScreenHeight System Resource Key /// public static ResourceKey VirtualScreenHeightKey { get { if (_cacheVirtualScreenHeight == null) { _cacheVirtualScreenHeight = CreateInstance(SystemResourceKeyID.VirtualScreenHeight); } return _cacheVirtualScreenHeight; } } ////// VerticalScrollBarWidth System Resource Key /// public static ResourceKey VerticalScrollBarWidthKey { get { if (_cacheVerticalScrollBarWidth == null) { _cacheVerticalScrollBarWidth = CreateInstance(SystemResourceKeyID.VerticalScrollBarWidth); } return _cacheVerticalScrollBarWidth; } } ////// VerticalScrollBarButtonHeight System Resource Key /// public static ResourceKey VerticalScrollBarButtonHeightKey { get { if (_cacheVerticalScrollBarButtonHeight == null) { _cacheVerticalScrollBarButtonHeight = CreateInstance(SystemResourceKeyID.VerticalScrollBarButtonHeight); } return _cacheVerticalScrollBarButtonHeight; } } ////// WindowCaptionHeight System Resource Key /// public static ResourceKey WindowCaptionHeightKey { get { if (_cacheWindowCaptionHeight == null) { _cacheWindowCaptionHeight = CreateInstance(SystemResourceKeyID.WindowCaptionHeight); } return _cacheWindowCaptionHeight; } } ////// KanjiWindowHeight System Resource Key /// public static ResourceKey KanjiWindowHeightKey { get { if (_cacheKanjiWindowHeight == null) { _cacheKanjiWindowHeight = CreateInstance(SystemResourceKeyID.KanjiWindowHeight); } return _cacheKanjiWindowHeight; } } ////// MenuBarHeight System Resource Key /// public static ResourceKey MenuBarHeightKey { get { if (_cacheMenuBarHeight == null) { _cacheMenuBarHeight = CreateInstance(SystemResourceKeyID.MenuBarHeight); } return _cacheMenuBarHeight; } } ////// SmallCaptionHeight System Resource Key /// public static ResourceKey SmallCaptionHeightKey { get { if (_cacheSmallCaptionHeight == null) { _cacheSmallCaptionHeight = CreateInstance(SystemResourceKeyID.SmallCaptionHeight); } return _cacheSmallCaptionHeight; } } ////// VerticalScrollBarThumbHeight System Resource Key /// public static ResourceKey VerticalScrollBarThumbHeightKey { get { if (_cacheVerticalScrollBarThumbHeight == null) { _cacheVerticalScrollBarThumbHeight = CreateInstance(SystemResourceKeyID.VerticalScrollBarThumbHeight); } return _cacheVerticalScrollBarThumbHeight; } } ////// IsImmEnabled System Resource Key /// public static ResourceKey IsImmEnabledKey { get { if (_cacheIsImmEnabled == null) { _cacheIsImmEnabled = CreateInstance(SystemResourceKeyID.IsImmEnabled); } return _cacheIsImmEnabled; } } ////// IsMediaCenter System Resource Key /// public static ResourceKey IsMediaCenterKey { get { if (_cacheIsMediaCenter == null) { _cacheIsMediaCenter = CreateInstance(SystemResourceKeyID.IsMediaCenter); } return _cacheIsMediaCenter; } } ////// IsMenuDropRightAligned System Resource Key /// public static ResourceKey IsMenuDropRightAlignedKey { get { if (_cacheIsMenuDropRightAligned == null) { _cacheIsMenuDropRightAligned = CreateInstance(SystemResourceKeyID.IsMenuDropRightAligned); } return _cacheIsMenuDropRightAligned; } } ////// IsMiddleEastEnabled System Resource Key /// public static ResourceKey IsMiddleEastEnabledKey { get { if (_cacheIsMiddleEastEnabled == null) { _cacheIsMiddleEastEnabled = CreateInstance(SystemResourceKeyID.IsMiddleEastEnabled); } return _cacheIsMiddleEastEnabled; } } ////// IsMousePresent System Resource Key /// public static ResourceKey IsMousePresentKey { get { if (_cacheIsMousePresent == null) { _cacheIsMousePresent = CreateInstance(SystemResourceKeyID.IsMousePresent); } return _cacheIsMousePresent; } } ////// IsMouseWheelPresent System Resource Key /// public static ResourceKey IsMouseWheelPresentKey { get { if (_cacheIsMouseWheelPresent == null) { _cacheIsMouseWheelPresent = CreateInstance(SystemResourceKeyID.IsMouseWheelPresent); } return _cacheIsMouseWheelPresent; } } ////// IsPenWindows System Resource Key /// public static ResourceKey IsPenWindowsKey { get { if (_cacheIsPenWindows == null) { _cacheIsPenWindows = CreateInstance(SystemResourceKeyID.IsPenWindows); } return _cacheIsPenWindows; } } ////// IsRemotelyControlled System Resource Key /// public static ResourceKey IsRemotelyControlledKey { get { if (_cacheIsRemotelyControlled == null) { _cacheIsRemotelyControlled = CreateInstance(SystemResourceKeyID.IsRemotelyControlled); } return _cacheIsRemotelyControlled; } } ////// IsRemoteSession System Resource Key /// public static ResourceKey IsRemoteSessionKey { get { if (_cacheIsRemoteSession == null) { _cacheIsRemoteSession = CreateInstance(SystemResourceKeyID.IsRemoteSession); } return _cacheIsRemoteSession; } } ////// ShowSounds System Resource Key /// public static ResourceKey ShowSoundsKey { get { if (_cacheShowSounds == null) { _cacheShowSounds = CreateInstance(SystemResourceKeyID.ShowSounds); } return _cacheShowSounds; } } ////// IsSlowMachine System Resource Key /// public static ResourceKey IsSlowMachineKey { get { if (_cacheIsSlowMachine == null) { _cacheIsSlowMachine = CreateInstance(SystemResourceKeyID.IsSlowMachine); } return _cacheIsSlowMachine; } } ////// SwapButtons System Resource Key /// public static ResourceKey SwapButtonsKey { get { if (_cacheSwapButtons == null) { _cacheSwapButtons = CreateInstance(SystemResourceKeyID.SwapButtons); } return _cacheSwapButtons; } } ////// IsTabletPC System Resource Key /// public static ResourceKey IsTabletPCKey { get { if (_cacheIsTabletPC == null) { _cacheIsTabletPC = CreateInstance(SystemResourceKeyID.IsTabletPC); } return _cacheIsTabletPC; } } ////// VirtualScreenLeft System Resource Key /// public static ResourceKey VirtualScreenLeftKey { get { if (_cacheVirtualScreenLeft == null) { _cacheVirtualScreenLeft = CreateInstance(SystemResourceKeyID.VirtualScreenLeft); } return _cacheVirtualScreenLeft; } } ////// VirtualScreenTop System Resource Key /// public static ResourceKey VirtualScreenTopKey { get { if (_cacheVirtualScreenTop == null) { _cacheVirtualScreenTop = CreateInstance(SystemResourceKeyID.VirtualScreenTop); } return _cacheVirtualScreenTop; } } #endregion #region Theme Style Keys ////// Resource Key for the FocusVisualStyle /// public static ResourceKey FocusVisualStyleKey { get { if (_cacheFocusVisualStyle == null) { _cacheFocusVisualStyle = new SystemThemeKey(SystemResourceKeyID.FocusVisualStyle); } return _cacheFocusVisualStyle; } } ////// Resource Key for the browser window style /// ///public static ResourceKey NavigationChromeStyleKey { get { if (_cacheNavigationChromeStyle == null) { _cacheNavigationChromeStyle = new SystemThemeKey(SystemResourceKeyID.NavigationChromeStyle); } return _cacheNavigationChromeStyle; } } /// /// Resource Key for the down level browser window style /// ///public static ResourceKey NavigationChromeDownLevelStyleKey { get { if (_cacheNavigationChromeDownLevelStyle == null) { _cacheNavigationChromeDownLevelStyle = new SystemThemeKey(SystemResourceKeyID.NavigationChromeDownLevelStyle); } return _cacheNavigationChromeDownLevelStyle; } } #endregion #region Power Parameters /// /// Indicates current Power Status /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static PowerLineStatus PowerLineStatus { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PowerLineStatus]) { NativeMethods.SYSTEM_POWER_STATUS status = new NativeMethods.SYSTEM_POWER_STATUS(); if (UnsafeNativeMethods.GetSystemPowerStatus(ref status)) { _powerLineStatus = (PowerLineStatus)status.ACLineStatus; _cacheValid[(int)CacheSlot.PowerLineStatus] = true; } else { throw new Win32Exception(); } } } return _powerLineStatus; } } #endregion #region PowerKeys ////// Resource Key for the PowerLineStatus property /// ///public static ResourceKey PowerLineStatusKey { get { if (_cachePowerLineStatus == null) { _cachePowerLineStatus = CreateInstance(SystemResourceKeyID.PowerLineStatus); } return _cachePowerLineStatus; } } #endregion #pragma warning restore 6523 #pragma warning restore 6503 #region Cache and Implementation internal static void InvalidateCache() { // Invalidate all Parameters int[] param = { NativeMethods.SPI_SETFOCUSBORDERWIDTH, NativeMethods.SPI_SETFOCUSBORDERHEIGHT, NativeMethods.SPI_SETHIGHCONTRAST, NativeMethods.SPI_SETMOUSEVANISH, NativeMethods.SPI_SETDROPSHADOW, NativeMethods.SPI_SETFLATMENU, NativeMethods.SPI_SETWORKAREA, NativeMethods.SPI_SETICONMETRICS, NativeMethods.SPI_SETKEYBOARDCUES, NativeMethods.SPI_SETKEYBOARDDELAY, NativeMethods.SPI_SETKEYBOARDPREF, NativeMethods.SPI_SETKEYBOARDSPEED, NativeMethods.SPI_SETSNAPTODEFBUTTON, NativeMethods.SPI_SETWHEELSCROLLLINES, NativeMethods.SPI_SETMOUSEHOVERTIME, NativeMethods.SPI_SETMOUSEHOVERHEIGHT, NativeMethods.SPI_SETMOUSEHOVERWIDTH, NativeMethods.SPI_SETMENUDROPALIGNMENT, NativeMethods.SPI_SETMENUFADE, NativeMethods.SPI_SETMENUSHOWDELAY, NativeMethods.SPI_SETCOMBOBOXANIMATION, NativeMethods.SPI_SETCLIENTAREAANIMATION, NativeMethods.SPI_SETCURSORSHADOW, NativeMethods.SPI_SETGRADIENTCAPTIONS, NativeMethods.SPI_SETHOTTRACKING, NativeMethods.SPI_SETLISTBOXSMOOTHSCROLLING, NativeMethods.SPI_SETMENUANIMATION, NativeMethods.SPI_SETSELECTIONFADE, NativeMethods.SPI_SETSTYLUSHOTTRACKING, NativeMethods.SPI_SETTOOLTIPANIMATION, NativeMethods.SPI_SETTOOLTIPFADE, NativeMethods.SPI_SETUIEFFECTS, NativeMethods.SPI_SETANIMATION, NativeMethods.SPI_SETCARETWIDTH, NativeMethods.SPI_SETFOREGROUNDFLASHCOUNT, NativeMethods.SPI_SETDRAGFULLWINDOWS, NativeMethods.SPI_SETBORDER, NativeMethods.SPI_SETNONCLIENTMETRICS, NativeMethods.SPI_SETDRAGWIDTH, NativeMethods.SPI_SETDRAGHEIGHT, NativeMethods.SPI_SETPENWINDOWS, NativeMethods.SPI_SETSHOWSOUNDS, NativeMethods.SPI_SETMOUSEBUTTONSWAP}; for (int i = 0; i < param.Length; i++) { InvalidateCache(param[i]); } } internal static bool InvalidateDeviceDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMousePresent)) changed |= _isMousePresent != IsMousePresent; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMouseWheelPresent)) changed |= _isMousePresent != IsMousePresent; return changed; } internal static bool InvalidateDisplayDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WorkAreaInternal)) { NativeMethods.RECT oldRect = _workAreaInternal; NativeMethods.RECT newRect = WorkAreaInternal; changed |= oldRect.left != newRect.left; changed |= oldRect.top != newRect.top; changed |= oldRect.right != newRect.right; changed |= oldRect.bottom != newRect.bottom; } if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WorkArea)) changed |= _workArea != WorkArea; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FullPrimaryScreenWidth)) changed |= _fullPrimaryScreenWidth != FullPrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FullPrimaryScreenHeight)) changed |= _fullPrimaryScreenHeight != FullPrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximizedPrimaryScreenWidth)) changed |= _maximizedPrimaryScreenWidth != MaximizedPrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximizedPrimaryScreenHeight)) changed |= _maximizedPrimaryScreenHeight != MaximizedPrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PrimaryScreenWidth)) changed |= _primaryScreenWidth != PrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PrimaryScreenHeight)) changed |= _primaryScreenHeight != PrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenWidth)) changed |= _virtualScreenWidth != VirtualScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenHeight)) changed |= _virtualScreenHeight != VirtualScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenLeft)) changed |= _virtualScreenLeft != VirtualScreenLeft; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenTop)) changed |= _virtualScreenTop != VirtualScreenTop; return changed; } internal static bool InvalidatePowerDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PowerLineStatus)) changed |= _powerLineStatus != PowerLineStatus; return changed; } internal static bool InvalidateCache(int param) { // FxCop: Hashtable of callbacks would not be performant, using switch instead switch (param) { case NativeMethods.SPI_SETFOCUSBORDERWIDTH: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusBorderWidth); // Invalidate SystemMetrics if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusHorizontalBorderHeight)) changed |= _focusHorizontalBorderHeight != FocusHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusVerticalBorderWidth)) changed |= _focusVerticalBorderWidth != FocusVerticalBorderWidth; return changed; } case NativeMethods.SPI_SETFOCUSBORDERHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusBorderHeight); case NativeMethods.SPI_SETHIGHCONTRAST: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HighContrast); case NativeMethods.SPI_SETMOUSEVANISH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseVanish); case NativeMethods.SPI_SETDROPSHADOW: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.DropShadow); case NativeMethods.SPI_SETFLATMENU: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FlatMenu); case NativeMethods.SPI_SETWORKAREA: return InvalidateDisplayDependentCache(); case NativeMethods.SPI_SETICONMETRICS: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconMetrics); if (changed) { SystemFonts.InvalidateIconMetrics(); } if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconWidth)) changed |= _iconWidth != IconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconHeight)) changed |= _iconHeight != IconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridWidth)) changed |= _iconGridWidth != IconGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridHeight)) changed |= _iconGridHeight != IconGridHeight; return changed; } case NativeMethods.SPI_SETKEYBOARDCUES: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardCues); case NativeMethods.SPI_SETKEYBOARDDELAY: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardDelay); case NativeMethods.SPI_SETKEYBOARDPREF: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardPreference); case NativeMethods.SPI_SETKEYBOARDSPEED: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardSpeed); case NativeMethods.SPI_SETSNAPTODEFBUTTON: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SnapToDefaultButton); case NativeMethods.SPI_SETWHEELSCROLLLINES: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WheelScrollLines); case NativeMethods.SPI_SETMOUSEHOVERTIME: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverTime); case NativeMethods.SPI_SETMOUSEHOVERHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverHeight); case NativeMethods.SPI_SETMOUSEHOVERWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverWidth); case NativeMethods.SPI_SETMENUDROPALIGNMENT: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuDropAlignment); if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMenuDropRightAligned)) changed |= _isMenuDropRightAligned != IsMenuDropRightAligned; return changed; } case NativeMethods.SPI_SETMENUFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuFade); case NativeMethods.SPI_SETMENUSHOWDELAY: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuShowDelay); case NativeMethods.SPI_SETCOMBOBOXANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ComboBoxAnimation); case NativeMethods.SPI_SETCLIENTAREAANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ClientAreaAnimation); case NativeMethods.SPI_SETCURSORSHADOW: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorShadow); case NativeMethods.SPI_SETGRADIENTCAPTIONS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.GradientCaptions); case NativeMethods.SPI_SETHOTTRACKING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HotTracking); case NativeMethods.SPI_SETLISTBOXSMOOTHSCROLLING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ListBoxSmoothScrolling); case NativeMethods.SPI_SETMENUANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuAnimation); case NativeMethods.SPI_SETSELECTIONFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SelectionFade); case NativeMethods.SPI_SETSTYLUSHOTTRACKING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.StylusHotTracking); case NativeMethods.SPI_SETTOOLTIPANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ToolTipAnimation); case NativeMethods.SPI_SETTOOLTIPFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ToolTipFade); case NativeMethods.SPI_SETUIEFFECTS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.UIEffects); case NativeMethods.SPI_SETANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizeAnimation); case NativeMethods.SPI_SETCARETWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CaretWidth); case NativeMethods.SPI_SETFOREGROUNDFLASHCOUNT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ForegroundFlashCount); case NativeMethods.SPI_SETDRAGFULLWINDOWS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.DragFullWindows); case NativeMethods.SPI_SETBORDER: case NativeMethods.SPI_SETNONCLIENTMETRICS: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.NonClientMetrics); if (changed) { SystemFonts.InvalidateNonClientMetrics(); } changed |= SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.Border); // Invalidate SystemMetrics if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThinHorizontalBorderHeight)) changed |= _thinHorizontalBorderHeight != ThinHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThinVerticalBorderWidth)) changed |= _thinVerticalBorderWidth != ThinVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorWidth)) changed |= _cursorWidth != CursorWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorHeight)) changed |= _cursorHeight != CursorHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThickHorizontalBorderHeight)) changed |= _thickHorizontalBorderHeight != ThickHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThickVerticalBorderWidth)) changed |= _thickVerticalBorderWidth != ThickVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FixedFrameHorizontalBorderHeight)) changed |= _fixedFrameHorizontalBorderHeight != FixedFrameHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FixedFrameVerticalBorderWidth)) changed |= _fixedFrameVerticalBorderWidth != FixedFrameVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarButtonWidth)) changed |= _horizontalScrollBarButtonWidth != HorizontalScrollBarButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarHeight)) changed |= _horizontalScrollBarHeight != HorizontalScrollBarHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarThumbWidth)) changed |= _horizontalScrollBarThumbWidth != HorizontalScrollBarThumbWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconWidth)) changed |= _iconWidth != IconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconHeight)) changed |= _iconHeight != IconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridWidth)) changed |= _iconGridWidth != IconGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridHeight)) changed |= _iconGridHeight != IconGridHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximumWindowTrackWidth)) changed |= _maximumWindowTrackWidth != MaximumWindowTrackWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximumWindowTrackHeight)) changed |= _maximumWindowTrackHeight != MaximumWindowTrackHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuCheckmarkWidth)) changed |= _menuCheckmarkWidth != MenuCheckmarkWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuCheckmarkHeight)) changed |= _menuCheckmarkHeight != MenuCheckmarkHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuButtonWidth)) changed |= _menuButtonWidth != MenuButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuButtonHeight)) changed |= _menuButtonHeight != MenuButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowWidth)) changed |= _minimumWindowWidth != MinimumWindowWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowHeight)) changed |= _minimumWindowHeight != MinimumWindowHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedWindowWidth)) changed |= _minimizedWindowWidth != MinimizedWindowWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedWindowHeight)) changed |= _minimizedWindowHeight != MinimizedWindowHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedGridWidth)) changed |= _minimizedGridWidth != MinimizedGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedGridHeight)) changed |= _minimizedGridHeight != MinimizedGridHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowTrackWidth)) changed |= _minimumWindowTrackWidth != MinimumWindowTrackWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowTrackHeight)) changed |= _minimumWindowTrackHeight != MinimumWindowTrackHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionButtonWidth)) changed |= _windowCaptionButtonWidth != WindowCaptionButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionButtonHeight)) changed |= _windowCaptionButtonHeight != WindowCaptionButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ResizeFrameHorizontalBorderHeight)) changed |= _resizeFrameHorizontalBorderHeight != ResizeFrameHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ResizeFrameVerticalBorderWidth)) changed |= _resizeFrameVerticalBorderWidth != ResizeFrameVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallIconWidth)) changed |= _smallIconWidth != SmallIconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallIconHeight)) changed |= _smallIconHeight != SmallIconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallWindowCaptionButtonWidth)) changed |= _smallWindowCaptionButtonWidth != SmallWindowCaptionButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallWindowCaptionButtonHeight)) changed |= _smallWindowCaptionButtonHeight != SmallWindowCaptionButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarWidth)) changed |= _verticalScrollBarWidth != VerticalScrollBarWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarButtonHeight)) changed |= _verticalScrollBarButtonHeight != VerticalScrollBarButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionHeight)) changed |= _windowCaptionHeight != WindowCaptionHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuBarHeight)) changed |= _menuBarHeight != MenuBarHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarThumbHeight)) changed |= _verticalScrollBarThumbHeight != VerticalScrollBarThumbHeight; changed |= InvalidateDisplayDependentCache(); return changed; } case NativeMethods.SPI_SETDRAGWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumHorizontalDragDistance); case NativeMethods.SPI_SETDRAGHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumVerticalDragDistance); case NativeMethods.SPI_SETPENWINDOWS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsPenWindows); case NativeMethods.SPI_SETSHOWSOUNDS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ShowSounds); case NativeMethods.SPI_SETMOUSEBUTTONSWAP: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SwapButtons); } return false; } internal static int Dpi { get { return MS.Internal.FontCache.Util.Dpi; } } /// /// Critical as this accesses Native methods. /// TreatAsSafe - it would be ok to expose this information - DPI in partial trust /// internal static int DpiX { [SecurityCritical, SecurityTreatAsSafe] get { if (_setDpiX) { lock (_cacheValid) { if (_setDpiX) { _setDpiX = false; HandleRef desktopWnd = new HandleRef(null, IntPtr.Zero); // Win32Exception will get the Win32 error code so we don't have to #pragma warning disable 6523 IntPtr dc = UnsafeNativeMethods.GetDC(desktopWnd); // Detecting error case from unmanaged call, required by PREsharp to throw a Win32Exception #pragma warning disable 6503 if (dc == IntPtr.Zero) { throw new Win32Exception(); } #pragma warning restore 6503 #pragma warning restore 6523 try { _dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(null, dc), NativeMethods.LOGPIXELSX); _cacheValid[(int)CacheSlot.DpiX] = true; } finally { UnsafeNativeMethods.ReleaseDC(desktopWnd, new HandleRef(null, dc)); } } } } return _dpiX; } } internal static double ConvertPixel(int pixel) { int dpi = Dpi; if (dpi != 0) { return (double)pixel * 96 / dpi; } return pixel; } private enum CacheSlot : int { DpiX, FocusBorderWidth, FocusBorderHeight, HighContrast, MouseVanish, DropShadow, FlatMenu, WorkAreaInternal, WorkArea, IconMetrics, KeyboardCues, KeyboardDelay, KeyboardPreference, KeyboardSpeed, SnapToDefaultButton, WheelScrollLines, MouseHoverTime, MouseHoverHeight, MouseHoverWidth, MenuDropAlignment, MenuFade, MenuShowDelay, ComboBoxAnimation, ClientAreaAnimation, CursorShadow, GradientCaptions, HotTracking, ListBoxSmoothScrolling, MenuAnimation, SelectionFade, StylusHotTracking, ToolTipAnimation, ToolTipFade, UIEffects, MinimizeAnimation, Border, CaretWidth, ForegroundFlashCount, DragFullWindows, NonClientMetrics, ThinHorizontalBorderHeight, ThinVerticalBorderWidth, CursorWidth, CursorHeight, ThickHorizontalBorderHeight, ThickVerticalBorderWidth, MinimumHorizontalDragDistance, MinimumVerticalDragDistance, FixedFrameHorizontalBorderHeight, FixedFrameVerticalBorderWidth, FocusHorizontalBorderHeight, FocusVerticalBorderWidth, FullPrimaryScreenWidth, FullPrimaryScreenHeight, HorizontalScrollBarButtonWidth, HorizontalScrollBarHeight, HorizontalScrollBarThumbWidth, IconWidth, IconHeight, IconGridWidth, IconGridHeight, MaximizedPrimaryScreenWidth, MaximizedPrimaryScreenHeight, MaximumWindowTrackWidth, MaximumWindowTrackHeight, MenuCheckmarkWidth, MenuCheckmarkHeight, MenuButtonWidth, MenuButtonHeight, MinimumWindowWidth, MinimumWindowHeight, MinimizedWindowWidth, MinimizedWindowHeight, MinimizedGridWidth, MinimizedGridHeight, MinimumWindowTrackWidth, MinimumWindowTrackHeight, PrimaryScreenWidth, PrimaryScreenHeight, WindowCaptionButtonWidth, WindowCaptionButtonHeight, ResizeFrameHorizontalBorderHeight, ResizeFrameVerticalBorderWidth, SmallIconWidth, SmallIconHeight, SmallWindowCaptionButtonWidth, SmallWindowCaptionButtonHeight, VirtualScreenWidth, VirtualScreenHeight, VerticalScrollBarWidth, VerticalScrollBarButtonHeight, WindowCaptionHeight, KanjiWindowHeight, MenuBarHeight, VerticalScrollBarThumbHeight, IsImmEnabled, IsMediaCenter, IsMenuDropRightAligned, IsMiddleEastEnabled, IsMousePresent, IsMouseWheelPresent, IsPenWindows, IsRemotelyControlled, IsRemoteSession, ShowSounds, IsSlowMachine, SwapButtons, IsTabletPC, VirtualScreenLeft, VirtualScreenTop, PowerLineStatus, NumSlots } private static BitArray _cacheValid = new BitArray((int)CacheSlot.NumSlots); private static int _dpiX; private static bool _setDpiX = true; private static double _focusBorderWidth; private static double _focusBorderHeight; private static bool _highContrast; private static bool _mouseVanish; private static bool _dropShadow; private static bool _flatMenu; private static NativeMethods.RECT _workAreaInternal; private static Rect _workArea; private static NativeMethods.ICONMETRICS _iconMetrics; private static bool _keyboardCues; private static int _keyboardDelay; private static bool _keyboardPref; private static int _keyboardSpeed; private static bool _snapToDefButton; private static int _wheelScrollLines; private static int _mouseHoverTime; private static double _mouseHoverHeight; private static double _mouseHoverWidth; private static bool _menuDropAlignment; private static bool _menuFade; private static int _menuShowDelay; private static bool _comboBoxAnimation; private static bool _clientAreaAnimation; private static bool _cursorShadow; private static bool _gradientCaptions; private static bool _hotTracking; private static bool _listBoxSmoothScrolling; private static bool _menuAnimation; private static bool _selectionFade; private static bool _stylusHotTracking; private static bool _toolTipAnimation; private static bool _tooltipFade; private static bool _uiEffects; private static bool _minAnimation; private static int _border; private static double _caretWidth; private static bool _dragFullWindows; private static int _foregroundFlashCount; private static NativeMethods.NONCLIENTMETRICS _ncm; private static double _thinHorizontalBorderHeight; private static double _thinVerticalBorderWidth; private static double _cursorWidth; private static double _cursorHeight; private static double _thickHorizontalBorderHeight; private static double _thickVerticalBorderWidth; private static double _minimumHorizontalDragDistance; private static double _minimumVerticalDragDistance; private static double _fixedFrameHorizontalBorderHeight; private static double _fixedFrameVerticalBorderWidth; private static double _focusHorizontalBorderHeight; private static double _focusVerticalBorderWidth; private static double _fullPrimaryScreenHeight; private static double _fullPrimaryScreenWidth; private static double _horizontalScrollBarHeight; private static double _horizontalScrollBarButtonWidth; private static double _horizontalScrollBarThumbWidth; private static double _iconWidth; private static double _iconHeight; private static double _iconGridWidth; private static double _iconGridHeight; private static double _maximizedPrimaryScreenWidth; private static double _maximizedPrimaryScreenHeight; private static double _maximumWindowTrackWidth; private static double _maximumWindowTrackHeight; private static double _menuCheckmarkWidth; private static double _menuCheckmarkHeight; private static double _menuButtonWidth; private static double _menuButtonHeight; private static double _minimumWindowWidth; private static double _minimumWindowHeight; private static double _minimizedWindowWidth; private static double _minimizedWindowHeight; private static double _minimizedGridWidth; private static double _minimizedGridHeight; private static double _minimumWindowTrackWidth; private static double _minimumWindowTrackHeight; private static double _primaryScreenWidth; private static double _primaryScreenHeight; private static double _windowCaptionButtonWidth; private static double _windowCaptionButtonHeight; private static double _resizeFrameHorizontalBorderHeight; private static double _resizeFrameVerticalBorderWidth; private static double _smallIconWidth; private static double _smallIconHeight; private static double _smallWindowCaptionButtonWidth; private static double _smallWindowCaptionButtonHeight; private static double _virtualScreenWidth; private static double _virtualScreenHeight; private static double _verticalScrollBarWidth; private static double _verticalScrollBarButtonHeight; private static double _windowCaptionHeight; private static double _kanjiWindowHeight; private static double _menuBarHeight; private static double _verticalScrollBarThumbHeight; private static bool _isImmEnabled; private static bool _isMediaCenter; private static bool _isMenuDropRightAligned; private static bool _isMiddleEastEnabled; private static bool _isMousePresent; private static bool _isMouseWheelPresent; private static bool _isPenWindows; private static bool _isRemotelyControlled; private static bool _isRemoteSession; private static bool _showSounds; private static bool _isSlowMachine; private static bool _swapButtons; private static bool _isTabletPC; private static double _virtualScreenLeft; private static double _virtualScreenTop; private static PowerLineStatus _powerLineStatus; private static SystemResourceKey _cacheThinHorizontalBorderHeight; private static SystemResourceKey _cacheThinVerticalBorderWidth; private static SystemResourceKey _cacheCursorWidth; private static SystemResourceKey _cacheCursorHeight; private static SystemResourceKey _cacheThickHorizontalBorderHeight; private static SystemResourceKey _cacheThickVerticalBorderWidth; private static SystemResourceKey _cacheFixedFrameHorizontalBorderHeight; private static SystemResourceKey _cacheFixedFrameVerticalBorderWidth; private static SystemResourceKey _cacheFocusHorizontalBorderHeight; private static SystemResourceKey _cacheFocusVerticalBorderWidth; private static SystemResourceKey _cacheFullPrimaryScreenWidth; private static SystemResourceKey _cacheFullPrimaryScreenHeight; private static SystemResourceKey _cacheHorizontalScrollBarButtonWidth; private static SystemResourceKey _cacheHorizontalScrollBarHeight; private static SystemResourceKey _cacheHorizontalScrollBarThumbWidth; private static SystemResourceKey _cacheIconWidth; private static SystemResourceKey _cacheIconHeight; private static SystemResourceKey _cacheIconGridWidth; private static SystemResourceKey _cacheIconGridHeight; private static SystemResourceKey _cacheMaximizedPrimaryScreenWidth; private static SystemResourceKey _cacheMaximizedPrimaryScreenHeight; private static SystemResourceKey _cacheMaximumWindowTrackWidth; private static SystemResourceKey _cacheMaximumWindowTrackHeight; private static SystemResourceKey _cacheMenuCheckmarkWidth; private static SystemResourceKey _cacheMenuCheckmarkHeight; private static SystemResourceKey _cacheMenuButtonWidth; private static SystemResourceKey _cacheMenuButtonHeight; private static SystemResourceKey _cacheMinimumWindowWidth; private static SystemResourceKey _cacheMinimumWindowHeight; private static SystemResourceKey _cacheMinimizedWindowWidth; private static SystemResourceKey _cacheMinimizedWindowHeight; private static SystemResourceKey _cacheMinimizedGridWidth; private static SystemResourceKey _cacheMinimizedGridHeight; private static SystemResourceKey _cacheMinimumWindowTrackWidth; private static SystemResourceKey _cacheMinimumWindowTrackHeight; private static SystemResourceKey _cachePrimaryScreenWidth; private static SystemResourceKey _cachePrimaryScreenHeight; private static SystemResourceKey _cacheWindowCaptionButtonWidth; private static SystemResourceKey _cacheWindowCaptionButtonHeight; private static SystemResourceKey _cacheResizeFrameHorizontalBorderHeight; private static SystemResourceKey _cacheResizeFrameVerticalBorderWidth; private static SystemResourceKey _cacheSmallIconWidth; private static SystemResourceKey _cacheSmallIconHeight; private static SystemResourceKey _cacheSmallWindowCaptionButtonWidth; private static SystemResourceKey _cacheSmallWindowCaptionButtonHeight; private static SystemResourceKey _cacheVirtualScreenWidth; private static SystemResourceKey _cacheVirtualScreenHeight; private static SystemResourceKey _cacheVerticalScrollBarWidth; private static SystemResourceKey _cacheVerticalScrollBarButtonHeight; private static SystemResourceKey _cacheWindowCaptionHeight; private static SystemResourceKey _cacheKanjiWindowHeight; private static SystemResourceKey _cacheMenuBarHeight; private static SystemResourceKey _cacheSmallCaptionHeight; private static SystemResourceKey _cacheVerticalScrollBarThumbHeight; private static SystemResourceKey _cacheIsImmEnabled; private static SystemResourceKey _cacheIsMediaCenter; private static SystemResourceKey _cacheIsMenuDropRightAligned; private static SystemResourceKey _cacheIsMiddleEastEnabled; private static SystemResourceKey _cacheIsMousePresent; private static SystemResourceKey _cacheIsMouseWheelPresent; private static SystemResourceKey _cacheIsPenWindows; private static SystemResourceKey _cacheIsRemotelyControlled; private static SystemResourceKey _cacheIsRemoteSession; private static SystemResourceKey _cacheShowSounds; private static SystemResourceKey _cacheIsSlowMachine; private static SystemResourceKey _cacheSwapButtons; private static SystemResourceKey _cacheIsTabletPC; private static SystemResourceKey _cacheVirtualScreenLeft; private static SystemResourceKey _cacheVirtualScreenTop; private static SystemResourceKey _cacheFocusBorderWidth; private static SystemResourceKey _cacheFocusBorderHeight; private static SystemResourceKey _cacheHighContrast; private static SystemResourceKey _cacheDropShadow; private static SystemResourceKey _cacheFlatMenu; private static SystemResourceKey _cacheWorkArea; private static SystemResourceKey _cacheIconHorizontalSpacing; private static SystemResourceKey _cacheIconVerticalSpacing; private static SystemResourceKey _cacheIconTitleWrap; private static SystemResourceKey _cacheKeyboardCues; private static SystemResourceKey _cacheKeyboardDelay; private static SystemResourceKey _cacheKeyboardPreference; private static SystemResourceKey _cacheKeyboardSpeed; private static SystemResourceKey _cacheSnapToDefaultButton; private static SystemResourceKey _cacheWheelScrollLines; private static SystemResourceKey _cacheMouseHoverTime; private static SystemResourceKey _cacheMouseHoverHeight; private static SystemResourceKey _cacheMouseHoverWidth; private static SystemResourceKey _cacheMenuDropAlignment; private static SystemResourceKey _cacheMenuFade; private static SystemResourceKey _cacheMenuShowDelay; private static SystemResourceKey _cacheComboBoxAnimation; private static SystemResourceKey _cacheClientAreaAnimation; private static SystemResourceKey _cacheCursorShadow; private static SystemResourceKey _cacheGradientCaptions; private static SystemResourceKey _cacheHotTracking; private static SystemResourceKey _cacheListBoxSmoothScrolling; private static SystemResourceKey _cacheMenuAnimation; private static SystemResourceKey _cacheSelectionFade; private static SystemResourceKey _cacheStylusHotTracking; private static SystemResourceKey _cacheToolTipAnimation; private static SystemResourceKey _cacheToolTipFade; private static SystemResourceKey _cacheUIEffects; private static SystemResourceKey _cacheMinimizeAnimation; private static SystemResourceKey _cacheBorder; private static SystemResourceKey _cacheCaretWidth; private static SystemResourceKey _cacheForegroundFlashCount; private static SystemResourceKey _cacheDragFullWindows; private static SystemResourceKey _cacheBorderWidth; private static SystemResourceKey _cacheScrollWidth; private static SystemResourceKey _cacheScrollHeight; private static SystemResourceKey _cacheCaptionWidth; private static SystemResourceKey _cacheCaptionHeight; private static SystemResourceKey _cacheSmallCaptionWidth; private static SystemResourceKey _cacheMenuWidth; private static SystemResourceKey _cacheMenuHeight; private static SystemResourceKey _cacheComboBoxPopupAnimation; private static SystemResourceKey _cacheMenuPopupAnimation; private static SystemResourceKey _cacheToolTipPopupAnimation; private static SystemResourceKey _cachePowerLineStatus; private static SystemThemeKey _cacheFocusVisualStyle; private static SystemThemeKey _cacheNavigationChromeStyle; private static SystemThemeKey _cacheNavigationChromeDownLevelStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.ComponentModel; using System.Runtime.InteropServices; using System.Windows.Media; using System.Security; using System.Windows.Controls.Primitives; using Microsoft.Win32; using MS.Win32; using MS.Internal; using MS.Internal.Interop; using MS.Internal.KnownBoxes; // Disable pragma warnings to enable PREsharp pragmas #pragma warning disable 1634, 1691 namespace System.Windows { ////// Indicates whether the system power is online, or that the system power status is unknown. /// public enum PowerLineStatus { ////// The system is offline. /// Offline = 0x00, ////// The system is online. /// Online = 0x01, ////// The power status of the system is unknown. /// Unknown = 0xFF, } ////// Contains properties that are queries into the system's various settings. /// public static class SystemParameters { // Disable Warning 6503 Property get methods should not throw exceptions. // By design properties below throw Win32Exception if there is an error when calling the native method #pragma warning disable 6503 // Win32Exception will get the last Win32 error code in case of errors, so we don't have to. #pragma warning disable 6523 #region Accessibility Parameters ////// Maps to SPI_GETFOCUSBORDERWIDTH /// ////// PublicOK -- Determined safe: getting the size of the dotted rectangle around a selected obj /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusBorderWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusBorderWidth]) { int focusBorderWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOCUSBORDERWIDTH, 0, ref focusBorderWidth, 0)) { _focusBorderWidth = ConvertPixel(focusBorderWidth); _cacheValid[(int)CacheSlot.FocusBorderWidth] = true; } else { throw new Win32Exception(); } } } return _focusBorderWidth; } } ////// Maps to SPI_GETFOCUSBORDERHEIGHT /// ////// PublicOK -- Determined safe: getting the size of the dotted rectangle around a selected obj /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusBorderHeight { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusBorderHeight]) { int focusBorderHeight = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOCUSBORDERHEIGHT, 0, ref focusBorderHeight, 0)) { _focusBorderHeight = ConvertPixel(focusBorderHeight); _cacheValid[(int)CacheSlot.FocusBorderHeight] = true; } else { throw new Win32Exception(); } } } return _focusBorderHeight; } } ////// Maps to SPI_GETHIGHCONTRAST -> HCF_HIGHCONTRASTON /// ////// Critical as this code does an elevation. /// PublicOK - considered ok to expose since the method doesn't take user input and only /// returns a boolean value which indicates the current high contrast mode. /// public static bool HighContrast { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HighContrast]) { NativeMethods.HIGHCONTRAST_I highContrast = new NativeMethods.HIGHCONTRAST_I(); highContrast.cbSize = Marshal.SizeOf(typeof(NativeMethods.HIGHCONTRAST_I)); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHIGHCONTRAST, highContrast.cbSize, ref highContrast, 0)) { _highContrast = (highContrast.dwFlags & NativeMethods.HCF_HIGHCONTRASTON) == NativeMethods.HCF_HIGHCONTRASTON; _cacheValid[(int)CacheSlot.HighContrast] = true; } else { throw new Win32Exception(); } } } return _highContrast; } } ////// Maps to SPI_GETMOUSEVANISH. /// ////// Critical -- calling UnsafeNativeMethods /// PublicOK - considered ok to expose. /// // internal static bool MouseVanish { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseVanish]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEVANISH, 0, ref _mouseVanish, 0)) { _cacheValid[(int)CacheSlot.MouseVanish] = true; } else { throw new Win32Exception(); } } } return _mouseVanish; } } #endregion [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static SystemResourceKey CreateInstance(SystemResourceKeyID KeyId) { return new SystemResourceKey(KeyId); } #region Accessibility Keys ////// FocusBorderWidth System Resource Key /// public static ResourceKey FocusBorderWidthKey { get { if (_cacheFocusBorderWidth == null) { _cacheFocusBorderWidth = CreateInstance(SystemResourceKeyID.FocusBorderWidth); } return _cacheFocusBorderWidth; } } ////// FocusBorderHeight System Resource Key /// public static ResourceKey FocusBorderHeightKey { get { if (_cacheFocusBorderHeight == null) { _cacheFocusBorderHeight = CreateInstance(SystemResourceKeyID.FocusBorderHeight); } return _cacheFocusBorderHeight; } } ////// HighContrast System Resource Key /// public static ResourceKey HighContrastKey { get { if (_cacheHighContrast == null) { _cacheHighContrast = CreateInstance(SystemResourceKeyID.HighContrast); } return _cacheHighContrast; } } #endregion #region Desktop Parameters ////// Maps to SPI_GETDROPSHADOW /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool DropShadow { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.DropShadow]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDROPSHADOW, 0, ref _dropShadow, 0)) { _cacheValid[(int)CacheSlot.DropShadow] = true; } else { throw new Win32Exception(); } } } return _dropShadow; } } ////// Maps to SPI_GETFLATMENU /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool FlatMenu { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FlatMenu]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFLATMENU, 0, ref _flatMenu, 0)) { _cacheValid[(int)CacheSlot.FlatMenu] = true; } else { throw new Win32Exception(); } } } return _flatMenu; } } ////// Maps to SPI_GETWORKAREA /// ////// SecurityCritical because it calls an unsafe native method. /// TreatAsSafe - Okay to expose info to internet callers. /// internal static NativeMethods.RECT WorkAreaInternal { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WorkAreaInternal]) { _workAreaInternal = new NativeMethods.RECT(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWORKAREA, 0, ref _workAreaInternal, 0)) { _cacheValid[(int)CacheSlot.WorkAreaInternal] = true; } else { throw new Win32Exception(); } } } return _workAreaInternal; } } ////// Maps to SPI_GETWORKAREA /// public static Rect WorkArea { get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WorkArea]) { NativeMethods.RECT workArea = WorkAreaInternal; _workArea = new Rect(ConvertPixel(workArea.left), ConvertPixel(workArea.top), ConvertPixel(workArea.right - workArea.left + 1), ConvertPixel(workArea.bottom - workArea.top + 1)); _cacheValid[(int)CacheSlot.WorkArea] = true; } } return _workArea; } } #endregion #region Desktop Keys ////// DropShadow System Resource Key /// public static ResourceKey DropShadowKey { get { if (_cacheDropShadow == null) { _cacheDropShadow = CreateInstance(SystemResourceKeyID.DropShadow); } return _cacheDropShadow; } } ////// FlatMenu System Resource Key /// public static ResourceKey FlatMenuKey { get { if (_cacheFlatMenu == null) { _cacheFlatMenu = CreateInstance(SystemResourceKeyID.FlatMenu); } return _cacheFlatMenu; } } ////// WorkArea System Resource Key /// public static ResourceKey WorkAreaKey { get { if (_cacheWorkArea == null) { _cacheWorkArea = CreateInstance(SystemResourceKeyID.WorkArea); } return _cacheWorkArea; } } #endregion #region Icon Parameters ////// Maps to SPI_GETICONMETRICS /// ////// SecurityCritical because it calls an unsafe native method. /// TreatAsSafe - Okay to expose info to internet callers. /// internal static NativeMethods.ICONMETRICS IconMetrics { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconMetrics]) { _iconMetrics = new NativeMethods.ICONMETRICS(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETICONMETRICS, _iconMetrics.cbSize, _iconMetrics, 0)) { _cacheValid[(int)CacheSlot.IconMetrics] = true; } else { throw new Win32Exception(); } } } return _iconMetrics; } } ////// Maps to SPI_GETICONMETRICS -> iHorzSpacing or SPI_ICONHORIZONTALSPACING /// public static double IconHorizontalSpacing { get { return ConvertPixel(IconMetrics.iHorzSpacing); } } ////// Maps to SPI_GETICONMETRICS -> iVertSpacing or SPI_ICONVERTICALSPACING /// public static double IconVerticalSpacing { get { return ConvertPixel(IconMetrics.iVertSpacing); } } ////// Maps to SPI_GETICONMETRICS -> iTitleWrap or SPI_GETICONTITLEWRAP /// public static bool IconTitleWrap { get { return IconMetrics.iTitleWrap != 0; } } #endregion #region Icon Keys ////// IconHorizontalSpacing System Resource Key /// public static ResourceKey IconHorizontalSpacingKey { get { if (_cacheIconHorizontalSpacing == null) { _cacheIconHorizontalSpacing = CreateInstance(SystemResourceKeyID.IconHorizontalSpacing); } return _cacheIconHorizontalSpacing; } } ////// IconVerticalSpacing System Resource Key /// public static ResourceKey IconVerticalSpacingKey { get { if (_cacheIconVerticalSpacing == null) { _cacheIconVerticalSpacing = CreateInstance(SystemResourceKeyID.IconVerticalSpacing); } return _cacheIconVerticalSpacing; } } ////// IconTitleWrap System Resource Key /// public static ResourceKey IconTitleWrapKey { get { if (_cacheIconTitleWrap == null) { _cacheIconTitleWrap = CreateInstance(SystemResourceKeyID.IconTitleWrap); } return _cacheIconTitleWrap; } } #endregion #region Input Parameters ////// Maps to SPI_GETKEYBOARDCUES /// /// ////// Demanding unmanaged code permission because calling an unsafe native method. /// SecurityCritical because it calls an unsafe native method. PublicOK because is demanding unmanaged code perm. /// PublicOK: This information is ok to give out /// public static bool KeyboardCues { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardCues]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDCUES, 0, ref _keyboardCues, 0)) { _cacheValid[(int)CacheSlot.KeyboardCues] = true; } else { throw new Win32Exception(); } } } return _keyboardCues; } } ////// Maps to SPI_GETKEYBOARDDELAY /// ////// PublicOK -- Determined safe: getting keyboard repeat delay /// Security Critical -- Calling UnsafeNativeMethods /// public static int KeyboardDelay { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardDelay]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDDELAY, 0, ref _keyboardDelay, 0)) { _cacheValid[(int)CacheSlot.KeyboardDelay] = true; } else { throw new Win32Exception(); } } } return _keyboardDelay; } } ////// Maps to SPI_GETKEYBOARDPREF /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool KeyboardPreference { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardPreference]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDPREF, 0, ref _keyboardPref, 0)) { _cacheValid[(int)CacheSlot.KeyboardPreference] = true; } else { throw new Win32Exception(); } } } return _keyboardPref; } } ////// Maps to SPI_GETKEYBOARDSPEED /// ////// PublicOK -- Determined safe: getting keyboard repeat-speed /// Security Critical -- Calling UnsafeNativeMethods /// public static int KeyboardSpeed { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KeyboardSpeed]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETKEYBOARDSPEED, 0, ref _keyboardSpeed, 0)) { _cacheValid[(int)CacheSlot.KeyboardSpeed] = true; } else { throw new Win32Exception(); } } } return _keyboardSpeed; } } ////// Maps to SPI_GETSNAPTODEFBUTTON /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool SnapToDefaultButton { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SnapToDefaultButton]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSNAPTODEFBUTTON, 0, ref _snapToDefButton, 0)) { _cacheValid[(int)CacheSlot.SnapToDefaultButton] = true; } else { throw new Win32Exception(); } } } return _snapToDefButton; } } ////// Maps to SPI_GETWHEELSCROLLLINES /// ////// Get is PublicOK -- Determined safe: Geting the number of lines to scroll when the mouse wheel is rotated. \ /// Get is Critical -- Calling unsafe native methods. /// public static int WheelScrollLines { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WheelScrollLines]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETWHEELSCROLLLINES, 0, ref _wheelScrollLines, 0)) { _cacheValid[(int)CacheSlot.WheelScrollLines] = true; } else { throw new Win32Exception(); } } } return _wheelScrollLines; } } ////// Maps to SPI_GETMOUSEHOVERTIME. /// public static TimeSpan MouseHoverTime { get { return TimeSpan.FromMilliseconds(MouseHoverTimeMilliseconds); } } ////// TreatAsSafe -- Determined safe: getting time mouse pointer has to stay in the hover rectangle for TrackMouseEvent to generate a WM_MOUSEHOVER message. /// Security Critical -- Calling UnsafeNativeMethods /// internal static int MouseHoverTimeMilliseconds { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverTime]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERTIME, 0, ref _mouseHoverTime, 0)) { _cacheValid[(int)CacheSlot.MouseHoverTime] = true; } else { throw new Win32Exception(); } } } return _mouseHoverTime; } } ////// Maps to SPI_GETMOUSEHOVERHEIGHT. /// ////// PublicOK -- Determined safe: gettingthe height, in pixels, of the rectangle within which the mouse pointer has to stay for TrackMouseEvent to generate a WM_MOUSEHOVER message /// Security Critical -- Calling UnsafeNativeMethods /// public static double MouseHoverHeight { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverHeight]) { int mouseHoverHeight = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERHEIGHT, 0, ref mouseHoverHeight, 0)) { _mouseHoverHeight = ConvertPixel(mouseHoverHeight); _cacheValid[(int)CacheSlot.MouseHoverHeight] = true; } else { throw new Win32Exception(); } } } return _mouseHoverHeight; } } ////// Maps to SPI_GETMOUSEHOVERWIDTH. /// /// ////// PublicOK -- Determined safe: getting the width, in pixels, of the rectangle within which the mouse pointer has to stay for TrackMouseEvent to generate a WM_MOUSEHOVER message /// Security Critical -- Calling UnsafeNativeMethods /// public static double MouseHoverWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MouseHoverWidth]) { int mouseHoverWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMOUSEHOVERWIDTH, 0, ref mouseHoverWidth, 0)) { _mouseHoverWidth = ConvertPixel(mouseHoverWidth); _cacheValid[(int)CacheSlot.MouseHoverWidth] = true; } else { throw new Win32Exception(); } } } return _mouseHoverWidth; } } #endregion #region Input Keys ////// KeyboardCues System Resource Key /// public static ResourceKey KeyboardCuesKey { get { if (_cacheKeyboardCues == null) { _cacheKeyboardCues = CreateInstance(SystemResourceKeyID.KeyboardCues); } return _cacheKeyboardCues; } } ////// KeyboardDelay System Resource Key /// public static ResourceKey KeyboardDelayKey { get { if (_cacheKeyboardDelay == null) { _cacheKeyboardDelay = CreateInstance(SystemResourceKeyID.KeyboardDelay); } return _cacheKeyboardDelay; } } ////// KeyboardPreference System Resource Key /// public static ResourceKey KeyboardPreferenceKey { get { if (_cacheKeyboardPreference == null) { _cacheKeyboardPreference = CreateInstance(SystemResourceKeyID.KeyboardPreference); } return _cacheKeyboardPreference; } } ////// KeyboardSpeed System Resource Key /// public static ResourceKey KeyboardSpeedKey { get { if (_cacheKeyboardSpeed == null) { _cacheKeyboardSpeed = CreateInstance(SystemResourceKeyID.KeyboardSpeed); } return _cacheKeyboardSpeed; } } ////// SnapToDefaultButton System Resource Key /// public static ResourceKey SnapToDefaultButtonKey { get { if (_cacheSnapToDefaultButton == null) { _cacheSnapToDefaultButton = CreateInstance(SystemResourceKeyID.SnapToDefaultButton); } return _cacheSnapToDefaultButton; } } ////// WheelScrollLines System Resource Key /// public static ResourceKey WheelScrollLinesKey { get { if (_cacheWheelScrollLines == null) { _cacheWheelScrollLines = CreateInstance(SystemResourceKeyID.WheelScrollLines); } return _cacheWheelScrollLines; } } ////// MouseHoverTime System Resource Key /// public static ResourceKey MouseHoverTimeKey { get { if (_cacheMouseHoverTime == null) { _cacheMouseHoverTime = CreateInstance(SystemResourceKeyID.MouseHoverTime); } return _cacheMouseHoverTime; } } ////// MouseHoverHeight System Resource Key /// public static ResourceKey MouseHoverHeightKey { get { if (_cacheMouseHoverHeight == null) { _cacheMouseHoverHeight = CreateInstance(SystemResourceKeyID.MouseHoverHeight); } return _cacheMouseHoverHeight; } } ////// MouseHoverWidth System Resource Key /// public static ResourceKey MouseHoverWidthKey { get { if (_cacheMouseHoverWidth == null) { _cacheMouseHoverWidth = CreateInstance(SystemResourceKeyID.MouseHoverWidth); } return _cacheMouseHoverWidth; } } #endregion #region Menu Parameters ////// Maps to SPI_GETMENUDROPALIGNMENT /// ////// Demanding unmanaged code permission because calling an unsafe native method. /// Critical - get: it calls an unsafe native method /// PublicOK - get: it's safe to expose a menu drop alignment of a system. /// public static bool MenuDropAlignment { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuDropAlignment]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUDROPALIGNMENT, 0, ref _menuDropAlignment, 0)) { _cacheValid[(int)CacheSlot.MenuDropAlignment] = true; } else { throw new Win32Exception(); } } } return _menuDropAlignment; } } ////// Maps to SPI_GETMENUFADE /// ////// Critical - because it calls an unsafe native method /// PublicOK - ok to return menu fade data /// public static bool MenuFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUFADE, 0, ref _menuFade, 0)) { _cacheValid[(int)CacheSlot.MenuFade] = true; } else { throw new Win32Exception(); } } } return _menuFade; } } ////// Maps to SPI_GETMENUSHOWDELAY /// ////// Critical - calls a method that perfoms an elevation. /// PublicOK - considered ok to expose in partial trust. /// public static int MenuShowDelay { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuShowDelay]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUSHOWDELAY, 0, ref _menuShowDelay, 0)) { _cacheValid[(int)CacheSlot.MenuShowDelay] = true; } else { throw new Win32Exception(); } } } return _menuShowDelay; } } #endregion #region Menu Keys ////// MenuDropAlignment System Resource Key /// public static ResourceKey MenuDropAlignmentKey { get { if (_cacheMenuDropAlignment == null) { _cacheMenuDropAlignment = CreateInstance(SystemResourceKeyID.MenuDropAlignment); } return _cacheMenuDropAlignment; } } ////// MenuFade System Resource Key /// public static ResourceKey MenuFadeKey { get { if (_cacheMenuFade == null) { _cacheMenuFade = CreateInstance(SystemResourceKeyID.MenuFade); } return _cacheMenuFade; } } ////// MenuShowDelay System Resource Key /// public static ResourceKey MenuShowDelayKey { get { if (_cacheMenuShowDelay == null) { _cacheMenuShowDelay = CreateInstance(SystemResourceKeyID.MenuShowDelay); } return _cacheMenuShowDelay; } } #endregion #region UI Effects Parameters ////// Returns the system value of PopupAnimation for ComboBoxes. /// public static PopupAnimation ComboBoxPopupAnimation { get { if (ComboBoxAnimation) { return PopupAnimation.Slide; } return PopupAnimation.None; } } ////// Maps to SPI_GETCOMBOBOXANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool ComboBoxAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ComboBoxAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCOMBOBOXANIMATION, 0, ref _comboBoxAnimation, 0)) { _cacheValid[(int)CacheSlot.ComboBoxAnimation] = true; } else { throw new Win32Exception(); } } } return _comboBoxAnimation; } } ////// Maps to SPI_GETCLIENTAREAANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK: This information is ok to give out /// public static bool ClientAreaAnimation { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ClientAreaAnimation]) { // This parameter is only available on Windows Versions >= 0x0600 (Vista) if (System.Environment.OSVersion.Version.Major >= 6) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCLIENTAREAANIMATION, 0, ref _clientAreaAnimation, 0)) { _cacheValid[(int)CacheSlot.ClientAreaAnimation] = true; } else { throw new Win32Exception(); } } else // Windows XP, assume value is true { _clientAreaAnimation = true; _cacheValid[(int)CacheSlot.ClientAreaAnimation] = true; } } } return _clientAreaAnimation; } } ////// Maps to SPI_GETCURSORSHADOW /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool CursorShadow { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorShadow]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCURSORSHADOW, 0, ref _cursorShadow, 0)) { _cacheValid[(int)CacheSlot.CursorShadow] = true; } else { throw new Win32Exception(); } } } return _cursorShadow; } } ////// Maps to SPI_GETGRADIENTCAPTIONS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool GradientCaptions { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.GradientCaptions]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETGRADIENTCAPTIONS, 0, ref _gradientCaptions, 0)) { _cacheValid[(int)CacheSlot.GradientCaptions] = true; } else { throw new Win32Exception(); } } } return _gradientCaptions; } } ////// Maps to SPI_GETHOTTRACKING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool HotTracking { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HotTracking]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETHOTTRACKING, 0, ref _hotTracking, 0)) { _cacheValid[(int)CacheSlot.HotTracking] = true; } else { throw new Win32Exception(); } } } return _hotTracking; } } ////// Maps to SPI_GETLISTBOXSMOOTHSCROLLING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool ListBoxSmoothScrolling { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ListBoxSmoothScrolling]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETLISTBOXSMOOTHSCROLLING, 0, ref _listBoxSmoothScrolling, 0)) { _cacheValid[(int)CacheSlot.ListBoxSmoothScrolling] = true; } else { throw new Win32Exception(); } } } return _listBoxSmoothScrolling; } } ////// Returns the PopupAnimation value for Menus. /// public static PopupAnimation MenuPopupAnimation { get { if (MenuAnimation) { if (MenuFade) { return PopupAnimation.Fade; } else { return PopupAnimation.Scroll; } } return PopupAnimation.None; } } ////// Maps to SPI_GETMENUANIMATION /// ////// Critical - calls SystemParametersInfo /// PublicOK - net information returned is whether menu-animation is enabled. Considered safe. /// public static bool MenuAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETMENUANIMATION, 0, ref _menuAnimation, 0)) { _cacheValid[(int)CacheSlot.MenuAnimation] = true; } else { throw new Win32Exception(); } } } return _menuAnimation; } } ////// Maps to SPI_GETSELECTIONFADE /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool SelectionFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SelectionFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSELECTIONFADE, 0, ref _selectionFade, 0)) { _cacheValid[(int)CacheSlot.SelectionFade] = true; } else { throw new Win32Exception(); } } } return _selectionFade; } } ////// Maps to SPI_GETSTYLUSHOTTRACKING /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool StylusHotTracking { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.StylusHotTracking]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETSTYLUSHOTTRACKING, 0, ref _stylusHotTracking, 0)) { _cacheValid[(int)CacheSlot.StylusHotTracking] = true; } else { throw new Win32Exception(); } } } return _stylusHotTracking; } } ////// Returns the PopupAnimation value for ToolTips. /// public static PopupAnimation ToolTipPopupAnimation { get { // Win32 ToolTips do not appear to scroll, only fade if (ToolTipAnimation && ToolTipFade) { return PopupAnimation.Fade; } return PopupAnimation.None; } } ////// Maps to SPI_GETTOOLTIPANIMATION /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static bool ToolTipAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ToolTipAnimation]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETTOOLTIPANIMATION, 0, ref _toolTipAnimation, 0)) { _cacheValid[(int)CacheSlot.ToolTipAnimation] = true; } else { throw new Win32Exception(); } } } return _toolTipAnimation; } } ////// Maps to SPI_GETTOOLTIPFADE /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static bool ToolTipFade { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ToolTipFade]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETTOOLTIPFADE, 0, ref _tooltipFade, 0)) { _cacheValid[(int)CacheSlot.ToolTipFade] = true; } else { throw new Win32Exception(); } } } return _tooltipFade; } } ////// Maps to SPI_GETUIEFFECTS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool UIEffects { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.UIEffects]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETUIEFFECTS, 0, ref _uiEffects, 0)) { _cacheValid[(int)CacheSlot.UIEffects] = true; } else { throw new Win32Exception(); } } } return _uiEffects; } } #endregion #region UI Effects Keys ////// ComboBoxAnimation System Resource Key /// public static ResourceKey ComboBoxAnimationKey { get { if (_cacheComboBoxAnimation == null) { _cacheComboBoxAnimation = CreateInstance(SystemResourceKeyID.ComboBoxAnimation); } return _cacheComboBoxAnimation; } } ////// ClientAreaAnimation System Resource Key /// public static ResourceKey ClientAreaAnimationKey { get { if (_cacheClientAreaAnimation == null) { _cacheClientAreaAnimation = CreateInstance(SystemResourceKeyID.ClientAreaAnimation); } return _cacheClientAreaAnimation; } } ////// CursorShadow System Resource Key /// public static ResourceKey CursorShadowKey { get { if (_cacheCursorShadow == null) { _cacheCursorShadow = CreateInstance(SystemResourceKeyID.CursorShadow); } return _cacheCursorShadow; } } ////// GradientCaptions System Resource Key /// public static ResourceKey GradientCaptionsKey { get { if (_cacheGradientCaptions == null) { _cacheGradientCaptions = CreateInstance(SystemResourceKeyID.GradientCaptions); } return _cacheGradientCaptions; } } ////// HotTracking System Resource Key /// public static ResourceKey HotTrackingKey { get { if (_cacheHotTracking == null) { _cacheHotTracking = CreateInstance(SystemResourceKeyID.HotTracking); } return _cacheHotTracking; } } ////// ListBoxSmoothScrolling System Resource Key /// public static ResourceKey ListBoxSmoothScrollingKey { get { if (_cacheListBoxSmoothScrolling == null) { _cacheListBoxSmoothScrolling = CreateInstance(SystemResourceKeyID.ListBoxSmoothScrolling); } return _cacheListBoxSmoothScrolling; } } ////// MenuAnimation System Resource Key /// public static ResourceKey MenuAnimationKey { get { if (_cacheMenuAnimation == null) { _cacheMenuAnimation = CreateInstance(SystemResourceKeyID.MenuAnimation); } return _cacheMenuAnimation; } } ////// SelectionFade System Resource Key /// public static ResourceKey SelectionFadeKey { get { if (_cacheSelectionFade == null) { _cacheSelectionFade = CreateInstance(SystemResourceKeyID.SelectionFade); } return _cacheSelectionFade; } } ////// StylusHotTracking System Resource Key /// public static ResourceKey StylusHotTrackingKey { get { if (_cacheStylusHotTracking == null) { _cacheStylusHotTracking = CreateInstance(SystemResourceKeyID.StylusHotTracking); } return _cacheStylusHotTracking; } } ////// ToolTipAnimation System Resource Key /// public static ResourceKey ToolTipAnimationKey { get { if (_cacheToolTipAnimation == null) { _cacheToolTipAnimation = CreateInstance(SystemResourceKeyID.ToolTipAnimation); } return _cacheToolTipAnimation; } } ////// ToolTipFade System Resource Key /// public static ResourceKey ToolTipFadeKey { get { if (_cacheToolTipFade == null) { _cacheToolTipFade = CreateInstance(SystemResourceKeyID.ToolTipFade); } return _cacheToolTipFade; } } ////// UIEffects System Resource Key /// public static ResourceKey UIEffectsKey { get { if (_cacheUIEffects == null) { _cacheUIEffects = CreateInstance(SystemResourceKeyID.UIEffects); } return _cacheUIEffects; } } ////// ComboBoxPopupAnimation System Resource Key /// public static ResourceKey ComboBoxPopupAnimationKey { get { if (_cacheComboBoxPopupAnimation == null) { _cacheComboBoxPopupAnimation = CreateInstance(SystemResourceKeyID.ComboBoxPopupAnimation); } return _cacheComboBoxPopupAnimation; } } ////// MenuPopupAnimation System Resource Key /// public static ResourceKey MenuPopupAnimationKey { get { if (_cacheMenuPopupAnimation == null) { _cacheMenuPopupAnimation = CreateInstance(SystemResourceKeyID.MenuPopupAnimation); } return _cacheMenuPopupAnimation; } } ////// ToolTipPopupAnimation System Resource Key /// public static ResourceKey ToolTipPopupAnimationKey { get { if (_cacheToolTipPopupAnimation == null) { _cacheToolTipPopupAnimation = CreateInstance(SystemResourceKeyID.ToolTipPopupAnimation); } return _cacheToolTipPopupAnimation; } } #endregion #region Window Parameters ////// Maps to SPI_GETANIMATION /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool MinimizeAnimation { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizeAnimation]) { NativeMethods.ANIMATIONINFO animInfo = new NativeMethods.ANIMATIONINFO(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETANIMATION, animInfo.cbSize, animInfo, 0)) { _minAnimation = animInfo.iMinAnimate != 0; _cacheValid[(int)CacheSlot.MinimizeAnimation] = true; } else { throw new Win32Exception(); } } } return _minAnimation; } } ////// Maps to SPI_GETBORDER /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static int Border { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.Border]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETBORDER, 0, ref _border, 0)) { _cacheValid[(int)CacheSlot.Border] = true; } else { throw new Win32Exception(); } } } return _border; } } ////// Maps to SPI_GETCARETWIDTH /// ////// PublicOK -- Determined safe: getting width of caret /// Security Critical -- Calling UnsafeNativeMethods /// public static double CaretWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CaretWidth]) { int caretWidth = 0; if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETCARETWIDTH, 0, ref caretWidth, 0)) { _caretWidth = ConvertPixel(caretWidth); _cacheValid[(int)CacheSlot.CaretWidth] = true; } else { throw new Win32Exception(); } } } return _caretWidth; } } ////// Maps to SPI_GETDRAGFULLWINDOWS /// ////// SecurityCritical because it calls an unsafe native method. /// PublicOK - Okay to expose info to internet callers. /// public static bool DragFullWindows { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.DragFullWindows]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETDRAGFULLWINDOWS, 0, ref _dragFullWindows, 0)) { _cacheValid[(int)CacheSlot.DragFullWindows] = true; } else { throw new Win32Exception(); } } } return _dragFullWindows; } } ////// Maps to SPI_GETFOREGROUNDFLASHCOUNT /// ////// Get is PublicOK -- Getting # of times taskbar button will flash when rejecting a forecground switch request. /// Get is Security Critical -- Calling UnsafeNativeMethods /// public static int ForegroundFlashCount { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ForegroundFlashCount]) { if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETFOREGROUNDFLASHCOUNT, 0, ref _foregroundFlashCount, 0)) { _cacheValid[(int)CacheSlot.ForegroundFlashCount] = true; } else { throw new Win32Exception(); } } } return _foregroundFlashCount; } } ////// Maps to SPI_GETNONCLIENTMETRICS /// ////// SecurityCritical because it calls an unsafe native method. /// SecurityTreatAsSafe as we think this would be ok to expose publically - and this is ok for consumption in partial trust. /// internal static NativeMethods.NONCLIENTMETRICS NonClientMetrics { [SecurityCritical, SecurityTreatAsSafe] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.NonClientMetrics]) { _ncm = new NativeMethods.NONCLIENTMETRICS(); if (UnsafeNativeMethods.SystemParametersInfo(NativeMethods.SPI_GETNONCLIENTMETRICS, _ncm.cbSize, _ncm, 0)) { _cacheValid[(int)CacheSlot.NonClientMetrics] = true; } else { throw new Win32Exception(); } } } return _ncm; } } ////// From SPI_GETNONCLIENTMETRICS /// public static double BorderWidth { get { return ConvertPixel(NonClientMetrics.iBorderWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double ScrollWidth { get { return ConvertPixel(NonClientMetrics.iScrollWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double ScrollHeight { get { return ConvertPixel(NonClientMetrics.iScrollHeight); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double CaptionWidth { get { return ConvertPixel(NonClientMetrics.iCaptionWidth); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double CaptionHeight { get { return ConvertPixel(NonClientMetrics.iCaptionHeight); } } ////// From SPI_GETNONCLIENTMETRICS /// public static double SmallCaptionWidth { get { return ConvertPixel(NonClientMetrics.iSmCaptionWidth); } } ////// From SPI_NONCLIENTMETRICS /// public static double SmallCaptionHeight { get { return ConvertPixel(NonClientMetrics.iSmCaptionHeight); } } ////// From SPI_NONCLIENTMETRICS /// public static double MenuWidth { get { return ConvertPixel(NonClientMetrics.iMenuWidth); } } ////// From SPI_NONCLIENTMETRICS /// public static double MenuHeight { get { return ConvertPixel(NonClientMetrics.iMenuHeight); } } #endregion #region Window Parameters Keys ////// MinimizeAnimation System Resource Key /// public static ResourceKey MinimizeAnimationKey { get { if (_cacheMinimizeAnimation == null) { _cacheMinimizeAnimation = CreateInstance(SystemResourceKeyID.MinimizeAnimation); } return _cacheMinimizeAnimation; } } ////// Border System Resource Key /// public static ResourceKey BorderKey { get { if (_cacheBorder == null) { _cacheBorder = CreateInstance(SystemResourceKeyID.Border); } return _cacheBorder; } } ////// CaretWidth System Resource Key /// public static ResourceKey CaretWidthKey { get { if (_cacheCaretWidth == null) { _cacheCaretWidth = CreateInstance(SystemResourceKeyID.CaretWidth); } return _cacheCaretWidth; } } ////// ForegroundFlashCount System Resource Key /// public static ResourceKey ForegroundFlashCountKey { get { if (_cacheForegroundFlashCount == null) { _cacheForegroundFlashCount = CreateInstance(SystemResourceKeyID.ForegroundFlashCount); } return _cacheForegroundFlashCount; } } ////// DragFullWindows System Resource Key /// public static ResourceKey DragFullWindowsKey { get { if (_cacheDragFullWindows == null) { _cacheDragFullWindows = CreateInstance(SystemResourceKeyID.DragFullWindows); } return _cacheDragFullWindows; } } ////// BorderWidth System Resource Key /// public static ResourceKey BorderWidthKey { get { if (_cacheBorderWidth == null) { _cacheBorderWidth = CreateInstance(SystemResourceKeyID.BorderWidth); } return _cacheBorderWidth; } } ////// ScrollWidth System Resource Key /// public static ResourceKey ScrollWidthKey { get { if (_cacheScrollWidth == null) { _cacheScrollWidth = CreateInstance(SystemResourceKeyID.ScrollWidth); } return _cacheScrollWidth; } } ////// ScrollHeight System Resource Key /// public static ResourceKey ScrollHeightKey { get { if (_cacheScrollHeight == null) { _cacheScrollHeight = CreateInstance(SystemResourceKeyID.ScrollHeight); } return _cacheScrollHeight; } } ////// CaptionWidth System Resource Key /// public static ResourceKey CaptionWidthKey { get { if (_cacheCaptionWidth == null) { _cacheCaptionWidth = CreateInstance(SystemResourceKeyID.CaptionWidth); } return _cacheCaptionWidth; } } ////// CaptionHeight System Resource Key /// public static ResourceKey CaptionHeightKey { get { if (_cacheCaptionHeight == null) { _cacheCaptionHeight = CreateInstance(SystemResourceKeyID.CaptionHeight); } return _cacheCaptionHeight; } } ////// SmallCaptionWidth System Resource Key /// public static ResourceKey SmallCaptionWidthKey { get { if (_cacheSmallCaptionWidth == null) { _cacheSmallCaptionWidth = CreateInstance(SystemResourceKeyID.SmallCaptionWidth); } return _cacheSmallCaptionWidth; } } ////// MenuWidth System Resource Key /// public static ResourceKey MenuWidthKey { get { if (_cacheMenuWidth == null) { _cacheMenuWidth = CreateInstance(SystemResourceKeyID.MenuWidth); } return _cacheMenuWidth; } } ////// MenuHeight System Resource Key /// public static ResourceKey MenuHeightKey { get { if (_cacheMenuHeight == null) { _cacheMenuHeight = CreateInstance(SystemResourceKeyID.MenuHeight); } return _cacheMenuHeight; } } #endregion #region Metrics ////// Maps to SM_CXBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThinHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThinHorizontalBorderHeight]) { _thinHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXBORDER)); _cacheValid[(int)CacheSlot.ThinHorizontalBorderHeight] = true; } } return _thinHorizontalBorderHeight; } } ////// Maps to SM_CYBORDER /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThinVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThinVerticalBorderWidth]) { _thinVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYBORDER)); _cacheValid[(int)CacheSlot.ThinVerticalBorderWidth] = true; } } return _thinVerticalBorderWidth; } } ////// Maps to SM_CXCURSOR /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double CursorWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorWidth]) { _cursorWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXCURSOR)); _cacheValid[(int)CacheSlot.CursorWidth] = true; } } return _cursorWidth; } } ////// Maps to SM_CYCURSOR /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double CursorHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.CursorHeight]) { _cursorHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYCURSOR)); _cacheValid[(int)CacheSlot.CursorHeight] = true; } } return _cursorHeight; } } ////// Maps to SM_CXEDGE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThickHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThickHorizontalBorderHeight]) { _thickHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXEDGE)); _cacheValid[(int)CacheSlot.ThickHorizontalBorderHeight] = true; } } return _thickHorizontalBorderHeight; } } ////// Maps to SM_CYEDGE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ThickVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ThickVerticalBorderWidth]) { _thickVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYEDGE)); _cacheValid[(int)CacheSlot.ThickVerticalBorderWidth] = true; } } return _thickVerticalBorderWidth; } } ////// Maps to SM_CXDRAG /// ////// Critical - calls into native code (GetSystemMetrics) /// PublicOK - Safe data to expose /// public static double MinimumHorizontalDragDistance { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumHorizontalDragDistance]) { _minimumHorizontalDragDistance = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXDRAG)); _cacheValid[(int)CacheSlot.MinimumHorizontalDragDistance] = true; } } return _minimumHorizontalDragDistance; } } ////// Maps to SM_CYDRAG /// ////// Critical - calls into native code (GetSystemMetrics) /// PublicOK - Safe data to expose /// public static double MinimumVerticalDragDistance { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumVerticalDragDistance]) { _minimumVerticalDragDistance = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYDRAG)); _cacheValid[(int)CacheSlot.MinimumVerticalDragDistance] = true; } } return _minimumVerticalDragDistance; } } ////// Maps to SM_CXFIXEDFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FixedFrameHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FixedFrameHorizontalBorderHeight]) { _fixedFrameHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFIXEDFRAME)); _cacheValid[(int)CacheSlot.FixedFrameHorizontalBorderHeight] = true; } } return _fixedFrameHorizontalBorderHeight; } } ////// Maps to SM_CYFIXEDFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FixedFrameVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FixedFrameVerticalBorderWidth]) { _fixedFrameVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFIXEDFRAME)); _cacheValid[(int)CacheSlot.FixedFrameVerticalBorderWidth] = true; } } return _fixedFrameVerticalBorderWidth; } } ////// Maps to SM_CXFOCUSBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusHorizontalBorderHeight]) { _focusHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFOCUSBORDER)); _cacheValid[(int)CacheSlot.FocusHorizontalBorderHeight] = true; } } return _focusHorizontalBorderHeight; } } ////// Maps to SM_CYFOCUSBORDER /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double FocusVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FocusVerticalBorderWidth]) { _focusVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFOCUSBORDER)); _cacheValid[(int)CacheSlot.FocusVerticalBorderWidth] = true; } } return _focusVerticalBorderWidth; } } ////// Maps to SM_CXFULLSCREEN /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double FullPrimaryScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FullPrimaryScreenWidth]) { _fullPrimaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXFULLSCREEN)); _cacheValid[(int)CacheSlot.FullPrimaryScreenWidth] = true; } } return _fullPrimaryScreenWidth; } } ////// Maps to SM_CYFULLSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double FullPrimaryScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.FullPrimaryScreenHeight]) { _fullPrimaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYFULLSCREEN)); _cacheValid[(int)CacheSlot.FullPrimaryScreenHeight] = true; } } return _fullPrimaryScreenHeight; } } ////// Maps to SM_CXHSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarButtonWidth]) { _horizontalScrollBarButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXHSCROLL)); _cacheValid[(int)CacheSlot.HorizontalScrollBarButtonWidth] = true; } } return _horizontalScrollBarButtonWidth; } } ////// Maps to SM_CYHSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarHeight]) { _horizontalScrollBarHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYHSCROLL)); _cacheValid[(int)CacheSlot.HorizontalScrollBarHeight] = true; } } return _horizontalScrollBarHeight; } } ////// Maps to SM_CXHTHUMB /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double HorizontalScrollBarThumbWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.HorizontalScrollBarThumbWidth]) { _horizontalScrollBarThumbWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXHTHUMB)); _cacheValid[(int)CacheSlot.HorizontalScrollBarThumbWidth] = true; } } return _horizontalScrollBarThumbWidth; } } ////// Maps to SM_CXICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconWidth]) { _iconWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXICON)); _cacheValid[(int)CacheSlot.IconWidth] = true; } } return _iconWidth; } } ////// Maps to SM_CYICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconHeight]) { _iconHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYICON)); _cacheValid[(int)CacheSlot.IconHeight] = true; } } return _iconHeight; } } ////// Maps to SM_CXICONSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconGridWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconGridWidth]) { _iconGridWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXICONSPACING)); _cacheValid[(int)CacheSlot.IconGridWidth] = true; } } return _iconGridWidth; } } ////// Maps to SM_CYICONSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double IconGridHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IconGridHeight]) { _iconGridHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYICONSPACING)); _cacheValid[(int)CacheSlot.IconGridHeight] = true; } } return _iconGridHeight; } } ////// Maps to SM_CXMAXIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximizedPrimaryScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximizedPrimaryScreenWidth]) { _maximizedPrimaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMAXIMIZED)); _cacheValid[(int)CacheSlot.MaximizedPrimaryScreenWidth] = true; } } return _maximizedPrimaryScreenWidth; } } ////// Maps to SM_CYMAXIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximizedPrimaryScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximizedPrimaryScreenHeight]) { _maximizedPrimaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMAXIMIZED)); _cacheValid[(int)CacheSlot.MaximizedPrimaryScreenHeight] = true; } } return _maximizedPrimaryScreenHeight; } } ////// Maps to SM_CXMAXTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximumWindowTrackWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximumWindowTrackWidth]) { _maximumWindowTrackWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMAXTRACK)); _cacheValid[(int)CacheSlot.MaximumWindowTrackWidth] = true; } } return _maximumWindowTrackWidth; } } ////// Maps to SM_CYMAXTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MaximumWindowTrackHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MaximumWindowTrackHeight]) { _maximumWindowTrackHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMAXTRACK)); _cacheValid[(int)CacheSlot.MaximumWindowTrackHeight] = true; } } return _maximumWindowTrackHeight; } } ////// Maps to SM_CXMENUCHECK /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuCheckmarkWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuCheckmarkWidth]) { _menuCheckmarkWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMENUCHECK)); _cacheValid[(int)CacheSlot.MenuCheckmarkWidth] = true; } } return _menuCheckmarkWidth; } } ////// Maps to SM_CYMENUCHECK /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuCheckmarkHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuCheckmarkHeight]) { _menuCheckmarkHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENUCHECK)); _cacheValid[(int)CacheSlot.MenuCheckmarkHeight] = true; } } return _menuCheckmarkHeight; } } ////// Maps to SM_CXMENUSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuButtonWidth]) { _menuButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMENUSIZE)); _cacheValid[(int)CacheSlot.MenuButtonWidth] = true; } } return _menuButtonWidth; } } ////// Maps to SM_CYMENUSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuButtonHeight]) { _menuButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENUSIZE)); _cacheValid[(int)CacheSlot.MenuButtonHeight] = true; } } return _menuButtonHeight; } } ////// Maps to SM_CXMIN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowWidth]) { _minimumWindowWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMIN)); _cacheValid[(int)CacheSlot.MinimumWindowWidth] = true; } } return _minimumWindowWidth; } } ////// Maps to SM_CYMIN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowHeight]) { _minimumWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMIN)); _cacheValid[(int)CacheSlot.MinimumWindowHeight] = true; } } return _minimumWindowHeight; } } ////// Maps to SM_CXMINIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK -- There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedWindowWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedWindowWidth]) { _minimizedWindowWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINIMIZED)); _cacheValid[(int)CacheSlot.MinimizedWindowWidth] = true; } } return _minimizedWindowWidth; } } ////// Maps to SM_CYMINIMIZED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedWindowHeight]) { _minimizedWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINIMIZED)); _cacheValid[(int)CacheSlot.MinimizedWindowHeight] = true; } } return _minimizedWindowHeight; } } ////// Maps to SM_CXMINSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedGridWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedGridWidth]) { _minimizedGridWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINSPACING)); _cacheValid[(int)CacheSlot.MinimizedGridWidth] = true; } } return _minimizedGridWidth; } } ////// Maps to SM_CYMINSPACING /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimizedGridHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimizedGridHeight]) { _minimizedGridHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINSPACING)); _cacheValid[(int)CacheSlot.MinimizedGridHeight] = true; } } return _minimizedGridHeight; } } ////// Maps to SM_CXMINTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exist a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowTrackWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowTrackWidth]) { _minimumWindowTrackWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXMINTRACK)); _cacheValid[(int)CacheSlot.MinimumWindowTrackWidth] = true; } } return _minimumWindowTrackWidth; } } ////// Maps to SM_CYMINTRACK /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MinimumWindowTrackHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MinimumWindowTrackHeight]) { _minimumWindowTrackHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMINTRACK)); _cacheValid[(int)CacheSlot.MinimumWindowTrackHeight] = true; } } return _minimumWindowTrackHeight; } } ////// Maps to SM_CXSCREEN /// ////// PublicOK -- This is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// public static double PrimaryScreenWidth { [SecurityCritical ] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PrimaryScreenWidth]) { _primaryScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSCREEN)); _cacheValid[(int)CacheSlot.PrimaryScreenWidth] = true; } } return _primaryScreenWidth; } } ////// Maps to SM_CYSCREEN /// ////// PublicOK --This is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// public static double PrimaryScreenHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PrimaryScreenHeight]) { _primaryScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSCREEN)); _cacheValid[(int)CacheSlot.PrimaryScreenHeight] = true; } } return _primaryScreenHeight; } } ////// Maps to SM_CXSIZE /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionButtonWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionButtonWidth]) { _windowCaptionButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSIZE)); _cacheValid[(int)CacheSlot.WindowCaptionButtonWidth] = true; } } return _windowCaptionButtonWidth; } } ////// Maps to SM_CYSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionButtonHeight]) { _windowCaptionButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSIZE)); _cacheValid[(int)CacheSlot.WindowCaptionButtonHeight] = true; } } return _windowCaptionButtonHeight; } } ////// Maps to SM_CXSIZEFRAME /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ResizeFrameHorizontalBorderHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ResizeFrameHorizontalBorderHeight]) { _resizeFrameHorizontalBorderHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSIZEFRAME)); _cacheValid[(int)CacheSlot.ResizeFrameHorizontalBorderHeight] = true; } } return _resizeFrameHorizontalBorderHeight; } } ////// Maps to SM_CYSIZEFRAME /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double ResizeFrameVerticalBorderWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ResizeFrameVerticalBorderWidth]) { _resizeFrameVerticalBorderWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSIZEFRAME)); _cacheValid[(int)CacheSlot.ResizeFrameVerticalBorderWidth] = true; } } return _resizeFrameVerticalBorderWidth; } } ////// Maps to SM_CXSMICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallIconWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallIconWidth]) { _smallIconWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSMICON)); _cacheValid[(int)CacheSlot.SmallIconWidth] = true; } } return _smallIconWidth; } } ////// Maps to SM_CYSMICON /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallIconHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallIconHeight]) { _smallIconHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSMICON)); _cacheValid[(int)CacheSlot.SmallIconHeight] = true; } } return _smallIconHeight; } } ////// Maps to SM_CXSMSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallWindowCaptionButtonWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallWindowCaptionButtonWidth]) { _smallWindowCaptionButtonWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXSMSIZE)); _cacheValid[(int)CacheSlot.SmallWindowCaptionButtonWidth] = true; } } return _smallWindowCaptionButtonWidth; } } ////// Maps to SM_CYSMSIZE /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double SmallWindowCaptionButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SmallWindowCaptionButtonHeight]) { _smallWindowCaptionButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYSMSIZE)); _cacheValid[(int)CacheSlot.SmallWindowCaptionButtonHeight] = true; } } return _smallWindowCaptionButtonHeight; } } ////// Maps to SM_CXVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenWidth { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenWidth]) { _virtualScreenWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenWidth] = true; } } return _virtualScreenWidth; } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenHeight]) { _virtualScreenHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenHeight] = true; } } return _virtualScreenHeight; } } ////// Maps to SM_CXVSCROLL /// /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarWidth { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarWidth]) { _verticalScrollBarWidth = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CXVSCROLL)); _cacheValid[(int)CacheSlot.VerticalScrollBarWidth] = true; } } return _verticalScrollBarWidth; } } ////// Maps to SM_CYVSCROLL /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarButtonHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarButtonHeight]) { _verticalScrollBarButtonHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVSCROLL)); _cacheValid[(int)CacheSlot.VerticalScrollBarButtonHeight] = true; } } return _verticalScrollBarButtonHeight; } } ////// Maps to SM_CYCAPTION /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double WindowCaptionHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.WindowCaptionHeight]) { _windowCaptionHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYCAPTION)); _cacheValid[(int)CacheSlot.WindowCaptionHeight] = true; } } return _windowCaptionHeight; } } ////// Maps to SM_CYKANJIWINDOW /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double KanjiWindowHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.KanjiWindowHeight]) { _kanjiWindowHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYKANJIWINDOW)); _cacheValid[(int)CacheSlot.KanjiWindowHeight] = true; } } return _kanjiWindowHeight; } } ////// Maps to SM_CYMENU /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static double MenuBarHeight { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.MenuBarHeight]) { _menuBarHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYMENU)); _cacheValid[(int)CacheSlot.MenuBarHeight] = true; } } return _menuBarHeight; } } ////// Maps to SM_CYVTHUMB /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static double VerticalScrollBarThumbHeight { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VerticalScrollBarThumbHeight]) { _verticalScrollBarThumbHeight = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.CYVTHUMB)); _cacheValid[(int)CacheSlot.VerticalScrollBarThumbHeight] = true; } } return _verticalScrollBarThumbHeight; } } ////// Maps to SM_IMMENABLED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand in this code. /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsImmEnabled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsImmEnabled]) { _isImmEnabled = UnsafeNativeMethods.GetSystemMetrics(SM.IMMENABLED) != 0; _cacheValid[(int)CacheSlot.IsImmEnabled] = true; } } return _isImmEnabled; } } ////// Maps to SM_MEDIACENTER /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMediaCenter { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMediaCenter]) { _isMediaCenter = UnsafeNativeMethods.GetSystemMetrics(SM.MEDIACENTER) != 0; _cacheValid[(int)CacheSlot.IsMediaCenter] = true; } } return _isMediaCenter; } } ////// Maps to SM_MENUDROPALIGNMENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMenuDropRightAligned { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMenuDropRightAligned]) { _isMenuDropRightAligned = UnsafeNativeMethods.GetSystemMetrics(SM.MENUDROPALIGNMENT) != 0; _cacheValid[(int)CacheSlot.IsMenuDropRightAligned] = true; } } return _isMenuDropRightAligned; } } ////// Maps to SM_MIDEASTENABLED /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMiddleEastEnabled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMiddleEastEnabled]) { _isMiddleEastEnabled = UnsafeNativeMethods.GetSystemMetrics(SM.MIDEASTENABLED) != 0; _cacheValid[(int)CacheSlot.IsMiddleEastEnabled] = true; } } return _isMiddleEastEnabled; } } ////// Maps to SM_MOUSEPRESENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMousePresent { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMousePresent]) { _isMousePresent = UnsafeNativeMethods.GetSystemMetrics(SM.MOUSEPRESENT) != 0; _cacheValid[(int)CacheSlot.IsMousePresent] = true; } } return _isMousePresent; } } ////// Maps to SM_MOUSEWHEELPRESENT /// ////// PublicOK --System Metrics are deemed safe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsMouseWheelPresent { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsMouseWheelPresent]) { _isMouseWheelPresent = UnsafeNativeMethods.GetSystemMetrics(SM.MOUSEWHEELPRESENT) != 0; _cacheValid[(int)CacheSlot.IsMouseWheelPresent] = true; } } return _isMouseWheelPresent; } } ////// Maps to SM_PENWINDOWS /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Deemed as unsafe /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsPenWindows { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsPenWindows]) { _isPenWindows = UnsafeNativeMethods.GetSystemMetrics(SM.PENWINDOWS) != 0; _cacheValid[(int)CacheSlot.IsPenWindows] = true; } } return _isPenWindows; } } ////// Maps to SM_REMOTECONTROL /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsRemotelyControlled { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsRemotelyControlled]) { _isRemotelyControlled = UnsafeNativeMethods.GetSystemMetrics(SM.REMOTECONTROL) != 0; _cacheValid[(int)CacheSlot.IsRemotelyControlled] = true; } } return _isRemotelyControlled; } } ////// Maps to SM_REMOTESESSION /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demand Unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsRemoteSession { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsRemoteSession]) { _isRemoteSession = UnsafeNativeMethods.GetSystemMetrics(SM.REMOTESESSION) != 0; _cacheValid[(int)CacheSlot.IsRemoteSession] = true; } } return _isRemoteSession; } } ////// Maps to SM_SHOWSOUNDS /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demand Unmanaged Code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool ShowSounds { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.ShowSounds]) { _showSounds = UnsafeNativeMethods.GetSystemMetrics(SM.SHOWSOUNDS) != 0; _cacheValid[(int)CacheSlot.ShowSounds] = true; } } return _showSounds; } } ////// Maps to SM_SLOWMACHINE /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsSlowMachine { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsSlowMachine]) { _isSlowMachine = UnsafeNativeMethods.GetSystemMetrics(SM.SLOWMACHINE) != 0; _cacheValid[(int)CacheSlot.IsSlowMachine] = true; } } return _isSlowMachine; } } ////// Maps to SM_SWAPBUTTON /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool SwapButtons { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.SwapButtons]) { _swapButtons = UnsafeNativeMethods.GetSystemMetrics(SM.SWAPBUTTON) != 0; _cacheValid[(int)CacheSlot.SwapButtons] = true; } } return _swapButtons; } } ////// Maps to SM_TABLETPC /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK -- Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static bool IsTabletPC { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.IsTabletPC]) { _isTabletPC = UnsafeNativeMethods.GetSystemMetrics(SM.TABLETPC) != 0; _cacheValid[(int)CacheSlot.IsTabletPC] = true; } } return _isTabletPC; } } ////// Maps to SM_XVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenLeft { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenLeft]) { _virtualScreenLeft = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.XVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenLeft] = true; } } return _virtualScreenLeft; } } ////// Maps to SM_YVIRTUALSCREEN /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// PublicOK --Demands unmanaged code /// Security Critical -- Calling UnsafeNativeMethods /// public static double VirtualScreenTop { [SecurityCritical] get { SecurityHelper.DemandUnmanagedCode(); lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.VirtualScreenTop]) { _virtualScreenTop = SystemParameters.ConvertPixel(UnsafeNativeMethods.GetSystemMetrics(SM.YVIRTUALSCREEN)); _cacheValid[(int)CacheSlot.VirtualScreenTop] = true; } } return _virtualScreenTop; } } #endregion #region Metrics Keys ////// ThinHorizontalBorderHeight System Resource Key /// public static ResourceKey ThinHorizontalBorderHeightKey { get { if (_cacheThinHorizontalBorderHeight == null) { _cacheThinHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ThinHorizontalBorderHeight); } return _cacheThinHorizontalBorderHeight; } } ////// ThinVerticalBorderWidth System Resource Key /// public static ResourceKey ThinVerticalBorderWidthKey { get { if (_cacheThinVerticalBorderWidth == null) { _cacheThinVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ThinVerticalBorderWidth); } return _cacheThinVerticalBorderWidth; } } ////// CursorWidth System Resource Key /// public static ResourceKey CursorWidthKey { get { if (_cacheCursorWidth == null) { _cacheCursorWidth = CreateInstance(SystemResourceKeyID.CursorWidth); } return _cacheCursorWidth; } } ////// CursorHeight System Resource Key /// public static ResourceKey CursorHeightKey { get { if (_cacheCursorHeight == null) { _cacheCursorHeight = CreateInstance(SystemResourceKeyID.CursorHeight); } return _cacheCursorHeight; } } ////// ThickHorizontalBorderHeight System Resource Key /// public static ResourceKey ThickHorizontalBorderHeightKey { get { if (_cacheThickHorizontalBorderHeight == null) { _cacheThickHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ThickHorizontalBorderHeight); } return _cacheThickHorizontalBorderHeight; } } ////// ThickVerticalBorderWidth System Resource Key /// public static ResourceKey ThickVerticalBorderWidthKey { get { if (_cacheThickVerticalBorderWidth == null) { _cacheThickVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ThickVerticalBorderWidth); } return _cacheThickVerticalBorderWidth; } } ////// FixedFrameHorizontalBorderHeight System Resource Key /// public static ResourceKey FixedFrameHorizontalBorderHeightKey { get { if (_cacheFixedFrameHorizontalBorderHeight == null) { _cacheFixedFrameHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.FixedFrameHorizontalBorderHeight); } return _cacheFixedFrameHorizontalBorderHeight; } } ////// FixedFrameVerticalBorderWidth System Resource Key /// public static ResourceKey FixedFrameVerticalBorderWidthKey { get { if (_cacheFixedFrameVerticalBorderWidth == null) { _cacheFixedFrameVerticalBorderWidth = CreateInstance(SystemResourceKeyID.FixedFrameVerticalBorderWidth); } return _cacheFixedFrameVerticalBorderWidth; } } ////// FocusHorizontalBorderHeight System Resource Key /// public static ResourceKey FocusHorizontalBorderHeightKey { get { if (_cacheFocusHorizontalBorderHeight == null) { _cacheFocusHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.FocusHorizontalBorderHeight); } return _cacheFocusHorizontalBorderHeight; } } ////// FocusVerticalBorderWidth System Resource Key /// public static ResourceKey FocusVerticalBorderWidthKey { get { if (_cacheFocusVerticalBorderWidth == null) { _cacheFocusVerticalBorderWidth = CreateInstance(SystemResourceKeyID.FocusVerticalBorderWidth); } return _cacheFocusVerticalBorderWidth; } } ////// FullPrimaryScreenWidth System Resource Key /// public static ResourceKey FullPrimaryScreenWidthKey { get { if (_cacheFullPrimaryScreenWidth == null) { _cacheFullPrimaryScreenWidth = CreateInstance(SystemResourceKeyID.FullPrimaryScreenWidth); } return _cacheFullPrimaryScreenWidth; } } ////// FullPrimaryScreenHeight System Resource Key /// public static ResourceKey FullPrimaryScreenHeightKey { get { if (_cacheFullPrimaryScreenHeight == null) { _cacheFullPrimaryScreenHeight = CreateInstance(SystemResourceKeyID.FullPrimaryScreenHeight); } return _cacheFullPrimaryScreenHeight; } } ////// HorizontalScrollBarButtonWidth System Resource Key /// public static ResourceKey HorizontalScrollBarButtonWidthKey { get { if (_cacheHorizontalScrollBarButtonWidth == null) { _cacheHorizontalScrollBarButtonWidth = CreateInstance(SystemResourceKeyID.HorizontalScrollBarButtonWidth); } return _cacheHorizontalScrollBarButtonWidth; } } ////// HorizontalScrollBarHeight System Resource Key /// public static ResourceKey HorizontalScrollBarHeightKey { get { if (_cacheHorizontalScrollBarHeight == null) { _cacheHorizontalScrollBarHeight = CreateInstance(SystemResourceKeyID.HorizontalScrollBarHeight); } return _cacheHorizontalScrollBarHeight; } } ////// HorizontalScrollBarThumbWidth System Resource Key /// public static ResourceKey HorizontalScrollBarThumbWidthKey { get { if (_cacheHorizontalScrollBarThumbWidth == null) { _cacheHorizontalScrollBarThumbWidth = CreateInstance(SystemResourceKeyID.HorizontalScrollBarThumbWidth); } return _cacheHorizontalScrollBarThumbWidth; } } ////// IconWidth System Resource Key /// public static ResourceKey IconWidthKey { get { if (_cacheIconWidth == null) { _cacheIconWidth = CreateInstance(SystemResourceKeyID.IconWidth); } return _cacheIconWidth; } } ////// IconHeight System Resource Key /// public static ResourceKey IconHeightKey { get { if (_cacheIconHeight == null) { _cacheIconHeight = CreateInstance(SystemResourceKeyID.IconHeight); } return _cacheIconHeight; } } ////// IconGridWidth System Resource Key /// public static ResourceKey IconGridWidthKey { get { if (_cacheIconGridWidth == null) { _cacheIconGridWidth = CreateInstance(SystemResourceKeyID.IconGridWidth); } return _cacheIconGridWidth; } } ////// IconGridHeight System Resource Key /// public static ResourceKey IconGridHeightKey { get { if (_cacheIconGridHeight == null) { _cacheIconGridHeight = CreateInstance(SystemResourceKeyID.IconGridHeight); } return _cacheIconGridHeight; } } ////// MaximizedPrimaryScreenWidth System Resource Key /// public static ResourceKey MaximizedPrimaryScreenWidthKey { get { if (_cacheMaximizedPrimaryScreenWidth == null) { _cacheMaximizedPrimaryScreenWidth = CreateInstance(SystemResourceKeyID.MaximizedPrimaryScreenWidth); } return _cacheMaximizedPrimaryScreenWidth; } } ////// MaximizedPrimaryScreenHeight System Resource Key /// public static ResourceKey MaximizedPrimaryScreenHeightKey { get { if (_cacheMaximizedPrimaryScreenHeight == null) { _cacheMaximizedPrimaryScreenHeight = CreateInstance(SystemResourceKeyID.MaximizedPrimaryScreenHeight); } return _cacheMaximizedPrimaryScreenHeight; } } ////// MaximumWindowTrackWidth System Resource Key /// public static ResourceKey MaximumWindowTrackWidthKey { get { if (_cacheMaximumWindowTrackWidth == null) { _cacheMaximumWindowTrackWidth = CreateInstance(SystemResourceKeyID.MaximumWindowTrackWidth); } return _cacheMaximumWindowTrackWidth; } } ////// MaximumWindowTrackHeight System Resource Key /// public static ResourceKey MaximumWindowTrackHeightKey { get { if (_cacheMaximumWindowTrackHeight == null) { _cacheMaximumWindowTrackHeight = CreateInstance(SystemResourceKeyID.MaximumWindowTrackHeight); } return _cacheMaximumWindowTrackHeight; } } ////// MenuCheckmarkWidth System Resource Key /// public static ResourceKey MenuCheckmarkWidthKey { get { if (_cacheMenuCheckmarkWidth == null) { _cacheMenuCheckmarkWidth = CreateInstance(SystemResourceKeyID.MenuCheckmarkWidth); } return _cacheMenuCheckmarkWidth; } } ////// MenuCheckmarkHeight System Resource Key /// public static ResourceKey MenuCheckmarkHeightKey { get { if (_cacheMenuCheckmarkHeight == null) { _cacheMenuCheckmarkHeight = CreateInstance(SystemResourceKeyID.MenuCheckmarkHeight); } return _cacheMenuCheckmarkHeight; } } ////// MenuButtonWidth System Resource Key /// public static ResourceKey MenuButtonWidthKey { get { if (_cacheMenuButtonWidth == null) { _cacheMenuButtonWidth = CreateInstance(SystemResourceKeyID.MenuButtonWidth); } return _cacheMenuButtonWidth; } } ////// MenuButtonHeight System Resource Key /// public static ResourceKey MenuButtonHeightKey { get { if (_cacheMenuButtonHeight == null) { _cacheMenuButtonHeight = CreateInstance(SystemResourceKeyID.MenuButtonHeight); } return _cacheMenuButtonHeight; } } ////// MinimumWindowWidth System Resource Key /// public static ResourceKey MinimumWindowWidthKey { get { if (_cacheMinimumWindowWidth == null) { _cacheMinimumWindowWidth = CreateInstance(SystemResourceKeyID.MinimumWindowWidth); } return _cacheMinimumWindowWidth; } } ////// MinimumWindowHeight System Resource Key /// public static ResourceKey MinimumWindowHeightKey { get { if (_cacheMinimumWindowHeight == null) { _cacheMinimumWindowHeight = CreateInstance(SystemResourceKeyID.MinimumWindowHeight); } return _cacheMinimumWindowHeight; } } ////// MinimizedWindowWidth System Resource Key /// public static ResourceKey MinimizedWindowWidthKey { get { if (_cacheMinimizedWindowWidth == null) { _cacheMinimizedWindowWidth = CreateInstance(SystemResourceKeyID.MinimizedWindowWidth); } return _cacheMinimizedWindowWidth; } } ////// MinimizedWindowHeight System Resource Key /// public static ResourceKey MinimizedWindowHeightKey { get { if (_cacheMinimizedWindowHeight == null) { _cacheMinimizedWindowHeight = CreateInstance(SystemResourceKeyID.MinimizedWindowHeight); } return _cacheMinimizedWindowHeight; } } ////// MinimizedGridWidth System Resource Key /// public static ResourceKey MinimizedGridWidthKey { get { if (_cacheMinimizedGridWidth == null) { _cacheMinimizedGridWidth = CreateInstance(SystemResourceKeyID.MinimizedGridWidth); } return _cacheMinimizedGridWidth; } } ////// MinimizedGridHeight System Resource Key /// public static ResourceKey MinimizedGridHeightKey { get { if (_cacheMinimizedGridHeight == null) { _cacheMinimizedGridHeight = CreateInstance(SystemResourceKeyID.MinimizedGridHeight); } return _cacheMinimizedGridHeight; } } ////// MinimumWindowTrackWidth System Resource Key /// public static ResourceKey MinimumWindowTrackWidthKey { get { if (_cacheMinimumWindowTrackWidth == null) { _cacheMinimumWindowTrackWidth = CreateInstance(SystemResourceKeyID.MinimumWindowTrackWidth); } return _cacheMinimumWindowTrackWidth; } } ////// MinimumWindowTrackHeight System Resource Key /// public static ResourceKey MinimumWindowTrackHeightKey { get { if (_cacheMinimumWindowTrackHeight == null) { _cacheMinimumWindowTrackHeight = CreateInstance(SystemResourceKeyID.MinimumWindowTrackHeight); } return _cacheMinimumWindowTrackHeight; } } ////// PrimaryScreenWidth System Resource Key /// public static ResourceKey PrimaryScreenWidthKey { get { if (_cachePrimaryScreenWidth == null) { _cachePrimaryScreenWidth = CreateInstance(SystemResourceKeyID.PrimaryScreenWidth); } return _cachePrimaryScreenWidth; } } ////// PrimaryScreenHeight System Resource Key /// public static ResourceKey PrimaryScreenHeightKey { get { if (_cachePrimaryScreenHeight == null) { _cachePrimaryScreenHeight = CreateInstance(SystemResourceKeyID.PrimaryScreenHeight); } return _cachePrimaryScreenHeight; } } ////// WindowCaptionButtonWidth System Resource Key /// public static ResourceKey WindowCaptionButtonWidthKey { get { if (_cacheWindowCaptionButtonWidth == null) { _cacheWindowCaptionButtonWidth = CreateInstance(SystemResourceKeyID.WindowCaptionButtonWidth); } return _cacheWindowCaptionButtonWidth; } } ////// WindowCaptionButtonHeight System Resource Key /// public static ResourceKey WindowCaptionButtonHeightKey { get { if (_cacheWindowCaptionButtonHeight == null) { _cacheWindowCaptionButtonHeight = CreateInstance(SystemResourceKeyID.WindowCaptionButtonHeight); } return _cacheWindowCaptionButtonHeight; } } ////// ResizeFrameHorizontalBorderHeight System Resource Key /// public static ResourceKey ResizeFrameHorizontalBorderHeightKey { get { if (_cacheResizeFrameHorizontalBorderHeight == null) { _cacheResizeFrameHorizontalBorderHeight = CreateInstance(SystemResourceKeyID.ResizeFrameHorizontalBorderHeight); } return _cacheResizeFrameHorizontalBorderHeight; } } ////// ResizeFrameVerticalBorderWidth System Resource Key /// public static ResourceKey ResizeFrameVerticalBorderWidthKey { get { if (_cacheResizeFrameVerticalBorderWidth == null) { _cacheResizeFrameVerticalBorderWidth = CreateInstance(SystemResourceKeyID.ResizeFrameVerticalBorderWidth); } return _cacheResizeFrameVerticalBorderWidth; } } ////// SmallIconWidth System Resource Key /// public static ResourceKey SmallIconWidthKey { get { if (_cacheSmallIconWidth == null) { _cacheSmallIconWidth = CreateInstance(SystemResourceKeyID.SmallIconWidth); } return _cacheSmallIconWidth; } } ////// SmallIconHeight System Resource Key /// public static ResourceKey SmallIconHeightKey { get { if (_cacheSmallIconHeight == null) { _cacheSmallIconHeight = CreateInstance(SystemResourceKeyID.SmallIconHeight); } return _cacheSmallIconHeight; } } ////// SmallWindowCaptionButtonWidth System Resource Key /// public static ResourceKey SmallWindowCaptionButtonWidthKey { get { if (_cacheSmallWindowCaptionButtonWidth == null) { _cacheSmallWindowCaptionButtonWidth = CreateInstance(SystemResourceKeyID.SmallWindowCaptionButtonWidth); } return _cacheSmallWindowCaptionButtonWidth; } } ////// SmallWindowCaptionButtonHeight System Resource Key /// public static ResourceKey SmallWindowCaptionButtonHeightKey { get { if (_cacheSmallWindowCaptionButtonHeight == null) { _cacheSmallWindowCaptionButtonHeight = CreateInstance(SystemResourceKeyID.SmallWindowCaptionButtonHeight); } return _cacheSmallWindowCaptionButtonHeight; } } ////// VirtualScreenWidth System Resource Key /// public static ResourceKey VirtualScreenWidthKey { get { if (_cacheVirtualScreenWidth == null) { _cacheVirtualScreenWidth = CreateInstance(SystemResourceKeyID.VirtualScreenWidth); } return _cacheVirtualScreenWidth; } } ////// VirtualScreenHeight System Resource Key /// public static ResourceKey VirtualScreenHeightKey { get { if (_cacheVirtualScreenHeight == null) { _cacheVirtualScreenHeight = CreateInstance(SystemResourceKeyID.VirtualScreenHeight); } return _cacheVirtualScreenHeight; } } ////// VerticalScrollBarWidth System Resource Key /// public static ResourceKey VerticalScrollBarWidthKey { get { if (_cacheVerticalScrollBarWidth == null) { _cacheVerticalScrollBarWidth = CreateInstance(SystemResourceKeyID.VerticalScrollBarWidth); } return _cacheVerticalScrollBarWidth; } } ////// VerticalScrollBarButtonHeight System Resource Key /// public static ResourceKey VerticalScrollBarButtonHeightKey { get { if (_cacheVerticalScrollBarButtonHeight == null) { _cacheVerticalScrollBarButtonHeight = CreateInstance(SystemResourceKeyID.VerticalScrollBarButtonHeight); } return _cacheVerticalScrollBarButtonHeight; } } ////// WindowCaptionHeight System Resource Key /// public static ResourceKey WindowCaptionHeightKey { get { if (_cacheWindowCaptionHeight == null) { _cacheWindowCaptionHeight = CreateInstance(SystemResourceKeyID.WindowCaptionHeight); } return _cacheWindowCaptionHeight; } } ////// KanjiWindowHeight System Resource Key /// public static ResourceKey KanjiWindowHeightKey { get { if (_cacheKanjiWindowHeight == null) { _cacheKanjiWindowHeight = CreateInstance(SystemResourceKeyID.KanjiWindowHeight); } return _cacheKanjiWindowHeight; } } ////// MenuBarHeight System Resource Key /// public static ResourceKey MenuBarHeightKey { get { if (_cacheMenuBarHeight == null) { _cacheMenuBarHeight = CreateInstance(SystemResourceKeyID.MenuBarHeight); } return _cacheMenuBarHeight; } } ////// SmallCaptionHeight System Resource Key /// public static ResourceKey SmallCaptionHeightKey { get { if (_cacheSmallCaptionHeight == null) { _cacheSmallCaptionHeight = CreateInstance(SystemResourceKeyID.SmallCaptionHeight); } return _cacheSmallCaptionHeight; } } ////// VerticalScrollBarThumbHeight System Resource Key /// public static ResourceKey VerticalScrollBarThumbHeightKey { get { if (_cacheVerticalScrollBarThumbHeight == null) { _cacheVerticalScrollBarThumbHeight = CreateInstance(SystemResourceKeyID.VerticalScrollBarThumbHeight); } return _cacheVerticalScrollBarThumbHeight; } } ////// IsImmEnabled System Resource Key /// public static ResourceKey IsImmEnabledKey { get { if (_cacheIsImmEnabled == null) { _cacheIsImmEnabled = CreateInstance(SystemResourceKeyID.IsImmEnabled); } return _cacheIsImmEnabled; } } ////// IsMediaCenter System Resource Key /// public static ResourceKey IsMediaCenterKey { get { if (_cacheIsMediaCenter == null) { _cacheIsMediaCenter = CreateInstance(SystemResourceKeyID.IsMediaCenter); } return _cacheIsMediaCenter; } } ////// IsMenuDropRightAligned System Resource Key /// public static ResourceKey IsMenuDropRightAlignedKey { get { if (_cacheIsMenuDropRightAligned == null) { _cacheIsMenuDropRightAligned = CreateInstance(SystemResourceKeyID.IsMenuDropRightAligned); } return _cacheIsMenuDropRightAligned; } } ////// IsMiddleEastEnabled System Resource Key /// public static ResourceKey IsMiddleEastEnabledKey { get { if (_cacheIsMiddleEastEnabled == null) { _cacheIsMiddleEastEnabled = CreateInstance(SystemResourceKeyID.IsMiddleEastEnabled); } return _cacheIsMiddleEastEnabled; } } ////// IsMousePresent System Resource Key /// public static ResourceKey IsMousePresentKey { get { if (_cacheIsMousePresent == null) { _cacheIsMousePresent = CreateInstance(SystemResourceKeyID.IsMousePresent); } return _cacheIsMousePresent; } } ////// IsMouseWheelPresent System Resource Key /// public static ResourceKey IsMouseWheelPresentKey { get { if (_cacheIsMouseWheelPresent == null) { _cacheIsMouseWheelPresent = CreateInstance(SystemResourceKeyID.IsMouseWheelPresent); } return _cacheIsMouseWheelPresent; } } ////// IsPenWindows System Resource Key /// public static ResourceKey IsPenWindowsKey { get { if (_cacheIsPenWindows == null) { _cacheIsPenWindows = CreateInstance(SystemResourceKeyID.IsPenWindows); } return _cacheIsPenWindows; } } ////// IsRemotelyControlled System Resource Key /// public static ResourceKey IsRemotelyControlledKey { get { if (_cacheIsRemotelyControlled == null) { _cacheIsRemotelyControlled = CreateInstance(SystemResourceKeyID.IsRemotelyControlled); } return _cacheIsRemotelyControlled; } } ////// IsRemoteSession System Resource Key /// public static ResourceKey IsRemoteSessionKey { get { if (_cacheIsRemoteSession == null) { _cacheIsRemoteSession = CreateInstance(SystemResourceKeyID.IsRemoteSession); } return _cacheIsRemoteSession; } } ////// ShowSounds System Resource Key /// public static ResourceKey ShowSoundsKey { get { if (_cacheShowSounds == null) { _cacheShowSounds = CreateInstance(SystemResourceKeyID.ShowSounds); } return _cacheShowSounds; } } ////// IsSlowMachine System Resource Key /// public static ResourceKey IsSlowMachineKey { get { if (_cacheIsSlowMachine == null) { _cacheIsSlowMachine = CreateInstance(SystemResourceKeyID.IsSlowMachine); } return _cacheIsSlowMachine; } } ////// SwapButtons System Resource Key /// public static ResourceKey SwapButtonsKey { get { if (_cacheSwapButtons == null) { _cacheSwapButtons = CreateInstance(SystemResourceKeyID.SwapButtons); } return _cacheSwapButtons; } } ////// IsTabletPC System Resource Key /// public static ResourceKey IsTabletPCKey { get { if (_cacheIsTabletPC == null) { _cacheIsTabletPC = CreateInstance(SystemResourceKeyID.IsTabletPC); } return _cacheIsTabletPC; } } ////// VirtualScreenLeft System Resource Key /// public static ResourceKey VirtualScreenLeftKey { get { if (_cacheVirtualScreenLeft == null) { _cacheVirtualScreenLeft = CreateInstance(SystemResourceKeyID.VirtualScreenLeft); } return _cacheVirtualScreenLeft; } } ////// VirtualScreenTop System Resource Key /// public static ResourceKey VirtualScreenTopKey { get { if (_cacheVirtualScreenTop == null) { _cacheVirtualScreenTop = CreateInstance(SystemResourceKeyID.VirtualScreenTop); } return _cacheVirtualScreenTop; } } #endregion #region Theme Style Keys ////// Resource Key for the FocusVisualStyle /// public static ResourceKey FocusVisualStyleKey { get { if (_cacheFocusVisualStyle == null) { _cacheFocusVisualStyle = new SystemThemeKey(SystemResourceKeyID.FocusVisualStyle); } return _cacheFocusVisualStyle; } } ////// Resource Key for the browser window style /// ///public static ResourceKey NavigationChromeStyleKey { get { if (_cacheNavigationChromeStyle == null) { _cacheNavigationChromeStyle = new SystemThemeKey(SystemResourceKeyID.NavigationChromeStyle); } return _cacheNavigationChromeStyle; } } /// /// Resource Key for the down level browser window style /// ///public static ResourceKey NavigationChromeDownLevelStyleKey { get { if (_cacheNavigationChromeDownLevelStyle == null) { _cacheNavigationChromeDownLevelStyle = new SystemThemeKey(SystemResourceKeyID.NavigationChromeDownLevelStyle); } return _cacheNavigationChromeDownLevelStyle; } } #endregion #region Power Parameters /// /// Indicates current Power Status /// ////// Critical as this code elevates. /// PublicOK - as we think this is ok to expose. /// public static PowerLineStatus PowerLineStatus { [SecurityCritical] get { lock (_cacheValid) { if (!_cacheValid[(int)CacheSlot.PowerLineStatus]) { NativeMethods.SYSTEM_POWER_STATUS status = new NativeMethods.SYSTEM_POWER_STATUS(); if (UnsafeNativeMethods.GetSystemPowerStatus(ref status)) { _powerLineStatus = (PowerLineStatus)status.ACLineStatus; _cacheValid[(int)CacheSlot.PowerLineStatus] = true; } else { throw new Win32Exception(); } } } return _powerLineStatus; } } #endregion #region PowerKeys ////// Resource Key for the PowerLineStatus property /// ///public static ResourceKey PowerLineStatusKey { get { if (_cachePowerLineStatus == null) { _cachePowerLineStatus = CreateInstance(SystemResourceKeyID.PowerLineStatus); } return _cachePowerLineStatus; } } #endregion #pragma warning restore 6523 #pragma warning restore 6503 #region Cache and Implementation internal static void InvalidateCache() { // Invalidate all Parameters int[] param = { NativeMethods.SPI_SETFOCUSBORDERWIDTH, NativeMethods.SPI_SETFOCUSBORDERHEIGHT, NativeMethods.SPI_SETHIGHCONTRAST, NativeMethods.SPI_SETMOUSEVANISH, NativeMethods.SPI_SETDROPSHADOW, NativeMethods.SPI_SETFLATMENU, NativeMethods.SPI_SETWORKAREA, NativeMethods.SPI_SETICONMETRICS, NativeMethods.SPI_SETKEYBOARDCUES, NativeMethods.SPI_SETKEYBOARDDELAY, NativeMethods.SPI_SETKEYBOARDPREF, NativeMethods.SPI_SETKEYBOARDSPEED, NativeMethods.SPI_SETSNAPTODEFBUTTON, NativeMethods.SPI_SETWHEELSCROLLLINES, NativeMethods.SPI_SETMOUSEHOVERTIME, NativeMethods.SPI_SETMOUSEHOVERHEIGHT, NativeMethods.SPI_SETMOUSEHOVERWIDTH, NativeMethods.SPI_SETMENUDROPALIGNMENT, NativeMethods.SPI_SETMENUFADE, NativeMethods.SPI_SETMENUSHOWDELAY, NativeMethods.SPI_SETCOMBOBOXANIMATION, NativeMethods.SPI_SETCLIENTAREAANIMATION, NativeMethods.SPI_SETCURSORSHADOW, NativeMethods.SPI_SETGRADIENTCAPTIONS, NativeMethods.SPI_SETHOTTRACKING, NativeMethods.SPI_SETLISTBOXSMOOTHSCROLLING, NativeMethods.SPI_SETMENUANIMATION, NativeMethods.SPI_SETSELECTIONFADE, NativeMethods.SPI_SETSTYLUSHOTTRACKING, NativeMethods.SPI_SETTOOLTIPANIMATION, NativeMethods.SPI_SETTOOLTIPFADE, NativeMethods.SPI_SETUIEFFECTS, NativeMethods.SPI_SETANIMATION, NativeMethods.SPI_SETCARETWIDTH, NativeMethods.SPI_SETFOREGROUNDFLASHCOUNT, NativeMethods.SPI_SETDRAGFULLWINDOWS, NativeMethods.SPI_SETBORDER, NativeMethods.SPI_SETNONCLIENTMETRICS, NativeMethods.SPI_SETDRAGWIDTH, NativeMethods.SPI_SETDRAGHEIGHT, NativeMethods.SPI_SETPENWINDOWS, NativeMethods.SPI_SETSHOWSOUNDS, NativeMethods.SPI_SETMOUSEBUTTONSWAP}; for (int i = 0; i < param.Length; i++) { InvalidateCache(param[i]); } } internal static bool InvalidateDeviceDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMousePresent)) changed |= _isMousePresent != IsMousePresent; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMouseWheelPresent)) changed |= _isMousePresent != IsMousePresent; return changed; } internal static bool InvalidateDisplayDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WorkAreaInternal)) { NativeMethods.RECT oldRect = _workAreaInternal; NativeMethods.RECT newRect = WorkAreaInternal; changed |= oldRect.left != newRect.left; changed |= oldRect.top != newRect.top; changed |= oldRect.right != newRect.right; changed |= oldRect.bottom != newRect.bottom; } if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WorkArea)) changed |= _workArea != WorkArea; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FullPrimaryScreenWidth)) changed |= _fullPrimaryScreenWidth != FullPrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FullPrimaryScreenHeight)) changed |= _fullPrimaryScreenHeight != FullPrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximizedPrimaryScreenWidth)) changed |= _maximizedPrimaryScreenWidth != MaximizedPrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximizedPrimaryScreenHeight)) changed |= _maximizedPrimaryScreenHeight != MaximizedPrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PrimaryScreenWidth)) changed |= _primaryScreenWidth != PrimaryScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PrimaryScreenHeight)) changed |= _primaryScreenHeight != PrimaryScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenWidth)) changed |= _virtualScreenWidth != VirtualScreenWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenHeight)) changed |= _virtualScreenHeight != VirtualScreenHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenLeft)) changed |= _virtualScreenLeft != VirtualScreenLeft; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VirtualScreenTop)) changed |= _virtualScreenTop != VirtualScreenTop; return changed; } internal static bool InvalidatePowerDependentCache() { bool changed = false; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.PowerLineStatus)) changed |= _powerLineStatus != PowerLineStatus; return changed; } internal static bool InvalidateCache(int param) { // FxCop: Hashtable of callbacks would not be performant, using switch instead switch (param) { case NativeMethods.SPI_SETFOCUSBORDERWIDTH: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusBorderWidth); // Invalidate SystemMetrics if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusHorizontalBorderHeight)) changed |= _focusHorizontalBorderHeight != FocusHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusVerticalBorderWidth)) changed |= _focusVerticalBorderWidth != FocusVerticalBorderWidth; return changed; } case NativeMethods.SPI_SETFOCUSBORDERHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FocusBorderHeight); case NativeMethods.SPI_SETHIGHCONTRAST: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HighContrast); case NativeMethods.SPI_SETMOUSEVANISH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseVanish); case NativeMethods.SPI_SETDROPSHADOW: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.DropShadow); case NativeMethods.SPI_SETFLATMENU: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FlatMenu); case NativeMethods.SPI_SETWORKAREA: return InvalidateDisplayDependentCache(); case NativeMethods.SPI_SETICONMETRICS: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconMetrics); if (changed) { SystemFonts.InvalidateIconMetrics(); } if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconWidth)) changed |= _iconWidth != IconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconHeight)) changed |= _iconHeight != IconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridWidth)) changed |= _iconGridWidth != IconGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridHeight)) changed |= _iconGridHeight != IconGridHeight; return changed; } case NativeMethods.SPI_SETKEYBOARDCUES: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardCues); case NativeMethods.SPI_SETKEYBOARDDELAY: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardDelay); case NativeMethods.SPI_SETKEYBOARDPREF: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardPreference); case NativeMethods.SPI_SETKEYBOARDSPEED: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.KeyboardSpeed); case NativeMethods.SPI_SETSNAPTODEFBUTTON: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SnapToDefaultButton); case NativeMethods.SPI_SETWHEELSCROLLLINES: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WheelScrollLines); case NativeMethods.SPI_SETMOUSEHOVERTIME: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverTime); case NativeMethods.SPI_SETMOUSEHOVERHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverHeight); case NativeMethods.SPI_SETMOUSEHOVERWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MouseHoverWidth); case NativeMethods.SPI_SETMENUDROPALIGNMENT: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuDropAlignment); if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsMenuDropRightAligned)) changed |= _isMenuDropRightAligned != IsMenuDropRightAligned; return changed; } case NativeMethods.SPI_SETMENUFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuFade); case NativeMethods.SPI_SETMENUSHOWDELAY: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuShowDelay); case NativeMethods.SPI_SETCOMBOBOXANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ComboBoxAnimation); case NativeMethods.SPI_SETCLIENTAREAANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ClientAreaAnimation); case NativeMethods.SPI_SETCURSORSHADOW: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorShadow); case NativeMethods.SPI_SETGRADIENTCAPTIONS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.GradientCaptions); case NativeMethods.SPI_SETHOTTRACKING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HotTracking); case NativeMethods.SPI_SETLISTBOXSMOOTHSCROLLING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ListBoxSmoothScrolling); case NativeMethods.SPI_SETMENUANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuAnimation); case NativeMethods.SPI_SETSELECTIONFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SelectionFade); case NativeMethods.SPI_SETSTYLUSHOTTRACKING: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.StylusHotTracking); case NativeMethods.SPI_SETTOOLTIPANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ToolTipAnimation); case NativeMethods.SPI_SETTOOLTIPFADE: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ToolTipFade); case NativeMethods.SPI_SETUIEFFECTS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.UIEffects); case NativeMethods.SPI_SETANIMATION: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizeAnimation); case NativeMethods.SPI_SETCARETWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CaretWidth); case NativeMethods.SPI_SETFOREGROUNDFLASHCOUNT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ForegroundFlashCount); case NativeMethods.SPI_SETDRAGFULLWINDOWS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.DragFullWindows); case NativeMethods.SPI_SETBORDER: case NativeMethods.SPI_SETNONCLIENTMETRICS: { bool changed = SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.NonClientMetrics); if (changed) { SystemFonts.InvalidateNonClientMetrics(); } changed |= SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.Border); // Invalidate SystemMetrics if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThinHorizontalBorderHeight)) changed |= _thinHorizontalBorderHeight != ThinHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThinVerticalBorderWidth)) changed |= _thinVerticalBorderWidth != ThinVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorWidth)) changed |= _cursorWidth != CursorWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.CursorHeight)) changed |= _cursorHeight != CursorHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThickHorizontalBorderHeight)) changed |= _thickHorizontalBorderHeight != ThickHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ThickVerticalBorderWidth)) changed |= _thickVerticalBorderWidth != ThickVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FixedFrameHorizontalBorderHeight)) changed |= _fixedFrameHorizontalBorderHeight != FixedFrameHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.FixedFrameVerticalBorderWidth)) changed |= _fixedFrameVerticalBorderWidth != FixedFrameVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarButtonWidth)) changed |= _horizontalScrollBarButtonWidth != HorizontalScrollBarButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarHeight)) changed |= _horizontalScrollBarHeight != HorizontalScrollBarHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.HorizontalScrollBarThumbWidth)) changed |= _horizontalScrollBarThumbWidth != HorizontalScrollBarThumbWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconWidth)) changed |= _iconWidth != IconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconHeight)) changed |= _iconHeight != IconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridWidth)) changed |= _iconGridWidth != IconGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IconGridHeight)) changed |= _iconGridHeight != IconGridHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximumWindowTrackWidth)) changed |= _maximumWindowTrackWidth != MaximumWindowTrackWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MaximumWindowTrackHeight)) changed |= _maximumWindowTrackHeight != MaximumWindowTrackHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuCheckmarkWidth)) changed |= _menuCheckmarkWidth != MenuCheckmarkWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuCheckmarkHeight)) changed |= _menuCheckmarkHeight != MenuCheckmarkHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuButtonWidth)) changed |= _menuButtonWidth != MenuButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuButtonHeight)) changed |= _menuButtonHeight != MenuButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowWidth)) changed |= _minimumWindowWidth != MinimumWindowWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowHeight)) changed |= _minimumWindowHeight != MinimumWindowHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedWindowWidth)) changed |= _minimizedWindowWidth != MinimizedWindowWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedWindowHeight)) changed |= _minimizedWindowHeight != MinimizedWindowHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedGridWidth)) changed |= _minimizedGridWidth != MinimizedGridWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimizedGridHeight)) changed |= _minimizedGridHeight != MinimizedGridHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowTrackWidth)) changed |= _minimumWindowTrackWidth != MinimumWindowTrackWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumWindowTrackHeight)) changed |= _minimumWindowTrackHeight != MinimumWindowTrackHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionButtonWidth)) changed |= _windowCaptionButtonWidth != WindowCaptionButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionButtonHeight)) changed |= _windowCaptionButtonHeight != WindowCaptionButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ResizeFrameHorizontalBorderHeight)) changed |= _resizeFrameHorizontalBorderHeight != ResizeFrameHorizontalBorderHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ResizeFrameVerticalBorderWidth)) changed |= _resizeFrameVerticalBorderWidth != ResizeFrameVerticalBorderWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallIconWidth)) changed |= _smallIconWidth != SmallIconWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallIconHeight)) changed |= _smallIconHeight != SmallIconHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallWindowCaptionButtonWidth)) changed |= _smallWindowCaptionButtonWidth != SmallWindowCaptionButtonWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SmallWindowCaptionButtonHeight)) changed |= _smallWindowCaptionButtonHeight != SmallWindowCaptionButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarWidth)) changed |= _verticalScrollBarWidth != VerticalScrollBarWidth; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarButtonHeight)) changed |= _verticalScrollBarButtonHeight != VerticalScrollBarButtonHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.WindowCaptionHeight)) changed |= _windowCaptionHeight != WindowCaptionHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MenuBarHeight)) changed |= _menuBarHeight != MenuBarHeight; if (SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.VerticalScrollBarThumbHeight)) changed |= _verticalScrollBarThumbHeight != VerticalScrollBarThumbHeight; changed |= InvalidateDisplayDependentCache(); return changed; } case NativeMethods.SPI_SETDRAGWIDTH: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumHorizontalDragDistance); case NativeMethods.SPI_SETDRAGHEIGHT: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.MinimumVerticalDragDistance); case NativeMethods.SPI_SETPENWINDOWS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.IsPenWindows); case NativeMethods.SPI_SETSHOWSOUNDS: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.ShowSounds); case NativeMethods.SPI_SETMOUSEBUTTONSWAP: return SystemResources.ClearSlot(_cacheValid, (int)CacheSlot.SwapButtons); } return false; } internal static int Dpi { get { return MS.Internal.FontCache.Util.Dpi; } } /// /// Critical as this accesses Native methods. /// TreatAsSafe - it would be ok to expose this information - DPI in partial trust /// internal static int DpiX { [SecurityCritical, SecurityTreatAsSafe] get { if (_setDpiX) { lock (_cacheValid) { if (_setDpiX) { _setDpiX = false; HandleRef desktopWnd = new HandleRef(null, IntPtr.Zero); // Win32Exception will get the Win32 error code so we don't have to #pragma warning disable 6523 IntPtr dc = UnsafeNativeMethods.GetDC(desktopWnd); // Detecting error case from unmanaged call, required by PREsharp to throw a Win32Exception #pragma warning disable 6503 if (dc == IntPtr.Zero) { throw new Win32Exception(); } #pragma warning restore 6503 #pragma warning restore 6523 try { _dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(null, dc), NativeMethods.LOGPIXELSX); _cacheValid[(int)CacheSlot.DpiX] = true; } finally { UnsafeNativeMethods.ReleaseDC(desktopWnd, new HandleRef(null, dc)); } } } } return _dpiX; } } internal static double ConvertPixel(int pixel) { int dpi = Dpi; if (dpi != 0) { return (double)pixel * 96 / dpi; } return pixel; } private enum CacheSlot : int { DpiX, FocusBorderWidth, FocusBorderHeight, HighContrast, MouseVanish, DropShadow, FlatMenu, WorkAreaInternal, WorkArea, IconMetrics, KeyboardCues, KeyboardDelay, KeyboardPreference, KeyboardSpeed, SnapToDefaultButton, WheelScrollLines, MouseHoverTime, MouseHoverHeight, MouseHoverWidth, MenuDropAlignment, MenuFade, MenuShowDelay, ComboBoxAnimation, ClientAreaAnimation, CursorShadow, GradientCaptions, HotTracking, ListBoxSmoothScrolling, MenuAnimation, SelectionFade, StylusHotTracking, ToolTipAnimation, ToolTipFade, UIEffects, MinimizeAnimation, Border, CaretWidth, ForegroundFlashCount, DragFullWindows, NonClientMetrics, ThinHorizontalBorderHeight, ThinVerticalBorderWidth, CursorWidth, CursorHeight, ThickHorizontalBorderHeight, ThickVerticalBorderWidth, MinimumHorizontalDragDistance, MinimumVerticalDragDistance, FixedFrameHorizontalBorderHeight, FixedFrameVerticalBorderWidth, FocusHorizontalBorderHeight, FocusVerticalBorderWidth, FullPrimaryScreenWidth, FullPrimaryScreenHeight, HorizontalScrollBarButtonWidth, HorizontalScrollBarHeight, HorizontalScrollBarThumbWidth, IconWidth, IconHeight, IconGridWidth, IconGridHeight, MaximizedPrimaryScreenWidth, MaximizedPrimaryScreenHeight, MaximumWindowTrackWidth, MaximumWindowTrackHeight, MenuCheckmarkWidth, MenuCheckmarkHeight, MenuButtonWidth, MenuButtonHeight, MinimumWindowWidth, MinimumWindowHeight, MinimizedWindowWidth, MinimizedWindowHeight, MinimizedGridWidth, MinimizedGridHeight, MinimumWindowTrackWidth, MinimumWindowTrackHeight, PrimaryScreenWidth, PrimaryScreenHeight, WindowCaptionButtonWidth, WindowCaptionButtonHeight, ResizeFrameHorizontalBorderHeight, ResizeFrameVerticalBorderWidth, SmallIconWidth, SmallIconHeight, SmallWindowCaptionButtonWidth, SmallWindowCaptionButtonHeight, VirtualScreenWidth, VirtualScreenHeight, VerticalScrollBarWidth, VerticalScrollBarButtonHeight, WindowCaptionHeight, KanjiWindowHeight, MenuBarHeight, VerticalScrollBarThumbHeight, IsImmEnabled, IsMediaCenter, IsMenuDropRightAligned, IsMiddleEastEnabled, IsMousePresent, IsMouseWheelPresent, IsPenWindows, IsRemotelyControlled, IsRemoteSession, ShowSounds, IsSlowMachine, SwapButtons, IsTabletPC, VirtualScreenLeft, VirtualScreenTop, PowerLineStatus, NumSlots } private static BitArray _cacheValid = new BitArray((int)CacheSlot.NumSlots); private static int _dpiX; private static bool _setDpiX = true; private static double _focusBorderWidth; private static double _focusBorderHeight; private static bool _highContrast; private static bool _mouseVanish; private static bool _dropShadow; private static bool _flatMenu; private static NativeMethods.RECT _workAreaInternal; private static Rect _workArea; private static NativeMethods.ICONMETRICS _iconMetrics; private static bool _keyboardCues; private static int _keyboardDelay; private static bool _keyboardPref; private static int _keyboardSpeed; private static bool _snapToDefButton; private static int _wheelScrollLines; private static int _mouseHoverTime; private static double _mouseHoverHeight; private static double _mouseHoverWidth; private static bool _menuDropAlignment; private static bool _menuFade; private static int _menuShowDelay; private static bool _comboBoxAnimation; private static bool _clientAreaAnimation; private static bool _cursorShadow; private static bool _gradientCaptions; private static bool _hotTracking; private static bool _listBoxSmoothScrolling; private static bool _menuAnimation; private static bool _selectionFade; private static bool _stylusHotTracking; private static bool _toolTipAnimation; private static bool _tooltipFade; private static bool _uiEffects; private static bool _minAnimation; private static int _border; private static double _caretWidth; private static bool _dragFullWindows; private static int _foregroundFlashCount; private static NativeMethods.NONCLIENTMETRICS _ncm; private static double _thinHorizontalBorderHeight; private static double _thinVerticalBorderWidth; private static double _cursorWidth; private static double _cursorHeight; private static double _thickHorizontalBorderHeight; private static double _thickVerticalBorderWidth; private static double _minimumHorizontalDragDistance; private static double _minimumVerticalDragDistance; private static double _fixedFrameHorizontalBorderHeight; private static double _fixedFrameVerticalBorderWidth; private static double _focusHorizontalBorderHeight; private static double _focusVerticalBorderWidth; private static double _fullPrimaryScreenHeight; private static double _fullPrimaryScreenWidth; private static double _horizontalScrollBarHeight; private static double _horizontalScrollBarButtonWidth; private static double _horizontalScrollBarThumbWidth; private static double _iconWidth; private static double _iconHeight; private static double _iconGridWidth; private static double _iconGridHeight; private static double _maximizedPrimaryScreenWidth; private static double _maximizedPrimaryScreenHeight; private static double _maximumWindowTrackWidth; private static double _maximumWindowTrackHeight; private static double _menuCheckmarkWidth; private static double _menuCheckmarkHeight; private static double _menuButtonWidth; private static double _menuButtonHeight; private static double _minimumWindowWidth; private static double _minimumWindowHeight; private static double _minimizedWindowWidth; private static double _minimizedWindowHeight; private static double _minimizedGridWidth; private static double _minimizedGridHeight; private static double _minimumWindowTrackWidth; private static double _minimumWindowTrackHeight; private static double _primaryScreenWidth; private static double _primaryScreenHeight; private static double _windowCaptionButtonWidth; private static double _windowCaptionButtonHeight; private static double _resizeFrameHorizontalBorderHeight; private static double _resizeFrameVerticalBorderWidth; private static double _smallIconWidth; private static double _smallIconHeight; private static double _smallWindowCaptionButtonWidth; private static double _smallWindowCaptionButtonHeight; private static double _virtualScreenWidth; private static double _virtualScreenHeight; private static double _verticalScrollBarWidth; private static double _verticalScrollBarButtonHeight; private static double _windowCaptionHeight; private static double _kanjiWindowHeight; private static double _menuBarHeight; private static double _verticalScrollBarThumbHeight; private static bool _isImmEnabled; private static bool _isMediaCenter; private static bool _isMenuDropRightAligned; private static bool _isMiddleEastEnabled; private static bool _isMousePresent; private static bool _isMouseWheelPresent; private static bool _isPenWindows; private static bool _isRemotelyControlled; private static bool _isRemoteSession; private static bool _showSounds; private static bool _isSlowMachine; private static bool _swapButtons; private static bool _isTabletPC; private static double _virtualScreenLeft; private static double _virtualScreenTop; private static PowerLineStatus _powerLineStatus; private static SystemResourceKey _cacheThinHorizontalBorderHeight; private static SystemResourceKey _cacheThinVerticalBorderWidth; private static SystemResourceKey _cacheCursorWidth; private static SystemResourceKey _cacheCursorHeight; private static SystemResourceKey _cacheThickHorizontalBorderHeight; private static SystemResourceKey _cacheThickVerticalBorderWidth; private static SystemResourceKey _cacheFixedFrameHorizontalBorderHeight; private static SystemResourceKey _cacheFixedFrameVerticalBorderWidth; private static SystemResourceKey _cacheFocusHorizontalBorderHeight; private static SystemResourceKey _cacheFocusVerticalBorderWidth; private static SystemResourceKey _cacheFullPrimaryScreenWidth; private static SystemResourceKey _cacheFullPrimaryScreenHeight; private static SystemResourceKey _cacheHorizontalScrollBarButtonWidth; private static SystemResourceKey _cacheHorizontalScrollBarHeight; private static SystemResourceKey _cacheHorizontalScrollBarThumbWidth; private static SystemResourceKey _cacheIconWidth; private static SystemResourceKey _cacheIconHeight; private static SystemResourceKey _cacheIconGridWidth; private static SystemResourceKey _cacheIconGridHeight; private static SystemResourceKey _cacheMaximizedPrimaryScreenWidth; private static SystemResourceKey _cacheMaximizedPrimaryScreenHeight; private static SystemResourceKey _cacheMaximumWindowTrackWidth; private static SystemResourceKey _cacheMaximumWindowTrackHeight; private static SystemResourceKey _cacheMenuCheckmarkWidth; private static SystemResourceKey _cacheMenuCheckmarkHeight; private static SystemResourceKey _cacheMenuButtonWidth; private static SystemResourceKey _cacheMenuButtonHeight; private static SystemResourceKey _cacheMinimumWindowWidth; private static SystemResourceKey _cacheMinimumWindowHeight; private static SystemResourceKey _cacheMinimizedWindowWidth; private static SystemResourceKey _cacheMinimizedWindowHeight; private static SystemResourceKey _cacheMinimizedGridWidth; private static SystemResourceKey _cacheMinimizedGridHeight; private static SystemResourceKey _cacheMinimumWindowTrackWidth; private static SystemResourceKey _cacheMinimumWindowTrackHeight; private static SystemResourceKey _cachePrimaryScreenWidth; private static SystemResourceKey _cachePrimaryScreenHeight; private static SystemResourceKey _cacheWindowCaptionButtonWidth; private static SystemResourceKey _cacheWindowCaptionButtonHeight; private static SystemResourceKey _cacheResizeFrameHorizontalBorderHeight; private static SystemResourceKey _cacheResizeFrameVerticalBorderWidth; private static SystemResourceKey _cacheSmallIconWidth; private static SystemResourceKey _cacheSmallIconHeight; private static SystemResourceKey _cacheSmallWindowCaptionButtonWidth; private static SystemResourceKey _cacheSmallWindowCaptionButtonHeight; private static SystemResourceKey _cacheVirtualScreenWidth; private static SystemResourceKey _cacheVirtualScreenHeight; private static SystemResourceKey _cacheVerticalScrollBarWidth; private static SystemResourceKey _cacheVerticalScrollBarButtonHeight; private static SystemResourceKey _cacheWindowCaptionHeight; private static SystemResourceKey _cacheKanjiWindowHeight; private static SystemResourceKey _cacheMenuBarHeight; private static SystemResourceKey _cacheSmallCaptionHeight; private static SystemResourceKey _cacheVerticalScrollBarThumbHeight; private static SystemResourceKey _cacheIsImmEnabled; private static SystemResourceKey _cacheIsMediaCenter; private static SystemResourceKey _cacheIsMenuDropRightAligned; private static SystemResourceKey _cacheIsMiddleEastEnabled; private static SystemResourceKey _cacheIsMousePresent; private static SystemResourceKey _cacheIsMouseWheelPresent; private static SystemResourceKey _cacheIsPenWindows; private static SystemResourceKey _cacheIsRemotelyControlled; private static SystemResourceKey _cacheIsRemoteSession; private static SystemResourceKey _cacheShowSounds; private static SystemResourceKey _cacheIsSlowMachine; private static SystemResourceKey _cacheSwapButtons; private static SystemResourceKey _cacheIsTabletPC; private static SystemResourceKey _cacheVirtualScreenLeft; private static SystemResourceKey _cacheVirtualScreenTop; private static SystemResourceKey _cacheFocusBorderWidth; private static SystemResourceKey _cacheFocusBorderHeight; private static SystemResourceKey _cacheHighContrast; private static SystemResourceKey _cacheDropShadow; private static SystemResourceKey _cacheFlatMenu; private static SystemResourceKey _cacheWorkArea; private static SystemResourceKey _cacheIconHorizontalSpacing; private static SystemResourceKey _cacheIconVerticalSpacing; private static SystemResourceKey _cacheIconTitleWrap; private static SystemResourceKey _cacheKeyboardCues; private static SystemResourceKey _cacheKeyboardDelay; private static SystemResourceKey _cacheKeyboardPreference; private static SystemResourceKey _cacheKeyboardSpeed; private static SystemResourceKey _cacheSnapToDefaultButton; private static SystemResourceKey _cacheWheelScrollLines; private static SystemResourceKey _cacheMouseHoverTime; private static SystemResourceKey _cacheMouseHoverHeight; private static SystemResourceKey _cacheMouseHoverWidth; private static SystemResourceKey _cacheMenuDropAlignment; private static SystemResourceKey _cacheMenuFade; private static SystemResourceKey _cacheMenuShowDelay; private static SystemResourceKey _cacheComboBoxAnimation; private static SystemResourceKey _cacheClientAreaAnimation; private static SystemResourceKey _cacheCursorShadow; private static SystemResourceKey _cacheGradientCaptions; private static SystemResourceKey _cacheHotTracking; private static SystemResourceKey _cacheListBoxSmoothScrolling; private static SystemResourceKey _cacheMenuAnimation; private static SystemResourceKey _cacheSelectionFade; private static SystemResourceKey _cacheStylusHotTracking; private static SystemResourceKey _cacheToolTipAnimation; private static SystemResourceKey _cacheToolTipFade; private static SystemResourceKey _cacheUIEffects; private static SystemResourceKey _cacheMinimizeAnimation; private static SystemResourceKey _cacheBorder; private static SystemResourceKey _cacheCaretWidth; private static SystemResourceKey _cacheForegroundFlashCount; private static SystemResourceKey _cacheDragFullWindows; private static SystemResourceKey _cacheBorderWidth; private static SystemResourceKey _cacheScrollWidth; private static SystemResourceKey _cacheScrollHeight; private static SystemResourceKey _cacheCaptionWidth; private static SystemResourceKey _cacheCaptionHeight; private static SystemResourceKey _cacheSmallCaptionWidth; private static SystemResourceKey _cacheMenuWidth; private static SystemResourceKey _cacheMenuHeight; private static SystemResourceKey _cacheComboBoxPopupAnimation; private static SystemResourceKey _cacheMenuPopupAnimation; private static SystemResourceKey _cacheToolTipPopupAnimation; private static SystemResourceKey _cachePowerLineStatus; private static SystemThemeKey _cacheFocusVisualStyle; private static SystemThemeKey _cacheNavigationChromeStyle; private static SystemThemeKey _cacheNavigationChromeDownLevelStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
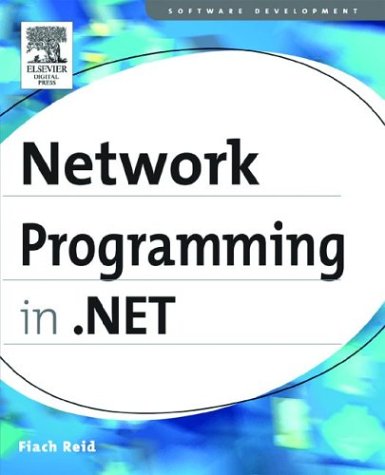
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlTemplateSerializer.cs
- SignatureHelper.cs
- WrappedIUnknown.cs
- CodeAccessSecurityEngine.cs
- GridView.cs
- TextDpi.cs
- LocalTransaction.cs
- HttpServerUtilityBase.cs
- Roles.cs
- ValidatedControlConverter.cs
- DbParameterCollectionHelper.cs
- SoapIncludeAttribute.cs
- Facet.cs
- ErrorRuntimeConfig.cs
- TextParaLineResult.cs
- TrackBar.cs
- GeneralTransformGroup.cs
- HighlightVisual.cs
- VariableBinder.cs
- RawUIStateInputReport.cs
- ChainedAsyncResult.cs
- EntityModelBuildProvider.cs
- BamlLocalizableResource.cs
- BitmapEffectInput.cs
- DiscoveryDocument.cs
- DeflateEmulationStream.cs
- CryptoApi.cs
- RMPermissions.cs
- Privilege.cs
- OdbcInfoMessageEvent.cs
- SmtpFailedRecipientException.cs
- PropVariant.cs
- CompatibleIComparer.cs
- NumberAction.cs
- TracedNativeMethods.cs
- HandlerElementCollection.cs
- DoWorkEventArgs.cs
- GACIdentityPermission.cs
- TypeLibraryHelper.cs
- JsonReaderWriterFactory.cs
- TagMapCollection.cs
- ConfigurationSchemaErrors.cs
- DataColumnCollection.cs
- DesignerObject.cs
- SecurityAlgorithmSuiteConverter.cs
- TextTreeRootTextBlock.cs
- HitTestWithPointDrawingContextWalker.cs
- FixedSchema.cs
- HttpApplicationFactory.cs
- MachineKeySection.cs
- Int32Converter.cs
- CardSpacePolicyElement.cs
- WebServiceHandlerFactory.cs
- CultureTable.cs
- SynchronizedDispatch.cs
- SourceFileBuildProvider.cs
- XmlChildNodes.cs
- ArgumentOutOfRangeException.cs
- CodeAccessSecurityEngine.cs
- CheckBoxPopupAdapter.cs
- TypeToken.cs
- XmlSchemaFacet.cs
- NotSupportedException.cs
- Constraint.cs
- FastPropertyAccessor.cs
- Item.cs
- VariableModifiersHelper.cs
- MimeMapping.cs
- ToolboxItem.cs
- FileChangesMonitor.cs
- QueryCreatedEventArgs.cs
- X509Extension.cs
- DrawingVisualDrawingContext.cs
- XmlLoader.cs
- UIElementIsland.cs
- LinkClickEvent.cs
- PanelDesigner.cs
- smtppermission.cs
- FontNamesConverter.cs
- SqlFlattener.cs
- SocketPermission.cs
- WebPartManager.cs
- SqlRemoveConstantOrderBy.cs
- QilChoice.cs
- StateItem.cs
- SqlError.cs
- XmlQueryStaticData.cs
- PtsCache.cs
- ExpandCollapseProviderWrapper.cs
- XPathNodeInfoAtom.cs
- TabletDevice.cs
- ApplicationCommands.cs
- LayoutInformation.cs
- IdnElement.cs
- CachedFontFamily.cs
- InvalidDataContractException.cs
- TransportReplyChannelAcceptor.cs
- HighlightVisual.cs
- ContextStack.cs
- DynamicQueryableWrapper.cs