Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Packaging / DeflateEmulationStream.cs / 1 / DeflateEmulationStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
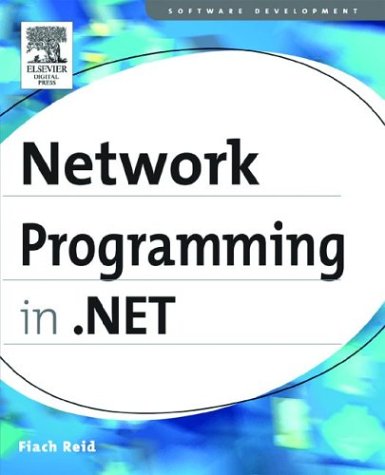
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Style.cs
- RangeValidator.cs
- BindingSource.cs
- TCPListener.cs
- KeyFrames.cs
- ColumnWidthChangingEvent.cs
- RichTextBoxAutomationPeer.cs
- ExclusiveTcpTransportManager.cs
- XmlTextReaderImplHelpers.cs
- Internal.cs
- UriExt.cs
- ValidatingCollection.cs
- CustomGrammar.cs
- SecurityIdentifierElementCollection.cs
- ModelItemCollectionImpl.cs
- BitStream.cs
- DropTarget.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ReadWriteObjectLock.cs
- CodeArrayIndexerExpression.cs
- JsonServiceDocumentSerializer.cs
- ValueSerializerAttribute.cs
- ItemsPanelTemplate.cs
- EastAsianLunisolarCalendar.cs
- ImportContext.cs
- ProfileWorkflowElement.cs
- ReadOnlyTernaryTree.cs
- ConfigurationFileMap.cs
- _ConnectionGroup.cs
- HttpApplication.cs
- WinHttpWebProxyFinder.cs
- BindingNavigator.cs
- DecoderReplacementFallback.cs
- AtlasWeb.Designer.cs
- DataGridViewCellLinkedList.cs
- LinkButton.cs
- XpsLiterals.cs
- AsyncContentLoadedEventArgs.cs
- TagNameToTypeMapper.cs
- FlowchartSizeFeature.cs
- PrintingPermission.cs
- XmlSerializerAssemblyAttribute.cs
- ByteRangeDownloader.cs
- BufferedResponseStream.cs
- FreezableCollection.cs
- TimeManager.cs
- DesignerPerfEventProvider.cs
- DataObjectEventArgs.cs
- ServiceHttpHandlerFactory.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- EndOfStreamException.cs
- DbConnectionInternal.cs
- PrintDocument.cs
- ScrollPattern.cs
- IResourceProvider.cs
- _RequestCacheProtocol.cs
- CorrelationRequestContext.cs
- JpegBitmapEncoder.cs
- ProfilePropertySettings.cs
- IndexedString.cs
- DynamicRenderer.cs
- UriParserTemplates.cs
- TimeSpanValidator.cs
- ToolStripDropDownMenu.cs
- Section.cs
- EdmItemCollection.OcAssemblyCache.cs
- XmlQueryContext.cs
- ResourceExpressionEditor.cs
- StrokeFIndices.cs
- MethodBuilder.cs
- SimpleNameService.cs
- DesignerProperties.cs
- RegistryDataKey.cs
- WebEventTraceProvider.cs
- GeometryHitTestParameters.cs
- ProcessThread.cs
- BindingMAnagerBase.cs
- Constants.cs
- Membership.cs
- HyperLink.cs
- SQLDateTime.cs
- PrivilegeNotHeldException.cs
- OdbcConnectionHandle.cs
- ScriptHandlerFactory.cs
- ScaleTransform.cs
- ResourceManagerWrapper.cs
- TableRow.cs
- XPathChildIterator.cs
- CodeParameterDeclarationExpressionCollection.cs
- CallbackValidator.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- PropertyMetadata.cs
- ClientFormsAuthenticationCredentials.cs
- DynamicDocumentPaginator.cs
- RelatedImageListAttribute.cs
- PeerUnsafeNativeMethods.cs
- ToolboxComponentsCreatedEventArgs.cs
- HttpProfileBase.cs
- XmlSignificantWhitespace.cs