Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / Inline.cs / 1 / Inline.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Inline element. // // History: // 06/06/2002 : MikeOrr - Created. // 07/10/2002 : MikeOrr - Renamed element/class 'Phrase' -> 'Inline'. // 06/25/2003 : ZhenbinX - Ported to /Rewrote for WCP tree // 10/28/2004 : grzegorz - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; namespace System.Windows.Documents { ////// Inline element. /// [TextElementEditingBehaviorAttribute(IsMergeable = true, IsTypographicOnly = true)] public abstract class Inline : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Public constructor. /// protected Inline() : base() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Inlines containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public InlineCollection SiblingInlines { get { if (this.Parent == null) { return null; } return new InlineCollection(this, /*isOwnerParent*/false); } } ////// Returns an Inline immediately following this one /// on the same level of siblings /// public Inline NextInline { get { return this.NextElement as Inline; } } ////// Returns an Inline immediately preceding this one /// on the same level of siblings /// public Inline PreviousInline { get { return this.PreviousElement as Inline; } } ////// DependencyProperty for public static readonly DependencyProperty BaselineAlignmentProperty = DependencyProperty.Register( "BaselineAlignment", typeof(BaselineAlignment), typeof(Inline), new FrameworkPropertyMetadata( BaselineAlignment.Baseline, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidBaselineAlignment)); ///property. /// /// /// public BaselineAlignment BaselineAlignment { get { return (BaselineAlignment) GetValue(BaselineAlignmentProperty); } set { SetValue(BaselineAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = DependencyProperty.Register( "TextDecorations", typeof(TextDecorationCollection), typeof(Inline), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Inline)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal static Run CreateImplicitRun(DependencyObject parent) { return new Run(); } internal static InlineUIContainer CreateImplicitInlineUIContainer(DependencyObject parent) { return new InlineUIContainer(); } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidBaselineAlignment(object o) { BaselineAlignment value = (BaselineAlignment)o; return value == BaselineAlignment.Baseline || value == BaselineAlignment.Bottom || value == BaselineAlignment.Center || value == BaselineAlignment.Subscript || value == BaselineAlignment.Superscript || value == BaselineAlignment.TextBottom || value == BaselineAlignment.TextTop || value == BaselineAlignment.Top; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
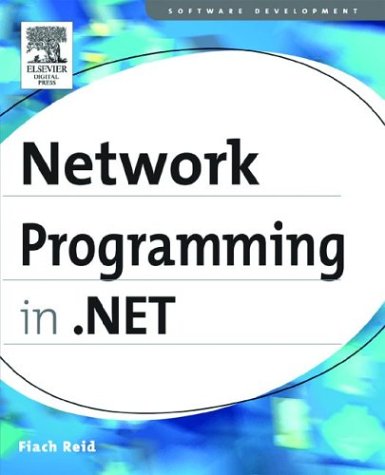
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _TimerThread.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- MouseDevice.cs
- Visual3D.cs
- ThrowHelper.cs
- ProcessInfo.cs
- TextFragmentEngine.cs
- DeploymentSectionCache.cs
- XmlSchemaAll.cs
- ProcessModuleCollection.cs
- WebScriptMetadataFormatter.cs
- HyperLink.cs
- ValidatedControlConverter.cs
- XmlException.cs
- ObjectListShowCommandsEventArgs.cs
- dataobject.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- CodeDomDecompiler.cs
- SafeRightsManagementQueryHandle.cs
- SqlMultiplexer.cs
- SerializationTrace.cs
- Rotation3DAnimationUsingKeyFrames.cs
- ChtmlPhoneCallAdapter.cs
- CalendarDateRangeChangingEventArgs.cs
- BaseParser.cs
- RegexWorker.cs
- FileBasedResourceGroveler.cs
- XmlValidatingReader.cs
- DataViewListener.cs
- SoundPlayer.cs
- TraceListener.cs
- UniqueIdentifierService.cs
- EnumBuilder.cs
- WebPartAuthorizationEventArgs.cs
- MessageBox.cs
- SocketPermission.cs
- BaseContextMenu.cs
- EditBehavior.cs
- _NetRes.cs
- XMLSyntaxException.cs
- XmlSerializationReader.cs
- ApplyImportsAction.cs
- ToolStripMenuItem.cs
- ValueType.cs
- WebContentFormatHelper.cs
- InlineUIContainer.cs
- ListView.cs
- BaseTransportHeaders.cs
- ProvideValueServiceProvider.cs
- Merger.cs
- ResourceExpressionBuilder.cs
- HttpListenerRequest.cs
- xsdvalidator.cs
- SQLDecimalStorage.cs
- Point3DValueSerializer.cs
- KoreanCalendar.cs
- ToggleProviderWrapper.cs
- ReadingWritingEntityEventArgs.cs
- ConnectionPointCookie.cs
- FilterException.cs
- BooleanFacetDescriptionElement.cs
- Function.cs
- CodeBlockBuilder.cs
- IteratorFilter.cs
- SolidBrush.cs
- RemotingSurrogateSelector.cs
- SolidBrush.cs
- Polygon.cs
- ConfigXmlCDataSection.cs
- HtmlTable.cs
- WebExceptionStatus.cs
- SqlNotificationEventArgs.cs
- WebSysDisplayNameAttribute.cs
- CodeTypeParameter.cs
- MenuItemStyleCollection.cs
- RadioButton.cs
- EventManager.cs
- CacheDependency.cs
- TemplateControlCodeDomTreeGenerator.cs
- ExpressionBuilder.cs
- NativeCompoundFileAPIs.cs
- ExpressionPrefixAttribute.cs
- InvalidProgramException.cs
- SingleObjectCollection.cs
- HashAlgorithm.cs
- MultipartContentParser.cs
- MessageBox.cs
- FlowLayoutPanel.cs
- NullableBoolConverter.cs
- ObjectPersistData.cs
- FontWeight.cs
- Simplifier.cs
- SerialStream.cs
- XmlHierarchicalEnumerable.cs
- Zone.cs
- Style.cs
- HtmlForm.cs
- NameValueFileSectionHandler.cs
- Point3DCollectionConverter.cs
- CTreeGenerator.cs