Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / ExpressionBindings.cs / 2 / ExpressionBindings.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Describes a binding for an expression. Conceptually similar to a foreach loop /// in C#. The DbExpression property defines the collection being iterated over, /// while the Var property provides a means to reference the current element /// of the collection during the iteration. DbExpressionBinding is used to describe the set arguments /// to relational expressions such as ///, /// and . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; internal DbExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable name is non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if(!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_CollectionRequired, "input"); } Debug.Assert(elementType.IsReadOnly, "DbExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; } /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } internal static void Check(string strName, DbExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } ///that references the element variable. /// /// Defines the binding for the input set to a [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; private string _groupVarName; internal DbGroupExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName, string groupVarName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); EntityUtil.CheckArgumentNull(groupVarName, "groupVarName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable and Group names are both non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } if (string.IsNullOrEmpty(groupVarName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_GroupVariableNameNotValid, "groupVarName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if (!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_CollectionRequired, "input"); } Debug.Assert((elementType.IsReadOnly), "DbGroupExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; _groupVarName = groupVarName; } ///. /// In addition to the properties of , DbGroupExpressionBinding /// also provides access to the group element via the variable reference. /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } ////// Gets the name assigned to the group element variable. /// public string GroupVariableName { get { return _groupVarName; } } ////// Gets the type metadata of the group element variable. /// public TypeUsage GroupVariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the group element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression GroupVariable { get { return this.Expression.CommandTree.CreateVariableReferenceExpression(_groupVarName, _varType); } } internal static void Check(string strName, DbGroupExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Describes a binding for an expression. Conceptually similar to a foreach loop /// in C#. The DbExpression property defines the collection being iterated over, /// while the Var property provides a means to reference the current element /// of the collection during the iteration. DbExpressionBinding is used to describe the set arguments /// to relational expressions such as ///, /// and . /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; internal DbExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable name is non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if(!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_CollectionRequired, "input"); } Debug.Assert(elementType.IsReadOnly, "DbExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; } /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } internal static void Check(string strName, DbExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } ///that references the element variable. /// /// Defines the binding for the input set to a [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupExpressionBinding { private ExpressionLink _expr; private string _varName; private TypeUsage _varType; private string _groupVarName; internal DbGroupExpressionBinding(DbCommandTree cmdTree, DbExpression input, string varName, string groupVarName) { // // Ensure no argument is null // EntityUtil.CheckArgumentNull(varName, "varName"); EntityUtil.CheckArgumentNull(groupVarName, "groupVarName"); _expr = new ExpressionLink("Expression", cmdTree, input); // // Ensure Variable and Group names are both non-empty // if (string.IsNullOrEmpty(varName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Binding_VariableNameNotValid, "varName"); } if (string.IsNullOrEmpty(groupVarName)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_GroupVariableNameNotValid, "groupVarName"); } // // Ensure the DbExpression has a collection result type // TypeUsage elementType = null; if (!TypeHelpers.TryGetCollectionElementType(input.ResultType, out elementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_GroupBinding_CollectionRequired, "input"); } Debug.Assert((elementType.IsReadOnly), "DbGroupExpressionBinding Expression ResultType has editable element type"); _varName = varName; _varType = elementType; _groupVarName = groupVarName; } ///. /// In addition to the properties of , DbGroupExpressionBinding /// also provides access to the group element via the variable reference. /// /// Gets or sets the ///that defines the input set. /// The expression is null ////// The expression is not associated with the binding's command tree, or its result type is not /// equal or promotable to the result type of the current value of the property. /// public DbExpression Expression { get { return _expr.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { _expr.Expression = value; } } ////// Gets the name assigned to the element variable. /// public string VariableName { get { return _varName; } } ////// Gets the type metadata of the element variable. /// public TypeUsage VariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression Variable { get { return _expr.Expression.CommandTree.CreateVariableReferenceExpression(_varName, _varType); } } ////// Gets the name assigned to the group element variable. /// public string GroupVariableName { get { return _groupVarName; } } ////// Gets the type metadata of the group element variable. /// public TypeUsage GroupVariableType { get { return _varType; } } ////// Creates a new DbVariableReferenceExpression that references the group element variable. /// /*CQT_PUBLIC_API(*/internal/*)*/ DbVariableReferenceExpression GroupVariable { get { return this.Expression.CommandTree.CreateVariableReferenceExpression(_groupVarName, _varType); } } internal static void Check(string strName, DbGroupExpressionBinding binding, DbCommandTree owner) { if (null == binding) { throw EntityUtil.ArgumentNull(strName); } if (owner != binding.Expression.CommandTree) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TreeMismatch, strName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
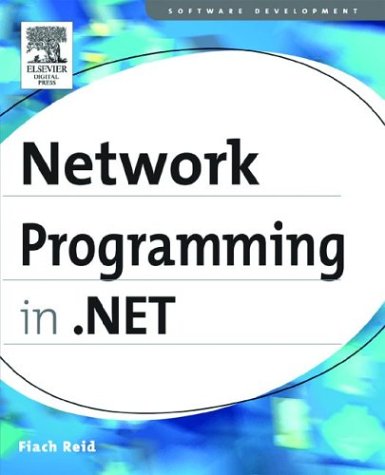
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Classification.cs
- OleDbDataReader.cs
- XmlSchemaImporter.cs
- ManagementQuery.cs
- ForeignConstraint.cs
- ParserHooks.cs
- ConfigurationValue.cs
- DocumentPaginator.cs
- SelectedDatesCollection.cs
- ToolStripDropDownClosedEventArgs.cs
- ListControlDataBindingHandler.cs
- RoleManagerSection.cs
- ViewBase.cs
- Propagator.Evaluator.cs
- PolicyLevel.cs
- IsolatedStorageException.cs
- TagNameToTypeMapper.cs
- TextEditorParagraphs.cs
- BmpBitmapDecoder.cs
- OutputCacheSettings.cs
- DataGridViewDataErrorEventArgs.cs
- SocketAddress.cs
- EntitySetBaseCollection.cs
- DisposableCollectionWrapper.cs
- Rect3DValueSerializer.cs
- PermissionAttributes.cs
- CodeDelegateCreateExpression.cs
- AsnEncodedData.cs
- EntityWrapperFactory.cs
- ValidationRuleCollection.cs
- CacheEntry.cs
- StateDesigner.TransitionInfo.cs
- SendMailErrorEventArgs.cs
- CachedBitmap.cs
- ListItemParagraph.cs
- ProviderSettingsCollection.cs
- X509Certificate2.cs
- HttpDigestClientCredential.cs
- OleDbWrapper.cs
- DesignTimeParseData.cs
- APCustomTypeDescriptor.cs
- AngleUtil.cs
- BorderGapMaskConverter.cs
- ExpressionNormalizer.cs
- HtmlSelect.cs
- StrongName.cs
- BufferBuilder.cs
- TextMarkerSource.cs
- TypeDescriptionProviderAttribute.cs
- ByteKeyFrameCollection.cs
- WebPartHelpVerb.cs
- ActiveXSite.cs
- PointCollection.cs
- OdbcConnection.cs
- StickyNote.cs
- TemplateColumn.cs
- BindingOperations.cs
- Token.cs
- KnownBoxes.cs
- DataBindingValueUIHandler.cs
- XmlSchemaSimpleType.cs
- FormsAuthenticationCredentials.cs
- StatusBar.cs
- Literal.cs
- ProtocolElement.cs
- DataGridColumnEventArgs.cs
- DataSourceCacheDurationConverter.cs
- SmiMetaData.cs
- GeometryGroup.cs
- AuthenticationModuleElementCollection.cs
- HashMembershipCondition.cs
- XsdBuilder.cs
- FontStyleConverter.cs
- PropertyChangedEventManager.cs
- Rotation3DKeyFrameCollection.cs
- DBSchemaRow.cs
- XmlSchemaAppInfo.cs
- SystemWebCachingSectionGroup.cs
- AtomServiceDocumentSerializer.cs
- Tracking.cs
- PagesSection.cs
- SimpleTypesSurrogate.cs
- ControlUtil.cs
- ReadWriteObjectLock.cs
- FixedSOMSemanticBox.cs
- __Filters.cs
- TimerEventSubscription.cs
- ChtmlCalendarAdapter.cs
- TrustLevelCollection.cs
- ServiceParser.cs
- ValueSerializerAttribute.cs
- TextServicesPropertyRanges.cs
- TextSelectionHighlightLayer.cs
- ErrorStyle.cs
- XmlEntityReference.cs
- SplitterPanel.cs
- HeaderedContentControl.cs
- Single.cs
- DispatcherHooks.cs
- safesecurityhelperavalon.cs