Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Result / SemanticValue.cs / 1 / SemanticValue.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Recognition { /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue"]/*' /> [Serializable] [DebuggerDisplay ("'{_keyName}'= {Value} - Children = {_dictionary.Count}")] [DebuggerTypeProxy (typeof (SemanticValueDebugDisplay))] public sealed class SemanticValue : IDictionary{ //******************************************************************* // // Constructors // //******************************************************************* #region Constructors #pragma warning disable 6504, 56507 /// /// TODOC /// /// /// /// public SemanticValue (string keyName, object value, float confidence) { Helpers.ThrowIfNull (keyName, "keyName"); _dictionary = new Dictionary(); _confidence = confidence; _keyName = keyName; _value = value; } #pragma warning restore 6504, 56507 /// /// TODOC /// /// public SemanticValue (object value) : this (string.Empty, value, -1f) { } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods ////// TODOC /// /// ///public override bool Equals (object obj) { SemanticValue refObj = obj as SemanticValue; if (refObj == null || refObj.Count != Count || refObj.Value == null && Value != null || (refObj.Value != null && !refObj.Value.Equals (Value))) { return false; } foreach (KeyValuePair kv in _dictionary) { if (!refObj.ContainsKey (kv.Key) || !refObj [kv.Key].Equals (this [kv.Key])) { return false; } } return true; } /// /// TODOC /// ///public override int GetHashCode () { return Count; } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region Public Properties // The value returned from the script / tags etc. // This can be cast to a more useful type {currently it will be string or int until we have .Net grammars}. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Value"]/*' /> public object Value { get { return _value; } internal set { _value = value; } } // Confidence score associated with the semantic item. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Confidence"]/*' /> public float Confidence { get { return _confidence; } } #endregion //******************************************************************* // // IDictionary implementation // //******************************************************************** #region IDictionary implementation // Expose the common query methods directly. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.this2"]/*' /> public SemanticValue this [string key] { get { return (SemanticValue) _dictionary [key]; } set { throw new InvalidOperationException (SR.Get (SRID.CollectionReadOnly)); } } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Contains"]/*' /> public bool Contains (KeyValuePair item) { return (_dictionary.ContainsKey (item.Key) && _dictionary.ContainsValue (item.Value)); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.ContainsKey"]/*' /> public bool ContainsKey (string key) { return _dictionary.ContainsKey (key); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Count"]/*' /> public int Count { get { return _dictionary.Count; } } // Other less common methods on IDictionary are also hidden from intellisense. // Read-only collection so throw on these methods. Also make then hidden through explicit interface declaration. void ICollection >.Add (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } void IDictionary .Add (string key, SemanticValue value) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } void ICollection >.Clear () { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } bool ICollection >.Remove (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } bool IDictionary .Remove (string key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.CopyTo"]/*' /> void ICollection >.CopyTo (KeyValuePair [] array, int index) { ((ICollection >) _dictionary).CopyTo (array, index); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.GetEnumerator"]/*' /> IEnumerator > IEnumerable >.GetEnumerator () { return _dictionary.GetEnumerator (); } bool ICollection >.IsReadOnly { get { return true; } } ICollection IDictionary .Keys { get { return (ICollection ) _dictionary.Keys; } } ICollection IDictionary .Values { get { return (ICollection ) _dictionary.Values; } } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.GetEnumerator"]/*' /> IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable >) this).GetEnumerator (); } bool IDictionary .TryGetValue (string key, out SemanticValue value) { return _dictionary.TryGetValue (key, out value); } #endregion //******************************************************************* // // Internal Properties // //******************************************************************* #region Internal Properties internal string KeyName { get { return _keyName; } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************** #region Internal Fields internal Dictionary _dictionary; internal bool _valueFieldSet; #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // Used by the debbugger display attribute private string _keyName; private float _confidence; private object _value; #endregion //******************************************************************** // // Private Types // //******************************************************************* #region Private Types // Used by the debbugger display attribute internal class SemanticValueDebugDisplay { public SemanticValueDebugDisplay (SemanticValue value) { _value = value.Value; _dictionary = value._dictionary; _name = value.KeyName; _confidence = value.Confidence; } public object Value { get { return _value; } } public object Count { get { return _dictionary.Count; } } public object KeyName { get { return _name; } } public object Confidence { get { return _confidence; } } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SemanticValue [] AKeys { get { SemanticValue [] keys = new SemanticValue [_dictionary.Count]; int i = 0; foreach (KeyValuePair kv in _dictionary) { keys [i++] = kv.Value; } return keys; } } private object _name; private object _value; private float _confidence; private IDictionary _dictionary; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Speech.Internal; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Recognition { /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue"]/*' /> [Serializable] [DebuggerDisplay ("'{_keyName}'= {Value} - Children = {_dictionary.Count}")] [DebuggerTypeProxy (typeof (SemanticValueDebugDisplay))] public sealed class SemanticValue : IDictionary{ //******************************************************************* // // Constructors // //******************************************************************* #region Constructors #pragma warning disable 6504, 56507 /// /// TODOC /// /// /// /// public SemanticValue (string keyName, object value, float confidence) { Helpers.ThrowIfNull (keyName, "keyName"); _dictionary = new Dictionary(); _confidence = confidence; _keyName = keyName; _value = value; } #pragma warning restore 6504, 56507 /// /// TODOC /// /// public SemanticValue (object value) : this (string.Empty, value, -1f) { } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods ////// TODOC /// /// ///public override bool Equals (object obj) { SemanticValue refObj = obj as SemanticValue; if (refObj == null || refObj.Count != Count || refObj.Value == null && Value != null || (refObj.Value != null && !refObj.Value.Equals (Value))) { return false; } foreach (KeyValuePair kv in _dictionary) { if (!refObj.ContainsKey (kv.Key) || !refObj [kv.Key].Equals (this [kv.Key])) { return false; } } return true; } /// /// TODOC /// ///public override int GetHashCode () { return Count; } #endregion //******************************************************************** // // Public Properties // //******************************************************************** #region Public Properties // The value returned from the script / tags etc. // This can be cast to a more useful type {currently it will be string or int until we have .Net grammars}. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Value"]/*' /> public object Value { get { return _value; } internal set { _value = value; } } // Confidence score associated with the semantic item. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Confidence"]/*' /> public float Confidence { get { return _confidence; } } #endregion //******************************************************************* // // IDictionary implementation // //******************************************************************** #region IDictionary implementation // Expose the common query methods directly. /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.this2"]/*' /> public SemanticValue this [string key] { get { return (SemanticValue) _dictionary [key]; } set { throw new InvalidOperationException (SR.Get (SRID.CollectionReadOnly)); } } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Contains"]/*' /> public bool Contains (KeyValuePair item) { return (_dictionary.ContainsKey (item.Key) && _dictionary.ContainsValue (item.Value)); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.ContainsKey"]/*' /> public bool ContainsKey (string key) { return _dictionary.ContainsKey (key); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.Count"]/*' /> public int Count { get { return _dictionary.Count; } } // Other less common methods on IDictionary are also hidden from intellisense. // Read-only collection so throw on these methods. Also make then hidden through explicit interface declaration. void ICollection >.Add (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } void IDictionary .Add (string key, SemanticValue value) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } void ICollection >.Clear () { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } bool ICollection >.Remove (KeyValuePair key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } bool IDictionary .Remove (string key) { throw new NotSupportedException (SR.Get (SRID.CollectionReadOnly)); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.CopyTo"]/*' /> void ICollection >.CopyTo (KeyValuePair [] array, int index) { ((ICollection >) _dictionary).CopyTo (array, index); } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.GetEnumerator"]/*' /> IEnumerator > IEnumerable >.GetEnumerator () { return _dictionary.GetEnumerator (); } bool ICollection >.IsReadOnly { get { return true; } } ICollection IDictionary .Keys { get { return (ICollection ) _dictionary.Keys; } } ICollection IDictionary .Values { get { return (ICollection ) _dictionary.Values; } } /// TODOC <_include file='doc\RecognitionResult.uex' path='docs/doc[@for="SemanticValue.GetEnumerator"]/*' /> IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable >) this).GetEnumerator (); } bool IDictionary .TryGetValue (string key, out SemanticValue value) { return _dictionary.TryGetValue (key, out value); } #endregion //******************************************************************* // // Internal Properties // //******************************************************************* #region Internal Properties internal string KeyName { get { return _keyName; } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************** #region Internal Fields internal Dictionary _dictionary; internal bool _valueFieldSet; #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // Used by the debbugger display attribute private string _keyName; private float _confidence; private object _value; #endregion //******************************************************************** // // Private Types // //******************************************************************* #region Private Types // Used by the debbugger display attribute internal class SemanticValueDebugDisplay { public SemanticValueDebugDisplay (SemanticValue value) { _value = value.Value; _dictionary = value._dictionary; _name = value.KeyName; _confidence = value.Confidence; } public object Value { get { return _value; } } public object Count { get { return _dictionary.Count; } } public object KeyName { get { return _name; } } public object Confidence { get { return _confidence; } } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SemanticValue [] AKeys { get { SemanticValue [] keys = new SemanticValue [_dictionary.Count]; int i = 0; foreach (KeyValuePair kv in _dictionary) { keys [i++] = kv.Value; } return keys; } } private object _name; private object _value; private float _confidence; private IDictionary _dictionary; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
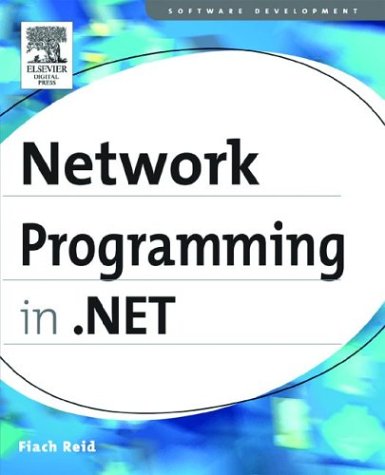
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializableAttribute.cs
- ProfileEventArgs.cs
- BufferedGraphicsContext.cs
- UserPersonalizationStateInfo.cs
- DataColumnMapping.cs
- CharUnicodeInfo.cs
- DbConnectionOptions.cs
- NamedObject.cs
- DefaultMergeHelper.cs
- PolicyManager.cs
- SafeSecurityHandles.cs
- TabPage.cs
- DataServiceStreamResponse.cs
- TileBrush.cs
- TextContainer.cs
- ToolStripSettings.cs
- MapPathBasedVirtualPathProvider.cs
- PersistChildrenAttribute.cs
- ToolboxItemCollection.cs
- XPathScanner.cs
- IDQuery.cs
- SQLDecimal.cs
- LoggedException.cs
- ObjectListItemCollection.cs
- RectIndependentAnimationStorage.cs
- MembershipValidatePasswordEventArgs.cs
- ListViewHitTestInfo.cs
- LocationEnvironment.cs
- HighContrastHelper.cs
- Literal.cs
- SerializationUtilities.cs
- FlowDocumentPageViewerAutomationPeer.cs
- KeyedCollection.cs
- DynamicRendererThreadManager.cs
- RolePrincipal.cs
- RectangleHotSpot.cs
- QuinticEase.cs
- ContextMenu.cs
- GB18030Encoding.cs
- ModuleBuilder.cs
- DescriptionAttribute.cs
- SystemUnicastIPAddressInformation.cs
- WebBrowserNavigatedEventHandler.cs
- TraceLog.cs
- ThreadStartException.cs
- DrawingState.cs
- TypeElement.cs
- CompareValidator.cs
- SystemUnicastIPAddressInformation.cs
- DataGridViewDataErrorEventArgs.cs
- CorePropertiesFilter.cs
- HitTestResult.cs
- Command.cs
- MouseDevice.cs
- XsltContext.cs
- XmlCharCheckingReader.cs
- DefaultValueAttribute.cs
- Descriptor.cs
- SecUtil.cs
- LineServicesRun.cs
- DbExpressionVisitor.cs
- FieldMetadata.cs
- Image.cs
- AuthenticatedStream.cs
- ComplexLine.cs
- CompilerGlobalScopeAttribute.cs
- webproxy.cs
- WhitespaceReader.cs
- TypefaceMetricsCache.cs
- ICspAsymmetricAlgorithm.cs
- Rectangle.cs
- ChangePassword.cs
- ArgumentException.cs
- ClientSideProviderDescription.cs
- DataRelationCollection.cs
- SafeNativeMethodsCLR.cs
- Compiler.cs
- Faults.cs
- TypefaceCollection.cs
- ConfigXmlReader.cs
- GatewayDefinition.cs
- SHA384.cs
- SortFieldComparer.cs
- CollectionEditVerbManager.cs
- CaretElement.cs
- UnitySerializationHolder.cs
- GlyphsSerializer.cs
- PolicyException.cs
- ThreadInterruptedException.cs
- DataProtectionSecurityStateEncoder.cs
- LinqDataSourceDisposeEventArgs.cs
- DataPagerFieldItem.cs
- FigureParaClient.cs
- ButtonChrome.cs
- EllipseGeometry.cs
- CodeTypeMemberCollection.cs
- Documentation.cs
- TextTreeNode.cs
- JsonStringDataContract.cs
- BaseCollection.cs