Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Text / TextLineResult.cs / 1 / TextLineResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Access to calculated information of a line of text created // by TextBlock. // // History: // 04/25/2003 : grzegorz - Moving from Avalon branch. // 06/25/2004 : grzegorz - Performance work. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Documents; namespace MS.Internal.Text { ////// Provides access to calculated information of a line of text created /// by TextBlock. /// internal sealed class TextLineResult : LineResult { //------------------------------------------------------------------- // // LineResult Methods // //------------------------------------------------------------------- #region LineResult Methods ////// Retrieves a position matching distance within the line. /// /// Distance within the line. ////// A text position and its orientation matching or closest to the distance. /// internal override ITextPointer GetTextPositionFromDistance(double distance) { return _owner.GetTextPositionFromDistance(_dcp, distance, _layoutBox.Top, _index); } ////// /// /// ///internal override bool IsAtCaretUnitBoundary(ITextPointer position) { Debug.Assert(false); return false; } /// /// /// /// /// ///internal override ITextPointer GetNextCaretUnitPosition(ITextPointer position, LogicalDirection direction) { Debug.Assert(false); return null; } /// /// /// /// ///internal override ITextPointer GetBackspaceCaretUnitPosition(ITextPointer position) { Debug.Assert(false); return null; } /// /// /// /// /// ///internal override ReadOnlyCollection GetGlyphRuns(ITextPointer start, ITextPointer end) { Debug.Assert(false); return null; } /// /// Retrieves the position after last content character of the line, /// not including any line breaks. /// ////// The position after last content character of the line, /// not including any line breaks. /// internal override ITextPointer GetContentEndPosition() { EnsureComplexData(); return _owner.TextContainer.CreatePointerAtOffset(_dcp + _cchContent, LogicalDirection.Backward); } ////// Retrieves the position in the line pointing to the beginning of content /// hidden by ellipses. /// ////// The position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override ITextPointer GetEllipsesPosition() { EnsureComplexData(); if (_cchEllipses != 0) { return _owner.TextContainer.CreatePointerAtOffset(_dcp + _cch - _cchEllipses, LogicalDirection.Forward); } return null; } ////// Retrieves the position after last content character of the line, /// not including any line breaks. /// ////// The position after last content character of the line, /// not including any line breaks. /// internal override int GetContentEndPositionCP() { EnsureComplexData(); return _dcp + _cchContent; } ////// Retrieves the position in the line pointing to the beginning of content /// hidden by ellipses. /// ////// The position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override int GetEllipsesPositionCP() { EnsureComplexData(); return _dcp + _cch - _cchEllipses; } #endregion LineResult Methods //-------------------------------------------------------------------- // // LineResult Properties // //------------------------------------------------------------------- #region LineResult Properties ////// ITextPointer representing the beginning of the Line's contents. /// internal override ITextPointer StartPosition { get { if (_startPosition == null) { _startPosition = _owner.TextContainer.CreatePointerAtOffset(_dcp, LogicalDirection.Forward); } return _startPosition; } } ////// ITextPointer representing the end of the Line's contents. /// internal override ITextPointer EndPosition { get { if (_endPosition == null) { _endPosition = _owner.TextContainer.CreatePointerAtOffset(_dcp + _cch, LogicalDirection.Backward); } return _endPosition; } } ////// Character position representing the beginning of the Line's contents. /// internal override int StartPositionCP { get { return _dcp; } } ////// Character position representing the end of the Line's contents. /// internal override int EndPositionCP { get { return _dcp + _cch; } } ////// The bounding rectangle of the line. /// internal override Rect LayoutBox { get { return _layoutBox; } } ////// The dominant baseline of the line. /// Distance from the top of the line to the baseline. /// internal override double Baseline { get { return _baseline; } } #endregion LineResult Properties //-------------------------------------------------------------------- // // Constructors // //-------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Owner of the line. /// Index of the first character in the line. /// Number of all characters in the line. /// Rectangle of the line within a text paragraph. /// Distance from the top of the line to the baseline. /// Index of the line within the text block internal TextLineResult(System.Windows.Controls.TextBlock owner, int dcp, int cch, Rect layoutBox, double baseline, int index) { _owner = owner; _dcp = dcp; _cch = cch; _layoutBox = layoutBox; _baseline = baseline; _index = index; _cchContent = _cchEllipses = -1; } #endregion Constructors //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private void EnsureComplexData() { if (_cchContent == -1) { _owner.GetLineDetails(_dcp, _index, _layoutBox.Top, out _cchContent, out _cchEllipses); } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// Owner of the line. /// private readonly System.Windows.Controls.TextBlock _owner; ////// Index of the first character in the line. /// private readonly int _dcp; ////// Number of all characters in the line. /// private readonly int _cch; ////// Rectangle of the line within a text paragraph. /// private readonly Rect _layoutBox; ////// Index of the line within the TextBlock /// private int _index; ////// The dominant baseline of the line. Distance from the top of the /// line to the baseline. /// private readonly double _baseline; ////// ITextPointer representing the beginning of the Line's contents. /// private ITextPointer _startPosition; ////// ITextPointer representing the end of the Line's contents. /// private ITextPointer _endPosition; ////// Number of characters of content of the line, not including any line breaks. /// private int _cchContent; ////// Number of characters hidden by ellipses. /// private int _cchEllipses; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Access to calculated information of a line of text created // by TextBlock. // // History: // 04/25/2003 : grzegorz - Moving from Avalon branch. // 06/25/2004 : grzegorz - Performance work. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Documents; namespace MS.Internal.Text { ////// Provides access to calculated information of a line of text created /// by TextBlock. /// internal sealed class TextLineResult : LineResult { //------------------------------------------------------------------- // // LineResult Methods // //------------------------------------------------------------------- #region LineResult Methods ////// Retrieves a position matching distance within the line. /// /// Distance within the line. ////// A text position and its orientation matching or closest to the distance. /// internal override ITextPointer GetTextPositionFromDistance(double distance) { return _owner.GetTextPositionFromDistance(_dcp, distance, _layoutBox.Top, _index); } ////// /// /// ///internal override bool IsAtCaretUnitBoundary(ITextPointer position) { Debug.Assert(false); return false; } /// /// /// /// /// ///internal override ITextPointer GetNextCaretUnitPosition(ITextPointer position, LogicalDirection direction) { Debug.Assert(false); return null; } /// /// /// /// ///internal override ITextPointer GetBackspaceCaretUnitPosition(ITextPointer position) { Debug.Assert(false); return null; } /// /// /// /// /// ///internal override ReadOnlyCollection GetGlyphRuns(ITextPointer start, ITextPointer end) { Debug.Assert(false); return null; } /// /// Retrieves the position after last content character of the line, /// not including any line breaks. /// ////// The position after last content character of the line, /// not including any line breaks. /// internal override ITextPointer GetContentEndPosition() { EnsureComplexData(); return _owner.TextContainer.CreatePointerAtOffset(_dcp + _cchContent, LogicalDirection.Backward); } ////// Retrieves the position in the line pointing to the beginning of content /// hidden by ellipses. /// ////// The position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override ITextPointer GetEllipsesPosition() { EnsureComplexData(); if (_cchEllipses != 0) { return _owner.TextContainer.CreatePointerAtOffset(_dcp + _cch - _cchEllipses, LogicalDirection.Forward); } return null; } ////// Retrieves the position after last content character of the line, /// not including any line breaks. /// ////// The position after last content character of the line, /// not including any line breaks. /// internal override int GetContentEndPositionCP() { EnsureComplexData(); return _dcp + _cchContent; } ////// Retrieves the position in the line pointing to the beginning of content /// hidden by ellipses. /// ////// The position in the line pointing to the beginning of content /// hidden by ellipses. /// internal override int GetEllipsesPositionCP() { EnsureComplexData(); return _dcp + _cch - _cchEllipses; } #endregion LineResult Methods //-------------------------------------------------------------------- // // LineResult Properties // //------------------------------------------------------------------- #region LineResult Properties ////// ITextPointer representing the beginning of the Line's contents. /// internal override ITextPointer StartPosition { get { if (_startPosition == null) { _startPosition = _owner.TextContainer.CreatePointerAtOffset(_dcp, LogicalDirection.Forward); } return _startPosition; } } ////// ITextPointer representing the end of the Line's contents. /// internal override ITextPointer EndPosition { get { if (_endPosition == null) { _endPosition = _owner.TextContainer.CreatePointerAtOffset(_dcp + _cch, LogicalDirection.Backward); } return _endPosition; } } ////// Character position representing the beginning of the Line's contents. /// internal override int StartPositionCP { get { return _dcp; } } ////// Character position representing the end of the Line's contents. /// internal override int EndPositionCP { get { return _dcp + _cch; } } ////// The bounding rectangle of the line. /// internal override Rect LayoutBox { get { return _layoutBox; } } ////// The dominant baseline of the line. /// Distance from the top of the line to the baseline. /// internal override double Baseline { get { return _baseline; } } #endregion LineResult Properties //-------------------------------------------------------------------- // // Constructors // //-------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Owner of the line. /// Index of the first character in the line. /// Number of all characters in the line. /// Rectangle of the line within a text paragraph. /// Distance from the top of the line to the baseline. /// Index of the line within the text block internal TextLineResult(System.Windows.Controls.TextBlock owner, int dcp, int cch, Rect layoutBox, double baseline, int index) { _owner = owner; _dcp = dcp; _cch = cch; _layoutBox = layoutBox; _baseline = baseline; _index = index; _cchContent = _cchEllipses = -1; } #endregion Constructors //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private void EnsureComplexData() { if (_cchContent == -1) { _owner.GetLineDetails(_dcp, _index, _layoutBox.Top, out _cchContent, out _cchEllipses); } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// Owner of the line. /// private readonly System.Windows.Controls.TextBlock _owner; ////// Index of the first character in the line. /// private readonly int _dcp; ////// Number of all characters in the line. /// private readonly int _cch; ////// Rectangle of the line within a text paragraph. /// private readonly Rect _layoutBox; ////// Index of the line within the TextBlock /// private int _index; ////// The dominant baseline of the line. Distance from the top of the /// line to the baseline. /// private readonly double _baseline; ////// ITextPointer representing the beginning of the Line's contents. /// private ITextPointer _startPosition; ////// ITextPointer representing the end of the Line's contents. /// private ITextPointer _endPosition; ////// Number of characters of content of the line, not including any line breaks. /// private int _cchContent; ////// Number of characters hidden by ellipses. /// private int _cchEllipses; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
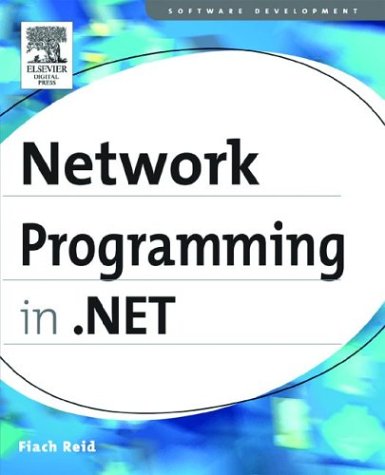
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlPoint3DCollectionSerializer.cs
- JoinSymbol.cs
- Utils.cs
- Knowncolors.cs
- IntPtr.cs
- FixedSOMPageConstructor.cs
- StylusCaptureWithinProperty.cs
- DependencyObjectPropertyDescriptor.cs
- FontStyle.cs
- ContentType.cs
- FlowDocumentPaginator.cs
- DataColumn.cs
- SqlUtils.cs
- MetadataPropertyvalue.cs
- RowToFieldTransformer.cs
- PropertyValueChangedEvent.cs
- InstanceDescriptor.cs
- _ConnectionGroup.cs
- GenericParameterDataContract.cs
- DynamicPropertyHolder.cs
- ResolveResponseInfo.cs
- ScriptingWebServicesSectionGroup.cs
- EditingScope.cs
- VideoDrawing.cs
- EtwTrackingParticipant.cs
- PersonalizableTypeEntry.cs
- LowerCaseStringConverter.cs
- ClickablePoint.cs
- TraceUtility.cs
- Monitor.cs
- ThreadInterruptedException.cs
- FormatException.cs
- HtmlFormParameterReader.cs
- DataGridSortingEventArgs.cs
- WindowsSlider.cs
- InputBindingCollection.cs
- MaterialCollection.cs
- MediaTimeline.cs
- EmptyStringExpandableObjectConverter.cs
- BinaryMethodMessage.cs
- SelectionProcessor.cs
- ScaleTransform.cs
- FontCollection.cs
- GradientStopCollection.cs
- PageAsyncTaskManager.cs
- TextDecorationCollection.cs
- Descriptor.cs
- SafeRightsManagementPubHandle.cs
- GPRECTF.cs
- HtmlHead.cs
- AutoFocusStyle.xaml.cs
- _NegotiateClient.cs
- XamlBrushSerializer.cs
- ParameterEditorUserControl.cs
- EmptyQuery.cs
- PointAnimationUsingKeyFrames.cs
- FileDialogPermission.cs
- ExtendedProperty.cs
- SafeTimerHandle.cs
- AssemblySettingAttributes.cs
- Package.cs
- TableItemStyle.cs
- RoutedEventHandlerInfo.cs
- OleDbStruct.cs
- BitmapVisualManager.cs
- Point3DIndependentAnimationStorage.cs
- ServicePoint.cs
- SID.cs
- ConnectivityStatus.cs
- WebBrowserPermission.cs
- Button.cs
- XmlnsPrefixAttribute.cs
- TextOnlyOutput.cs
- Point.cs
- NativeMethods.cs
- PerformanceCounterPermissionEntry.cs
- SpellerHighlightLayer.cs
- WindowInteractionStateTracker.cs
- OutArgument.cs
- MarginCollapsingState.cs
- CharacterString.cs
- IxmlLineInfo.cs
- CrossContextChannel.cs
- StringAttributeCollection.cs
- TableLayout.cs
- SyndicationSerializer.cs
- FileRecordSequenceCompletedAsyncResult.cs
- HtmlInputSubmit.cs
- IsolatedStorageFilePermission.cs
- ActivityContext.cs
- FontFamilyIdentifier.cs
- MessageBuffer.cs
- EventProxy.cs
- SqlFormatter.cs
- NCryptSafeHandles.cs
- OdbcException.cs
- CheckBoxBaseAdapter.cs
- InvalidTimeZoneException.cs
- GradientBrush.cs
- DataGridViewDataErrorEventArgs.cs