Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / ActivityContext.cs / 1305376 / ActivityContext.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Runtime; using System.Activities.Tracking; using System.Diagnostics.CodeAnalysis; using System.Runtime; [Fx.Tag.XamlVisible(false)] public class ActivityContext { ActivityInstance instance; ActivityExecutor executor; bool isDisposed; // Used by subclasses that are pooled. internal ActivityContext() { } // these can only be created by the WF Runtime internal ActivityContext(ActivityInstance instance, ActivityExecutor executor) { Fx.Assert(instance != null, "valid activity instance is required"); this.instance = instance; this.executor = executor; this.Activity = this.instance.Activity; } internal LocationEnvironment Environment { get { ThrowIfDisposed(); return this.instance.Environment; } } internal bool AllowChainedEnvironmentAccess { get; set; } internal Activity Activity { get; set; } internal ActivityInstance CurrentInstance { get { return this.instance; } } public string ActivityInstanceId { get { ThrowIfDisposed(); return this.instance.Id; } } public Guid WorkflowInstanceId { get { ThrowIfDisposed(); return this.executor.WorkflowInstanceId; } } public WorkflowDataContext DataContext { get { ThrowIfDisposed(); if (this.instance.DataContext == null) { this.instance.DataContext = new WorkflowDataContext(this.executor, this.instance); } return this.instance.DataContext; } } internal bool IsDisposed { get { return this.isDisposed; } } public T GetExtension() where T : class { ThrowIfDisposed(); return this.executor.GetExtension (); } internal void Reinitialize(ActivityInstance instance, ActivityExecutor executor) { this.isDisposed = false; this.instance = instance; this.executor = executor; this.Activity = this.instance.Activity; } // extra insurance against misuse (if someone stashes away the execution context to use later) internal void Dispose() { this.isDisposed = true; this.instance = null; this.executor = null; this.Activity = null; } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. public Location GetLocation (LocationReference locationReference) { ThrowIfDisposed(); if (locationReference == null) { throw FxTrace.Exception.ArgumentNull("locationReference"); } Location location = locationReference.GetLocation(this); Location typedLocation = location as Location ; if (typedLocation != null) { return typedLocation; } else { Fx.Assert(location != null, "The contract of LocationReference is that GetLocation never returns null."); if (locationReference.Type == typeof(T)) { return new TypedLocationWrapper (location); } else { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.LocationTypeMismatch(locationReference.Name, typeof(T), locationReference.Type))); } } } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. public T GetValue (LocationReference locationReference) { ThrowIfDisposed(); if (locationReference == null) { throw FxTrace.Exception.ArgumentNull("locationReference"); } return GetValueCore (locationReference); } internal T GetValueCore (LocationReference locationReference) { Location location = locationReference.GetLocation(this); Location typedLocation = location as Location ; if (typedLocation != null) { // If we hit this path we can avoid boxing value types return typedLocation.Value; } else { Fx.Assert(location != null, "The contract of LocationReference is that GetLocation never returns null."); return TypeHelper.Convert (location.Value); } } public void SetValue (LocationReference locationReference, T value) { ThrowIfDisposed(); if (locationReference == null) { throw FxTrace.Exception.ArgumentNull("locationReference"); } SetValueCore (locationReference, value); } internal void SetValueCore (LocationReference locationReference, T value) { Location location = locationReference.GetLocation(this); Location typedLocation = location as Location ; if (typedLocation != null) { // If we hit this path we can avoid boxing value types typedLocation.Value = value; } else { if (!TypeHelper.AreTypesCompatible(value, locationReference.Type)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.CannotSetValueToLocation(value != null ? value.GetType() : typeof(T), locationReference.Name, locationReference.Type))); } location.Value = value; } } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. [SuppressMessage(FxCop.Category.Design, FxCop.Rule.ConsiderPassingBaseTypesAsParameters, Justification = "Generic needed for type inference")] public T GetValue (OutArgument argument) { ThrowIfDisposed(); if (argument == null) { throw FxTrace.Exception.ArgumentNull("argument"); } argument.ThrowIfNotInTree(); return GetValueCore (argument.RuntimeArgument); } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. [SuppressMessage(FxCop.Category.Design, FxCop.Rule.ConsiderPassingBaseTypesAsParameters, Justification = "Generic needed for type inference")] public T GetValue (InOutArgument argument) { ThrowIfDisposed(); if (argument == null) { throw FxTrace.Exception.ArgumentNull("argument"); } argument.ThrowIfNotInTree(); return GetValueCore (argument.RuntimeArgument); } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. [SuppressMessage(FxCop.Category.Design, FxCop.Rule.ConsiderPassingBaseTypesAsParameters, Justification = "Generic needed for type inference")] public T GetValue (InArgument argument) { ThrowIfDisposed(); if (argument == null) { throw FxTrace.Exception.ArgumentNull("argument"); } argument.ThrowIfNotInTree(); return GetValueCore (argument.RuntimeArgument); } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. public object GetValue(Argument argument) { ThrowIfDisposed(); if (argument == null) { throw FxTrace.Exception.ArgumentNull("argument"); } argument.ThrowIfNotInTree(); return GetValueCore
Link Menu
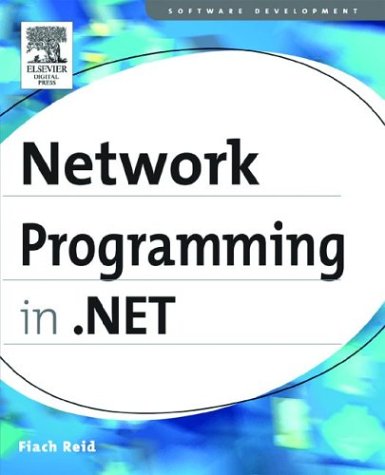
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDigitalSignatureProcessor.cs
- UInt32Storage.cs
- DisplayNameAttribute.cs
- ComponentEditorForm.cs
- XmlDictionary.cs
- RolePrincipal.cs
- ConditionalAttribute.cs
- EventMemberCodeDomSerializer.cs
- TextRangeSerialization.cs
- SignatureResourcePool.cs
- ImageInfo.cs
- ListViewItemCollectionEditor.cs
- PbrsForward.cs
- PerformanceCounterPermissionEntry.cs
- BitArray.cs
- TransactionState.cs
- SafePEFileHandle.cs
- ServiceParser.cs
- QuaternionKeyFrameCollection.cs
- FixedStringLookup.cs
- PropertyIDSet.cs
- PrintDialog.cs
- _SpnDictionary.cs
- XmlChildEnumerator.cs
- HttpApplicationFactory.cs
- SqlNodeTypeOperators.cs
- TextRunCacheImp.cs
- XPathQilFactory.cs
- XmlNodeChangedEventManager.cs
- AlignmentXValidation.cs
- CompositeClientFormatter.cs
- SqlReferenceCollection.cs
- ScalarOps.cs
- XsltSettings.cs
- ConfigErrorGlyph.cs
- Binding.cs
- XmlSignatureManifest.cs
- BindingMAnagerBase.cs
- Int64AnimationUsingKeyFrames.cs
- IProducerConsumerCollection.cs
- WebServiceTypeData.cs
- streamingZipPartStream.cs
- ToolTipAutomationPeer.cs
- PropertyInfo.cs
- CodeActivity.cs
- CompensateDesigner.cs
- TemplateContent.cs
- ModifiableIteratorCollection.cs
- RelationshipConverter.cs
- DependentList.cs
- HtmlGenericControl.cs
- ProfessionalColorTable.cs
- ReachPageContentSerializerAsync.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- WorkflowRuntimeServiceElementCollection.cs
- XmlComment.cs
- HttpRuntimeSection.cs
- PropertyGridView.cs
- SQLMembershipProvider.cs
- SendMailErrorEventArgs.cs
- UnsafeNativeMethods.cs
- AppSettingsSection.cs
- Math.cs
- RadioButtonBaseAdapter.cs
- CanonicalizationDriver.cs
- FunctionQuery.cs
- CompiledELinqQueryState.cs
- EventProviderClassic.cs
- DateTimeConstantAttribute.cs
- MultiDataTrigger.cs
- bidPrivateBase.cs
- EntityTransaction.cs
- VoiceChangeEventArgs.cs
- RSACryptoServiceProvider.cs
- StopStoryboard.cs
- CodeDelegateCreateExpression.cs
- SerialPort.cs
- TagNameToTypeMapper.cs
- ContextStack.cs
- ColorPalette.cs
- SignatureToken.cs
- XmlAnyElementAttribute.cs
- SqlDataSourceEnumerator.cs
- ReturnEventArgs.cs
- ListSortDescription.cs
- TextDecorationLocationValidation.cs
- SystemNetworkInterface.cs
- InstalledFontCollection.cs
- messageonlyhwndwrapper.cs
- BamlRecordWriter.cs
- EntityContainerAssociationSetEnd.cs
- ToolStripOverflow.cs
- EventMappingSettings.cs
- Types.cs
- SafeNativeMethods.cs
- SerializationInfo.cs
- LogLogRecordEnumerator.cs
- TimeSpan.cs
- ImpersonateTokenRef.cs
- ShaperBuffers.cs