Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / ChannelManager.cs / 1 / ChannelManager.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The ChannelManager is a helper structure internal to MediaContext // that abstracts out channel creation, storage and destruction. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal; using MS.Utility; using MS.Win32; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; namespace System.Windows.Media { partial class MediaContext { ////// A helper structure that abstracts channel management. /// ////// The common agreement (avcomptm, avpttrev) is that channel creation /// should be considered unsafe and therefore subject to control via /// the SecurityCritical/SecurityTreatAsSafe mechanism. /// /// On the other hand, using a channel that has been created by the /// MediaContext is considered safe. /// /// This class expresses the above policy by hiding channel creation /// and storage within the MediaContext while allowing to access /// the channels at will. /// private struct ChannelManager { ////// Opens an asynchronous channel. /// ////// Critical - Creates a channel, calls a Channel method, calls methods performing elevations. /// TreatAsSafe - It is safe for the media context to create and use a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateChannels(bool hasLayeredWindows) { Invariant.Assert(_asyncChannel == null); Invariant.Assert(_asyncOutOfBandChannel == null); // Create a channel into the async composition device. // Pass in a reference to the global mediasystem channel so that it uses // the same partition. _asyncChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, false, // not out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // [....] transport ); _asyncOutOfBandChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, true, // out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // [....] transport ); } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to [....] channels channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveSyncChannels() { // // Close the synchronous channels. // if (_freeSyncChannels != null) { while (_freeSyncChannels.Count > 0) { _freeSyncChannels.Dequeue().Close(); } _freeSyncChannels = null; } if (_syncServiceChannel != null) { _syncServiceChannel.Close(); _syncServiceChannel = null; } } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to close a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveChannels() { if (_asyncChannel != null) { _asyncChannel.Close(); _asyncChannel = null; } if (_asyncOutOfBandChannel != null) { _asyncOutOfBandChannel.Close(); _asyncOutOfBandChannel = null; } RemoveSyncChannels(); if (_pSyncConnection != IntPtr.Zero) { HRESULT.Check(UnsafeNativeMethods.MilTransport_DisconnectTransport( _pSyncConnection)); _pSyncConnection = IntPtr.Zero; } } ////// Create a fresh or fetch one from the pool synchronous channel. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Using a channel created by the media context is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel AllocateSyncChannel() { DUCE.Channel syncChannel; if (_pSyncConnection == IntPtr.Zero) { _pSyncConnection = System.Windows.Media.MediaSystem.ConnectSyncTransport( out _pSyncPacketTransport ); } if (_freeSyncChannels == null) { // // Ensure the free [....] channels queue... // _freeSyncChannels = new Queue(3); } if (_freeSyncChannels.Count > 0) { // // If there is a free [....] channel in the queue, we're done: // return _freeSyncChannels.Dequeue(); } else { // // Otherwise, create a new channel. We will try to cache it // when the ReleaseSyncChannel call is made. Also ensure the // synchronous service channel and glyph cache. // if (_syncServiceChannel == null) { _syncServiceChannel = new DUCE.Channel( null, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); } syncChannel = new DUCE.Channel( _syncServiceChannel, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); return syncChannel; } } /// /// Returns a [....] channel back to the pool. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Closing a channel is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void ReleaseSyncChannel(DUCE.Channel channel) { Invariant.Assert(_freeSyncChannels != null); // // A decision needs to be made whether or not we're interested // in re-using this channel later. For now, store up to three // synchronous channel in the queue. // if (_freeSyncChannels.Count <= 3) { // // Since we don't close the channel, verify that // the RemoveAndReleaseQueue is empty for resources. // Debug.Assert(channel.IsRemoveAndReleaseQueueEmpty()); _freeSyncChannels.Enqueue(channel); } else { channel.Close(); } } ////// Flushes the remove and release queues of all channels /// ////// Critical - Performs commands on channels. /// TreatAsSafe - Flushing commands that have already been enqueued is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void FlushAllRemoveReleaseQueues() { FlushRemoveAndReleaseQueues(_asyncChannel); FlushRemoveAndReleaseQueues(_asyncOutOfBandChannel); } ////// Private helper function to flush the remove and release queues of a channel /// ////// Critical - Performs commands on channels. /// [SecurityCritical] private void FlushRemoveAndReleaseQueues(DUCE.Channel channel) { if (channel != null) { channel.ProcessRemoveCommand(); channel.ProcessReleaseCommand(); channel.ClearRemoveAndReleaseQueue(); } } ////// Returns the asynchronous channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel Channel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncChannel; } } ////// Returns the asynchronous out-of-band channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel OutOfBandChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncOutOfBandChannel; } } ////// The asynchronous channel. /// ////// This field will be replaced with an asynchronous channel table as per task #26681. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncChannel; ////// The asynchronous out-of-band channel. /// ////// This is needed by CompositionTarget for handling WM_PAINT, etc. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncOutOfBandChannel; ////// We store the free synchronous channels for later re-use in this queue. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private Queue_freeSyncChannels; /// /// The service channel to the synchronous partition. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _syncServiceChannel; ////// Pointer to the unmanaged transport object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncPacketTransport; ////// Pointer to the unmanaged connection object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncConnection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The ChannelManager is a helper structure internal to MediaContext // that abstracts out channel creation, storage and destruction. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal; using MS.Utility; using MS.Win32; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; namespace System.Windows.Media { partial class MediaContext { ////// A helper structure that abstracts channel management. /// ////// The common agreement (avcomptm, avpttrev) is that channel creation /// should be considered unsafe and therefore subject to control via /// the SecurityCritical/SecurityTreatAsSafe mechanism. /// /// On the other hand, using a channel that has been created by the /// MediaContext is considered safe. /// /// This class expresses the above policy by hiding channel creation /// and storage within the MediaContext while allowing to access /// the channels at will. /// private struct ChannelManager { ////// Opens an asynchronous channel. /// ////// Critical - Creates a channel, calls a Channel method, calls methods performing elevations. /// TreatAsSafe - It is safe for the media context to create and use a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateChannels(bool hasLayeredWindows) { Invariant.Assert(_asyncChannel == null); Invariant.Assert(_asyncOutOfBandChannel == null); // Create a channel into the async composition device. // Pass in a reference to the global mediasystem channel so that it uses // the same partition. _asyncChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, false, // not out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // [....] transport ); _asyncOutOfBandChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, true, // out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // [....] transport ); } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to [....] channels channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveSyncChannels() { // // Close the synchronous channels. // if (_freeSyncChannels != null) { while (_freeSyncChannels.Count > 0) { _freeSyncChannels.Dequeue().Close(); } _freeSyncChannels = null; } if (_syncServiceChannel != null) { _syncServiceChannel.Close(); _syncServiceChannel = null; } } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to close a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveChannels() { if (_asyncChannel != null) { _asyncChannel.Close(); _asyncChannel = null; } if (_asyncOutOfBandChannel != null) { _asyncOutOfBandChannel.Close(); _asyncOutOfBandChannel = null; } RemoveSyncChannels(); if (_pSyncConnection != IntPtr.Zero) { HRESULT.Check(UnsafeNativeMethods.MilTransport_DisconnectTransport( _pSyncConnection)); _pSyncConnection = IntPtr.Zero; } } ////// Create a fresh or fetch one from the pool synchronous channel. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Using a channel created by the media context is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel AllocateSyncChannel() { DUCE.Channel syncChannel; if (_pSyncConnection == IntPtr.Zero) { _pSyncConnection = System.Windows.Media.MediaSystem.ConnectSyncTransport( out _pSyncPacketTransport ); } if (_freeSyncChannels == null) { // // Ensure the free [....] channels queue... // _freeSyncChannels = new Queue(3); } if (_freeSyncChannels.Count > 0) { // // If there is a free [....] channel in the queue, we're done: // return _freeSyncChannels.Dequeue(); } else { // // Otherwise, create a new channel. We will try to cache it // when the ReleaseSyncChannel call is made. Also ensure the // synchronous service channel and glyph cache. // if (_syncServiceChannel == null) { _syncServiceChannel = new DUCE.Channel( null, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); } syncChannel = new DUCE.Channel( _syncServiceChannel, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); return syncChannel; } } /// /// Returns a [....] channel back to the pool. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Closing a channel is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void ReleaseSyncChannel(DUCE.Channel channel) { Invariant.Assert(_freeSyncChannels != null); // // A decision needs to be made whether or not we're interested // in re-using this channel later. For now, store up to three // synchronous channel in the queue. // if (_freeSyncChannels.Count <= 3) { // // Since we don't close the channel, verify that // the RemoveAndReleaseQueue is empty for resources. // Debug.Assert(channel.IsRemoveAndReleaseQueueEmpty()); _freeSyncChannels.Enqueue(channel); } else { channel.Close(); } } ////// Flushes the remove and release queues of all channels /// ////// Critical - Performs commands on channels. /// TreatAsSafe - Flushing commands that have already been enqueued is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void FlushAllRemoveReleaseQueues() { FlushRemoveAndReleaseQueues(_asyncChannel); FlushRemoveAndReleaseQueues(_asyncOutOfBandChannel); } ////// Private helper function to flush the remove and release queues of a channel /// ////// Critical - Performs commands on channels. /// [SecurityCritical] private void FlushRemoveAndReleaseQueues(DUCE.Channel channel) { if (channel != null) { channel.ProcessRemoveCommand(); channel.ProcessReleaseCommand(); channel.ClearRemoveAndReleaseQueue(); } } ////// Returns the asynchronous channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel Channel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncChannel; } } ////// Returns the asynchronous out-of-band channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel OutOfBandChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncOutOfBandChannel; } } ////// The asynchronous channel. /// ////// This field will be replaced with an asynchronous channel table as per task #26681. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncChannel; ////// The asynchronous out-of-band channel. /// ////// This is needed by CompositionTarget for handling WM_PAINT, etc. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncOutOfBandChannel; ////// We store the free synchronous channels for later re-use in this queue. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private Queue_freeSyncChannels; /// /// The service channel to the synchronous partition. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _syncServiceChannel; ////// Pointer to the unmanaged transport object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncPacketTransport; ////// Pointer to the unmanaged connection object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncConnection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
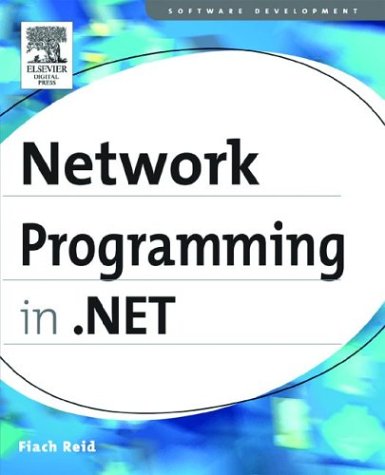
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementIsland.cs
- RawStylusSystemGestureInputReport.cs
- InputQueueChannelAcceptor.cs
- IdentifierCreationService.cs
- EdmProperty.cs
- CatalogPartDesigner.cs
- IgnoreFileBuildProvider.cs
- JsonServiceDocumentSerializer.cs
- AspNetHostingPermission.cs
- ChtmlTextWriter.cs
- PolicyLevel.cs
- MenuStrip.cs
- BitVector32.cs
- HttpResponse.cs
- ImageBrush.cs
- nulltextnavigator.cs
- HtmlControlAdapter.cs
- DetailsViewDeletedEventArgs.cs
- FileUpload.cs
- Mutex.cs
- DesignerDataTable.cs
- KeyValueSerializer.cs
- ScaleTransform3D.cs
- ValidationSummary.cs
- COM2Enum.cs
- SrgsSemanticInterpretationTag.cs
- HttpDictionary.cs
- AuthenticationModuleElement.cs
- DesignColumnCollection.cs
- DateTimeAutomationPeer.cs
- FieldInfo.cs
- RegexTree.cs
- GroupBoxAutomationPeer.cs
- GridViewColumn.cs
- IListConverters.cs
- AnnotationObservableCollection.cs
- UnauthorizedWebPart.cs
- StartUpEventArgs.cs
- SecurityUtils.cs
- FirstMatchCodeGroup.cs
- Quaternion.cs
- RootAction.cs
- Calendar.cs
- CompositeDataBoundControl.cs
- DataServiceHost.cs
- _BaseOverlappedAsyncResult.cs
- Accessible.cs
- Ticks.cs
- PathSegment.cs
- QueryCreatedEventArgs.cs
- lengthconverter.cs
- ToolboxItemCollection.cs
- ECDiffieHellmanCngPublicKey.cs
- DataGridToolTip.cs
- KeyValueConfigurationElement.cs
- Command.cs
- IMembershipProvider.cs
- DataGridTextBoxColumn.cs
- FormView.cs
- SchemaInfo.cs
- ClockController.cs
- HtmlAnchor.cs
- SafeIUnknown.cs
- DbProviderFactory.cs
- VariableDesigner.xaml.cs
- ReadOnlyDataSource.cs
- SqlWebEventProvider.cs
- FacetValueContainer.cs
- ImpersonationContext.cs
- Identity.cs
- DataSourceControl.cs
- _SpnDictionary.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ObfuscateAssemblyAttribute.cs
- XmlDataSourceNodeDescriptor.cs
- DescendentsWalkerBase.cs
- ConfigUtil.cs
- ToolbarAUtomationPeer.cs
- OpenTypeCommon.cs
- OracleBinary.cs
- WebServiceAttribute.cs
- PaintEvent.cs
- WebPartMenuStyle.cs
- SQLGuid.cs
- BuildDependencySet.cs
- XmlTypeAttribute.cs
- PropertyFilterAttribute.cs
- _AutoWebProxyScriptEngine.cs
- ConfigurationSettings.cs
- MILUtilities.cs
- RtfToXamlReader.cs
- MasterPage.cs
- Configuration.cs
- MetabaseServerConfig.cs
- JsonFormatReaderGenerator.cs
- AsyncInvokeOperation.cs
- DataRecordObjectView.cs
- Html32TextWriter.cs
- DataViewSetting.cs
- Logging.cs