Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / WindowsSecurityToken.cs / 1305376 / WindowsSecurityToken.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Security.Principal; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; public class WindowsSecurityToken : SecurityToken, IDisposable { string authenticationType; string id; DateTime effectiveTime; DateTime expirationTime; WindowsIdentity windowsIdentity; bool disposed = false; public WindowsSecurityToken(WindowsIdentity windowsIdentity) : this(windowsIdentity, SecurityUniqueId.Create().Value) { } public WindowsSecurityToken(WindowsIdentity windowsIdentity, string id) : this(windowsIdentity, id, null) { } public WindowsSecurityToken(WindowsIdentity windowsIdentity, string id, string authenticationType) { DateTime effectiveTime = DateTime.UtcNow; Initialize( id, authenticationType, effectiveTime, DateTime.UtcNow.AddHours( 10 ), windowsIdentity, true ); } protected WindowsSecurityToken() { } protected void Initialize(string id, DateTime effectiveTime, DateTime expirationTime, WindowsIdentity windowsIdentity, bool clone) { Initialize( id, null, effectiveTime, expirationTime, windowsIdentity, clone ); } protected void Initialize(string id, string authenticationType, DateTime effectiveTime, DateTime expirationTime, WindowsIdentity windowsIdentity, bool clone) { if (windowsIdentity == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("windowsIdentity"); if (id == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("id"); this.id = id; this.authenticationType = authenticationType; this.effectiveTime = effectiveTime; this.expirationTime = expirationTime; this.windowsIdentity = clone ? SecurityUtils.CloneWindowsIdentityIfNecessary(windowsIdentity, authenticationType) : windowsIdentity; } public override string Id { get { return this.id; } } public string AuthenticationType { get { return this.authenticationType; } } public override DateTime ValidFrom { get { return this.effectiveTime; } } public override DateTime ValidTo { get { return this.expirationTime; } } public virtual WindowsIdentity WindowsIdentity { get { ThrowIfDisposed(); return this.windowsIdentity; } } public override ReadOnlyCollectionSecurityKeys { get { return EmptyReadOnlyCollection .Instance; } } public virtual void Dispose() { if (!this.disposed) { this.disposed = true; if (this.windowsIdentity != null) { this.windowsIdentity.Dispose(); this.windowsIdentity = null; } } } protected void ThrowIfDisposed() { if (this.disposed) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.GetType().FullName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Security.Principal; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; public class WindowsSecurityToken : SecurityToken, IDisposable { string authenticationType; string id; DateTime effectiveTime; DateTime expirationTime; WindowsIdentity windowsIdentity; bool disposed = false; public WindowsSecurityToken(WindowsIdentity windowsIdentity) : this(windowsIdentity, SecurityUniqueId.Create().Value) { } public WindowsSecurityToken(WindowsIdentity windowsIdentity, string id) : this(windowsIdentity, id, null) { } public WindowsSecurityToken(WindowsIdentity windowsIdentity, string id, string authenticationType) { DateTime effectiveTime = DateTime.UtcNow; Initialize( id, authenticationType, effectiveTime, DateTime.UtcNow.AddHours( 10 ), windowsIdentity, true ); } protected WindowsSecurityToken() { } protected void Initialize(string id, DateTime effectiveTime, DateTime expirationTime, WindowsIdentity windowsIdentity, bool clone) { Initialize( id, null, effectiveTime, expirationTime, windowsIdentity, clone ); } protected void Initialize(string id, string authenticationType, DateTime effectiveTime, DateTime expirationTime, WindowsIdentity windowsIdentity, bool clone) { if (windowsIdentity == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("windowsIdentity"); if (id == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("id"); this.id = id; this.authenticationType = authenticationType; this.effectiveTime = effectiveTime; this.expirationTime = expirationTime; this.windowsIdentity = clone ? SecurityUtils.CloneWindowsIdentityIfNecessary(windowsIdentity, authenticationType) : windowsIdentity; } public override string Id { get { return this.id; } } public string AuthenticationType { get { return this.authenticationType; } } public override DateTime ValidFrom { get { return this.effectiveTime; } } public override DateTime ValidTo { get { return this.expirationTime; } } public virtual WindowsIdentity WindowsIdentity { get { ThrowIfDisposed(); return this.windowsIdentity; } } public override ReadOnlyCollection SecurityKeys { get { return EmptyReadOnlyCollection .Instance; } } public virtual void Dispose() { if (!this.disposed) { this.disposed = true; if (this.windowsIdentity != null) { this.windowsIdentity.Dispose(); this.windowsIdentity = null; } } } protected void ThrowIfDisposed() { if (this.disposed) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.GetType().FullName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
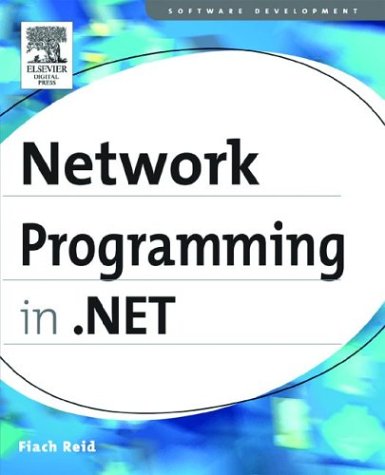
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDisplayMode.cs
- SwitchElementsCollection.cs
- XmlEntityReference.cs
- RegexMatchCollection.cs
- FocusWithinProperty.cs
- DataGridViewColumnStateChangedEventArgs.cs
- ProxyElement.cs
- ReadOnlyCollectionBase.cs
- SessionStateSection.cs
- HtmlWindowCollection.cs
- SecureStringHasher.cs
- ProcessInfo.cs
- AttributeCollection.cs
- AssemblyHash.cs
- MessageParameterAttribute.cs
- ListBoxItemWrapperAutomationPeer.cs
- OneWayChannelFactory.cs
- RowToParametersTransformer.cs
- TraceContextEventArgs.cs
- TextCharacters.cs
- StorageTypeMapping.cs
- InputScopeManager.cs
- DataControlFieldCell.cs
- ConfigXmlCDataSection.cs
- OleDbRowUpdatingEvent.cs
- CodeLabeledStatement.cs
- MDIClient.cs
- ZipIOExtraFieldElement.cs
- BuildProviderAppliesToAttribute.cs
- EngineSiteSapi.cs
- ContentDisposition.cs
- QilReference.cs
- DependencyPropertyChangedEventArgs.cs
- PerformanceCountersElement.cs
- WizardDesigner.cs
- TextBounds.cs
- Internal.cs
- DashStyle.cs
- HighlightComponent.cs
- AspNetRouteServiceHttpHandler.cs
- OdbcConnectionStringbuilder.cs
- RepeatInfo.cs
- DataRecordObjectView.cs
- MetaChildrenColumn.cs
- XPathMultyIterator.cs
- PasswordTextContainer.cs
- Binding.cs
- Int32CollectionConverter.cs
- CustomBindingElement.cs
- VisualStyleInformation.cs
- Hash.cs
- StylusEditingBehavior.cs
- StrokeNodeEnumerator.cs
- RestrictedTransactionalPackage.cs
- HttpCachePolicyElement.cs
- ZipIOExtraFieldZip64Element.cs
- itemelement.cs
- SQLResource.cs
- EraserBehavior.cs
- BrowserCapabilitiesFactory.cs
- ProviderSettingsCollection.cs
- SystemException.cs
- BufferedResponseStream.cs
- Condition.cs
- DesignerTransactionCloseEvent.cs
- DropDownList.cs
- PageCache.cs
- CSharpCodeProvider.cs
- EntityStoreSchemaGenerator.cs
- MenuItem.cs
- ControlTemplate.cs
- XmlHierarchicalDataSourceView.cs
- ClientRoleProvider.cs
- processwaithandle.cs
- ClientSponsor.cs
- Int32EqualityComparer.cs
- XPathArrayIterator.cs
- XsdCachingReader.cs
- EnumType.cs
- SinglePageViewer.cs
- EdmSchemaAttribute.cs
- ImageClickEventArgs.cs
- CacheEntry.cs
- connectionpool.cs
- WindowsTreeView.cs
- CollectionType.cs
- SqlComparer.cs
- FullTrustAssembly.cs
- HttpRuntime.cs
- TaskHelper.cs
- GenerateTemporaryAssemblyTask.cs
- entityreference_tresulttype.cs
- FileDataSourceCache.cs
- IndependentAnimationStorage.cs
- CFStream.cs
- WebPartCancelEventArgs.cs
- QueryCacheManager.cs
- ChannelDispatcherCollection.cs
- ReadOnlyTernaryTree.cs
- RegistryKey.cs