Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Configuration / SmtpNetworkElement.cs / 1 / SmtpNetworkElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Net; using System.Net.Mail; using System.Reflection; using System.Security.Permissions; public sealed class SmtpNetworkElement : ConfigurationElement { public SmtpNetworkElement() { this.properties.Add(this.defaultCredentials); this.properties.Add(this.host); this.properties.Add(this.password); this.properties.Add(this.port); this.properties.Add(this.userName); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation portPropertyInfo = ElementInformation.Properties[ConfigurationStrings.Port]; if (portPropertyInfo.ValueOrigin == PropertyValueOrigin.SetHere && (int)portPropertyInfo.Value != (int)portPropertyInfo.DefaultValue) { try { (new SmtpPermission(SmtpAccess.ConnectToUnrestrictedPort)).Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, portPropertyInfo.Name), exception); } } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } [ConfigurationProperty(ConfigurationStrings.DefaultCredentials, DefaultValue = false)] public bool DefaultCredentials { get { return (bool)this[this.defaultCredentials]; } set { this[this.defaultCredentials] = value; } } [ConfigurationProperty(ConfigurationStrings.Host)] public string Host { get { return (string)this[this.host]; } set { this[this.host] = value; } } [ConfigurationProperty(ConfigurationStrings.Password)] public string Password { get { return (string)this[this.password]; } set { this[this.password] = value; } } [ConfigurationProperty(ConfigurationStrings.Port, DefaultValue = 25)] public int Port { get { return (int)this[this.port]; } set { // this[this.port] = value; } } [ConfigurationProperty(ConfigurationStrings.UserName)] public string UserName { get { return (string)this[this.userName]; } set { this[this.userName] = value; } } // ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty defaultCredentials = new ConfigurationProperty(ConfigurationStrings.DefaultCredentials, typeof(bool), false, ConfigurationPropertyOptions.None); readonly ConfigurationProperty host = new ConfigurationProperty(ConfigurationStrings.Host, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty password = new ConfigurationProperty(ConfigurationStrings.Password, typeof(string), null, ConfigurationPropertyOptions.None); readonly ConfigurationProperty port = new ConfigurationProperty(ConfigurationStrings.Port, typeof(int), 25, null, new IntegerValidator(IPEndPoint.MinPort+1, IPEndPoint.MaxPort), ConfigurationPropertyOptions.None); readonly ConfigurationProperty userName = new ConfigurationProperty(ConfigurationStrings.UserName, typeof(string), null, ConfigurationPropertyOptions.None); } internal sealed class SmtpNetworkElementInternal { internal SmtpNetworkElementInternal(SmtpNetworkElement element) { this.host = element.Host; this.port = element.Port; if (element.DefaultCredentials) { this.credential = (NetworkCredential)CredentialCache.DefaultCredentials; } else if (element.UserName != null && element.UserName.Length > 0) { this.credential = new NetworkCredential(element.UserName, element.Password); } } internal NetworkCredential Credential { get { return this.credential; } } internal string Host { get { return this.host; } } internal int Port { get { return this.port; } } string host; int port; NetworkCredential credential = null; } }
Link Menu
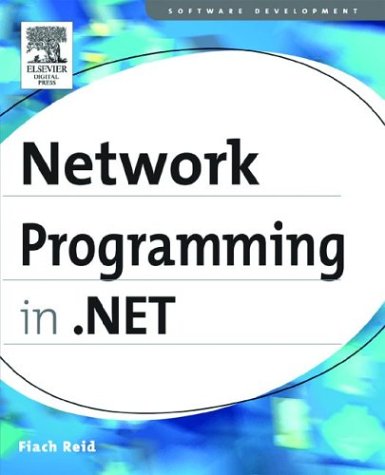
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextCompositionEventArgs.cs
- MsmqAppDomainProtocolHandler.cs
- Compensation.cs
- WebPartActionVerb.cs
- SubpageParagraph.cs
- FacetChecker.cs
- Rectangle.cs
- TrustLevel.cs
- FixedElement.cs
- BinHexEncoder.cs
- xdrvalidator.cs
- DetailsViewRow.cs
- MsmqIntegrationInputChannel.cs
- TextWriterTraceListener.cs
- CreateUserWizard.cs
- Currency.cs
- DocumentSequence.cs
- DataBindingCollection.cs
- EntityCommand.cs
- PrintEvent.cs
- SizeAnimationBase.cs
- ProtectedConfigurationSection.cs
- Aggregates.cs
- WebPartMovingEventArgs.cs
- FunctionOverloadResolver.cs
- StyleCollection.cs
- RectKeyFrameCollection.cs
- AddInBase.cs
- OptimizedTemplateContent.cs
- shaper.cs
- EntityClassGenerator.cs
- ValueQuery.cs
- XmlTextReader.cs
- CodeExpressionCollection.cs
- SpecialTypeDataContract.cs
- WebBrowserHelper.cs
- CompositionDesigner.cs
- DbgUtil.cs
- manifestimages.cs
- CheckableControlBaseAdapter.cs
- ProxyManager.cs
- MenuEventArgs.cs
- counter.cs
- BoundField.cs
- SqlCacheDependencyDatabase.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- PerfCounters.cs
- DropShadowBitmapEffect.cs
- Timer.cs
- UIElement.cs
- LabelLiteral.cs
- LocalizableResourceBuilder.cs
- Atom10FormatterFactory.cs
- basevalidator.cs
- PaintValueEventArgs.cs
- XamlGridLengthSerializer.cs
- FieldNameLookup.cs
- SoapSchemaExporter.cs
- TreeViewImageIndexConverter.cs
- PassportIdentity.cs
- ToolStripGripRenderEventArgs.cs
- FontUnitConverter.cs
- BufferedStream2.cs
- SoundPlayerAction.cs
- Command.cs
- PointConverter.cs
- DesignerObjectListAdapter.cs
- HeaderElement.cs
- GenericIdentity.cs
- ScriptingScriptResourceHandlerSection.cs
- ObjectViewQueryResultData.cs
- DataControlFieldCollection.cs
- DispatcherOperation.cs
- Attributes.cs
- HwndSubclass.cs
- LingerOption.cs
- LongCountAggregationOperator.cs
- ExpressionEvaluator.cs
- BasicHttpMessageCredentialType.cs
- WpfGeneratedKnownTypes.cs
- FamilyTypeface.cs
- RotateTransform.cs
- SerializationFieldInfo.cs
- EntityDataSourceChangingEventArgs.cs
- AsyncCompletedEventArgs.cs
- listitem.cs
- HtmlInputFile.cs
- SymbolEqualComparer.cs
- CannotUnloadAppDomainException.cs
- ProfilePropertySettingsCollection.cs
- ColorContext.cs
- SqlCacheDependencySection.cs
- WriteStateInfoBase.cs
- DataServiceQueryException.cs
- HandlerFactoryCache.cs
- TemplateXamlParser.cs
- TreeViewItemAutomationPeer.cs
- ConfigurationConverterBase.cs
- QuaternionIndependentAnimationStorage.cs
- ProbeDuplexCD1AsyncResult.cs