Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RequestSecurityTokenForRemoteTokenFactory.cs / 1 / RequestSecurityTokenForRemoteTokenFactory.cs
namespace Microsoft.InfoCards { using System; using System.Xml; using System.Collections; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This derviced variant of RequestSecurityToken is used to generate an RST to the remote token factory. // internal class RequestSecurityTokenForRemoteTokenFactory : RequestSecurityToken { public RequestSecurityTokenForRemoteTokenFactory( RequestSecurityTokenParameters rstParams ) : base( rstParams ) { } protected override void WriteAppliesToElement() { switch( AppliesToBehaviorDecisionTable.GetAppliesToBehaviorDecisionForRst( Policy, m_rstParams.Card.RequireAppliesto ) ) { case AppliesToBehaviorDecision.SendRPAppliesTo: IDT.TraceDebug( "IPSTSCLIENT: Writing RP AppliesTo to RST" ); Serializer.WriteAppliesToElement( Policy.PolicyAppliesTo, m_rstParams.Version ); break; case AppliesToBehaviorDecision.SendCustomAppliesTo: IDT.TraceDebug( "IPSTSCLIENT: Writing Recipient Identity to RST to create a custom AppliesTo" ); Serializer.WriteAppliesToElement( Policy.ImmediateTokenRecipient.Address, m_rstParams.Version ); break; default: // // Do not write AppliesTo // break; } } protected override void WriteSecondaryParametersElement() { // // Write out a copy of the policy XML to the SecondaryParameters element if we are using the // oasis 2007 version of WS-Trust. // if( XmlNames.WSSpecificationVersion.WSTrustOasis2007 == ProtocolVersionProfile.WSTrust.Version ) { // // If the policy contains optional claims but the user has elected to not sent optional claims, then we // cannot sent secondaryParameters (as we do not want accidental disclosure of information to a // non-auditing STS). // bool writeSeconaryParameters = true; if( Policy.OptionalClaims.Length > 0 ) { // // If the policy contains one or more optional claims, check to see if the user // is willing to send optional claims. // writeSeconaryParameters = m_rstParams.DiscloseOptionalClaims && !( OptionalClaimsExceedCard( m_rstParams.Card, m_rstParams.Policy ) ); } // // Write the secondaryParameters element only when AppliesTo is present // switch( AppliesToBehaviorDecisionTable.GetAppliesToBehaviorDecisionForRst( Policy, m_rstParams.Card.RequireAppliesto ) ) { case AppliesToBehaviorDecision.SendRPAppliesTo: case AppliesToBehaviorDecision.SendCustomAppliesTo: break; default: // // Do not write SecondaryParameters as the appliesTo decision is either 'not send' or 'failed match' // writeSeconaryParameters = false; break; } if( writeSeconaryParameters ) { if( null != Policy.RelyingPartyPolicy ) { Serializer.WriteSecondaryParametersElement( Policy.RelyingPartyPolicy.PolicyXml ); } else { // // If the incoming RST Template did not contain SecondaryParameters, we simply write the // original request. // Serializer.WriteSecondaryParametersElement( Policy.ClientPolicy.PolicyXml ); } } } } protected override void CustomWriteBodyContents( XmlDictionaryWriter writer ) { InitializeWriters( writer ); WriteRSTOpeningElement(); WriteRequestTypeElement(); WriteInfoCardReferenceElement(); WriteClaimsElement(); WriteKeyTypeElement(); WriteKeySupportingElements(); WriteAppliesToElement(); WritePPIDElement(); WriteEncryptionAlgorithmElement(); WritePassOnElements(); WriteDisplayTokenElement(); WriteUnprocessedPolicyElements(); WriteSecondaryParametersElement(); WriteEndElement(); } // // Summary // Checks to see if the optional claims in the policy exceed the claims present in the card. // // Returns // True if at least one optional claim in the policy is not present in the card. False otherwise. // private bool OptionalClaimsExceedCard( InfoCard card, InfoCardPolicy policy ) { // // Compare the set of requested optional claims in the policy against the card's claims. // InfoCardClaimCollection claims = card.GetClaims(); bool claimNotFoundInCard = false; foreach( string claimUri in policy.OptionalClaims ) { if( !claims.ContainsKey( claimUri ) ) { claimNotFoundInCard = true; } } return claimNotFoundInCard; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
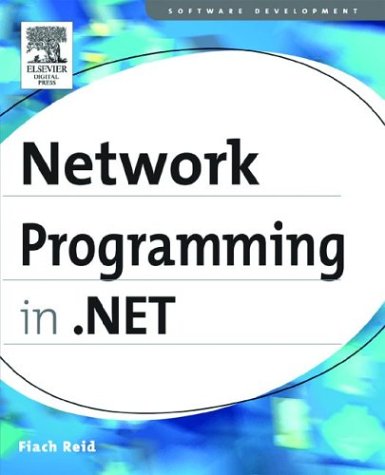
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- listitem.cs
- CharacterHit.cs
- StateBag.cs
- SoapFault.cs
- HtmlInputRadioButton.cs
- PlainXmlDeserializer.cs
- HttpInputStream.cs
- XmlQueryCardinality.cs
- OverflowException.cs
- HostingEnvironment.cs
- WebPartUserCapability.cs
- UnmanagedMemoryStreamWrapper.cs
- TextElementCollectionHelper.cs
- AutoCompleteStringCollection.cs
- SweepDirectionValidation.cs
- DescriptionAttribute.cs
- CharacterBufferReference.cs
- XomlCompilerError.cs
- SettingsPropertyCollection.cs
- SmtpReplyReaderFactory.cs
- AppDomainProtocolHandler.cs
- TimeStampChecker.cs
- DataQuery.cs
- CompilerTypeWithParams.cs
- InProcStateClientManager.cs
- DataFormats.cs
- FixedFlowMap.cs
- WindowsFormsHostPropertyMap.cs
- CollectionBase.cs
- XmlDigitalSignatureProcessor.cs
- BitmapEffectGeneralTransform.cs
- ImpersonateTokenRef.cs
- WebZoneDesigner.cs
- StreamWriter.cs
- ExpressionPrefixAttribute.cs
- StreamGeometry.cs
- AbsoluteQuery.cs
- HtmlForm.cs
- AliasExpr.cs
- StyleSelector.cs
- UnregisterInfo.cs
- MembershipAdapter.cs
- Color.cs
- CharacterShapingProperties.cs
- ByteStorage.cs
- SafeEventLogWriteHandle.cs
- EventManager.cs
- PropertyGridEditorPart.cs
- ButtonFieldBase.cs
- Int32AnimationBase.cs
- WizardStepBase.cs
- Msec.cs
- Command.cs
- DeploymentExceptionMapper.cs
- ResourceDescriptionAttribute.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- SHA384Managed.cs
- FlowPosition.cs
- ReaderContextStackData.cs
- GlyphsSerializer.cs
- XmlIterators.cs
- Renderer.cs
- ProxyHelper.cs
- CheckBoxDesigner.cs
- NetworkAddressChange.cs
- AsyncResult.cs
- MonitoringDescriptionAttribute.cs
- ToolBarButtonClickEvent.cs
- ConvertersCollection.cs
- TiffBitmapEncoder.cs
- DataGridTable.cs
- GradientStopCollection.cs
- BooleanToVisibilityConverter.cs
- ObjectNavigationPropertyMapping.cs
- OleDbCommand.cs
- SqlGenericUtil.cs
- Run.cs
- HttpServerVarsCollection.cs
- XamlTypeWithExplicitNamespace.cs
- EntityClassGenerator.cs
- ExpressionBinding.cs
- Padding.cs
- StreamHelper.cs
- ValueConversionAttribute.cs
- Size.cs
- BulletedList.cs
- EnumValAlphaComparer.cs
- EnumBuilder.cs
- ConnectionString.cs
- FlowLayout.cs
- DbConnectionPoolOptions.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- ReadOnlyHierarchicalDataSourceView.cs
- BaseCAMarshaler.cs
- AnnotationResource.cs
- ObjectHandle.cs
- ValueUnavailableException.cs
- StylusSystemGestureEventArgs.cs
- OpenTypeMethods.cs
- latinshape.cs