Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / DataServiceQueryProvider.cs / 1 / DataServiceQueryProvider.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)ci.Invoke(args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///the resulting Uri internal Uri Translate(Expression e) { // short cut analysis if just a resource set if (!(e is ResourceSetExpression)) { e = Evaluator.PartialEval(e); e = new ExpressionNormalizer().Visit(e); e = ResourceBinder.Bind(e); } string translation = UriWriter.Translate(this.Context, e); return Util.CreateUri(this.Context.BaseUriWithSlash, Util.CreateUri(translation, UriKind.Relative)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)ci.Invoke(args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///the resulting Uri internal Uri Translate(Expression e) { // short cut analysis if just a resource set if (!(e is ResourceSetExpression)) { e = Evaluator.PartialEval(e); e = new ExpressionNormalizer().Visit(e); e = ResourceBinder.Bind(e); } string translation = UriWriter.Translate(this.Context, e); return Util.CreateUri(this.Context.BaseUriWithSlash, Util.CreateUri(translation, UriKind.Relative)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
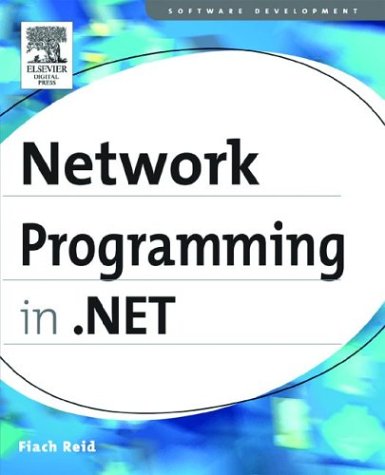
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Trace.cs
- TaskFormBase.cs
- XmlSerializationWriter.cs
- TimeSpanConverter.cs
- Cloud.cs
- EmbeddedMailObject.cs
- MemberPath.cs
- ProfileGroupSettingsCollection.cs
- PolyBezierSegmentFigureLogic.cs
- SqlCacheDependency.cs
- RayHitTestParameters.cs
- Geometry.cs
- ContentDisposition.cs
- CustomCategoryAttribute.cs
- TextDecorationCollection.cs
- QueryParameter.cs
- activationcontext.cs
- X509RawDataKeyIdentifierClause.cs
- RegexRunnerFactory.cs
- OdbcConnectionHandle.cs
- OleAutBinder.cs
- TdsRecordBufferSetter.cs
- ListBindingConverter.cs
- TextRangeEdit.cs
- StorageRoot.cs
- XmlBoundElement.cs
- WebPermission.cs
- VScrollProperties.cs
- RadioButtonBaseAdapter.cs
- StreamSecurityUpgradeInitiatorBase.cs
- PreservationFileWriter.cs
- Matrix.cs
- StylusEventArgs.cs
- StatusBarDrawItemEvent.cs
- TimeManager.cs
- baseaxisquery.cs
- SqlDataSourceCommandEventArgs.cs
- CommittableTransaction.cs
- Directory.cs
- Clipboard.cs
- ToolStripItemImageRenderEventArgs.cs
- CertificateManager.cs
- BaseValidator.cs
- IconBitmapDecoder.cs
- DisplayNameAttribute.cs
- SimpleType.cs
- MenuItemStyleCollection.cs
- DocumentViewer.cs
- MethodImplAttribute.cs
- loginstatus.cs
- TextBoxBase.cs
- HebrewNumber.cs
- SerializationAttributes.cs
- XmlSignatureProperties.cs
- RotateTransform3D.cs
- HeaderCollection.cs
- BaseTemplatedMobileComponentEditor.cs
- BufferedWebEventProvider.cs
- ClientTarget.cs
- TextServicesLoader.cs
- ValidationErrorEventArgs.cs
- TextClipboardData.cs
- ScriptingRoleServiceSection.cs
- WindowsListViewGroupHelper.cs
- DrawingCollection.cs
- SystemResourceHost.cs
- UseAttributeSetsAction.cs
- Compiler.cs
- PrivilegeNotHeldException.cs
- Overlapped.cs
- LexicalChunk.cs
- DataSysAttribute.cs
- XamlPathDataSerializer.cs
- DeviceSpecificDialogCachedState.cs
- HtmlProps.cs
- DependentList.cs
- InputGestureCollection.cs
- IndexerNameAttribute.cs
- Section.cs
- ConfigViewGenerator.cs
- CqlGenerator.cs
- Semaphore.cs
- PopupControlService.cs
- StaticDataManager.cs
- WorkflowHostingResponseContext.cs
- AccessedThroughPropertyAttribute.cs
- DefaultPropertyAttribute.cs
- DataRowView.cs
- CodeMemberField.cs
- _BufferOffsetSize.cs
- MethodRental.cs
- SelfIssuedSamlTokenFactory.cs
- MetadataArtifactLoaderComposite.cs
- NativeMethods.cs
- OleAutBinder.cs
- ReflectionPermission.cs
- BitmapMetadataBlob.cs
- WebPartTransformer.cs
- CodeCastExpression.cs
- ByteFacetDescriptionElement.cs