Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Commands / CommandHelpers.cs / 1 / CommandHelpers.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Windows.Media; using System.Security; using System.Security.Permissions; namespace MS.Internal.Commands { internal static class CommandHelpers { internal static void RegisterCommandHandler(Type controlType, RoutedCommand command, ExecutedRoutedEventHandler executedRoutedEventHandler, params InputGesture[] inputGestures) { RegisterCommandHandler(controlType, command, executedRoutedEventHandler, null, inputGestures); } internal static void RegisterCommandHandler(Type controlType, RoutedCommand command, Key key, ModifierKeys modifierKeys, ExecutedRoutedEventHandler executedRoutedEventHandler, CanExecuteRoutedEventHandler canExecuteRoutedEventHandler) { RegisterCommandHandler(controlType, command, executedRoutedEventHandler, canExecuteRoutedEventHandler, new KeyGesture(key, modifierKeys)); } internal static void RegisterCommandHandler(Type controlType, RoutedCommand command, ExecutedRoutedEventHandler executedRoutedEventHandler, CanExecuteRoutedEventHandler canExecuteRoutedEventHandler, params InputGesture[] inputGestures) { // Validate parameters Debug.Assert(controlType != null); Debug.Assert(command != null); Debug.Assert(executedRoutedEventHandler != null); // All other parameters may be null // Create command link for this command CommandManager.RegisterClassCommandBinding(controlType, new CommandBinding(command, executedRoutedEventHandler, canExecuteRoutedEventHandler)); // Create additional input binding for this command for (int i = 0; i < inputGestures.Length; i++) { CommandManager.RegisterClassInputBinding(controlType, new InputBinding(command, inputGestures[i])); } } internal static bool CanExecuteCommandSource(ICommandSource commandSource) { ICommand command = commandSource.Command; if (command != null) { object parameter = commandSource.CommandParameter; IInputElement target = commandSource.CommandTarget; RoutedCommand routed = command as RoutedCommand; if (routed != null) { if (target == null) { target = commandSource as IInputElement; } return routed.CanExecute(parameter, target); } else { return command.CanExecute(parameter); } } return false; } ////// Executes the command on the given command source. /// ////// Critical - calls critical function (ExecuteCommandSource) /// TreatAsSafe - always passes in false for userInitiated, which is safe /// [SecurityCritical, SecurityTreatAsSafe] internal static void ExecuteCommandSource(ICommandSource commandSource) { CriticalExecuteCommandSource(commandSource, false); } ////// Executes the command on the given command source. /// ////// Critical - sets the user initiated bit on a command, which is used /// for security purposes later. It is important to validate /// the callers of this, and the implementation to make sure /// that we only call MarkAsUserInitiated in the correct cases. /// [SecurityCritical] internal static void CriticalExecuteCommandSource(ICommandSource commandSource, bool userInitiated) { ICommand command = commandSource.Command; if (command != null) { object parameter = commandSource.CommandParameter; IInputElement target = commandSource.CommandTarget; RoutedCommand routed = command as RoutedCommand; if (routed != null) { if (target == null) { target = commandSource as IInputElement; } if (routed.CanExecute(parameter, target)) { routed.ExecuteCore(parameter, target, userInitiated); } } else if (command.CanExecute(parameter)) { command.Execute(parameter); } } } // This allows a caller to override its ICommandSource values (used by Button and ScrollBar) internal static void ExecuteCommand(ICommand command, object parameter, IInputElement target) { RoutedCommand routed = command as RoutedCommand; if (routed != null) { if (routed.CanExecute(parameter, target)) { routed.Execute(parameter, target); } } else if (command.CanExecute(parameter)) { command.Execute(parameter); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
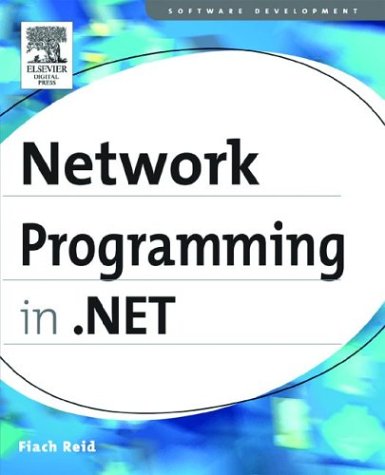
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseAsyncResult.cs
- XmlCharacterData.cs
- TimeoutTimer.cs
- MSAAWinEventWrap.cs
- ImageListDesigner.cs
- SaveWorkflowAsyncResult.cs
- SystemIPv6InterfaceProperties.cs
- TrackingProfile.cs
- ArrangedElementCollection.cs
- TextServicesDisplayAttribute.cs
- SqlTriggerAttribute.cs
- FormsAuthentication.cs
- TextRangeSerialization.cs
- HtmlInputButton.cs
- NodeInfo.cs
- DictionarySectionHandler.cs
- GetCryptoTransformRequest.cs
- SizeLimitedCache.cs
- Inflater.cs
- MouseActionConverter.cs
- FlatButtonAppearance.cs
- ValidationService.cs
- StringExpressionSet.cs
- AddressingProperty.cs
- SynchronizedInputHelper.cs
- NavigatingCancelEventArgs.cs
- SelectiveScrollingGrid.cs
- FastPropertyAccessor.cs
- ExpressionPrefixAttribute.cs
- XsdDuration.cs
- COM2IProvidePropertyBuilderHandler.cs
- Stacktrace.cs
- CodeLabeledStatement.cs
- ComponentChangingEvent.cs
- KeyboardNavigation.cs
- SafeBitVector32.cs
- ConstNode.cs
- TypeConverterAttribute.cs
- DBBindings.cs
- PasswordRecovery.cs
- EncodingInfo.cs
- ConstraintEnumerator.cs
- AnonymousIdentificationSection.cs
- XmlSecureResolver.cs
- AbandonedMutexException.cs
- OrderPreservingPipeliningSpoolingTask.cs
- EditorResources.cs
- MultiPageTextView.cs
- FontUnitConverter.cs
- ComplexType.cs
- LockCookie.cs
- EntityConnectionStringBuilder.cs
- RankException.cs
- XamlPathDataSerializer.cs
- Sentence.cs
- BitmapDownload.cs
- HtmlInputButton.cs
- LockRecursionException.cs
- EndpointDesigner.cs
- TrustSection.cs
- XPathMessageFilterElement.cs
- HostedElements.cs
- JsonFormatWriterGenerator.cs
- ReadOnlyHierarchicalDataSource.cs
- DataTableNewRowEvent.cs
- UserControlParser.cs
- DesignerDeviceConfig.cs
- Zone.cs
- XmlSchemaSimpleContent.cs
- ToolZone.cs
- DataObject.cs
- EventManager.cs
- DockAndAnchorLayout.cs
- FontFamilyValueSerializer.cs
- SourceElementsCollection.cs
- SmtpFailedRecipientsException.cs
- PerformanceCounterPermission.cs
- DataGridColumnsPage.cs
- ConfigurationConverterBase.cs
- BinaryNode.cs
- JavaScriptSerializer.cs
- StrongTypingException.cs
- LassoHelper.cs
- DbDataRecord.cs
- AccessorTable.cs
- RecordConverter.cs
- FunctionImportElement.cs
- AsynchronousChannel.cs
- ApplicationSecurityInfo.cs
- DefaultValidator.cs
- BamlVersionHeader.cs
- WrappedIUnknown.cs
- XPathNavigatorKeyComparer.cs
- ExpressionHelper.cs
- ToolStripStatusLabel.cs
- FileLogRecordEnumerator.cs
- CapabilitiesAssignment.cs
- Image.cs
- ThreadStaticAttribute.cs
- FormsAuthenticationConfiguration.cs