Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / SQLTypes / SQLBoolean.cs / 1 / SQLBoolean.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlBoolean.cs // // Create by: JunFang // // Purpose: Implementation of SqlBoolean which is equivalent to // data type "bit" in SQL Server // // Notes: // // History: // // 09/17/99 JunFang Created and implemented as first drop. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlBoolean : INullable, IComparable, IXmlSerializable { // m_value: 2 (true), 1 (false), 0 (unknown/Null) private byte m_value; private const byte x_Null = 0; private const byte x_False = 1; private const byte x_True = 2; // constructor ////// Represents an integer value that is either 1 or 0. /// ////// public SqlBoolean(bool value) { m_value = (byte)(value ? x_True : x_False); } ////// Initializes a new instance of the ///class. /// /// public SqlBoolean(int value) : this(value, false) { } private SqlBoolean(int value, bool fNull) { if (fNull) m_value = x_Null; else m_value = (value != 0) ? x_True : x_False; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return m_value == x_Null;} } // property: Value ////// Gets whether the current ///is . /// /// public bool Value { get { switch (m_value) { case x_True: return true; case x_False: return false; default: throw new SqlNullValueException(); } } } // property: IsTrue ////// Gets or sets the ///to be or /// . /// /// public bool IsTrue { get { return m_value == x_True;} } // property: IsFalse ////// Gets whether the current ///is . /// /// public bool IsFalse { get { return m_value == x_False;} } // Implicit conversion from bool to SqlBoolean ////// Gets whether the current ///is . /// /// public static implicit operator SqlBoolean(bool x) { return new SqlBoolean(x); } // Explicit conversion from SqlBoolean to bool. Throw exception if x is Null. ////// Converts a boolean to a ///. /// /// public static explicit operator bool(SqlBoolean x) { return x.Value; } // Unary operators ////// Converts a ////// to a boolean. /// /// public static SqlBoolean operator !(SqlBoolean x) { switch (x.m_value) { case x_True: return SqlBoolean.False; case x_False: return SqlBoolean.True; default: SQLDebug.Check(x.m_value == x_Null); return SqlBoolean.Null; } } ////// Performs a NOT operation on a ////// . /// /// public static bool operator true(SqlBoolean x) { return x.IsTrue; } ///[To be supplied.] ////// public static bool operator false(SqlBoolean x) { return x.IsFalse; } // Binary operators ///[To be supplied.] ////// public static SqlBoolean operator &(SqlBoolean x, SqlBoolean y) { if (x.m_value == x_False || y.m_value == x_False) return SqlBoolean.False; else if (x.m_value == x_True && y.m_value == x_True) return SqlBoolean.True; else return SqlBoolean.Null; } ////// Performs a bitwise AND operation on two instances of /// ////// . /// /// public static SqlBoolean operator |(SqlBoolean x, SqlBoolean y) { if (x.m_value == x_True || y.m_value == x_True) return SqlBoolean.True; else if (x.m_value == x_False && y.m_value == x_False) return SqlBoolean.False; else return SqlBoolean.Null; } // property: ByteValue ////// Performs /// a bitwise OR operation on two instances of a /// ////// . /// /// public byte ByteValue { get { if (!IsNull) return (m_value == x_True) ? (byte)1 : (byte)0; else throw new SqlNullValueException(); } } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : Value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlBoolean Parse(String s) { if (null == s) // Let Boolean.Parse throw exception return new SqlBoolean(Boolean.Parse(s)); if (s == SQLResource.NullString) return SqlBoolean.Null; s = s.TrimStart(); char wchFirst = s[0]; if (Char.IsNumber(wchFirst) || ('-' == wchFirst) || ('+' == wchFirst)) { return new SqlBoolean(Int32.Parse(s, (IFormatProvider)null)); } else { return new SqlBoolean(Boolean.Parse(s)); } } // Unary operators ///[To be supplied.] ////// public static SqlBoolean operator ~(SqlBoolean x) { return (!x); } // Binary operators ///[To be supplied.] ////// public static SqlBoolean operator ^(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? Null : new SqlBoolean(x.m_value != y.m_value); } // Implicit conversions // Explicit conversions // Explicit conversion from SqlByte to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlByte x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt16 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt16 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt32 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt32 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt64 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt64 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlDouble to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlDouble x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0.0); } // Explicit conversion from SqlSingle to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlSingle x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0.0); } // Explicit conversion from SqlMoney to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlMoney x) { return x.IsNull ? Null : (x != SqlMoney.Zero); } // Explicit conversion from SqlDecimal to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlDecimal x) { return x.IsNull ? SqlBoolean.Null : new SqlBoolean(x.m_data1 != 0 || x.m_data2 != 0 || x.m_data3 != 0 || x.m_data4 != 0); } // Explicit conversion from SqlString to SqlBoolean // Throws FormatException or OverflowException if necessary. ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlString x) { return x.IsNull ? Null : SqlBoolean.Parse(x.Value); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlBoolean x, SqlBoolean y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlBoolean OnesComplement(SqlBoolean x) { return ~x; } // Alternative method for operator & public static SqlBoolean And(SqlBoolean x, SqlBoolean y) { return x & y; } // Alternative method for operator | public static SqlBoolean Or(SqlBoolean x, SqlBoolean y) { return x | y; } // Alternative method for operator ^ public static SqlBoolean Xor(SqlBoolean x, SqlBoolean y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlBoolean x, SqlBoolean y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlBoolean x, SqlBoolean y) { return (x != y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlBoolean x, SqlBoolean y) { return (x > y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlBoolean x, SqlBoolean y) { return (x < y); } // Alternative method for operator <= public static SqlBoolean GreaterThanOrEquals(SqlBoolean x, SqlBoolean y) { return (x >= y); } // Alternative method for operator != public static SqlBoolean LessThanOrEquals(SqlBoolean x, SqlBoolean y) { return (x <= y); } // Alternative method for conversions. public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt16 ToSqlInt16() { return (SqlInt16)this; } public SqlInt32 ToSqlInt32() { return (SqlInt32)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlBoolean) { SqlBoolean i = (SqlBoolean)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlBoolean)); } public int CompareTo(SqlBoolean value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this.ByteValue < value.ByteValue) return -1; if (this.ByteValue > value.ByteValue) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlBoolean)) { return false; } SqlBoolean i = (SqlBoolean)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_value = x_Null; } else { m_value = (byte)(XmlConvert.ToBoolean(reader.ReadElementString()) ? x_True : x_False); } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(m_value == x_True ? "true" : "false"); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("boolean", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlBoolean True = new SqlBoolean(true); ////// Represents a true value that can be assigned to the /// ///property of an instance of /// the class. /// /// public static readonly SqlBoolean False = new SqlBoolean(false); ////// Represents a false value that can be assigned to the /// ///property of an instance of /// the class. /// /// public static readonly SqlBoolean Null = new SqlBoolean(0, true); ////// Represents a null value that can be assigned to the ///property of an instance of /// the class. /// /// public static readonly SqlBoolean Zero = new SqlBoolean(0); ///[To be supplied.] ////// public static readonly SqlBoolean One = new SqlBoolean(1); } // SqlBoolean } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlBoolean.cs // // Create by: JunFang // // Purpose: Implementation of SqlBoolean which is equivalent to // data type "bit" in SQL Server // // Notes: // // History: // // 09/17/99 JunFang Created and implemented as first drop. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Runtime.InteropServices; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [StructLayout(LayoutKind.Sequential)] [XmlSchemaProvider("GetXsdType")] public struct SqlBoolean : INullable, IComparable, IXmlSerializable { // m_value: 2 (true), 1 (false), 0 (unknown/Null) private byte m_value; private const byte x_Null = 0; private const byte x_False = 1; private const byte x_True = 2; // constructor ////// Represents an integer value that is either 1 or 0. /// ////// public SqlBoolean(bool value) { m_value = (byte)(value ? x_True : x_False); } ////// Initializes a new instance of the ///class. /// /// public SqlBoolean(int value) : this(value, false) { } private SqlBoolean(int value, bool fNull) { if (fNull) m_value = x_Null; else m_value = (value != 0) ? x_True : x_False; } // INullable ///[To be supplied.] ////// public bool IsNull { get { return m_value == x_Null;} } // property: Value ////// Gets whether the current ///is . /// /// public bool Value { get { switch (m_value) { case x_True: return true; case x_False: return false; default: throw new SqlNullValueException(); } } } // property: IsTrue ////// Gets or sets the ///to be or /// . /// /// public bool IsTrue { get { return m_value == x_True;} } // property: IsFalse ////// Gets whether the current ///is . /// /// public bool IsFalse { get { return m_value == x_False;} } // Implicit conversion from bool to SqlBoolean ////// Gets whether the current ///is . /// /// public static implicit operator SqlBoolean(bool x) { return new SqlBoolean(x); } // Explicit conversion from SqlBoolean to bool. Throw exception if x is Null. ////// Converts a boolean to a ///. /// /// public static explicit operator bool(SqlBoolean x) { return x.Value; } // Unary operators ////// Converts a ////// to a boolean. /// /// public static SqlBoolean operator !(SqlBoolean x) { switch (x.m_value) { case x_True: return SqlBoolean.False; case x_False: return SqlBoolean.True; default: SQLDebug.Check(x.m_value == x_Null); return SqlBoolean.Null; } } ////// Performs a NOT operation on a ////// . /// /// public static bool operator true(SqlBoolean x) { return x.IsTrue; } ///[To be supplied.] ////// public static bool operator false(SqlBoolean x) { return x.IsFalse; } // Binary operators ///[To be supplied.] ////// public static SqlBoolean operator &(SqlBoolean x, SqlBoolean y) { if (x.m_value == x_False || y.m_value == x_False) return SqlBoolean.False; else if (x.m_value == x_True && y.m_value == x_True) return SqlBoolean.True; else return SqlBoolean.Null; } ////// Performs a bitwise AND operation on two instances of /// ////// . /// /// public static SqlBoolean operator |(SqlBoolean x, SqlBoolean y) { if (x.m_value == x_True || y.m_value == x_True) return SqlBoolean.True; else if (x.m_value == x_False && y.m_value == x_False) return SqlBoolean.False; else return SqlBoolean.Null; } // property: ByteValue ////// Performs /// a bitwise OR operation on two instances of a /// ////// . /// /// public byte ByteValue { get { if (!IsNull) return (m_value == x_True) ? (byte)1 : (byte)0; else throw new SqlNullValueException(); } } ///[To be supplied.] ////// public override String ToString() { return IsNull ? SQLResource.NullString : Value.ToString((IFormatProvider)null); } ///[To be supplied.] ////// public static SqlBoolean Parse(String s) { if (null == s) // Let Boolean.Parse throw exception return new SqlBoolean(Boolean.Parse(s)); if (s == SQLResource.NullString) return SqlBoolean.Null; s = s.TrimStart(); char wchFirst = s[0]; if (Char.IsNumber(wchFirst) || ('-' == wchFirst) || ('+' == wchFirst)) { return new SqlBoolean(Int32.Parse(s, (IFormatProvider)null)); } else { return new SqlBoolean(Boolean.Parse(s)); } } // Unary operators ///[To be supplied.] ////// public static SqlBoolean operator ~(SqlBoolean x) { return (!x); } // Binary operators ///[To be supplied.] ////// public static SqlBoolean operator ^(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? Null : new SqlBoolean(x.m_value != y.m_value); } // Implicit conversions // Explicit conversions // Explicit conversion from SqlByte to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlByte x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt16 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt16 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt32 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt32 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlInt64 to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlInt64 x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0); } // Explicit conversion from SqlDouble to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlDouble x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0.0); } // Explicit conversion from SqlSingle to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlSingle x) { return x.IsNull ? Null : new SqlBoolean(x.Value != 0.0); } // Explicit conversion from SqlMoney to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlMoney x) { return x.IsNull ? Null : (x != SqlMoney.Zero); } // Explicit conversion from SqlDecimal to SqlBoolean ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlDecimal x) { return x.IsNull ? SqlBoolean.Null : new SqlBoolean(x.m_data1 != 0 || x.m_data2 != 0 || x.m_data3 != 0 || x.m_data4 != 0); } // Explicit conversion from SqlString to SqlBoolean // Throws FormatException or OverflowException if necessary. ///[To be supplied.] ////// public static explicit operator SqlBoolean(SqlString x) { return x.IsNull ? Null : SqlBoolean.Parse(x.Value); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value == y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlBoolean x, SqlBoolean y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value < y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value > y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value <= y.m_value); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlBoolean x, SqlBoolean y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(x.m_value >= y.m_value); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator ~ public static SqlBoolean OnesComplement(SqlBoolean x) { return ~x; } // Alternative method for operator & public static SqlBoolean And(SqlBoolean x, SqlBoolean y) { return x & y; } // Alternative method for operator | public static SqlBoolean Or(SqlBoolean x, SqlBoolean y) { return x | y; } // Alternative method for operator ^ public static SqlBoolean Xor(SqlBoolean x, SqlBoolean y) { return x ^ y; } // Alternative method for operator == public static SqlBoolean Equals(SqlBoolean x, SqlBoolean y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlBoolean x, SqlBoolean y) { return (x != y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlBoolean x, SqlBoolean y) { return (x > y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlBoolean x, SqlBoolean y) { return (x < y); } // Alternative method for operator <= public static SqlBoolean GreaterThanOrEquals(SqlBoolean x, SqlBoolean y) { return (x >= y); } // Alternative method for operator != public static SqlBoolean LessThanOrEquals(SqlBoolean x, SqlBoolean y) { return (x <= y); } // Alternative method for conversions. public SqlByte ToSqlByte() { return (SqlByte)this; } public SqlDouble ToSqlDouble() { return (SqlDouble)this; } public SqlInt16 ToSqlInt16() { return (SqlInt16)this; } public SqlInt32 ToSqlInt32() { return (SqlInt32)this; } public SqlInt64 ToSqlInt64() { return (SqlInt64)this; } public SqlMoney ToSqlMoney() { return (SqlMoney)this; } public SqlDecimal ToSqlDecimal() { return (SqlDecimal)this; } public SqlSingle ToSqlSingle() { return (SqlSingle)this; } public SqlString ToSqlString() { return (SqlString)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlBoolean) { SqlBoolean i = (SqlBoolean)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlBoolean)); } public int CompareTo(SqlBoolean value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this.ByteValue < value.ByteValue) return -1; if (this.ByteValue > value.ByteValue) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlBoolean)) { return false; } SqlBoolean i = (SqlBoolean)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_value = x_Null; } else { m_value = (byte)(XmlConvert.ToBoolean(reader.ReadElementString()) ? x_True : x_False); } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(m_value == x_True ? "true" : "false"); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("boolean", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlBoolean True = new SqlBoolean(true); ////// Represents a true value that can be assigned to the /// ///property of an instance of /// the class. /// /// public static readonly SqlBoolean False = new SqlBoolean(false); ////// Represents a false value that can be assigned to the /// ///property of an instance of /// the class. /// /// public static readonly SqlBoolean Null = new SqlBoolean(0, true); ////// Represents a null value that can be assigned to the ///property of an instance of /// the class. /// /// public static readonly SqlBoolean Zero = new SqlBoolean(0); ///[To be supplied.] ////// public static readonly SqlBoolean One = new SqlBoolean(1); } // SqlBoolean } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
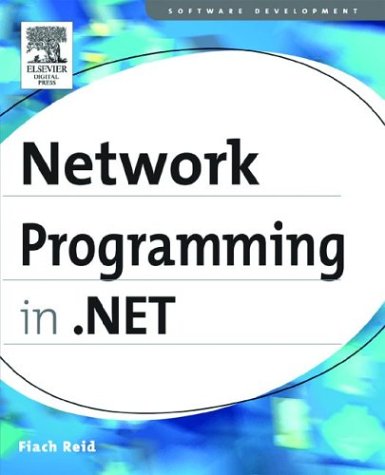
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionPoint.cs
- InkCanvasFeedbackAdorner.cs
- TreeNodeBindingDepthConverter.cs
- CodeExporter.cs
- UpdateTracker.cs
- NeutralResourcesLanguageAttribute.cs
- DataColumn.cs
- ProfileBuildProvider.cs
- BrowserInteropHelper.cs
- ShaderEffect.cs
- StickyNoteContentControl.cs
- TextTreeTextNode.cs
- HtmlWindowCollection.cs
- Pkcs7Recipient.cs
- LinearGradientBrush.cs
- Int16.cs
- RangeValuePattern.cs
- ArglessEventHandlerProxy.cs
- CheckBoxFlatAdapter.cs
- SafeNativeMethods.cs
- FixedNode.cs
- WorkflowHostingResponseContext.cs
- BamlLocalizableResource.cs
- DataBoundControl.cs
- SendActivityValidator.cs
- DeclaredTypeElementCollection.cs
- AbstractExpressions.cs
- SessionStateSection.cs
- XamlToRtfParser.cs
- xsdvalidator.cs
- MgmtConfigurationRecord.cs
- CancellationScope.cs
- TextProviderWrapper.cs
- PhysicalFontFamily.cs
- TextFormatterHost.cs
- DivideByZeroException.cs
- WindowsAltTab.cs
- ProjectionPathSegment.cs
- RequestNavigateEventArgs.cs
- DateTimeOffsetAdapter.cs
- CalendarDateRangeChangingEventArgs.cs
- WindowsTab.cs
- BoundPropertyEntry.cs
- ExitEventArgs.cs
- ReadWriteObjectLock.cs
- TableItemStyle.cs
- DbConnectionClosed.cs
- IISMapPath.cs
- MenuDesigner.cs
- OraclePermissionAttribute.cs
- assemblycache.cs
- FloaterParagraph.cs
- InputLangChangeRequestEvent.cs
- Sql8ExpressionRewriter.cs
- ItemContainerGenerator.cs
- MenuCommandService.cs
- FieldMetadata.cs
- NavigationWindowAutomationPeer.cs
- AppDomain.cs
- DataGridPagingPage.cs
- ScrollProperties.cs
- DocumentAutomationPeer.cs
- AnnotationHelper.cs
- ArrayTypeMismatchException.cs
- GridItemProviderWrapper.cs
- NotCondition.cs
- LoadWorkflowByKeyAsyncResult.cs
- GeneralTransform.cs
- UnsafeNativeMethods.cs
- RelationshipDetailsCollection.cs
- TypeConverterMarkupExtension.cs
- DataRowView.cs
- PrivateFontCollection.cs
- LogicalTreeHelper.cs
- HttpPostedFileBase.cs
- TextUtf8RawTextWriter.cs
- DataGridCaption.cs
- NumericUpDownAcceleration.cs
- ResourcePermissionBaseEntry.cs
- PartialCachingAttribute.cs
- IisTraceListener.cs
- EventsTab.cs
- TimeSpanSecondsConverter.cs
- DesignerActionMethodItem.cs
- AnimationTimeline.cs
- WebPartCatalogAddVerb.cs
- BaseResourcesBuildProvider.cs
- ExpandedWrapper.cs
- UseLicense.cs
- SchemaManager.cs
- AssemblyName.cs
- MappingSource.cs
- HtmlTableCell.cs
- ECDiffieHellman.cs
- XmlILAnnotation.cs
- NamespaceMapping.cs
- QilXmlWriter.cs
- OleAutBinder.cs
- StylusCollection.cs
- PropertyRef.cs