Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintingPermission.cs / 1 / PrintingPermission.cs
/* * Copyright (c) 2000 Microsoft Corporation. All Rights Reserved. * Microsoft Confidential. */ namespace System.Drawing.Printing { using System; using System.Security; using System.Security.Permissions; using System.IO; using System.Runtime.Serialization; using System.Reflection; using System.Collections; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [Serializable] public sealed class PrintingPermission : CodeAccessPermission, IUnrestrictedPermission { private PrintingPermissionLevel printingLevel; ///Controls the ability to use the printer. This class cannot be inherited. ////// /// public PrintingPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { printingLevel = PrintingPermissionLevel.AllPrinting; } else if (state == PermissionState.None) { printingLevel = PrintingPermissionLevel.NoPrinting; } else { throw new ArgumentException(SR.GetString(SR.InvalidPermissionState)); } } ///Initializes a new instance of the PrintingPermission class with either fully restricted /// or unrestricted access, as specified. ////// /// public PrintingPermission(PrintingPermissionLevel printingLevel) { VerifyPrintingLevel(printingLevel); this.printingLevel = printingLevel; } ///[To be supplied.] ////// /// public PrintingPermissionLevel Level { get { return printingLevel; } set { VerifyPrintingLevel(value); printingLevel = value; } } private static void VerifyPrintingLevel(PrintingPermissionLevel level) { if (level < PrintingPermissionLevel.NoPrinting || level > PrintingPermissionLevel.AllPrinting) { throw new ArgumentException(SR.GetString(SR.InvalidPermissionLevel)); } } //------------------------------------------------------ // // CODEACCESSPERMISSION IMPLEMENTATION // //----------------------------------------------------- ///[To be supplied.] ////// /// public bool IsUnrestricted() { return printingLevel == PrintingPermissionLevel.AllPrinting; } //----------------------------------------------------- // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- ///Gets a /// value indicating whether permission is unrestricted. ////// /// public override bool IsSubsetOf(IPermission target) { if (target == null) { return printingLevel == PrintingPermissionLevel.NoPrinting; } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } return this.printingLevel <= operand.printingLevel; } ///Determines whether the current permission object is a subset of /// the specified permission. ////// /// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } PrintingPermissionLevel isectLevels = printingLevel < operand.printingLevel ? printingLevel : operand.printingLevel; if (isectLevels == PrintingPermissionLevel.NoPrinting) return null; else return new PrintingPermission(isectLevels); } ///Creates and returns a permission that is the intersection of the current /// permission object and a target permission object. ////// /// public override IPermission Union(IPermission target) { if (target == null) { return this.Copy(); } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } PrintingPermissionLevel isectLevels = printingLevel > operand.printingLevel ? printingLevel : operand.printingLevel; if (isectLevels == PrintingPermissionLevel.NoPrinting) return null; else return new PrintingPermission(isectLevels); } ///Creates a permission that is the union of the permission object /// and the target parameter permission object. ////// /// [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] public override IPermission Copy() { return new PrintingPermission(this.printingLevel); } ///Creates and returns an identical copy of the current permission /// object. ////// /// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace('\"', '\'')); securityElement.AddAttribute("version", "1"); if (!IsUnrestricted()) { securityElement.AddAttribute("Level", Enum.GetName(typeof(PrintingPermissionLevel), printingLevel)); } else { securityElement.AddAttribute("Unrestricted", "true"); } return securityElement; } ///Creates an XML encoding of the security object and its current /// state. ////// /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public override void FromXml(SecurityElement esd) { if (esd == null) { throw new ArgumentNullException("esd"); } String className = esd.Attribute("class"); if (className == null || className.IndexOf(this.GetType().FullName) == -1) { throw new ArgumentException(SR.GetString(SR.InvalidClassName)); } String unrestricted = esd.Attribute("Unrestricted"); if (unrestricted != null && String.Equals(unrestricted, "true", StringComparison.OrdinalIgnoreCase)) { printingLevel = PrintingPermissionLevel.AllPrinting; return; } printingLevel = PrintingPermissionLevel.NoPrinting; String printing = esd.Attribute("Level"); if (printing != null) { printingLevel = (PrintingPermissionLevel)Enum.Parse(typeof(PrintingPermissionLevel), printing); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* * Copyright (c) 2000 Microsoft Corporation. All Rights Reserved. * Microsoft Confidential. */ namespace System.Drawing.Printing { using System; using System.Security; using System.Security.Permissions; using System.IO; using System.Runtime.Serialization; using System.Reflection; using System.Collections; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///Reconstructs a security object with a specified state from an XML /// encoding. ////// /// [Serializable] public sealed class PrintingPermission : CodeAccessPermission, IUnrestrictedPermission { private PrintingPermissionLevel printingLevel; ///Controls the ability to use the printer. This class cannot be inherited. ////// /// public PrintingPermission(PermissionState state) { if (state == PermissionState.Unrestricted) { printingLevel = PrintingPermissionLevel.AllPrinting; } else if (state == PermissionState.None) { printingLevel = PrintingPermissionLevel.NoPrinting; } else { throw new ArgumentException(SR.GetString(SR.InvalidPermissionState)); } } ///Initializes a new instance of the PrintingPermission class with either fully restricted /// or unrestricted access, as specified. ////// /// public PrintingPermission(PrintingPermissionLevel printingLevel) { VerifyPrintingLevel(printingLevel); this.printingLevel = printingLevel; } ///[To be supplied.] ////// /// public PrintingPermissionLevel Level { get { return printingLevel; } set { VerifyPrintingLevel(value); printingLevel = value; } } private static void VerifyPrintingLevel(PrintingPermissionLevel level) { if (level < PrintingPermissionLevel.NoPrinting || level > PrintingPermissionLevel.AllPrinting) { throw new ArgumentException(SR.GetString(SR.InvalidPermissionLevel)); } } //------------------------------------------------------ // // CODEACCESSPERMISSION IMPLEMENTATION // //----------------------------------------------------- ///[To be supplied.] ////// /// public bool IsUnrestricted() { return printingLevel == PrintingPermissionLevel.AllPrinting; } //----------------------------------------------------- // // IPERMISSION IMPLEMENTATION // //----------------------------------------------------- ///Gets a /// value indicating whether permission is unrestricted. ////// /// public override bool IsSubsetOf(IPermission target) { if (target == null) { return printingLevel == PrintingPermissionLevel.NoPrinting; } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } return this.printingLevel <= operand.printingLevel; } ///Determines whether the current permission object is a subset of /// the specified permission. ////// /// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } PrintingPermissionLevel isectLevels = printingLevel < operand.printingLevel ? printingLevel : operand.printingLevel; if (isectLevels == PrintingPermissionLevel.NoPrinting) return null; else return new PrintingPermission(isectLevels); } ///Creates and returns a permission that is the intersection of the current /// permission object and a target permission object. ////// /// public override IPermission Union(IPermission target) { if (target == null) { return this.Copy(); } PrintingPermission operand = target as PrintingPermission; if(operand == null) { throw new ArgumentException(SR.GetString(SR.TargetNotPrintingPermission)); } PrintingPermissionLevel isectLevels = printingLevel > operand.printingLevel ? printingLevel : operand.printingLevel; if (isectLevels == PrintingPermissionLevel.NoPrinting) return null; else return new PrintingPermission(isectLevels); } ///Creates a permission that is the union of the permission object /// and the target parameter permission object. ////// /// [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] public override IPermission Copy() { return new PrintingPermission(this.printingLevel); } ///Creates and returns an identical copy of the current permission /// object. ////// /// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace('\"', '\'')); securityElement.AddAttribute("version", "1"); if (!IsUnrestricted()) { securityElement.AddAttribute("Level", Enum.GetName(typeof(PrintingPermissionLevel), printingLevel)); } else { securityElement.AddAttribute("Unrestricted", "true"); } return securityElement; } ///Creates an XML encoding of the security object and its current /// state. ////// /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public override void FromXml(SecurityElement esd) { if (esd == null) { throw new ArgumentNullException("esd"); } String className = esd.Attribute("class"); if (className == null || className.IndexOf(this.GetType().FullName) == -1) { throw new ArgumentException(SR.GetString(SR.InvalidClassName)); } String unrestricted = esd.Attribute("Unrestricted"); if (unrestricted != null && String.Equals(unrestricted, "true", StringComparison.OrdinalIgnoreCase)) { printingLevel = PrintingPermissionLevel.AllPrinting; return; } printingLevel = PrintingPermissionLevel.NoPrinting; String printing = esd.Attribute("Level"); if (printing != null) { printingLevel = (PrintingPermissionLevel)Enum.Parse(typeof(PrintingPermissionLevel), printing); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Reconstructs a security object with a specified state from an XML /// encoding. ///
Link Menu
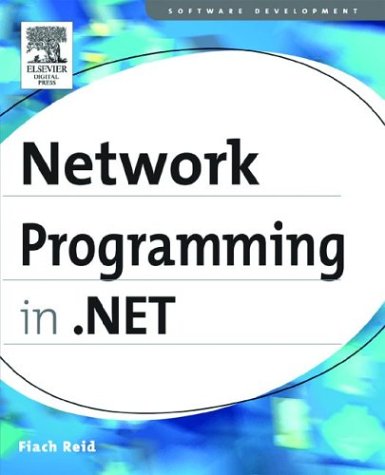
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- String.cs
- BinaryConverter.cs
- Enlistment.cs
- ThicknessAnimationUsingKeyFrames.cs
- _OverlappedAsyncResult.cs
- PrimaryKeyTypeConverter.cs
- XmlSchemaAnnotation.cs
- SkinBuilder.cs
- RewritingPass.cs
- XmlDocumentType.cs
- SequentialUshortCollection.cs
- _CommandStream.cs
- ColumnCollection.cs
- ReachSerializationUtils.cs
- OperandQuery.cs
- ListViewAutomationPeer.cs
- InvalidEnumArgumentException.cs
- EntityDataSourceDesignerHelper.cs
- IteratorAsyncResult.cs
- ZipIOFileItemStream.cs
- GenericWebPart.cs
- bidPrivateBase.cs
- StaticFileHandler.cs
- SingleConverter.cs
- ConfigurationValidatorBase.cs
- WebResourceAttribute.cs
- NextPreviousPagerField.cs
- UInt64Converter.cs
- ProxyWebPart.cs
- GenericTypeParameterConverter.cs
- MultiBindingExpression.cs
- Compiler.cs
- EDesignUtil.cs
- TextComposition.cs
- ProgressPage.cs
- Configuration.cs
- StateMachine.cs
- FieldMetadata.cs
- ItemDragEvent.cs
- SmtpFailedRecipientException.cs
- TableItemPatternIdentifiers.cs
- RequestCachingSection.cs
- StorageScalarPropertyMapping.cs
- GridView.cs
- FtpWebRequest.cs
- BuildProviderCollection.cs
- SqlCacheDependencySection.cs
- RelatedEnd.cs
- DependencyObject.cs
- CodeTypeDeclaration.cs
- DataTableTypeConverter.cs
- Site.cs
- DependencyPropertyConverter.cs
- Lease.cs
- UnsafeNativeMethods.cs
- CaretElement.cs
- InheritanceAttribute.cs
- WebPartAddingEventArgs.cs
- ItemsChangedEventArgs.cs
- DataGridRow.cs
- ResourceAttributes.cs
- DesignerActionTextItem.cs
- RenderDataDrawingContext.cs
- ObjectListCommandsPage.cs
- PeerNearMe.cs
- Normalization.cs
- LabelExpression.cs
- SqlDataSourceView.cs
- DbConnectionFactory.cs
- SpnegoTokenAuthenticator.cs
- StringPropertyBuilder.cs
- CodeTypeConstructor.cs
- LiteralControl.cs
- ReachSerializerAsync.cs
- ImageButton.cs
- TypeConverterHelper.cs
- XmlnsDictionary.cs
- CircleHotSpot.cs
- ProcessHostFactoryHelper.cs
- Path.cs
- EntityTypeEmitter.cs
- SharedPerformanceCounter.cs
- StagingAreaInputItem.cs
- SqlWorkflowInstanceStoreLock.cs
- EnumMember.cs
- CodeArrayIndexerExpression.cs
- MenuItem.cs
- ImageButton.cs
- HtmlShim.cs
- HtmlSelect.cs
- XmlSchemaSimpleTypeUnion.cs
- WebEventTraceProvider.cs
- Merger.cs
- InternalDispatchObject.cs
- InvokerUtil.cs
- KeyTime.cs
- OutputCacheSettings.cs
- WebBrowserDocumentCompletedEventHandler.cs
- FixUp.cs
- NetTcpBindingElement.cs