Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / InputGestureCollection.cs / 1305600 / InputGestureCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: InputGestureCollection serves the purpose of Storing/Retrieving InputGestures // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // // History: // 03/30/2004 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Collections.Generic; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// InputGestureCollection /// InputGestureCollection - Collection of InputGestures. /// Stores the InputGestures sequentially in a genric InputGesture List /// Will be changed to generic List implementation once the /// parser supports generic collections. /// public sealed class InputGestureCollection : IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public InputGestureCollection() { } ////// InputGestureCollection /// /// InputGesture array public InputGestureCollection( IList inputGestures ) { if (inputGestures != null && inputGestures.Count > 0) { this.AddRange(inputGestures as ICollection); } } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region Implementation of IList #region Implementation of ICollection ////// CopyTo - to copy the entire collection into an array /// /// /// void ICollection.CopyTo(System.Array array, int index) { if (_innerGestureList != null) ((ICollection)_innerGestureList).CopyTo(array, index); } #endregion Implementation of ICollection ////// IList.Contains /// /// key ///true - if found, false - otherwise bool IList.Contains(object key) { return this.Contains(key as InputGesture) ; } ////// IndexOf /// /// ///int IList.IndexOf(object value) { InputGesture inputGesture = value as InputGesture; return ((inputGesture != null) ? this.IndexOf(inputGesture) : -1) ; } /// /// Insert /// /// index at which to insert the item /// item value to insert void IList.Insert(int index, object value) { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); this.Insert(index, value as InputGesture); } ////// Add /// /// int IList.Add(object inputGesture) { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); return this.Add(inputGesture as InputGesture); } ////// Remove /// /// void IList.Remove(object inputGesture) { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); this.Remove(inputGesture as InputGesture); } ////// Indexing operator /// object IList.this[int index] { get { return this[index]; } set { InputGesture inputGesture = value as InputGesture; if (inputGesture == null) throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputGestures)); this[index] = inputGesture; } } #endregion Implementation of IList #region Implementation of Enumerable ////// IEnumerable.GetEnumerator - For Enumeration purposes /// ///public IEnumerator GetEnumerator() { if (_innerGestureList != null) return _innerGestureList.GetEnumerator(); List list = new List (0); return list.GetEnumerator(); } #endregion Implementation of IEnumberable /// /// Indexing operator /// public InputGesture this[int index] { get { return (_innerGestureList != null ? _innerGestureList[index] : null); } set { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); EnsureList(); if (_innerGestureList != null) { _innerGestureList[index] = value; } } } ////// ICollection.IsSynchronized /// public bool IsSynchronized { get { if (_innerGestureList != null) return ((IList)_innerGestureList).IsSynchronized; return false; } } ////// ICollection.SyncRoot /// public object SyncRoot { get { return _innerGestureList != null ? ((IList)_innerGestureList).SyncRoot : this; } } ////// IndexOf /// /// ///public int IndexOf(InputGesture value) { return (_innerGestureList != null) ? _innerGestureList.IndexOf(value) : -1; } /// /// RemoveAt - Removes the item at given index /// /// index at which item needs to be removed public void RemoveAt(int index) { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); if (_innerGestureList != null) _innerGestureList.RemoveAt(index); } ////// IsFixedSize - Fixed Capacity if ReadOnly, else false. /// public bool IsFixedSize { get { return IsReadOnly; } } ////// Add /// /// public int Add(InputGesture inputGesture) { if (IsReadOnly) { throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); } if (inputGesture == null) { throw new ArgumentNullException("inputGesture"); } EnsureList(); _innerGestureList.Add(inputGesture); return 0; // ICollection.Add no longer returns the indice } ////// Adds the elements of the given collection to the end of this list. If /// required, the capacity of the list is increased to twice the previous /// capacity or the new size, whichever is larger. /// /// collection to append public void AddRange(ICollection collection) { if (IsReadOnly) { throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); } if (collection == null) throw new ArgumentNullException("collection"); if( collection.Count > 0) { if (_innerGestureList == null) _innerGestureList = new System.Collections.Generic.List(collection.Count); IEnumerator collectionEnum = collection.GetEnumerator(); while(collectionEnum.MoveNext()) { InputGesture inputGesture = collectionEnum.Current as InputGesture; if (inputGesture != null) { _innerGestureList.Add(inputGesture); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputGestures)); } } } } /// /// Insert /// /// index at which to insert the item /// item value to insert public void Insert(int index, InputGesture inputGesture) { if (IsReadOnly) throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); if (inputGesture == null) throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputGestures)); if (_innerGestureList != null) _innerGestureList.Insert(index, inputGesture); } ////// IsReadOnly - Tells whether this is readonly Collection. /// public bool IsReadOnly { get { return (_isReadOnly); } } ////// Remove /// /// public void Remove(InputGesture inputGesture) { if (IsReadOnly) { throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); } if (inputGesture == null) { throw new ArgumentNullException("inputGesture"); } if (_innerGestureList != null && _innerGestureList.Contains(inputGesture)) { _innerGestureList.Remove(inputGesture as InputGesture); } } ////// Count /// public int Count { get { return (_innerGestureList != null ? _innerGestureList.Count : 0 ); } } ////// Clears the Entire InputGestureCollection /// public void Clear() { if (IsReadOnly) { throw new NotSupportedException(SR.Get(SRID.ReadOnlyInputGesturesCollection)); } if (_innerGestureList != null) { _innerGestureList.Clear(); _innerGestureList = null; } } ////// Contains /// /// key ///true - if found, false - otherwise public bool Contains(InputGesture key) { if (_innerGestureList != null && key != null) { return _innerGestureList.Contains(key) ; } return false; } ////// CopyTo - to copy the collection starting from given index into an array /// /// Array of InputGesture /// start index of items to copy public void CopyTo(InputGesture[] inputGestures, int index) { if (_innerGestureList != null) _innerGestureList.CopyTo(inputGestures, index); } ////// Seal the Collection by setting it as read-only. /// public void Seal() { _isReadOnly = true; } private void EnsureList() { if (_innerGestureList == null) _innerGestureList = new List(1); } #endregion Public //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Internal internal InputGesture FindMatch(object targetElement, InputEventArgs inputEventArgs) { for (int i = 0; i < Count; i++) { InputGesture inputGesture = this[i]; if (inputGesture.Matches(targetElement, inputEventArgs)) { return inputGesture; } } return null; } #endregion //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private System.Collections.Generic.List _innerGestureList; private bool _isReadOnly = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
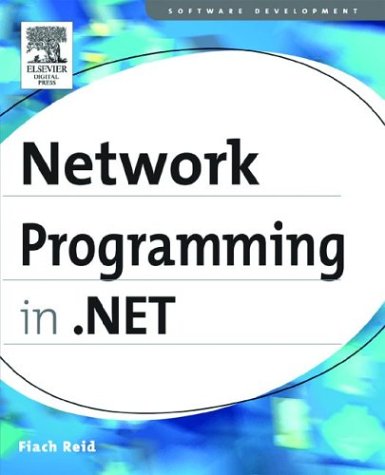
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Interlocked.cs
- MatrixTransform.cs
- BitmapEffectGroup.cs
- Polyline.cs
- RootProfilePropertySettingsCollection.cs
- DataTemplateSelector.cs
- sqlinternaltransaction.cs
- PropertyMapper.cs
- FixedPosition.cs
- Inline.cs
- HtmlEmptyTagControlBuilder.cs
- StateMachine.cs
- ObjectSpanRewriter.cs
- ScrollBar.cs
- ArithmeticException.cs
- PassportAuthentication.cs
- OperationContext.cs
- Point4DValueSerializer.cs
- SoapClientMessage.cs
- ParameterCollectionEditorForm.cs
- DispatchWrapper.cs
- DataRelationPropertyDescriptor.cs
- PerformanceCounterCategory.cs
- TraceContext.cs
- NetworkAddressChange.cs
- Timer.cs
- ResourceKey.cs
- PropertyGridCommands.cs
- Convert.cs
- DocumentViewerAutomationPeer.cs
- EditingCommands.cs
- Panel.cs
- SqlProcedureAttribute.cs
- ConfigurationSectionCollection.cs
- DataMisalignedException.cs
- EncoderExceptionFallback.cs
- Select.cs
- TransformCollection.cs
- SerializationAttributes.cs
- PersonalizationEntry.cs
- NativeMethods.cs
- Int32Rect.cs
- CodeSnippetTypeMember.cs
- LocatorGroup.cs
- Models.cs
- TemplateColumn.cs
- XslException.cs
- TemplateBaseAction.cs
- Validator.cs
- Stroke2.cs
- WebSysDisplayNameAttribute.cs
- SplitterPanelDesigner.cs
- Types.cs
- HtmlTable.cs
- Selection.cs
- MILUtilities.cs
- SystemTcpStatistics.cs
- ZipFileInfo.cs
- datacache.cs
- ObjectDataSourceFilteringEventArgs.cs
- InplaceBitmapMetadataWriter.cs
- StructuralCache.cs
- MDIClient.cs
- ToolboxComponentsCreatedEventArgs.cs
- DiscoveryClientReferences.cs
- ThousandthOfEmRealPoints.cs
- ProjectionCamera.cs
- PreservationFileWriter.cs
- ServerValidateEventArgs.cs
- VectorCollection.cs
- ImageCollectionEditor.cs
- LinkTarget.cs
- XamlClipboardData.cs
- NodeInfo.cs
- GridViewUpdatedEventArgs.cs
- DataServiceProcessingPipeline.cs
- LinqDataView.cs
- UiaCoreProviderApi.cs
- XmlSchemaExternal.cs
- ElementMarkupObject.cs
- WebPartHeaderCloseVerb.cs
- CustomAttributeBuilder.cs
- ListItemConverter.cs
- RoutingTable.cs
- ButtonBaseAutomationPeer.cs
- DisplayNameAttribute.cs
- HttpHandlerActionCollection.cs
- DisplayInformation.cs
- ComboBoxAutomationPeer.cs
- PassportPrincipal.cs
- EventProviderWriter.cs
- SqlSupersetValidator.cs
- CommandID.cs
- TreeBuilderXamlTranslator.cs
- SafeMILHandle.cs
- PeerApplicationLaunchInfo.cs
- MenuItem.cs
- EditorZone.cs
- DesignerForm.cs
- EventSchemaTraceListener.cs